Official documents:
Third, access to a global function is still using Get method, the difference is type mapping. 1. Mapping to delegate
This is the recommended approach, with much better performance and type safety. The disadvantage is to generate code (if no code is generated, an InvalidCastException exception is thrown).
How would delegate declare it? For each parameter of function, an input type parameter is declared.
How to deal with multiple return values? Mapping from left to right to the output parameters of c, the output parameters include return values, out parameters, ref parameters.
What are the parameters and return value types that support? It supports all kinds of complex types, out, ref modifiers, and even returns another delegate.
The use of delegate is simpler, just like a function. 2. The advantages and disadvantages of mapping to LuaFunction are just the opposite of the first one.
It is also simple to use. There is a variable parameter Call function on LuaFunction. It can pass any type and number of parameters. The return value is an array of object s, which corresponds to the multiple return value of lua.
4. Use Recommendation 1. Accessing lua global data, especially table s and function s, is costly. It is recommended to do as little as possible, such as initializing the lua to be invoked.
function is retrieved once (mapped to delegate), saved and then called directly. table is similar.
2. If the implementation part of lua measurement is provided by delegate and interface, the user can be completely decoupled from xlua: a special module is responsible for the initialization of xlua and the mapping of delegate and interface, and then these delegate and interface are set to where they are to be used.
Calling the global method without parameters in Lua |
|
|
|
private LuaEnv luaEnv;
void Start()
{
luaEnv = new LuaEnv();//Global uniqueness
luaEnv.AddLoader(CustomLoader);//Add custom paths
luaEnv.DoString("require '007' ");//Find 007 file in custom path
//Method is a delegate in type, in C #.
Action fun = luaEnv.Global.Get<Action>("init");//Parametric-free method init in mapping Lua
fun();//Executing the method of mapping C# to Lua
}
private byte[] CustomLoader(ref string filepath)
{
string path = Application.dataPath + "/Scripts/Xlua/" + filepath + ".lua";//Custom Lua File Path
return Encoding.UTF8.GetBytes(File.ReadAllText(path));//Returns byte [] data for the corresponding file
}

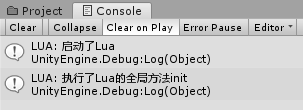
Calling global methods with parameters in Lua |
|
|
|
private LuaEnv luaEnv;
void Start()
{
luaEnv = new LuaEnv();//Global uniqueness
luaEnv.AddLoader(CustomLoader);//Add custom paths
luaEnv.DoString("require '007' ");//Find 007 files in custom paths
//Method is a delegate in type, in C #.
//Action fun = luaEnv. Global. Get < Action >("init"); and // Map the parametric method init in Lua
//fun(); // Execute the method of mapping C # to Lua
//=============================================================
//Call the Lua method with parameters and return values, and define how many out or ref return values to receive.
//You need to define your own delegate to map the corresponding method, and you need to add the [CSharpCallLua] label
add luaaddfunc = luaEnv.Global.Get<add>("add");//Executing Lua has parameters but does not return a worthwhile method
luaaddfunc(20, 30);
}
[CSharpCallLua]//To call methods in Lua, you need to add this label
public delegate void add(int a, int b);
private byte[] CustomLoader(ref string filepath)
{
string path = Application.dataPath + "/Scripts/Xlua/" + filepath + ".lua";//Custom Lua File Path
return Encoding.UTF8.GetBytes(File.ReadAllText(path));//Returns byte [] data for the corresponding file
}
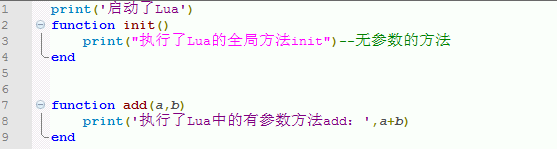
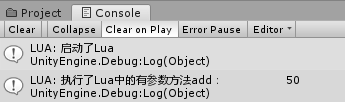
Calling a method with parameters and only one return value in Lua, you can accept the return directly, or you can use the out, ref keyword to accept it. |
|
|
|
private LuaEnv luaEnv;
void Start()
{
luaEnv = new LuaEnv();//Global uniqueness
luaEnv.AddLoader(CustomLoader);//Add custom paths
luaEnv.DoString("require '007' ");//Find 007 files in custom paths
//Call the Lua method with parameters and return values, and define how many out or ref return values to receive.
//You need to define your own delegate to map the corresponding method, and you need to add the [CSharpCallLua] label
addonereturn addreturn = luaEnv.Global.Get<addonereturn>("addonereturn");
//When there is a return value, you can return directly.
