Reprint address: http://blog.csdn.net/yuanzeyao/article/details/42418977
JNI technology for many Java Developed friends believe that it is not unfamiliar, that is( Java native
interface), the local call interface, has the following two main functions:
1. Calling C/C++ Layer Code in java Layer
2. C/C++ Layer Calls java Layer Code
Some people may think that JNI technology destroys the cross-platform nature of Java language. Maybe this idea is because you don't understand java well enough. If you look at the source code of jdk, you will find that JNI technology is widely used in jdk, and the Java virtual machine is written in local language, so jvm can't cross-platform. So the cross-platform nature of Java is not 100% cross-platform. Instead, you should see the advantages of using Jni:
1. Because C/C++ language was born earlier than java language, so many library codes are written in C/C++. With Jni, we can use it directly without repeating the wheel.
2. It is undeniable that C/C++ is more efficient than java. For some functions that require efficiency, C/C++ must be used.
As a result of intentional research Android How the java layer and native layer are connected, so I want to study the JNI Technology in Android (before reading, it's better to know the basic knowledge in jni, such as data type in jni, signature format, otherwise it may seem a little laborious). Because the work is related to MediaPlayer, let's use MediaPlayer as an example. .
Here's a diagram that shows how jni connects the Java layer to the local layer.
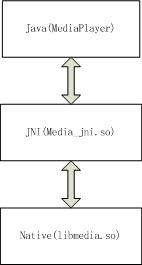
When our app wants to play video, we use the MediaPlayer class in the java layer. Let's go to MediaPlayer.java and have a look. (Reminder: I use source code 4.1 here)
There are two main points to be paid attention to:
1. Static code block:
-
static {
-
System.loadLibrary("media_jni");
-
native_init();
-
}
2. Signature of native_init:
-
private static native final void native_init();
After seeing the static code block, we can see that the JNI layer code corresponding to MediaPlayer is in the Media_jni.so library.
The so library corresponding to the local layer is libmedia.so, so MediaPlayer.java interacts with Media_jni.so and MediaPlayer.cpp(libmedia.so).
Let's go into the details. But before going into details, let me tell you a rule. In Android, the names of the Java layer and jni layer are usually related as follows. Take MediaPlayer as an example. The Java layer is called android.media.MediaPlayer.java, and the jni layer is called android_media_MediaPlayer.cpp.
Since native_init is a local method, let's go to android_media_MediaPlayer.cpp and find the corresponding method for native_init.
-
static void
-
android_media_MediaPlayer_native_init(JNIEnv *env)
-
{
-
jclass clazz;
-
-
clazz = env->FindClass("android/media/MediaPlayer");
-
if (clazz == NULL) {
-
return;
-
}
-
-
fields.context = env->GetFieldID(clazz, "mNativeContext", "I");
-
if (fields.context == NULL) {
-
return;
-
}
-
-
fields.post_event = env->GetStaticMethodID(clazz, "postEventFromNative",
-
"(Ljava/lang/Object;IIILjava/lang/Object;)V");
-
if (fields.post_event == NULL) {
-
return;
-
}
-
-
fields.surface_texture = env->GetFieldID(clazz, "mNativeSurfaceTexture", "I");
-
if (fields.surface_texture == NULL) {
-
return;
-
}
-
}
Corresponding to the above code, if you have a thorough understanding of reflection in java, you can actually understand it very well. First, find the lass object of MediaPlayer in the Java layer. jclass is the code of Java layer Class in the native layer. Then save the mNaviceContext field, postEventFromNative method and mNativeFaceTexture field respectively.
In fact, what I want to illustrate here is another question, how does the native_init method in MediaPlayer correspond to android_media_MediaPlayer.cpp's android_media_MediaPlayer_native_init, because we know that if we use the header file generated automatically by javah, the name in the jni layer should be java_and Roid_media_MediaPlayer_native_linit. In fact, this involves a dynamic registration process.
