1.Servlet annotation and configuration file writing
1.1 writing method of configuration file [if Servlet version is low]
1. You need to write the configuration in the web.xml file
Find the resource web.xml
First, create a test class to inherit HttpServlet and override doGet and doPost methods
package com.javacoffee.test; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; public class Test extends HttpServlet { @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doGet(req, resp); } @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { System.out.println("This is XML Configuration file writing"); } }
Then find the web.xml file for configuration
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <servlet> <!-- yes servlet Give a name, which should be accompanied by a label servlet-mapping corresponding--> <servlet-name>Test</servlet-name> <!-- servlet-class yes servle Corresponding class--> <servlet-class>com.javacoffee.test.Test</servlet-class> <!-- Set to 1 when tomcat This class will be loaded when the server is started--> <load-on-startup>1</load-on-startup> </servlet> <!-- url-pattern Find the introduction to this resource, similar to what we wrote@WebServlet("/webxml") --> <!-- Can write multiple url-pattern It is equivalent to giving many names to resources But there is only one request<servlet-name> Test --> <servlet-mapping> <servlet-name>Test</servlet-name> <url-pattern>/Test</url-pattern> </servlet-mapping> </web-app>
1.2 notation [use this later]
Annotations can only be used when the Servlet version is above 3.0,
Now we are all above 3.0. So use the notation later. From this place can illustrate a problem
Go to the writing method of xml. xml is too troublesome. Most of our ssm frameworks are xml, and a springboot came out later
xml is omitted and a lot of annotations are added.
package com.javacoffee.test; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet("/Test") public class Test extends HttpServlet { @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doGet(req, resp); } @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { System.out.println("This is XML Annotation writing method"); } }
2.Servlet life cycle
When the Servlet is executed, it goes through these processes:
1.construct
2.init
3.service
4.destory
Phase 1:
Servlet is instantiated in Tomcat and provided to Tomcat for use
Phase 2:
Initialization of Servlet
Phase 3:
Servlet s provide external services, which is equivalent to doGet and doPost methods
Phase 4:
Servlets can be destroyed manually by calling the destroy method. Generally, we don't need to call it. tomcat will destroy the servlet as long as it is closed
3. Get the parameters of the front-end request
If you want to learn Servlet well, you must focus on two things
Request and response.
Ask you a question? The code we are writing now is to make a request and then respond to the client.
Did you bring data to the Servlet when you requested it? No data is brought to the Servlet
Now let's talk about the front end bringing data to the Servlet when requesting. Our Servlet needs to accept the data from the front end
First, create an html file under the web file and write the form in it
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Sign in</title> </head> <body> <form action="TestServlet" method="get"> user name:<input type="text" name="username"><br> password:<input type="password" name="password"><br> <input type="submit" name="Submit"> </form> </body> </html>
The result is this
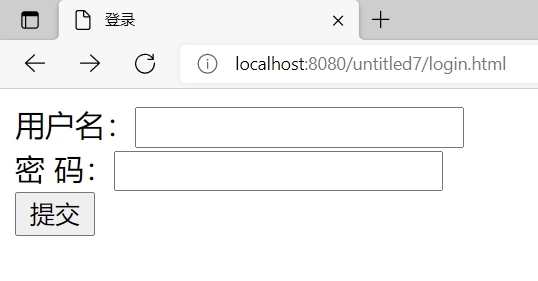
Next, create the TestServlet class
package com.javacoffee.servlet; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet("/TestServlet") public class TestServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request,response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //The front end sends data to my servlet. Can my servlet receive some data? //The front end sends the request, description and request object //http:localhost:8080/login.html?user=gouda&pwd=123456 //The getParameter method is used to obtain the parameters of the request object //Obtained from the attribute value of the front-end input tag name //Solve the garbled code in post request request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); String username = request.getParameter("username"); String password = request.getParameter("password"); //Output in TestServlet interface response.getWriter().println("full name:"+username+",password:"+password); } }
Because I use the get request method, I can see the user name and password in the url of the website
4. Solution to Chinese garbled code
Chinese garbled code during request:
The front end sends data to the Servlet If it is a post request, the data received by the Servlet is garbled when Chinese data is input.
request.setCharacterEncoding("utf-8");
Chinese garbled code in response:
When the Servlet responds to the data to the client, if it is in Chinese, it will be garbled
response.setContentType("text/html;charset=utf-8");
5. Redirection and forwarding [important]
There are some jump buttons on the web site. For example, after successful login, jump to the main page!!!
