Preface
float and double are designed for scientific calculation and engineering calculation. They provide fast and accurate calculations over a wide range of numerical values. However, they do not provide completely accurate results, so they can not be used in situations requiring accurate results. But commercial calculations require accurate results, and BigDecimal is needed at this time.
Let's start with a chestnut.
public static void main(String[] args){ System.out.println(0.2+0.1); System.out.println(0.3-0.1); System.out.println(0.2*0.1); System.out.println(0.3/0.1) }
The results are as follows:
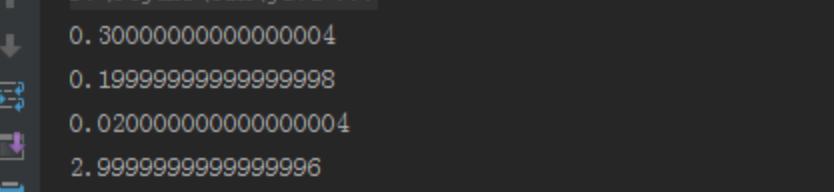
The reason for this result is that our computer is binary, and the calculation of floating point numbers usually loses some accuracy. For example, the binary representation of 2.4 is not the exact 2.4. On the contrary, the closest binary representation is 2.39999999.
In fact, java float can only be used for scientific or engineering calculation. In most commercial calculations, BigDecimal class is usually used for accurate calculation.
BigDecimal Construction Method
public BigDecimal(double val) converts the double representation to BigDecimal (not recommended) public BigDecimal(int val) converts int representation to BigDecimal public BigDecimal(String val) converts String representation to BigDecimal
The reason why the first method is not recommended is
public static void main(String[] args){ BigDecimal bDouble = new BigDecimal(2.3) System.out.println("bDouble="+bDouble) }
Operation result

Why did this happen?
JDK Description: The results of the construction method with parameter type double are somewhat unpredictable. One might think that the BigDecimal created by writing new BigDecimal (0.1) is exactly equal to 0.1, but it is actually equal to 0.1000000055555.
If the String construction method is completely predictable, writing new BigDecimal ("0.1") will create a BigDecimal, which is exactly equal to the expected 0.1.
When double must use BigDecimal's source, use Double.toString(double) to convert to String, then use String constructor, or use BigDecimal's static method valueOf.
BigDecimal bDouble = BigDecimal.value(2.3); BigDecimal bDouble = new BigDecimal(Double.toString(2.3));
BigDecimal addition, subtraction, multiplication and division operations
public BigDecimal add(BigDecimal value); //addition public BigDecimal subtract(BigDecimal value); //subtraction public BigDecimal multiply(BigDecimal value); //multiplication public BigDecimal divide(BigDecimal value); //division
The general usage is as follows
public static void main(String[] args){ BigDecimal a = new BigDecimal("4.5") BigDecimal a = new BigDecimal("1.5") System.out.println("a + b = " + a.add(b)) System.out.println("a - b = " + a.subtract(b)) System.out.println("a * b = " + a.multiply(b)) System.out.println("a / b = " + a.divide(b)) }
Operation result
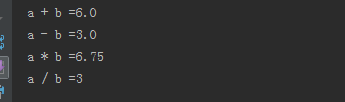
BigDecimal can also be used to intercept decimal digits, such as:
public static void main(String[] args){ BigDecimal a = new BigDecimal("4.5635"); a = a.setScale(3,RoundingMode.HALF_UP) System.out.println(a) }
The first parameter of the setScale function retains several decimal digits, and the second parameter is rounding mode, which contains the following
ROUND_CEILING //Rounding in a positive and infinite direction ROUND_DOWN //Round to zero ROUND_FLOOR //Rounding in the Negative Infinite Direction ROUND_HALF_DOWN //Round to the nearest side, unless both sides are equal. If so, round down. For example, 1.55 reserves a decimal result of 1.5. ROUND_HALF_EVEN //Round to the nearest side, unless both sides are equal. If so, if the number of reserved digits is odd, use ROUND_HALF_UP, if even, ROUND_HALF_DOWN. ROUND_HALF_UP //Round to the nearest side, unless both sides are equal. If so, round up. 1.55 reserves one decimal and results in 1.6. ROUND_UNNECESSARY //The results are accurate and do not require rounding mode. ROUND_UP //Round in the direction away from zero
It is also worth mentioning that the addition, subtraction, multiplication and division of BigDecimal returns a new BigDecimal object, which is immutable.
summary
1. Business computing uses BigDecimal
2. Use constructors with String as many parameters as possible
3. BigDecimal is immutable. Every step of operation produces a new object. Therefore, in addition, subtraction, multiplication and division, the value of the operation must be saved.
Last
In this interview season, I have collected a lot of information on the Internet and made documents and architectures for free to share with you [including advanced UI, performance optimization, architect courses, NDK, Kotlin, hybrid development (React Native + Weex), Flutter and other architectures technical information]. I hope to help you review before the interview and find a good job. It also saves time for people to search for information on the Internet.
Data acquisition method: join Android framework to exchange QQ group chat: 513088520, get information when you enter the group!!!
Click on the link to join the group chat [Android Mobile Architecture Group]: Join group chat
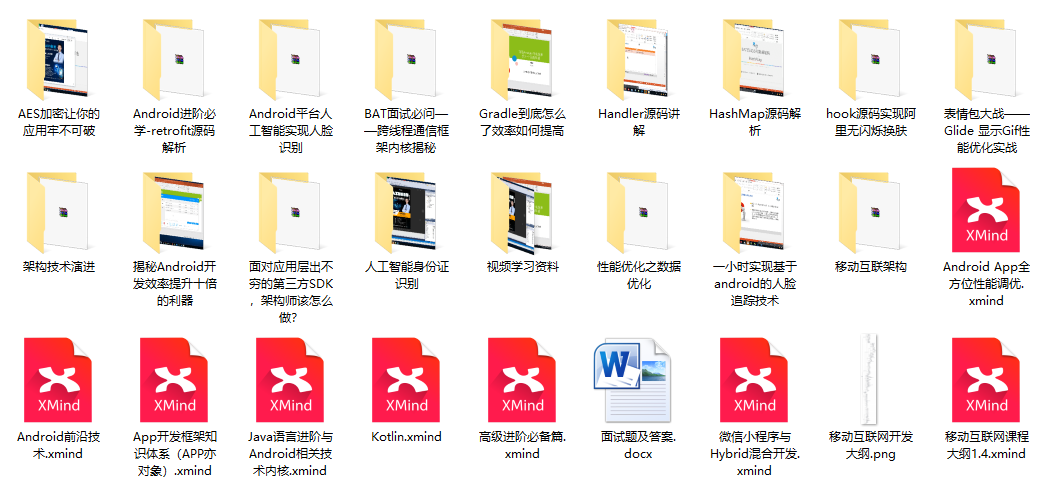