Java provides rich API s for users to develop quickly and efficiently. This blog will introduce the specific usage of String related classes in common classes, including String, StringBuffer, StringBuilder.
catalog:
☑ conversion of String to other types of data
☑ StringBuffer and StringBuilder
☑ String class
Properties of ▴ String
String class: represents a string. All string literals in Java programs, such as "abc," are implemented as instances of this class.
☄ String is a final class, representing immutable character sequence (immutability of string), which cannot be inherited.
☄ The string is a constant, represented by a double quotation mark ''. Their values cannot be changed after creation, that is, the operation of the calling method on the original string will create a new string without affecting the original string itself.
☄ The bottom layer of character content of String object is stored in a character array final char value [].
☄ String implements the Serializable interface: indicates that strings support serialization.
☄ String implements the compatible interface: it means that strings can compare sizes.
☄ A string is assigned a literal value (different from New), and the string value is declared in the string constant pool; a string is created by new String(), and the content is stored in the heap space.
☄ A string is assigned a literal value (different from New), and the string value is declared in the string constant pool; a string is created by new String(), and the content is stored in the heap space.
String underlying code:
public final class String implements java.io.Serializable, Comparable<String>, CharSequence { /** The value is used for character storage. */ private final char value[]; /** Cache the hash code for the string */ private int hash; // Default to 0 /** use serialVersionUID from JDK 1.0.2 for interoperability */ private static final long serialVersionUID = -6849794470754667710L; .... }
▴ creation of String object
Literal value assignment
Description: at this time, the string data is declared in the string constant pool in the method area
Assignment of the way to create an object (calling constructors of different strings)
Note: at this time, the string variable stores the address value, which is the corresponding address value of the data after opening the memory space in the heap space
☃ Null parameter constructor new String(); -- essentially: this.value = new char[0];
☃ String parameter constructor new String(String original); -- essentially: this.value = original.value ;
☃ char character array parameter constructor new String(String original); -- essentially: this.value = Arrays.copyOf(value, value.length);
...
public void testString2(){ //1. Literal value assignment String st1 = "hello world"; //2. How to create an object assignment (constructors of different strings) /*2-1: Null constructor new String(); Essentially this.value =New char [0]; defines a character array of length 0 */ String st2 = new String(); System.out.println("new String():" + st2 + "length:" + st2.length()); //new String():length:0 /*2-2: String String parameter constructor new String(String original); In essence this.value = original.value */ String st3 = new String("serializable"); // System.out.println("new String(String original):" + st3 + " length:"+ st3.length()); //new String(String original):serializable length:12 /*2-3: char Character array parameter constructor new String(String original); * Essentially this.value = Arrays.copyOf(value,value.length); */ String st4 = new String(new char[]{'s', 'y', 'n', 'c', 'h', 'r', 'o', 'n', 'i', 'z', 'e', 'd'}); System.out.println("new String(String original):" + st4 + " length:"+ st4.length()); //new String(String original):synchronized length:12 //Compare the difference between literal value assignment method and String constructor method in instantiating String String t1 = "Java"; String t2 = "Java"; String t3 = new String("Java"); String t4 = new String("Java"); // System.out.println("t1 == t2:" + (t1 == t2)); //true System.out.println("t3 == t4:" + (t3 == t4)); //false System.out.println("t1 == t3:" + (t1 == t3)); //false Person p1 = new Person(11,"Tom"); Person p2 = new Person(11,"Tom"); System.out.println("p1==p2:" + (p1 == p2)); //The false object is stored in the heap, and the address of the opened memory space is different System.out.println("p1.name==p2.name:" + (p1.name == p2.name)); //true, the name in the Person object is defined in literal form, so it is stored in the string constant pool in the method area } class Person{ int age; String name; public Person(int age, String name) { this.age = age; this.name = name; } }
Create String object memory Demo:
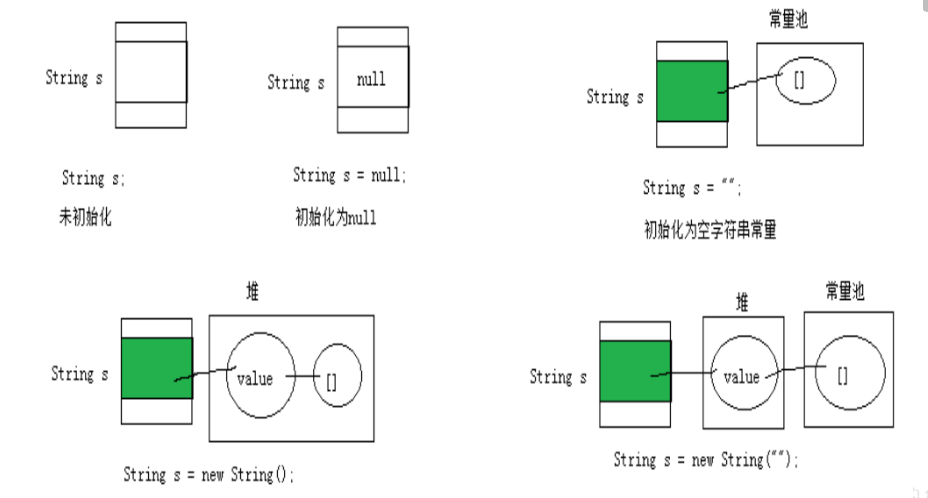

➹ thinking:
1. String s = new String("abc"); how many objects are created in memory?
Two: one is the new String() object, and the other is the data in the constant pool corresponding to char value []
✦ string constants are stored in
String constant pool for sharing
✦ string non constant object is stored in the heap (new String object and string object after operation)
Invariance of▴ String object
The string is immutable. Any operation on the string that causes the string to change must reassign the memory area. The original value [] cannot be used for assignment
➣ embodiment 2:1. When the string is reassigned, the value of the memory area needs to be reassigned, and the original value [] cannot be used for assignment
➣ embodiment 2: when splicing the existing strings, it is also necessary to re specify the memory area assignment, instead of using the original value [] assignment. The spliced strings are new strings
➣ embodiment 3: when the string specified character or string is modified by calling the string such as replace() method, the memory area assignment should also be specified again. The original value [] assignment cannot be used, and a new string is returned, and the original string remains unchanged
➣ embodiment 4: when other methods of String are called to operate the current String, a new String is returned, and the original String is unchanged
public void testString1(){ String s1 = "abc"; //Literal definition method String s2 = "abc"; //If the values of string variables are equal, they use the same address //Reason: the literal defined string value is declared in the string constant pool in the method area, and the string constant pool will not store the same string System.out.println(s1 == s2); //Compare address values of s1 and s2; true String st1 = new String("abc"); String st2 = new String("abc"); System.out.println(st1==st2); //Compare the address values of st1 and st2: false System.out.println(st1==s1); //Compare the address values of st1 and s1: false System.out.println("-----------"); //String invariance in splicing String s3 = "abc"; s3 += "def"; System.out.println(s3); //abcdef System.out.println(s2); //Invariant abc System.out.println("-----------"); //Invariance when calling String method to manipulate String String s4 = "abc"; String s5 = s4.replace("c","def"); //s4.replace('c','d'); System.out.println(s4); //Invariance: abc System.out.println(s5); //abdef }
▴ splicing of String objects
/* Conclusion: 1,The splicing result of constant and constant is in constant pool, and there will be no constant with the same content in constant pool 2,As long as one of the string splices is a variable (not final), the result is in the heap 3,If the concatenated result calls the intern() method, the return value is in the constant pool */ public void test3(){ String s1 = "java"; String s2 = "web"; String s3 = "javaweb"; String s4 = "java" + "web"; String s5 = s1 + "web"; String s6 = "java" + s2; String s7 = s1 + s2; System.out.println("Output 1:" + (s3==s4)); //true System.out.println("Output 3:" + (s3==s6)); //false System.out.println("Output 4:" + (s5==s6)); //false System.out.println("Output 5:" + (s3==s7)); //false System.out.println("Output 6:" + (s4==s7)); //false System.out.println("Output 7:" + (s5==s7)); //false //Because s4 is a literal value assigned to s4 after two strings are spliced, it exists in the string constant pool, which is consistent with s3 address, // While s5-s7 is the splicing of string / character variable + character variable, its storage location is in the heap, and the storage address changes String s8 = s5.intern(); //At this time, the return value s8 uses the value that already exists in the constant System.out.println("Output 8:" + (s3 == s8)); //true }
▴ trap in use of String
➣ String s1 = "a";
Description: a string with literal "a" is created in the string constant pool.
