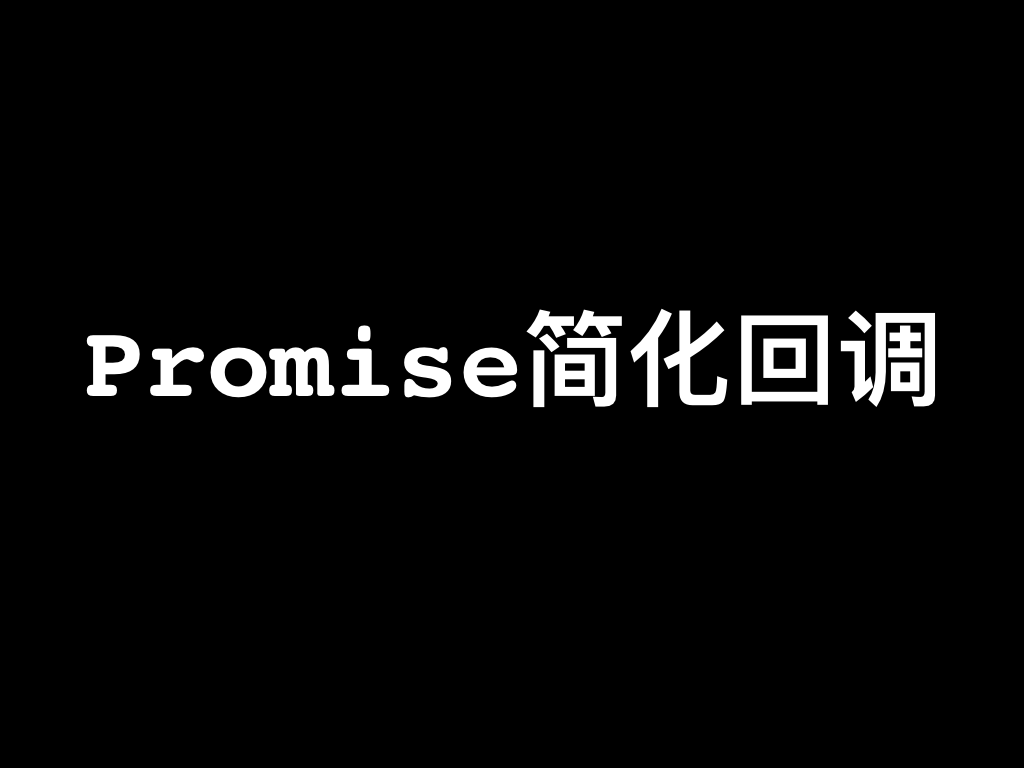
Understand what Promise objects are
In the project, there will be various asynchronous operations. If there are asynchronous operations in the callback of an asynchronous operation, there will be a callback pyramid.
Like the following
// Simulate to get the code, then pass the code to the background, get userinfo after success, and then pass userinfo to the background // Sign in wx.login({ success: res => { let code = res.code // request imitationPost({ url: '/test/loginWithCode', data: { code }, success: data => { // Get userInfo wx.getUserInfo({ success: res => { let userInfo = res.userInfo // request imitationPost({ url: '/test/saveUserInfo', data: { userInfo }, success: data => { console.log(data) }, fail: res => { console.log(res) } }) }, fail: res => { console.log(res) } }) }, fail: res => { console.log(res) } }) }, fail: res => { console.log(res) } })
Here's how to simplify the code with Promise
Because the asynchronous api of wechat applets is in the form of success and fail, everyone encapsulates such a method:
promisify.js
module.exports = (api) => { return (options, ...params) => { return new Promise((resolve, reject) => { api(Object.assign({}, options, { success: resolve, fail: reject }), ...params); }); } }