6.1 redirection
The user sends a request through the browser, and the Tomcat server receives the request and sends a status code 302 to the browser
And set a redirect path. If the browser receives the 302 status code, it will automatically load the path set by the server.
Jump from one page to another (scene)
The login page jumps to the home page
denglu.html===>TestLoginServlet=>target.html
features:
1. The redirection process is the behavior of the browser (client)
2. In fact, the browser made several requests (twice when clicking the login button) (TestServlet,loginSeccess.html)
3. Note that the request object of the last request will be lost
4. Redirection has a very obvious feature. The url of the browser has changed
5. Redirection can redirect to any resource on the network (such as JD Taobao and other network resources)
response.sendRedirect("target.html"); Just this line of code, but this line of code must be written in doget or dopost
The first is a landing page
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Sign in</title> </head> <body> <form action="TestServlet" method="post"> user name:<input type="text" name="username"><br> password:<input type="password" name="password"><br> <input type="submit" name="Submit"> </form> </body> </html>
Then the login success page
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Login success page</title> </head> <body> <div style="color: red; font-size: 30px">This is the redirect page!</div> </body> </html>
Then comes the TestServlet class
package com.javacoffee.servlet; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet("/TestServlet") public class TestServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request,response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //The front end sends data to my servlet. Can my servlet receive some data? //The front end sends the request, description and request object //http:localhost:8080/login.html?user=gouda&pwd=123456 //The getParameter method is used to obtain the parameters of the request object //Obtained from the attribute value of the front-end input tag name //Solve the garbled code in post request request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); //After logging in, jump to the home page //redirect //The data in the TestServlet cannot be passed to loginsecess.html response.sendRedirect("loginSeccess.html"); } }
6.2 forwarding
The user sends a request to the server
The server receives the current request
The internal method (forwarding) is invoked to process the request
Finally, send the response to the client
features:
1. Forwarding is the behavior of the server
2. The browser only acts once in that process
3. Forwarding can be in the request object with data
4. The URL will not change
5. Forwarding can only be carried out in the current project, not external resources
package com.javacoffee.servlet; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet("/TestServlet") public class TestServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request,response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); String username = request.getParameter("username"); String password = request.getParameter("password"); //Set some data for the current request object. The request object contains data request.setAttribute("username",username); /* It can be forwarded to other resources (another Servlet) through the request object * */ //Forwarding will bring the data in one servlet to another servlet request.getRequestDispatcher("TestServlet1").forward(request,response); } }
TestServlet1 class
package com.javacoffee.servlet; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet("/TestServlet1") public class TestServlet1 extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); //Accept data from TestServlet String username1 = (String) request.getAttribute("username"); //Displayed on the interface response.getWriter().append(username1); } }
6.3 comprehensive case (realize simple registration, login, modification and deletion interface)
Simple registration, login, deletion and modification page
Register and store data in the database
Click the login button (judge whether the data sent to me by the front end exists in the database) (through query)
If the user name and password are correct, I will let you log in and forward to the main page
Forward to home page
Then you can add or delete
Front end interface
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Registration interface</title> <style> body{ background-color: pink; } div{ position: absolute; top: 10px; left: 500px; width: 500px; height: 500px; background-color:darkkhaki; } </style> </head> <body> <div> <p style="font-size: 50px;color: red">Welcome to the registration interface!</p> <form action="RegServlet" method="post"> Please enter user name:<input type="text" name="username" id="input1"><br> Please input a password:<input type="password" name="password" id="input2"><br> <input type="submit" name="Confirm registration" style="width: 100px"> <input type="reset" name="empty" style="width: 100px"> <br> </form> </div> </body> </html>
