The programming interface Canvas API with HTML5 canvas element has a very broad application prospect. Simply put, the canvas element can create a rectangular area in a web page, which can be called a canvas, where various graphics can be drawn. <canvas> </canvas> is HTML5 Like all dom objects, new tags have their own attributes, methods and events. Among them, there are drawing methods, which can be called by js for drawing. .
1. Add canvas elements to the page
If you want to add canvas elements to the page, you can use the following code. By default, the rectangular area created by Canvas is 300 PX wide and 150 PX high. Width and height attributes can also be used to customize its width and height.
The above code simply creates a Canvas object that will not show anything on the page opened in the browser. You can set the border style for canvas by using the border attribute. The code is as follows:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="200"></canvas> </body> </html>
The results are as follows:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="200" style="border: 2px solid blue"></canvas> </body> </html>
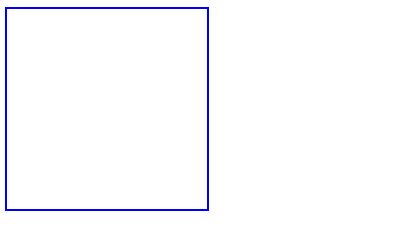
2. How Canvas Draws Graphics
Before we know how Canvas draws graphics, we have a preliminary understanding of several ranking concepts.
1) Context: context is an object that encapsulates many drawing functions. The way to get this object is to
var context = canvas. getContext ("2d"); and //2d means that html5 currently supports only 2d, but does not provide 3d display.
2) There are two ways to draw images with canvas elements, one is to draw images with canvas elements, the other is to draw images with canvas elements.
context.fill()// Fill
context.stroke()// Draw borders
3) Style: Before drawing, set the drawing style.
context.fillStyle// Filling Style
context.strokeStyle//Border Style
4) The expression of color:
Use color names directly: "red", "green" and "blue"
Hexadecimal color value: " EEEEFF"
rgb(1-255,1-255,1-255)
rgba(1-255,1-255,1-255, 1-255, transparency)
Step 1: To add the canvas element to the HTML5 page, you must define the id attribute value of the canvas element for subsequent invocation.
Step 2: Use id to find canvas elements. Canvas elements can be found in JavaScript through the document.getElementById() method.<canvas id="myCanvas" width="200" height="200" style="border: 2px solid blue"></canvas>
Step 3: Get the context through the getContext() method of the canvas element, that is, create the Context object to get the 2D environment allowed to draw.var myCanvas = document.getElementById("myCanvas");
Step 4: Draw graphics using JavaScript. For example, you can draw a rectangle in the center of the canvas with a bit of code.var cxt=canvas.getContext("2d");
Effect:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="100" style="border: 2px solid #34b1ff"></canvas> <script> var canvas = document.getElementById("myCanvas"); var cxt=canvas.getContext("2d"); cxt.fillStyle="#34b1ff"; cxt.fillRect(50,25,100,50); </script> </body> </html>
3. Check whether the browser supports
According to the definition on W3c, we can know that the current browser support of canvas is as follows:
4. Drawing Simple Graphicsvar canvas = document.getElementById("myCanvas"); if(canvas.getContext){ alert("Your browser support Canvas.") }else{ alert("Your browser does not support it Canvas.") }
We can see many special effects implemented by canvas, and the effect is very good. However, just as the so-called "Ten Thousand-Zhang Tall Building Begins on Flat Ground", all things need to be constructed from the simplest and most basic part. We start with the simplest straight line, rectangle and circle.
1) Drawing Lines
When drawing a straight line, three methods need to be called: moveTo(), lineTo(), stroke().
- The moveTo() method is used to create a new subpath and specifies its starting point as (x,y).
- The lineTo() method is used to draw a line with specified coordinates from the starting point specified by the moveTo () method. If the starting point of the subpath is not specified by the moveTo () method before, the lineTo() method is equivalent to the moveTo () method.
- stroke() method is used to draw a straight line along the path.
Effect:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="100" style="border: 2px solid #ff54c5"> //Your browser does not support it. </canvas> <script> var canvas = document.getElementById("myCanvas"); var cxt=canvas.getContext("2d"); cxt.strokeStyle="#ff54c5"; cxt.moveTo(0,0); cxt.lineTo(200,100); cxt.stroke(); </script> </body> </html>
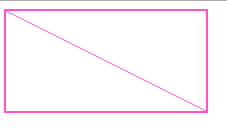
2) Drawing Rectangles
Effect:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="100" style="border: 2px solid #34b1ff"></canvas> <script> var canvas = document.getElementById("myCanvas"); var cxt=canvas.getContext("2d"); cxt.fillStyle="#34b1ff"; cxt.fillRect(0,0,100,50); </script> </body> </html>
Example 2 code is as follows (lines):
Effect:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="100" style="border: 2px solid #66d3ff"></canvas> <script> var canvas = document.getElementById("myCanvas"); var cxt=canvas.getContext("2d"); cxt.strokeStyle="#66d3ff"; cxt.strokeRect(0,0,100,50); </script> </body> </html>
3) Drawing a circle
Round drawing still uses the method of drawing paths and filling colors. Four key methods, beginPath(), arc(), closePath(), and fill(), are used in the process of drawing a circle.
- The beginPath() method is used to start drawing the path, and the closePath() method is used to end drawing the path. After calling the beginPath() method, a series of circles are drawn in Canvas. After drawing is completed, the closePath() method should be called to close the graph.
- arc() method is intended to draw arcs, when appropriate parameters are used, the circle can be drawn. In this method, the specific method is: arc (x,y, radius, start Angle, engAngle, anticlockwise), a total of six parameters, the parameters of x,y for the starting coordinates, radius for the radius of the circle, start Angle for the starting angle, end Angle for the end angle, anticlockwise for whether to draw clockwise direction.
Effect:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 canvas</title> </head> <body> <canvas id="myCanvas" width="200" height="200"></canvas> <script> var canvas = document.getElementById("myCanvas"); var cxt=canvas.getContext("2d"); cxt.fillStyle="#66d3ff"; cxt.beginPath(); cxt.arc(100,100,75,0,Math.PI*2,true); cxt.closePath(); cxt.fill(); </script> </body> </html>
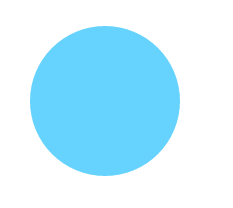