JAVA Learning Notes--First Identity Container Class Library
Keywords:
Java
JDK
less
Eclipse
1. Preface
Since everything in JAVA is an object, holding objects is particularly important.
In JAVA, we can hold objects by creating a reference to them:
HoldingObject holding;
You can also create an array of objects to hold a specific set of objects:
HoldingObject holding = new HoldingObject[10];
However, an object reference can only hold one object, and an object array can only hold a fixed number of objects of a certain type; however, we often encounter this situation, when writing a program, we do not know how many objects to create, or even the type of object to create, becauseIn this case, we need to be able to create any number of objects at any time and anywhere, which is not possible with the above two methods.The JAVA utility class library provides a fairly complete set of container classes to solve this problem. The basic types are List,Set,Queue,Map, which we call containers.
Classification of containers
As shown in the figure above, it is a simple container classification.As you can see, there are actually only two containers, Collection and Map, and Collection can be divided into List,Set, and Queue.They have two or three implementations, for example, ArrayList,LinkedList is the implementation of List, HashMap,TreeMap is the implementation of Map.In the figure above, commonly used containers are represented by thick black wireframes; point wireframes represent interfaces; solid wireframes represent specific classes; hollow arrows point lines indicate that a particular class implements an interface; solid arrows indicate that a class can generate objects of the class to which the arrows point.*
1)Collection:
Collection maintains a separate sequence of elements that obey one or more rules.Collection is an interface that represents a set of objects, also known as collections'elements. Some collections allow duplicate elements, while others do not.JDK does not provide any direct implementation of this interface; it provides more specific subinterface implementations (List, Set, Queue), which are often used to pass collections and operate on them where maximum universality is required.Here is a brief list of some of Collection's methods (screenshots from J2SE6_API):
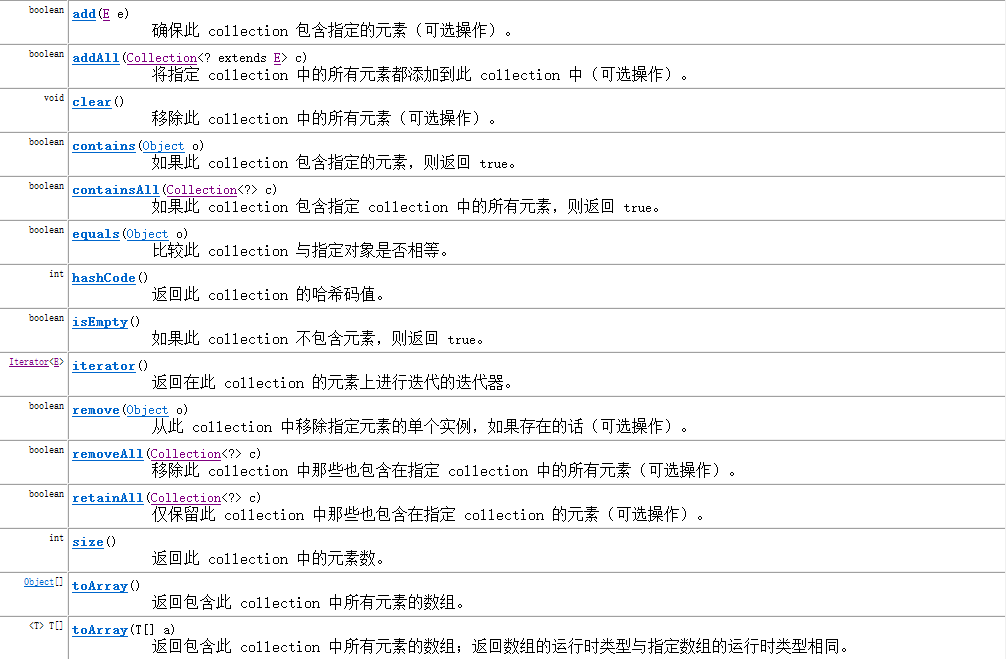
a) List: elements must be saved in the order they are inserted
A List is an ordered collection, where users have precise control over where each element in the list is inserted. Users can access elements based on their integer index (position in the list) and search for elements in the list.The List interface adds a number of methods to the Collection, enabling elements to be inserted and removed from the middle of the List.Lists allow elements to be added, removed, or self-resized after they are created, which is exactly what they are worth: a modifiable sequence.There are two types of List implementations:
ArrayList: Random access to elements is faster, but inserting and removing elements is slower;
ArrayList has three constructions:
ArrayList(); // Construct an empty list with an initial capacity of 10
ArrayList(int x); // Construct an initial capacity of x Empty List
ArrayList(Collection<? extends E> c); // Construct a containing specification collection List of elements of the collection Iterators of
The following is a simple example of how ArrayList works:

package com.tongye.holding;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class HoldObject {
public static void main(String[] args){
Random rand = new Random(47);
Circle circle = new Circle();
Square square = new Square();
Triangle triangle = new Triangle();