<%-- Created by IntelliJ IDEA. User: 86156 Date: 2021/9/10 Time: 22:01 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Login success page</title> </head> <body> <div style="background-color: pink;font-size: 80px">congratulations<%=request.getAttribute("username")%>Registration succeeded!!!</div> <form action="Login.html" method="get"> <input type="submit" name="Go log in" value="Go log in!" style="width: 100px;height: 30px"> </form> </body> </html>
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Sign in</title> <style> body{ background-color: pink; } div{ position: absolute; top: 10px; left: 500px; } </style> </head> <body> <div> <p style="font-size: 50px;color: red;background-color: pink">Welcome to the login interface!</p> <form action="LoginServlet" method="post"> user name:<input type="text" name="username"><br> dense Code:<input type="password" name="pwd"><br> <input type="submit" name="Sign in"> </form> </div> </body> </html>
<%-- Created by IntelliJ IDEA. User: 86156 Date: 2021/9/10 Time: 22:09 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Login successful</title> </head> <body> <div > <p style="font-size: 50px">delete user</p> <form action="DeleteServlet" method="post"> User name of the user to delete <input type="text" name="username"><br> <input type="submit" name="delete" value="Delete!" style="width: 100px"> </form> </div> <br><br><br> <div > <p style="font-size: 50px">Modify user</p> <form action="UpdateServlet" method="post"> The user name of the user to modify <input type="text" name="lastUsername"><br> New user name: <input type="text" name="newUsername"><br> New password: <input type="password" name="newPassword"><br> <input type="submit" name="modify" value="Modify!" style="width: 100px"> </form> </div> </body> </html>
<%-- Created by IntelliJ IDEA. User: 86156 Date: 2021/9/10 Time: 22:45 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Delete succeeded</title> </head> <body> <form action="LoginSuccess.jsp" method="get"> <input type="submit" name="return" value="return" style="width: 100px;height: 50px"> </form> </body> </html>
<%-- Created by IntelliJ IDEA. User: 86156 Date: 2021/9/10 Time: 22:47 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Modified successfully</title> </head> <body> <form action="LoginSuccess.jsp" method="get"> <input type="submit" name="return" value="return" style="width: 100px;height: 50px"> </form> </body> </html>
I connect to the database through c3p0. How to connect? You can check my previous blog
The next step is to create a database table
The util layer uses its own encapsulated JdbcUtil BaseDao class to obtain addition, deletion, modification and query operations
package com.javacoffee.util; import com.mchange.v2.c3p0.ComboPooledDataSource; import java.io.FileInputStream; import java.io.IOException; import java.sql.*; import java.util.Properties; /** * 1.Complete the automatic loading of the driver * 2.Complete the necessary data processing URL user password * 3.Complete the connection method * 4.Complete the unified close method */ public class JdbcUtil { //Why? Is it static? Because getConnection is a static method, //Non static post member properties cannot be used in static methods private static ComboPooledDataSource pool = new ComboPooledDataSource(); //Simplify the writing of getConnection method //Encapsulate a static method to obtain the connection object public static Connection getConnection() { Connection connection = null; try { connection = pool.getConnection(); } catch (SQLException e) { e.printStackTrace(); } return connection; } //Now when encapsulating, I don't know which resource needs to be closed //Some need to close one, some need to close two, and some need to close three //Close a resource connection public static void close(Connection connection) { close(connection, null, null); } //Close the two resource connection statement s public static void close(Connection connection, Statement statement) { close(connection, statement, null); } //Close the connection statement resultset of the three resources public static void close(Connection connection, Statement statement, ResultSet resultSet) { if (connection != null) { try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } if (statement != null) { try { statement.close(); } catch (SQLException e) { e.printStackTrace(); } } if (resultSet != null) { try { resultSet.close(); } catch (SQLException e) { e.printStackTrace(); } } } }