In fact, when the java layer succeeds in replacing System. load Library, the JNI_onLoad method in the jni file will be called, and the JNI_onLoad method in android_media_MediaPlayer.cpp will be as follows (interception part)
-
jint JNI_OnLoad(JavaVM* vm, void* reserved)
-
{
-
JNIEnv* env = NULL;
-
jint result = -1;
-
-
if (vm->GetEnv((void**) &env, JNI_VERSION_1_4) != JNI_OK) {
-
ALOGE("ERROR: GetEnv failed\n");
-
goto bail;
-
}
-
assert(env != NULL);
-
-
if (register_android_media_MediaPlayer(env) < 0) {
-
ALOGE("ERROR: MediaPlayer native registration failed\n");
-
goto bail;
-
}
-
-
-
-
-
result = JNI_VERSION_1_4;
-
-
bail:
-
return result;
-
}
Here's a method called register_android_media_MediaPlayer. Let's go into this method and see what's registered.
-
static int register_android_media_MediaPlayer(JNIEnv *env)
-
{
-
return AndroidRuntime::registerNativeMethods(env,
-
"android/media/MediaPlayer", gMethods, NELEM(gMethods));
-
}
Here's a call to the registerNativeMethods method provided by Android Runtime, which involves a variable of gMethods, which is actually a structure.
-
typedef struct {
-
const char* name;
-
const char* signature;
-
void* fnPtr;
-
} JNINativeMethod;
Name: is the method name at the java layer
signature: means that the method is signing
fnPtr: The name of the function corresponding to the jni layer
So let's find the value of native_init in gMethods
-
static JNINativeMethod gMethods[] = {
-
{
-
"_setDataSource",
-
"(Ljava/lang/String;[Ljava/lang/String;[Ljava/lang/String;)V",
-
(void *)android_media_MediaPlayer_setDataSourceAndHeaders
-
},
-
-
....
-
{"native_init", "()V", (void *)android_media_MediaPlayer_native_init},
-
...
-
};
Next, let's look at what registerNative Methods in Android Runtime has done.
-
int AndroidRuntime::registerNativeMethods(JNIEnv* env,
-
const char* className, const JNINativeMethod* gMethods, int numMethods)
-
{
-
return jniRegisterNativeMethods(env, className, gMethods, numMethods);
-
}
Called jniRegister Native Methods
-
extern "C" int jniRegisterNativeMethods(C_JNIEnv* env, const char* className,
-
const JNINativeMethod* gMethods, int numMethods)
-
{
-
JNIEnv* e = reinterpret_cast<JNIEnv*>(env);
-
-
ALOGV("Registering %s natives", className);
-
-
scoped_local_ref<jclass> c(env, findClass(env, className));
-
if (c.get() == NULL) {
-
ALOGE("Native registration unable to find class '%s', aborting", className);
-
abort();
-
}
-
-
if ((*env)->RegisterNatives(e, c.get(), gMethods, numMethods) < 0) {
-
ALOGE("RegisterNatives failed for '%s', aborting", className);
-
abort();
-
}
-
-
return 0;
-
}
Eventually, the Register Nativers of env was invoked to complete the registration.
As a matter of fact, we already know how the java layer and jni are connected. Next, I want to talk about how jni connects the java layer and native. Let's take MediaPlayer as an example. Let's go into the constructor of MediaPlayer.
-
public MediaPlayer() {
-
-
Looper looper;
-
if ((looper = Looper.myLooper()) != null) {
-
mEventHandler = new EventHandler(this, looper);
-
} else if ((looper = Looper.getMainLooper()) != null) {
-
mEventHandler = new EventHandler(this, looper);
-
} else {
-
mEventHandler = null;
-
}
-
-
-
-
-
native_setup(new WeakReference<MediaPlayer>(this));
-
}
Here we create an mEventHandler object and call the native_setup method. Let's go to the corresponding method of android_media_MediaPlayer.cpp.
-
static void
-
android_media_MediaPlayer_native_setup(JNIEnv *env, jobject thiz, jobject weak_this)
-
{
-
ALOGV("native_setup");
-
sp<MediaPlayer> mp = new MediaPlayer();
-
if (mp == NULL) {
-
jniThrowException(env, "java/lang/RuntimeException", "Out of memory");
-
return;
-
}
-
-
-
sp<JNIMediaPlayerListener> listener = new JNIMediaPlayerListener(env, thiz, weak_this);
-
mp->setListener(listener);
-
-
-
setMediaPlayer(env, thiz, mp);
-
}
Here we create a local MediaPlayer object and set up a listener. (If a former player knows what the listener should know, it doesn't matter.) Finally, we call the setMediaPlayer method, which is what we need to pay attention to.
