Two months ago, I completed the Spring Framework with the Spring Framework tutorial video of Mr. Wang He, the power node of station b. During the learning process, I tapped the code involved in the video line by line, and sorted out the materials related to the introduction of Spring. Here I record my notes and some insights during the learning process of Spring Framework, hoping to help you to pass the text.Getting Started with Word Tutorial Spring is a companion of this framework!
Video address: The latest Spring Framework Tutorial 2020 [IDEA Edition]-Spring Framework from Getting Started to Proficient
1.Introduction to IoC
Inversion of Control Is a design idea, not a specific technical implementation. refers to the transfer of object invocation rights traditionally directly controlled by program code to containers, through which object assembly and management can be achieved. Reverse control means control over objects.Transfer, from the program code itself to the external container. Containers are used to create objects, assign attributes, and manage dependencies.
However, IoC is not unique to Spirng and is also used in other languages.
Control: refers to the right to create (instantiate, manage) objects Reverse: Transfer the original developer-managed and object-creation privileges to container implementations outside of code. Containers manage objects, create objects, and assign values to properties instead of developers. Forward: Used by developers in code new Construction methods create objects and developers actively manage them. public static void main(String args[]){ Student student = new Student(); // In the code, create the object. --Forward. } Container: is a server software, a framework ( Spring)
Why use ioc: The goal is to reduce code changes and also to achieve different functions for decoupling.
Give the interdependencies between objects to the IoC container to manage and the IoC container to inject the objects. This greatly simplifies the development of applications and frees them from complex dependencies. The IoC container is like a factory. When we need to create an object, we just need to configure the configuration file/annotations to complete it.It doesn't matter how the object was created.
In a real project, a Service class may depend on many other classes. If we need to instantiate this service, you may need to know the constructors of all the underlying classes of this Service every time, which may drive people crazy. If you use IoC All you need to do is configure it and reference it where you need it, which greatly increases the maintainability of the project and reduces the difficulty of development.
In Spring, the IoC container is the carrier that Spring uses to implement IoC. The IoC container is actually a Map (key, value), where various objects are stored.
In the Spring era, we used to configure beans with XML files, but later developers found XML files poorly configured, and SpringBoot annotation configurations slowly became popular.
How to create objects in java:
- Using the construction method, new Student()
- reflex
- serialize
- Clone
- IoC: Container creation object
- Dynamic Proxy
The representation of IoC:
Serlet 1: Create class inherits HttpServlet
2: Register the servlet in web.xml, using
<servlet-name> myservlet </servlet-name> <servelt-class>com.kaho.controller.MyServlet</servlet-class>
3: No Servlet object was created during the process of using Servlet, that is, no MyServlet myservlet = new MyServlet();
4:Servlet s are created by Tomcat servers. Tomcat is also known as a container
Tomcat serves as a container: it holds Servlet objects, Listener s, Filter s, and so on
Technical implementation of IoC:
IoC technology is implemented by Dependent Injection (DI)
DI (Dependency Injection): Dependent on injection, you only need to provide the name of the object you want to use in your program.As for how objects are created, assigned, and found in containers, they are implemented inside the container. Program code does not make location queries, but the container does the work itself. When a program runs, it is not necessary to create the callee in the code when another object is called to assist it, but rather depends on the external container, which is created by the external container and passed to the program.
Spring implements IoC using Dependent Injection (DI). Spring creates objects at the bottom, using a reflection mechanism.
The Spring Container is a super-large factory responsible for creating and managing all Java objects called Bean s. The Spring Container manages the dependencies between beans in the container. Spring manages the dependencies between beans by using **"Dependent Injection"**. It uses IoC to decouple objects.
2.Preliminary Spring Program
1) Create a Maven Project
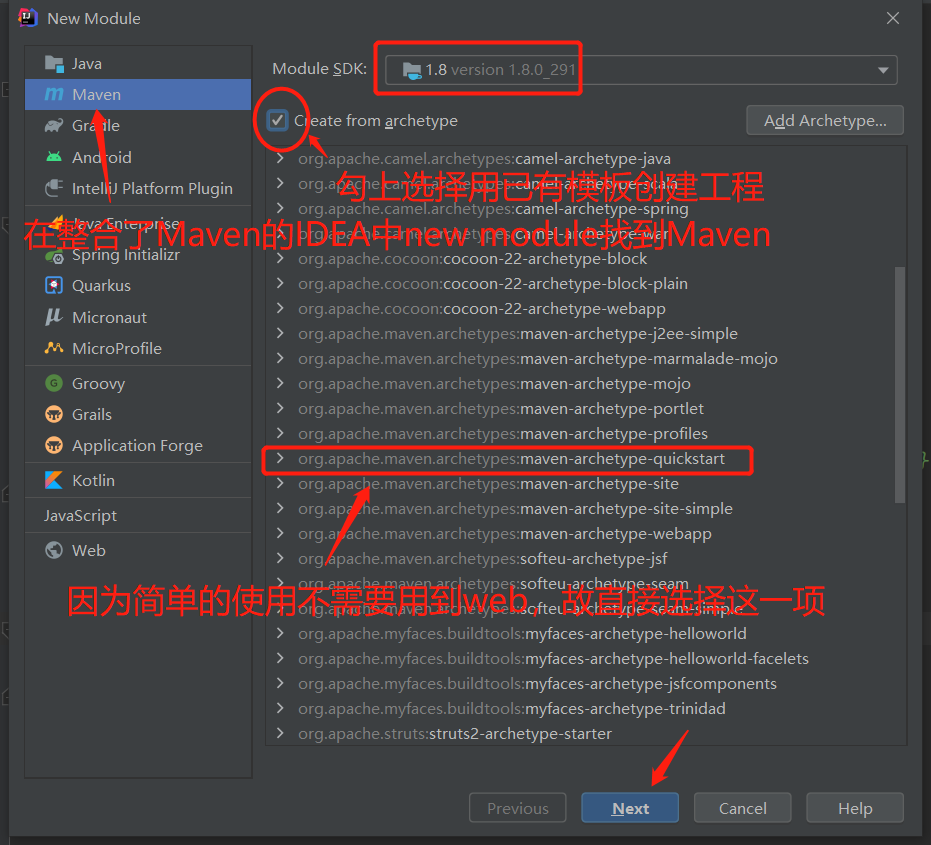
2) Introducing related Maven dependencies
pom.xml:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.kaho</groupId> <artifactId>ch01-hello-spring</artifactId> <version>1.0-SNAPSHOT</version> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <!--unit testing--> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.11</version> <scope>test</scope> </dependency> <!--spring rely on--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.2.5.RELEASE</version> </dependency> </dependencies> <build> <plugins> <!--Plug-in unit--> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> </plugins> </build> </project>
3) Define interfaces and their implementation classes
Interface:
package com.kaho.service; public interface SomeService { void doSome(); }