package com.javacoffee.util; import org.apache.commons.beanutils.BeanUtils; import java.lang.reflect.InvocationTargetException; import java.sql.*; import java.util.ArrayList; import java.util.List; public class BaseDao { /** * Complete a unified method of addition, deletion and modification * The method name is update * Method requires parameters: * 1.String sql * 2.The parameter corresponding to the sql statement is required * The parameters are uncertain because you don't know the sql statement you write. How many parameters are required * Put an array in, Object [] because Object can store different types of data * 3.Return value * int Number of rows affected * */ public int update(String sql, Object[] parameters) throws SQLException { if (sql == null) { throw new SQLException("sql is null"); } //1. Get database connection Connection connection = JdbcUtil.getConnection(); //2. Get database preprocessing Porter object PreparedStatement preparedStatement = connection.prepareStatement(sql); //3. Now I don't know how many sql statements are there? //3. Get the parameter metadata object and the number of parameters //insert into work(name, age,info) values(?,?,?) int parameterCount = preparedStatement.getParameterMetaData().getParameterCount(); //4. What is in sql? Assign three? setObject three times two? setObject two for (int i = 1; i <= parameterCount; i++) { /* * String sql = "insert into work(name, age, info) values(?,?,?)"; Object[] objs = {"Yang Zi, "78," born in the police station "}; * * */ // preparedStatement.setObject(1, "Yang Zi"); // preparedStatement.setObject(2,78); // preparedStatement.setObject(3, "born police station"); preparedStatement.setObject(i, parameters[i-1]); } //5. Execute sql statement int i = preparedStatement.executeUpdate(); //6. Close resources JdbcUtil.close(connection, preparedStatement); //7. Return the number of affected rows return i; } /** * Complete unified query method * 1,You can query a piece of data * 2,You can query multiple pieces of data * 3.Query any type of data * Method analysis: * Method name: query * Parameters of the method: * 1.sql * 2.sql Placeholder, Object array used * 3.A third parameter is required * Let's think about a problem. For example, if the work table is queried, assign the data of the work 'table to the work entity class * Then query the person table. Let's assign the data in the person table to the person class. Is the assigned entity class fixed? * To make it universal, you need an Object. If it's an Object, you need to turn it strongly, so you're afraid of making mistakes * Use reflection!!! Use class < T > * Return value of method: * List<T> * * */ public <T> List<T> query(String sql, Object[] parameters, Class<T> cls) throws SQLException { if (sql == null || cls == null) { throw new NullPointerException(); } //1. Get the connection object Connection connection = JdbcUtil.getConnection(); //2. Preprocessing sql statement select* from work where id =? PreparedStatement preparedStatement = connection.prepareStatement(sql); //3. Process the number of "get parameters" in the sql statement int parameterCount = preparedStatement.getParameterMetaData().getParameterCount(); //4. Assign a value to //When will this for loop be executed for setObject? //select * from work where id = ? //Objects[] objs = {10,20} //Write a bit more robust to judge whether the number in the array is consistent with the sql parameters //Is it because the value in the array is just assigned to sql? if(parameters != null && parameterCount == parameters.length) { for (int i = 1; i <= parameterCount; i++) { preparedStatement.setObject(i, parameters[i - 1]); } } //5. Executing sql statements is different from adding, deleting and modifying, because a ResultSet object is returned during query ResultSet resultSet = preparedStatement.executeQuery(); //6. Prepare a List set to place objects, and put multiple objects or an object into this set //A question? Do you know which object it is? ArrayList<T> ts = new ArrayList<>(); //7. Obtain the metadata object of the result set, the name of the field and the number of columns (number of fields) to obtain the row data in the data table ResultSetMetaData metaData = resultSet.getMetaData(); //8. Get the number of fields int columnCount = metaData.getColumnCount(); //9. Traverse data while (resultSet.next()) {//Number of control rows //10. Obtain the Work.class object of a Class through the Class object // Person.class //Teacher.class // Is a Class object // Work work = new Work(); T t = null;//t is equivalent to a new object. For example, you pass Work.class //This t new work object //For example, you pass Person.class, and this t is the person object of new try { t = cls.getConstructor(null).newInstance(null); for (int i = 1; i <= columnCount; i++) {//Number of columns controlled //For example, the fields ID, name, age info are obtained through the for loop //11. Get the name of the field and the name of the database through the result set //When i=1 gets the id field and i=2 gets the name field String columnName = metaData.getColumnName(i);//The name of the field //12. Obtain the corresponding value through the field Object value = resultSet.getObject(columnName); //13. Save the value to the object and the object to the collection, but there is no object now //new Work(); / / it is equivalent to writing it dead, encapsulation and universality. //t.setId() / / now, start