➣ s1 = s1 + "b";
Note: in fact, the original "a" string object has been discarded, and now a character is generated in the heap space
String s1 + "b" (that is, "ab"). If you perform these operations to change the contents of the string multiple times, you will result in a large number of copies
String objects remain in memory, reducing efficiency. If such an operation is placed in a loop, it will greatly affect
Performance of the program.
➣ String s2 = "ab";s2 = "abc";
Create a string with literal "ab" directly in the string constant pool, discard the original string "ab" object after assigning value, re create a string with literal "abc" in the constant pool and assign address to s2.
➣ String s3 = "a" + "b";
Note: s3 points to the string of "ab" that has been created in the string constant pool.
➣ String s4 = s1.intern();
Note: after the s1 object of heap space calls intern(), it will assign the existing "ab" string in constant pool to s4. If not, it will create a new string in constant pool.
example:
The results of the following programs:
public class StringTest { String str = new String("good"); char[] ch = { 't', 'e', 's', 't' }; public void change(String str,String string, char ch[]){ System.out.println(this.string == string); //true this.str = "awesome"; string = "love"; ch[0] = 'b'; } public static void main(String[] args) { StringTest st = new StringTest(); st.change(st.str, st.string, st.ch); System.out.println(st.str); //test member variable assignment System.out.println(st.string); //The invariance of lucky string is to assign values to parameter variables System.out.println(st.ch); //best } }
The results show that:
public class StringTest { String str = new String("good"); String string = new String("lucky"); char []ch = {'t','e','s','t'}; public void change(String str,String string, char ch[]){ System.out.println(this.string == string); //true this.str = "awesome"; string = "love"; //Parameter string literal value assignment, content exists in constant pool ch[0] = 'b'; System.out.println("Inside:"+string); //love System.out.println(this.string == string); //false String s = "love"; System.out.println(string == s); //True string and s are in the same constant pool memory System.out.println("---------------------------"); /*Note: for String string, it is similar to: String s1 = new String("lucky"); //Corresponding member variable this.string , content in heap space String s2 = s1; //The corresponding parameter string points to the same heap memory address s2 = "love"; //Literal value assignment, content in constant pool This is a special case of String, because its literal value assignment is different from the new content storage location, This is not the case for other ch [] in this case. Their values are in the heap space */ } public static void main(String[] args) { StringTest st = new StringTest(); st.change(st.str, st.string, st.ch); System.out.println(st.str); //Member variable assignment System.out.println(st.string); //lucky is to assign a literal value to a parameter variable System.out.println(st.ch); //best } } /*Output: true Inside: love false true --------------------------- awesome lucky best */
☑ conversion of String to other types of data
▾ conversion between string and basic data type, wrapper class
String ➟ basic data type, wrapper class
➣ public static int parseInt(String s) of Integer wrapper class: string composed of "number" characters can be converted to Integer type.
➣ similarly, use java.lang The Byte, Short, Long, Float and Double classes in the package correspond to each other
The string composed of "number" characters can be converted to the corresponding basic data type.
Basic data type, wrapper class ➟ string
➣ call public String valueOf(int n) of String class to convert int type to String.
➣ corresponding valueOf(byte b), valueOf(long l), valueOf(float f), valueOf(double)
d) , valueOf(boolean b) can be converted from the corresponding type of the parameter to the string.
/** * @Description: * Conversion between basic data type and wrapper class: the basic data type wrapper class to be converted. Parsexxx (string string string) * 1,string-->Basic data type wrapper class.parseXxx(String string) * 2,Basic data type -- > string String.valueOf (basic data type) */ public void stingAndBaseNum(){ String s1 = "123"; //String to basic data type wrapper class.parseXxx(String string) int num1 = Integer.parseInt(s1); System.out.println(num1); double d1 = Double.parseDouble(s1); System.out.println(d1); //2. To convert a wrapper class to a string string: String.valueOf (packaging object) int i1 = 2020; char c1 = 'in'; System.out.println(String.valueOf(i1)); System.out.println(String.valueOf(c1)); System.out.println((s1+"")==(String.valueOf(123)));//false }
▾ String and character array conversion
Character array ➟ string
➣ constructors of String class: String(char []) and String(char [], int offset, int
length) creates a string object with all and part of the characters in the character array, respectively.
String ➟ character array
➣ public char[] toCharArray(): a method to store all characters in a string in a character array.
➣ public void getChars(int srcBegin, int srcEnd, char[] dst,
int dstBegin): provides a way to store strings within the specified index range into an array.