string return1 = addreturn(20, 30);
Debug.Log("return1:[" + return1 + "] ");
}
[CSharpCallLua]//This tag needs to be added to invoke methods in Lua when there are multiple revert values
public delegate string addonereturn(int a, int b);
private byte[] CustomLoader(ref string filepath)
{
string path = Application.dataPath + "/Scripts/Xlua/" + filepath + ".lua";//Custom Lua File Path
return Encoding.UTF8.GetBytes(File.ReadAllText(path));//Returns byte [] data for the corresponding file
}
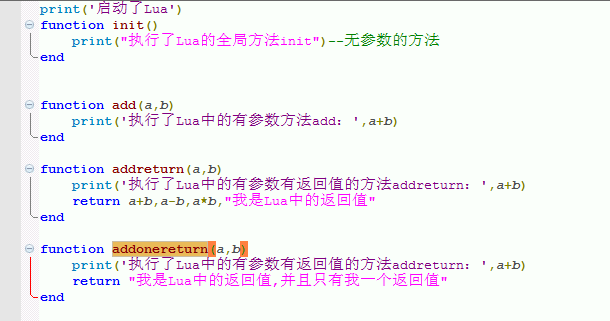
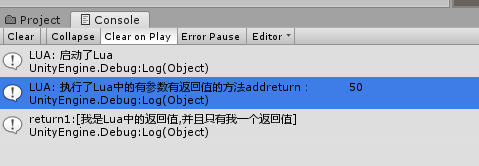
Call the global method out keyword with multiple return values for parameters in Lua |
|
|
|
private LuaEnv luaEnv;
void Start()
{
luaEnv = new LuaEnv();//Global uniqueness
luaEnv.AddLoader(CustomLoader);//Add custom paths
luaEnv.DoString("require '007' ");//Find 007 files in custom paths
//Method is a delegate in type, in C #.
//Action fun = luaEnv. Global. Get < Action >("init"); and // Map the parametric method init in Lua
//fun(); // Execute the method of mapping C # to Lua
//=============================================================
//Call the Lua method with parameters and return values, and define how many out or ref return values to receive.
//You need to define your own delegate to map the corresponding method, and you need to add the [CSharpCallLua] label
//Add luaaddfunc = luaEnv. Global. Get < add >("add"); // Execute Lua with parameters but without returning a worthwhile method
//luaaddfunc(20, 30);
int return1;
int return2;
int return3;
string return4;
addreturn addreturn = luaEnv.Global.Get<addreturn>("addreturn");
//Executing Lua has parameters, but there are several methods worth returning
//When there is a return value, you can return directly.
addreturn(20, 30,out return1,out return2,out return3,out return4);
Debug.Log("return1:["+return1+ "] return2:[" + return2+ "] return3:[" + return3+ "] return4:[" + return4+"]");
}
[CSharpCallLua]//When there are multiple return values
public delegate void addreturn(int a, int b,out int return1, out int return2, out int return3,out string return4);
[CSharpCallLua]//To call methods in Lua, you need to add this label
public delegate void add(int a, int b);
private byte[] CustomLoader(ref string filepath)
{
string path = Application.dataPath + "/Scripts/Xlua/" + filepath + ".lua";//Custom Lua File Path
return Encoding.UTF8.GetBytes(File.ReadAllText(path));//Returns byte [] data for the corresponding file
}
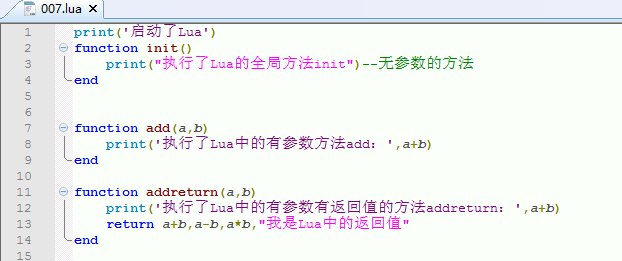
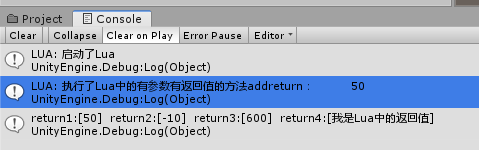
Call the global method ref keyword with multiple return values for parameters in Lua |
|
|
|
private LuaEnv luaEnv;
void Start()
{
luaEnv = new LuaEnv();//Global uniqueness
luaEnv.AddLoader(CustomLoader);//Add custom paths
luaEnv.DoString("require '007' ");//Find 007 files in custom paths
//Call the Lua method with parameters and return values, and define how many out or ref return values to receive.
//You need to define your own delegate to map the corresponding method, and you need to add the [CSharpCallLua] label
int return1=0;
int return2=0;
int return3=0;
string return4="";
addreturnref addreturn = luaEnv.Global.Get<addreturnref>("addreturn");
//Executing Lua has parameters, but there are several methods worth returning
//When there is a return value, you can return directly.
addreturn(20, 30, ref return1, ref return2, ref return3, ref return4);
Debug.Log("return1:["+return1+ "] return2:[" + return2+ "] return3:[" + return3+ "] return4:[" + return4+"]");
}
[CSharpCallLua]//This tag needs to be added to invoke methods in Lua when there are multiple revert values
public delegate void addreturnref(int a, int b, ref int return1, ref int return2, ref int return3, ref string return4);
private byte[] CustomLoader(ref string filepath)
{
string path = Application.dataPath + "/Scripts/Xlua/" + filepath + ".lua";//Custom Lua File Path
return Encoding.UTF8.GetBytes(File.ReadAllText(path));//Returns byte [] data for the corresponding file
}
The results of execution are the same.
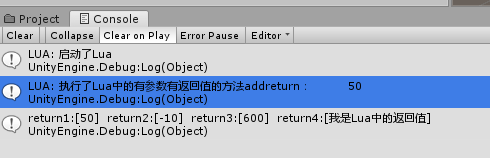