Implementation class:
package com.kaho.service.impl; import com.kaho.service.SomeService; public class SomeServiceImpl implements SomeService { public SomeServiceImpl(){ super(); System.out.println("SomeServiceImpl Parameterless construction method"); } @Override public void doSome() { System.out.println("====Business methods doSome()===="); } }
4) Create Spring Profile
Create a Spring configuration file in the src/main/resources/directory as follows:
File names can be arbitrary, but Spring recommends the name applicationContext.xml. Name it beans.xml for now
Configure information about the file:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- spring Profile 1.beans: Is the root tag, spring hold java The object is called bean. 2.spring-beans.xsd Is the constraint file, and mybatis Designated dtd It's the same. --> <!--register bean Object (tells) spring Create Object) statement bean,Is to tell spring Object to create a class id: Custom name of object, unique value. spring Access by this name Bean(Find Object),Bean and Bean Interdependence Dependency is also through id Attribute associated. class: Fully qualified name of the class (cannot be an interface because spring Is to use reflection mechanism to create objects, must use classes) Registration details: spring Will be completed SomeService someService = new SomeServiceImpl(); spring The frame has one map Used to store objects, spring Will place the created object in map Medium. springMap.put(id Value of,object); For example: springMap.put("someService",new SomeServiceImpl()); One bean Label declares an object --> <bean id="someService" class="com.kaho.service.impl.SomeServiceImpl" /> <bean id="someService1" class="com.kaho.service.impl.SomeServiceImpl" scope="prototype" /> <!--scope="prototype"That is, multiple-case mode, which means every call getBean()Will create a new object--> <!-- spring Can you create an object of a non-custom class and an object of an existing class? --> <bean id="mydate" class="java.util.Date" /> </beans>
5) Testing
package com.kaho; import com.kaho.service.SomeService; import com.kaho.service.impl.SomeServiceImpl; import org.junit.Test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MyTest { @Test public void test01(){ //Traditional ways of constructing objects SomeService service = new SomeServiceImpl(); service.doSome(); } /** * spring Default object creation time: All objects in the configuration file are created when the spring container is created. * spring Create object: The default call is to construct without parameters */ @Test public void test02(){ //Objects created using the spring container //1. Specify the name of the spring configuration file String config = "beans.xml"; //2. Create an object representing the spring container, ApplicationContext // The ApplicationContext is the Spring container through which objects are retrieved // ClassPathXmlApplicationContext: Represents a configuration file that loads spring from a class path ApplicationContext ac = new ClassPathXmlApplicationContext(config); //Get an object from a container by calling the method of the ApplicationContext object //getBean("id value of bean s in configuration file") returns Object type objects by default, so force SomeService service = (SomeService) ac.getBean("someService"); //Objects created with spring service.doSome(); } /** * Get information about java objects in the spring container */ @Test public void test03(){ String config = "beans.xml"; ApplicationContext ac = new ClassPathXmlApplicationContext(config); //Get the number of objects defined in the container, getBeanDefinitionCount(), using the method provided by spring int nums = ac.getBeanDefinitionCount(); System.out.println("Number of objects defined in container:" + nums); //The name of each defined object in the container, getBeanDefinitionNames(), returns an array of String types String[] names = ac.getBeanDefinitionNames(); for (String name : names){ System.out.println(name); } } //Get a non-custom class object @Test public void test04(){ String config = "beans.xml"; ApplicationContext ac = new ClassPathXmlApplicationContext(config); //Using getBean() Date date = (Date) ac.getBean("mydate"); System.out.println("Date:" + date); } }
3.Container interfaces and implementation classes
ApplicationContext interface (container)
The ApplicationContext loads Spring's configuration file and acts as a "container" in the program. It has two implementation classes.
1) Configuration file in class path
If the Spring configuration file is stored in the project's class path, the ClassPathXmlApplicationContext implementation class is used to load it.
@Test public void test02(){ //spring configuration file in class path //1. Specify the name of the spring configuration file String config = "beans.xml"; //2. Create objects that represent spring containers ApplicationContext ac = new ClassPathXmlApplicationContext(config); //Get an object from a container by calling the method of the ApplicationContext object //getBean("id value of bean s in configuration file") SomeService service = (SomeService) ac.getBean("someService"); service.doSome(); }
2) Assembly timing of objects in the ApplicationContext container
The ApplicationContext container, which assembles all the objects in the container object at once when it is initialized (spring creates the object: the default call is the parameterless construction method). To use these objects in future code, you only need to get them directly from memory. It is efficient to execute, but it takes up memory.