1. Talk about the experience of String Utils in org. apache. commons. Lang
- StringUtils.isEmpty(String str)
- StringUtils.isBlank(String str)
- str == null && str.length==0
- """""""""""""""""and so on (i.e. space characters)
- "t", "f", "r", "n"//tab, line break, page change, carriage return.
NumberUtils.toInt (AppResource.resource.getString ("SLEEP_INTERVAL_TIME"), can avoid getting a given default value if it is empty: 10, will not throw an exception
<?xml version="1.0" encoding="UTF-8" ?> <configuration debug="true"> <property name="logs" value="logs" /> <logger name="com.alibaba.jstorm.daemon.worker.metrics" level="ERROR"/> <logger name="com.alibaba.jstorm.task.heartbeat" level="ERROR"/> <logger name="com.alibaba.jstorm.daemon.worker.hearbeat" level="ERROR"/> <logger name="com.alibaba.jstorm.metric" level="ERROR"/> <!--TRACE<DEBUG<INFO<WARN<ERROR--> <appender name="info" class="ch.qos.logback.core.rolling.RollingFileAppender"> <File>${logs}/datapreparation-ng.log</File> <encoder class="ch.qos.logback.classic.encoder.PatternLayoutEncoder"> <!--Format output:%d Indicates the date.%thread Represents the thread name.%-5level: Level shows 5 character widths from left%msg: Log messages,%n Newline character--> <pattern>%d{yyyy-MM-dd HH:mm:ss.SSS} [%thread] %-5level %logger{0} - %msg%n</pattern> </encoder> <filter class="ch.qos.logback.classic.filter.ThresholdFilter"> <level>INFO</level> </filter> <rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy"> <FileNamePattern>${logs}/datapreparation-ng.%d{yyyy-MM-dd}.log</FileNamePattern> <maxHistory>7</maxHistory> </rollingPolicy> </appender> <appender name="error" class="ch.qos.logback.core.rolling.RollingFileAppender"> <File>${logs}/datapreparation-ng_error.log</File> <encoder class="ch.qos.logback.classic.encoder.PatternLayoutEncoder"> <!--Format output:%d Indicates the date.%thread Represents the thread name.%-5level: Level shows 5 character widths from left%msg: Log messages,%n Newline character--> <pattern>%d{yyyy-MM-dd HH:mm:ss.SSS} [%thread] %logger{0} - %msg%n</pattern> </encoder> <filter class="ch.qos.logback.classic.filter.ThresholdFilter"> <level>ERROR</level> </filter> <rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy"> <FileNamePattern>${logs}/datapreparation-ng.%d{yyyy-MM-dd}.log</FileNamePattern> <maxHistory>7</maxHistory> </rollingPolicy> </appender> <appender name="console" class="ch.qos.logback.core.ConsoleAppender"> <encoder class="ch.qos.logback.classic.encoder.PatternLayoutEncoder"> <!--Format output:%d Indicates the date.%thread Represents the thread name.%-5level: Level shows 5 character widths from left%msg: Log messages,%n Newline character--> <pattern>%d{yyyy-MM-dd HH:mm:ss.SSS} [%thread] %logger{0} - %msg%n</pattern> </encoder> </appender> <root level="INFO"> <appender-ref ref="info"/> <appender-ref ref="error"/> <appender-ref ref="console"/> </root> </configuration>
4. How to empty StringBuilder content
Because StringBuilder does not provide clear or empty methods, it needs to take other methods to deal with it. Specifically, I summarize the following three ways:
1) Create a new one, and the old one is automatically recycled by the system. 2) Use delete 3) Use setLength
Three methods are used for 10 million cycles of stress testing:
Way1=9438ms
Way2=6281ms Optimal
Way3=6469ms
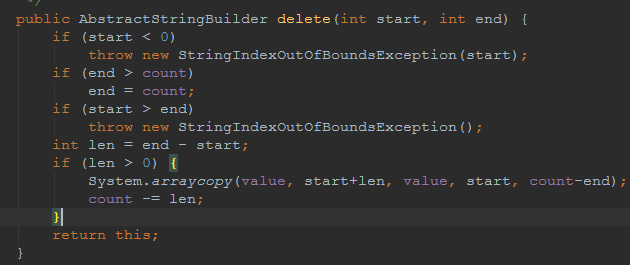
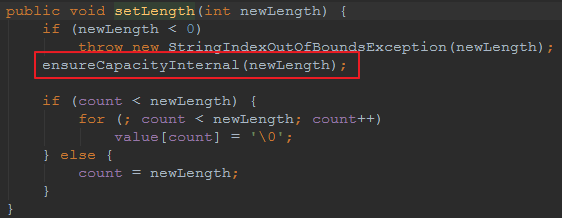
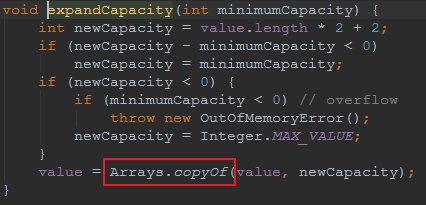
StringUtils.removeEnd(sortBuilder.toString(), ",")
Or when you add commas, decide if you need to add:
1 Iterator<String> iterator = result.iterator(); //result Could be list,set 2 StringBuilder collectionSb = new StringBuilder(); 3 int index = 0; 4 while (iterator.hasNext()) { 5 if (index > 0) { 6 collectionSb.append(","); 7 } 8 String collectionName = iterator.next(); 9 collectionSb.append(collectionName); 10 index++; 11 }
6. Digital comparison
Do not use X-Y for similar comparisons, because the number may be very large and opposite symbols, which may go beyond the scope. The following methods are recommended:
Integer.compare(int x, int y)
See the source code as follows:
1 public static int compare(int x, int y) { 2 return (x < y) ? -1 : ((x == y) ? 0 : 1); 3 }
Similarly, there are objects corresponding to basic data types such as Double and Float.
Method 1:
Utilizing the Array class that comes with JDK
String str = "a,b,c";
List<String> result = Arrays.asList(str.split(","));
Method 2:
Utilizing the Splitter of Guava
String str = "a, b, c";
List<String> result = Splitter.on(",").trimResults().splitToList(str);
Method 3:
StringUtils using Apache Commons (split only)
String str = "a,b,c";
List<String> result = Arrays.asList(StringUtils.split(str,","));
StringUtils Using Spring Framework
String str = "a,b,c";
List<String> str = Arrays.asList(StringUtils.commaDelimitedListToStringArray(str));
8. List converts to a comma-delimited string
Method 1:
Using JDK (there seems to be no good way to do this, but it needs to be implemented step by step)
NA
Method 2:
Joiner using Guava
List<String> list = new ArrayList<String>(); list.add("a"); list.add("b"); list.add("c"); String str = Joiner.on(",").join(list);
Method 3:
StringUtils with Apache Commons
List<String> list = new ArrayList<String>(); list.add("a"); list.add("b"); list.add("c"); String str = StringUtils.join(list.toArray(), ",");
Method 4:
String Utils Using Spring Framework
List<String> list = new ArrayList<String>(); list.add("a"); list.add("b"); list.add("c"); String str = StringUtils.collectionToDelimitedString(list, ",");
In comparison, my point of view is that Guava libraries are more flexible and applicable. If Guava is not introduced into the project, add it.