/** * @Description: * Conversion between string and character array * 1,string-->chars[] string.toCharArray(); * 2,chars[]-->string new String(chars[])constructor */ public void stringAndChars(){ String s1 = "Java China 2020"; //string to chars [] array 1 char c1[] = s1.toCharArray(); for (int i = 0; i < c1.length; i++) { System.out.print(c1[i] + "|"); } System.out.println(); //string to chars [] array 1 char c2[] = new char[s1.length()]; s1.getChars(0,s1.length(),c2,0); for (int i = 0; i < c2.length; i++) { System.out.print(c2[i] + "|"); } System.out.println(); //chars [] array to string String s2 = new String(c1); System.out.println(s2); }
▾ String and byte array conversion
Byte array ➟ string
➣ String(byte []): decode the specified byte array with the default character set of the platform through the String constructor, and construct
Make a new String.
➣ String(byte[],String charsetName): use the specified character set to decode the specified byte array through the String constructor to construct a new String.
➣ String(byte [], int offset, int length): a string object is constructed by taking the length bytes from the offset of the starting position specified in the array.
String ➟ byte array
ζ public byte[] getBytes(): use the default character set of the platform to encode this String as a byte sequence and store the result in a new byte[] array.
➣ public byte[] getBytes(String charsetName): encodes this String into a byte sequence using the specified character set, and stores the result in a new byte [] array..
public void stringAndBytes() throws UnsupportedEncodingException { String s1 = "Java China 2020"; //String string to bytes [] array, the default character set is set by the system (here utf-8) byte []b1 = s1.getBytes(); System.out.println(Arrays.toString(b1)); //Specifies character set rules for string to bytes [] byte b2[] = s1.getBytes("gbk"); System.out.println(Arrays.toString(b2)); //Arrays.toString (array); convert array to string with [] //byte b3[] = s1.getBytes("gbs"); / / unsupported encodingexception character set exception reported //bytes [] array to string string System.out.println(new String(b1)); //bytes [] array converted to string string, character set does not correspond System.out.println(new String(b2)); //There is disorder //The bytes [] array is converted to a string string, and the corresponding character set is specified System.out.println(new String(b2,"gbk")); }
☑ StringBuffer and StringBuilder
☄ java.lang.StringBuffer and java.lang.StringBuilder Represents a variable character sequence, which is declared in JDK 1.0. When the string content can be added or deleted, no new objects will be generated at this time.
☄ There are many ways for both to be the same as String.
☄ When passed as a parameter, the value can be changed inside the method. (as opposed to the String example above)
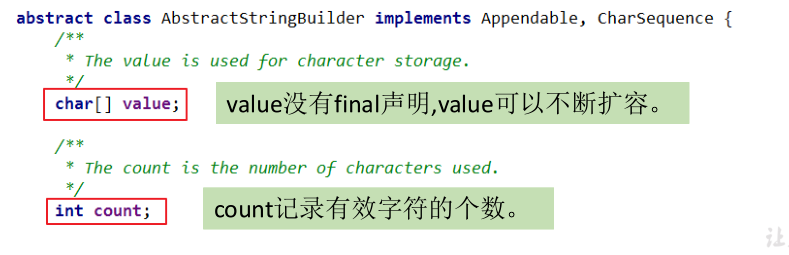
☃ The StringBuffer class is different from String, and its objects must be generated using a constructor. There are three constructors:
➣ StringBuffer(): initially a string buffer with a capacity of 16
➣ StringBuffer(int size): construct a string buffer of the specified capacity
➣ StringBuffer(String str): initializes the content to the specified string content
☃ StringBuilder is similar to StringBuffer
Similarities and differences of String, StringBuffer and StringBuilder:
☄ String: immutable character sequence; the bottom layer is stored with char [] array.
☄ StringBuffer: variable character sequence; thread safety, relatively low phase ratio; the bottom layer uses char [] array storage.
☄ StringBuilder: variable character sequence; new in jdk5.0, unsafe thread, relatively high efficiency; bottom layer uses char [] array storage.