Rectangle rectangle = new Rectangle();
// Establish List Example
List<Integer> list1 = new ArrayList<Integer>(); // Create a Storage Only int List of type objects
List<Shape> list2 = new ArrayList<Shape>(); // Create a Storage Only Shape List of type objects
List list3 = new ArrayList(4); // Create a list with an initial capacity of 4 without specifying the type of storage
// To List list1 Add elements to
for(int i = 0;i < 10;i++)
list1.add(rand.nextInt(20)); // add(E e)Method that adds the specified element to the end of this list
for(Integer i : list1) // Be-all collection All available foreach Traverse
System.out.print(i + " ");
System.out.println("");
// use subList Method intercept part list1 View
List<Integer> list4 = list1.subList(3, 8); // subList(int fromIndex,int toIndex),Return to List
// from fromIndex(Include) to toIndex(Exclude) Partial views between
for(Integer i : list4)
System.out.print(i + " ");
System.out.println("");
// To List list2 Add elements to
list2.add(circle); // Add an object to the list
list2.add(square);
list2.add(triangle);
list2.add(1,rectangle); // add(int index,E e)Method to insert a specified element to a specified location in the list
for(Shape i : list2) // Be-all collection All available foreach Traverse
System.out.println(i);
System.out.println(list2.isEmpty()); // isEmpty()Method, checks if there are elements in the list, returns if there are no elements true
System.out.println(list2.contains(circle)); // contains()Method checks to see if the list contains the specified element, or returns true
System.out.println(list2.indexOf(square)); // indexOf()Method that returns the index number of an element in the list
list2.get(1).showShape();
list2.remove(rectangle); // remove(Object o)Method to delete the specified element that first appears in the list
list2.get(1).showShape();
list2.remove(1);
list2.get(1).showShape(); // remove(int index)Method to delete the element in the list at the specified index
// towards list3 Add elements to
list3.add(100);
list3.add("Add a string to the list");
list3.add(rectangle);
list3.add(1.048596);
list3.add("Automatic Capacity Growth");
for(int i = 0;i < 5;i++)
System.out.println(list3.get(i));
}
}
class Shape{
public void showShape(){
System.out.println("Shape class");
}
}
class Circle extends Shape{
public void showShape(){
System.out.println("Circle class");
}
}
class Square extends Shape{
public void showShape(){
System.out.println("Square class");
}
}
class Triangle extends Shape{
public void showShape(){
System.out.println("Triangle class");
}
}
class Rectangle extends Shape{
public void showShape(){
System.out.println("Rectangle class");
}
}
ArrayList Code
LinkedList: Provides optimized sequential access through cheaper insert and delete operations in the middle of the List.LinkedList also implements the basic List interface like ArrayList, but it performs some operations more efficiently (inserts and deletes in lists) than ArrayList, but less efficiently than random access operations.If we need to use LinkedList, we only need to modify the implementation of List in the program, which is also a benefit of using the interface:
List <Integer> list = new LinkedList <Integer>(); implement List with LinkedList
b) Set: No duplicate elements
Set is a collection that does not contain duplicate elements. More precisely, Set does not contain elements satisfying e1.equals(e2) for E1 and e2, and contains at most one null element. Set has exactly the same interface as Collection, so there is no additional functionality. Set is actually a Collection, but behaves differently; it has three implementations: HashSet, TreeSet, LinkedHashSet.