-
static sp<MediaPlayer> setMediaPlayer(JNIEnv* env, jobject thiz, const sp<MediaPlayer>& player)
-
{
-
Mutex::Autolock l(sLock);
-
sp<MediaPlayer> old = (MediaPlayer*)env->GetIntField(thiz, fields.context);
-
if (player.get()) {
-
player->incStrong(thiz);
-
}
-
if (old != 0) {
-
old->decStrong(thiz);
-
}
-
env->SetIntField(thiz, fields.context, (int)player.get());
-
return old;
-
}
In fact, we get the corresponding value of fields.context first. Do you remember what this value is? If you don't remember, you can go back and see it.
-
fields.context = env->GetFieldID(clazz, "mNativeContext", "I");
In fact, the value corresponding to the mNativeContext in the java layer is to store the address of the local MediaPlayer in the mNativeContext.
Now join us to play a local Mp4 video, then use the following code
-
mediaPlayer.setDataSource("/mnt/sdcard/a.mp4");
-
mediaPlayer.setDisplay(surface1.getHolder());
-
mediaPlayer.prepare();
-
mediaPlayer.start();
In fact, several of the methods invoked here are local methods. Here I use the prepare d method as an example to explain the interaction between MediaPlaeyr.java and MediaPlayer.cpp.
When the prepare method is invoked at the java layer, the following method is invoked at the jni layer
-
static void
-
android_media_MediaPlayer_prepare(JNIEnv *env, jobject thiz)
-
{
-
sp<MediaPlayer> mp = getMediaPlayer(env, thiz);
-
if (mp == NULL ) {
-
jniThrowException(env, "java/lang/IllegalStateException", NULL);
-
return;
-
}
-
-
-
-
sp<ISurfaceTexture> st = getVideoSurfaceTexture(env, thiz);
-
mp->setVideoSurfaceTexture(st);
-
-
process_media_player_call( env, thiz, mp->prepare(), "java/io/IOException", "Prepare failed." );
-
}
Here we get the local MediaPlayer object through the getMediaPlayer method, call the local method process_media_player_call, and pass the result of the local MediaPlayer calling the pare method to this method.
-
static void process_media_player_call(JNIEnv *env, jobject thiz, status_t opStatus, const char* exception, const char *message)
-
{
-
if (exception == NULL) {
-
if (opStatus != (status_t) OK) {
-
sp<MediaPlayer> mp = getMediaPlayer(env, thiz);
-
if (mp != 0) mp->notify(MEDIA_ERROR, opStatus, 0);
-
}
-
} else {
-
if ( opStatus == (status_t) INVALID_OPERATION ) {
-
jniThrowException(env, "java/lang/IllegalStateException", NULL);
-
} else if ( opStatus == (status_t) PERMISSION_DENIED ) {
-
jniThrowException(env, "java/lang/SecurityException", NULL);
-
} else if ( opStatus != (status_t) OK ) {
-
if (strlen(message) > 230) {
-
-
jniThrowException( env, exception, message);
-
} else {
-
char msg[256];
-
-
sprintf(msg, "%s: status=0x%X", message, opStatus);
-
jniThrowException( env, exception, msg);
-
}
-
}
-
}
-
}
In this case, according to the status returned by prepares, if exception==null and prepares fail to execute, the test does not throw an exception, but calls the notify method of the local MediaPlayer.
-
void MediaPlayer::notify(int msg, int ext1, int ext2, const Parcel *obj)
-
{
-
ALOGV("message received msg=%d, ext1=%d, ext2=%d", msg, ext1, ext2);
-
bool send = true;
-
bool locked = false;
-
-
...
