1, Foreword
The primary goal in developing Physijs is to keep it as simple and user-friendly as possible. Physics engines can be daunting
and difficult to set up, with so many options and configurations it is easy to feel overwhelmed. Physijs abstracts all of that extra logic out so you can focus on the rest of your project.
In short, the misspelled name of physijs is a set of physical engine that abstracts the underlying interface in ammo.js and encapsulates it for three.js.
2, GitHub address
3, Compatibility experience
1. The physical engine is simulated based on worker. However, the worker in wechat development tool has bug s and cannot be created. It can be fixed in the latest version.
2. Physi JS includes physi.js, ammo.js and physijs_worker.js. Apart from the basic physical library of ammo, the other two have some source codes that need to be modified, mainly for wx adaptation.
4, Code
The main difference between using physijs is that you can change the interface that originally used THREE to Physiji:
let THREE = require('./three/three') import Physijs from './three/physi' export default class game3d { constructor() { Physijs.scripts.worker = 'workers/request/physijs_worker.js'; // Here ammo.js needs to be bound directly in the worker // Physijs.scripts.ammo = './ammo.js'; // scene this.scene = new Physijs.Scene(); console.log(this.scene) // Perspective camera this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); // webGL renderer // At the same time, it specifies canvas as the canvas exposed by the game this.renderer = new THREE.WebGLRenderer({ canvas: canvas, antialias: true }); this.renderer.shadowMapEnabled = true; this.light = new THREE.AmbientLight(0xffffff); this.scene.add(this.light); this.start() } start() { // Add fog effects to the scene; use the same color as the background on the style this.renderer.setSize(window.innerWidth, window.innerHeight); var geometry = new THREE.CubeGeometry(1, 1, 1); // Load texture map var texture = new THREE.TextureLoader().load("images/metal.jpg"); var material = new THREE.MeshBasicMaterial({ map: texture }); this.ground = this.createGround() this.stage = this.createStage() this.scene.add(this.ground); this.scene.add(this.stage); // Set the height of the camera. If it is lower than the height of the current scene, fart will not be seen this.camera.position.z = 15; this.camera.position.y = 10; this.box = new Physijs.BoxMesh(new THREE.CubeGeometry(1, 1, 1), material); this.box.position.y = 10 this.scene.add(this.box); window.requestAnimationFrame(this.loop.bind(this), canvas); } createGround() { var geometry = new THREE.BoxGeometry(30, 1, 30); var texture = new THREE.TextureLoader().load("images/metal.jpg"); var material = new THREE.MeshBasicMaterial({ map: texture }); let cylinder = new Physijs.BoxMesh (geometry, material, 0); cylinder.position.y = 0 cylinder.position.x = 0.5 return cylinder } createStage() { var geometry = new THREE.CylinderGeometry(3, 1, 3); var texture = new THREE.TextureLoader().load("images/metal.jpg"); var material = new THREE.MeshBasicMaterial({ map: texture }); let cylinder = new Physijs.CylinderMesh(geometry, material); cylinder.position.y = 5 cylinder.position.x = 0.5 return cylinder } update() { this.stage.setAngularVelocity(new THREE.Vector3(0, 5, 0)) // this.ground.setLinearVelocity(new THREE.Vector3(1, 1, 0)) // Can't change the rotation angle directly // this.box.rotation.z += 0.06; } loop() { this.update() this.scene.simulate(); // run physics this.renderer.render(this.scene, this.camera); window.requestAnimationFrame(this.loop.bind(this), canvas); } }
5, Effect
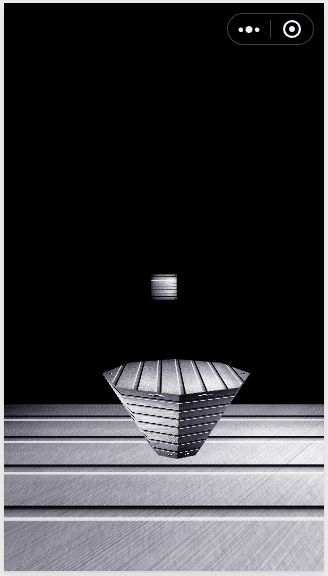