assigning values to objects. What should I do? //columnName is the name of the field, so it is required that the name of the field in the data table must be the same as //The member properties in the entity class are consistent. // //BeanUtils.setProperty(work, id, 1); //BeanUtils.setProperty(work, name, "dog egg"); BeanUtils.setProperty(t, columnName, value); } } catch (Exception e) { e.printStackTrace(); } //The first while loop assigns a value to t object, and the second while loop assigns a value to t again //14. Save this t into the list set ts.add(t); } //15.close JdbcUtil.close(connection, preparedStatement, resultSet); //16. Return value //If the length of the set is not equal to 0, it proves that there is data in the set and returns the whole set. //If the length of the collection is 0, it proves that there is no data in the collection and returns null return ts.size()!= 0 ? ts: null;//ternary operator } }
Dao layer
package com.javacoffee.dao; import com.javacoffee.entity.User; import com.javacoffee.util.BaseDao; import java.sql.SQLException; import java.util.List; public class UserDao extends BaseDao { public void regint(Object[] parameters) throws SQLException { String sql = "insert into user(username,password) values (?,?)"; super.update(sql,parameters); } public void delete(Object[] parameters) throws SQLException { String sql = "delete from user where username = ?"; super.update(sql,parameters); } public void update(Object[] parameters) throws SQLException { String sql = "update user set username = ?,password = ? where username = ?"; super.update(sql,parameters); } public List<User> login(Object[] parameters) throws SQLException, ClassNotFoundException { String sql = "select * from user where username = ? and password = ?"; return super.query(sql, parameters,User.class); } }
entity layer
package com.javacoffee.entity; public class User { private int id; private String username; private String password; public User(){} public User(int id,String name, String password) { this.id=id; this.username = name; this.password = password; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } @Override public String toString() { return "User{" + "name='" + username + '\'' + ", password='" + password + '\'' + '}'; } }
service layer
package com.javacoffee.servlet; import com.javacoffee.dao.UserDao; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.sql.SQLException; @WebServlet("/DeleteServlet") public class DeleteServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); String username = request.getParameter("username"); Object[] objects = {username}; UserDao userDao = new UserDao(); try { userDao.delete(objects); request.getRequestDispatcher("DeleteSuccess.jsp").forward(request,response); } catch (SQLException e) { e.printStackTrace(); } } }
package com.javacoffee.servlet; import com.javacoffee.dao.UserDao; import com.javacoffee.entity.User; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.sql.SQLException; import java.util.List; @WebServlet("/LoginServlet") public class LoginServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); String username = request.getParameter("username"); String pwd = request.getParameter("pwd"); Object[] objects = {username,pwd}; UserDao userDao = new UserDao(); try { List<User> login = userDao.login(objects); if (login != null){ request.getRequestDispatcher("/LoginSuccess.jsp").forward(request,response); }else { response.getWriter().append("Login failed!"); } } catch (SQLException | ClassNotFoundException e) { e.printStackTrace(); } } }
package com.javacoffee.servlet; import com.javacoffee.dao.UserDao; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.sql.SQLException; @WebServlet("/RegServlet") public class RegServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); String username = request.getParameter("username"); String password = request.getParameter("password"); Object[] objects = {username,password}; UserDao userDao = new UserDao(); try { userDao.regint(objects); } catch (SQLException e) { e.printStackTrace(); } request.setAttribute("username",username); request.getRequestDispatcher("/success.jsp").forward(request,response); } }
package com.javacoffee.servlet; import com.javacoffee.dao.UserDao; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.sql.SQLException; @WebServlet("/UpdateServlet") public class UpdateServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); response.setContentType("text/html;charset=utf-8"); String lastUsername = request.getParameter("lastUsername"); String newUsername = request.getParameter("newUsername"); String newPassword = request.getParameter("newPassword"); Object[] objects = {newUsername,newPassword,lastUsername}; UserDao userDao = new UserDao(); try { userDao.update(objects); request.getRequestDispatcher("UpdateSuccess.jsp").forward(request,response); } catch (SQLException e) { e.printStackTrace(); } } }
Effect picture: I just did it. There are many bug s that haven't been repaired, and the interface is ugly, ha ha ha