-
-
switch (msg) {
-
case MEDIA_NOP:
-
break;
-
case MEDIA_PREPARED:
-
ALOGV("prepared");
-
mCurrentState = MEDIA_PLAYER_PREPARED;
-
if (mPrepareSync) {
-
ALOGV("signal application thread");
-
mPrepareSync = false;
-
mPrepareStatus = NO_ERROR;
-
mSignal.signal();
-
}
-
break;
-
case MEDIA_PLAYBACK_COMPLETE:
-
ALOGV("playback complete");
-
if (mCurrentState == MEDIA_PLAYER_IDLE) {
-
ALOGE("playback complete in idle state");
-
}
-
if (!mLoop) {
-
mCurrentState = MEDIA_PLAYER_PLAYBACK_COMPLETE;
-
}
-
break;
-
case MEDIA_ERROR:
-
-
-
-
ALOGE("error (%d, %d)", ext1, ext2);
-
mCurrentState = MEDIA_PLAYER_STATE_ERROR;
-
if (mPrepareSync)
-
{
-
ALOGV("signal application thread");
-
mPrepareSync = false;
-
mPrepareStatus = ext1;
-
mSignal.signal();
-
send = false;
-
}
-
break;
-
case MEDIA_INFO:
-
-
-
if (ext1 != MEDIA_INFO_VIDEO_TRACK_LAGGING) {
-
ALOGW("info/warning (%d, %d)", ext1, ext2);
-
}
-
break;
-
case MEDIA_SEEK_COMPLETE:
-
ALOGV("Received seek complete");
-
if (mSeekPosition != mCurrentPosition) {
-
ALOGV("Executing queued seekTo(%d)", mSeekPosition);
-
mSeekPosition = -1;
-
seekTo_l(mCurrentPosition);
-
}
-
else {
-
ALOGV("All seeks complete - return to regularly scheduled program");
-
mCurrentPosition = mSeekPosition = -1;
-
}
-
break;
-
case MEDIA_BUFFERING_UPDATE:
-
ALOGV("buffering %d", ext1);
-
break;
-
case MEDIA_SET_VIDEO_SIZE:
-
ALOGV("New video size %d x %d", ext1, ext2);
-
mVideoWidth = ext1;
-
mVideoHeight = ext2;
-
break;
-
case MEDIA_TIMED_TEXT:
-
ALOGV("Received timed text message");
-
break;
-
default:
-
ALOGV("unrecognized message: (%d, %d, %d)", msg, ext1, ext2);
-
break;
-
}
-
-
sp<MediaPlayerListener> listener = mListener;
-
if (locked) mLock.unlock();
-
-
-
if ((listener != 0) && send) {
-
Mutex::Autolock _l(mNotifyLock);
-
ALOGV("callback application");
-
listener->notify(msg, ext1, ext2, obj);
-
ALOGV("back from callback");
-
}
-
}
Students who have played the player should be familiar with the above several messages. Because the call to prepare method failed just now, the MEDIA_ERROR branch should be executed here. Finally, the notify code of listener should be called. This listener is set up in native_setup.
-
void JNIMediaPlayerListener::notify(int msg, int ext1, int ext2, const Parcel *obj)
-
{
-
JNIEnv *env = AndroidRuntime::getJNIEnv();
-
if (obj && obj->dataSize() > 0) {
-
jobject jParcel = createJavaParcelObject(env);
-
if (jParcel != NULL) {
-
Parcel* nativeParcel = parcelForJavaObject(env, jParcel);
-
nativeParcel->setData(obj->data(), obj->dataSize());
-
env->CallStaticVoidMethod(mClass, fields.post_event, mObject,
-
msg, ext1, ext2, jParcel);
-
}
-
} else {
-
env->CallStaticVoidMethod(mClass, fields.post_event, mObject,
-
msg, ext1, ext2, NULL);
-
}
-
if (env->ExceptionCheck()) {
-
ALOGW("An exception occurred while notifying an event.");
-
LOGW_EX(env);
-
env->ExceptionClear();
-
}
-
}
Remember what fields.post_event saves?
-
fields.post_event = env->GetStaticMethodID(clazz, "postEventFromNative",
-
"(Ljava/lang/Object;IIILjava/lang/Object;)V");
It's the postEventFromNative method of the java layer MediaPlayer, that is, if the playback is wrong, then call the postEventFromNative method to tell the java layer MediaPlayer.
-
private static void postEventFromNative(Object mediaplayer_ref,
-
int what, int arg1, int arg2, Object obj)
-
{
-
MediaPlayer mp = (MediaPlayer)((WeakReference)mediaplayer_ref).get();
-
if (mp == null) {
-
return;
-
}
-
-
if (what == MEDIA_INFO && arg1 == MEDIA_INFO_STARTED_AS_NEXT) {
-
-
mp.start();
-
}
-
if (mp.mEventHandler != null) {
-
Message m = mp.mEventHandler.obtainMessage(what, arg1, arg2, obj);
-
mp.mEventHandler.sendMessage(m);
-
}
-
}
This time is finally handled by mEventHandler, which is to handle this error in our app process.
As I write here, I believe you should have a good understanding of the interaction between the java layer and the native layer.