✦ if passed as a parameter, the value of String in the method will not be changed, and StringBuffer and StringBuilder will change its value
/* Source code analysis: String str = new String(); ---- new char[0]; String str1 = new String("abc"); ---- new char[]{'a','b','c'}; StringBuffer sb1 = new StringBuffer(); ---- new char[16] By default, a char array with a length of 16 is created sb1.append('a'); ---- value[0] = 'a'; sb2.append('b'); ---- value[1] ='b'; System.out.println(sb1.length); //sb1 The length of is 0. Note that it is not 16, 16 is the length of char []value. StringBuffer sb2 = new String("abc"); ---- char []value = new char["abc".length + 16]; StringBuilder Very similar to StringBuffer usage, most methods are the same, and they also have most methods of String Question 1: System.out.println(sb2.length); //3 Problem 2: expansion problem: if the added data exceeds the initial space of the underlying array, you need to expand the array By default, the capacity is expanded to 2 times the original capacity + 2, and the elements in the original array are copied to the new array Recommendation: StringBuffer(int capacity) or StringBuilder(int capacity) is recommended for development Avoid efficiency reduction caused by array expansion */ public class StringBufferAndStringBuilder { @Test public void test1(){ StringBuffer sb1 = new StringBuffer("abc123"); sb1.setCharAt(0,'m'); sb1.append("earth"); System.out.println(sb1); //mbc, the operation on StringBuffer object will affect the original StringBuffer object StringBuilder sb2 = new StringBuilder("abc123"); sb2.setCharAt(0,'m'); sb2.append("China"); System.out.println(sb2); }
▾ common methods of StringBuffer and StringBuilder classes
No | method | explain |
---|---|---|
1 | append(xxx) | Many append() methods are provided for string splicing |
2 | deleteCharAt(int index) | Delete characters at the specified index location |
3 | delete(int start,int end) | Delete the contents of the specified range |
4 | setCharAt(int index, char ch) | Sets the character value for the specified index location |
5 | replace(int start, int end, String str) | Replace the string in the [start,end) position with another string str |
6 | insert(int offset, xxx) | Insert element xxx at the specified index location |
7 | reverse() | Reverse character sequence |
public void methods(){ StringBuffer sb1 = new StringBuffer("abc"); //Add character / string at the end sb1.append(1234); sb1.append('in'); sb1.append("$@#"); System.out.println(sb1); //In abc1234$@# //Delete characters at the specified index location sb1.deleteCharAt(2); System.out.println(sb1); //ab1234$@# //Sets the character value for the specified index location sb1.setCharAt(0,'A'); //Delete the characters from startIndex to endIndex position, [startIndex,endIndex) before closing and then opening sb1.delete(3,5); System.out.println(sb1); //Ab14 middle$@# //Replace the string at the [start,end) position with another string str:replace(int start, int end, String str) sb1.replace(5,8,"country"); System.out.println(sb1); //Ab14 China //Inserts an element (number, character, string, Boolean) at the specified index location sb1.insert(4,"China"); System.out.println(sb1); //Ab14China China //Reverse character sequence sb1.reverse(); System.out.println(sb1); //Junior high school anihC41bA }
▾ comparison of execution efficiency of String, StringBuffer and StringBuilder classes
Execution efficiency: StringBuilder > StringBuffer > > string
/** * @Description: String,StringBuffer,StringBuilder Comparison of the three execution efficiency * StringBuilder > StringBuffer >> String * Reason: StringBuffer and StringBuilder are multithreaded, so the efficiency is much higher than String * StringBuffer It is thread safe. StringBuilder is not thread safe, so StringBuilder is more efficient */ @Test public void strCompare(){ //Initial settings long startTime = 0L; long endTime = 0L; String text = ""; StringBuffer buffer = new StringBuffer(""); StringBuilder builder = new StringBuilder(""); //Start to compare startTime = System.currentTimeMillis(); for (int i = 0; i < 20000; i++) { buffer.append(String.valueOf(i)); } endTime = System.currentTimeMillis(); System.out.println("StringBuffer Execution time of:" + (endTime - startTime)); //StringBuffer execution time: 35 startTime = System.currentTimeMillis(); for (int i = 0; i < 20000; i++) { builder.append(String.valueOf(i)); } endTime = System.currentTimeMillis(); System.out.println("StringBuilder Execution time of:" + (endTime - startTime)); //StringBuilder execution time: 15 startTime = System.currentTimeMillis(); for (int i = 0; i < 20000; i++) { text = text + i; } endTime = System.currentTimeMillis(); System.out.println("String Execution time of:" + (endTime - startTime)); //String execution time: 1342 }
Common methods of String class Portal
Blog and blog Park He's the king A kind of Fiber filter Synchronous Publishing
Dream is a beautiful journey. Everyone is just a lonely teenager before finding it.