
Copyright Statement: This is an original article created by a blogger and may not be reproduced without the permission of the blogger.
Topic Review
This is an open question. Obviously, there is more than one answer. Check your resilience and come up with more solutions to get the interviewer's fancy.Here are three solutions.
Solution
1. map+area
- <img src="t.jpg" width="1366" height="768" border="0" usemap="#Map" />
- <map name="Map" id="Map">
- <area shape="circle" coords="821,289,68" href="www.baidu.com" target="_blank" />
- </map>
<img src="t.jpg" width="1366" height="768" border="0" usemap="#Map" /> <map name="Map" id="Map"> <area shape="circle" coords="821,289,68" href="www.baidu.com" target="_blank" /> </map>
Making hot spots with Dreamweaver becomes very easy and will eventually result in the code above, which you can refer to in video at http://www.chuanke.com/3885380-190205.html.
2. border-radius(H5)
- <style>
- .disc{
- width:100px;
- height:100px;
- background-color:dimgray;
- border-radius: 50%;
- cursor: pointer;
- position: absolute;
- left:50px;
- top:50px;
- line-height: 100px;
- text-align: center;
- color: white;
- }
- </style>
- <div class="disc">Smart without worry</div>
Running effect<style> .disc{ width:100px; height:100px; background-color:dimgray; border-radius: 50%; cursor: pointer; position: absolute; left:50px; top:50px; line-height: 100px; text-align: center; color: white; } </style> <div class="disc">Smart without worry</div>
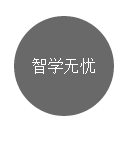
3. Pure js implementation
Requires a simple algorithm for finding a point on a circle, getting mouse coordinates, etc.
Formula for calculating the distance between two points
For JavaScript The code is as follows:

|AB|=Math.abs(Math.sqrt(Math.pow(X2-X1),2)+Math.pow(Y2-Y1,2)))
Math.abs() for absolute values
Math.pow (base, exponent)
Math.sqrt() for square root
Example:
Assuming the center of the circle is (100,100) and the radius is 50, click inside the circle to pop up the corresponding information, and display the information outside the circle that is not inside the circle.
- document.onclick=function(e){
- var r=50;//The radius of a circle
- var x1=100,y1=100,x2= e.clientX;y2= e.clientY;
- //Calculates the distance between the mouse point's position and the center of the circle
- var len=Math.abs(Math.sqrt(Math.pow(x2-x1,2)+Math.pow(y2-y1,2)));
- if(len<=50){
- console.log("within")
- }else{
- console.log("abroad")
- }
- }
document.onclick=function(e){ var r=50;//The radius of a circle var x1=100,y1=100,x2= e.clientX;y2= e.clientY; //Calculates the distance between the mouse point's position and the center of the circle var len=Math.abs(Math.sqrt(Math.pow(x2-x1,2)+Math.pow(y2-y1,2))); if(len<=50){ console.log("within") }else{ console.log("abroad") } }
- Previous Web Front End Interview Guide (41): What are the new features of html5 and what elements have been removed?
- Next Web Front End Interview Guide (43): Describe the differences between cookies, session store and local store?
- • Web Front End Interview Guide (41): What are the new features of html5 and what elements have been removed?
- • Web Front End Interview Guide (38): What are the ways js are delayed loading?
- • Web Front End Interview Guide (39): What exactly did the new operator do?
- • Web Front End Interview Guide (40): What are the new features of CSS3?
- • Web Front End Interview Guide (43): Describe the differences between cookies, session store and local store?
- • Responsive Layout of Several Layout Modes for web Front End Development
- • Web Front End Interview Guide (30): How many types of values does JavaScript have?Can you draw their memory maps?
- • Web Front End Interview Guide (17): How to design a full screen layout?
- • Talking about three ways to achieve a circular clickable area
- • Web front-end interview guide (5): Master interview skills to make interviews easier