①,HashSet:
HashSet is a set implementation based on HashMap, which does not guarantee the iteration order of the set, especially that the order is not permanent;
HashSet allows null elements;
HashSet provides stability (add, remove, contain, size) for basic operations;
The time required for HashSet iteration is proportional to the size of the HashSet instance (number of elements) and the "capacity" of the underlying HashMap instance, so if iteration performance is important, don't say that the initial capacity is set too high (or the load factor is set too low);
HashSet is not synchronous. When accessing a HashSet in multiple threads at the same time, a synchronization statement is required to lock it. You can wrap the set using the Collection.synchronizedSet method, preferably at creation time, while making unexpected asynchronous access to the set: Set s = Collection s.synchronizedSet.New HashSet ();
The iterator returned by HashSet's iterator method fails quickly: if a set is modified after it is created, the Iteractor will throw an exception at any time and in any way unless it is modified by the iterator's own remove method.
HashSet has four construction methods:
HashSet(); // Create an empty set,Its underlying layer HashMap The default initial capacity of the instance is 16, and the load factor is 0.75
HashSet(Collection<? extends E> c); // Create one containing the specified collection New for elements in set
HashSet(int intialCapacity); // Create one with specified initial capacity and default load factor set
HashSet(int intialCapacity,float loadFactor); // Create one with specified initial capacity and load factor set
②,TreeSet:
TreeSet is a set implementation based on TreeMap;
TreeSet provides guaranteed time overhead (add, remove, contain) for basic operations;
The TreeSet uses the natural order of elements to sort them, or according to the Comparator provided when the element was created, depending on the construction method used;
TreeSet is not synchronous;
The iterator returned by TreeSet's iterator method failed quickly;
There are four ways to construct a TreeSet:
TreeSet(); // Create a new empty set,this set Sort according to the natural order of its elements
TreeSet(Collection<? extends E> c); // Create one containing the specified collection New elements TreeSet,It is sorted in the natural order of its elements
TreeSet(Compartor<? super E> compartor); // Create a new empty TreeSet,It is sorted according to the specified comparer
TreeSet(SortedSet<E> s); // Create an order with the specified set New with same mapping relationship and same sort TreeSet
③,LinkedHashSet:
LinkedHashSet is a hash table and link list implementation of set interface with predictable iteration order;
LinkedHashSet defines the order of iteration, that is, the order in which elements are inserted into the set, which is not affected by the elements that are re-inserted in the set.
The time required for LinkedHashSet iteration is proportional to the size of the set and independent of capacity;
LinkedHashSet provides all optional set operations and allows null elements; provides stable performance for basic operations; is out of sync; iterators fail quickly
It is constructed similarly to HashSet.
Here is a simple example of how Set works:

package com.tongye.holding;
import java.util.Random;
import java.util.Set;
import java.util.HashSet;
import java.util.TreeSet;
public class SetExample {
public static void main(String[] args){
Random rand = new Random(47);
Set<Integer> hashSetInt = new HashSet<Integer>();
for(int i = 0;i < 100;i++)
hashSetInt.add(rand.nextInt(20));
System.out.println(hashSetInt);
Set<Integer> treeSetInt = new TreeSet<Integer>();
for(int i = 0;i < 100;i++)
treeSetInt.add(rand.nextInt(20));
System.out.println(treeSetInt);
}
}
Set Code
Note: Actually, when I run the above code on eclipse, I find that elements of the output are arranged in natural order whether HashSet or TreeSet. Looking at the data, I find that this may be the reason of JDK version. There are some changes in HashMap implementation in JDK8. These new features cause HashSet to input integer data in accordance with theArrange in natural order.
c) Queue: Queue rules determine the order in which objects are generated (usually in the same order in which they are inserted)
Queue is a typical first-in-first-out container with two implementations of LinkedList and PriorityQueue.LinkedList provides a method to support the behavior of the queue and implements the Queue interface; PriorityQueue declares that the next pop-up element is the most needed element (with the highest priority).
2) Map: Saves a set of paired Key-Value Pair objects, allowing the use of keys to find values
Map can be used to map one object to another.The Map interface provides three collection views that allow you to view a mapped relationship as a keyset, a value set, or a key-value mapping relationship set.The mapping order is the order in which the iterator returns its elements on the mapping collection view.It has two implementations: HashMap and TreeMap.
A Hashtable-based Map implementation that provides all optional mapping operations and allows the use of null values and null keys; the order of mappings is not guaranteed, especially if it is not guaranteed to remain constant.
Map implementations based on red and black trees that sort by the natural order of their keys or by the Compartor provided when the map was created, depending on the construction method used.