First look at the simplest:
// Get system information wx.getSystemInfo({ success: res => { // success console.log(res) }, fail: res => { } })
After simplification with promise.js above:
const promisify = require('./promisify') const getSystemInfo = promisify(wx.getSystemInfo) getSystemInfo().then(res=>{ // success console.log(res) }).catch(res=>{ })
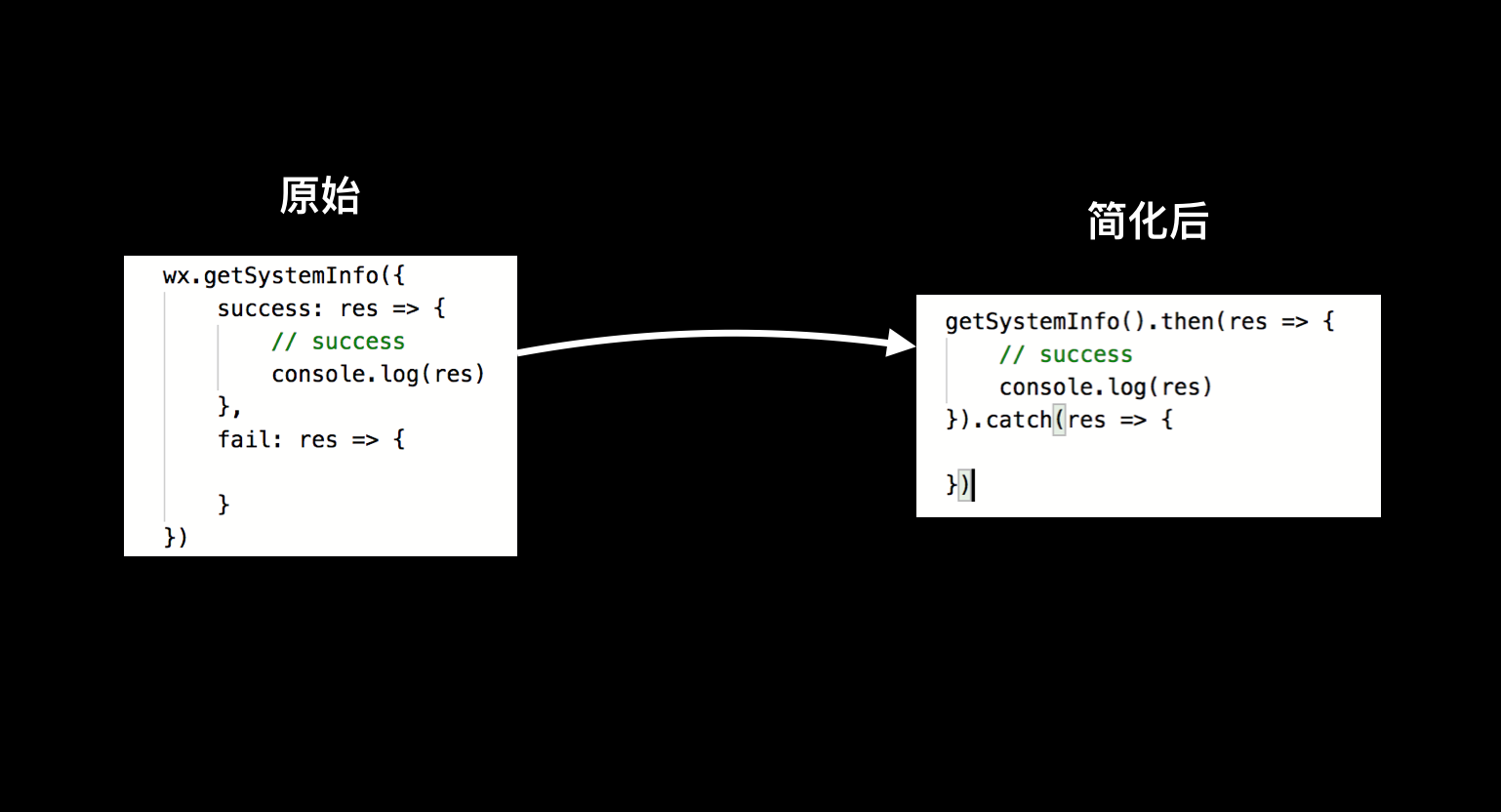
You can see that there is less indentation in the simplified callback, and the callback function is reduced from 9 lines to 6 lines.
Simplification effect of callback pyramid
So let's take a look at the first callback pyramid
const promisify = require('./promisify') const login = promisify(wx.login) const getSystemInfo = promisify(wx.getSystemInfo) // Sign in login().then(res => { let code = res.code // request pImitationPost({ url: '/test/loginWithCode', data: { code }, }).then(data => { // Get userInfo getUserInfo().then(res => { let userInfo = res.userInfo // request pImitationPost({ url: '/test/saveUserInfo', data: { userInfo }, }).then(data => { console.log(data) }).catch(res => { console.log(res) }) }).catch(res => { console.log(res) }) }).catch(res => { console.log(res) }) }).catch(res => { console.log(res) })
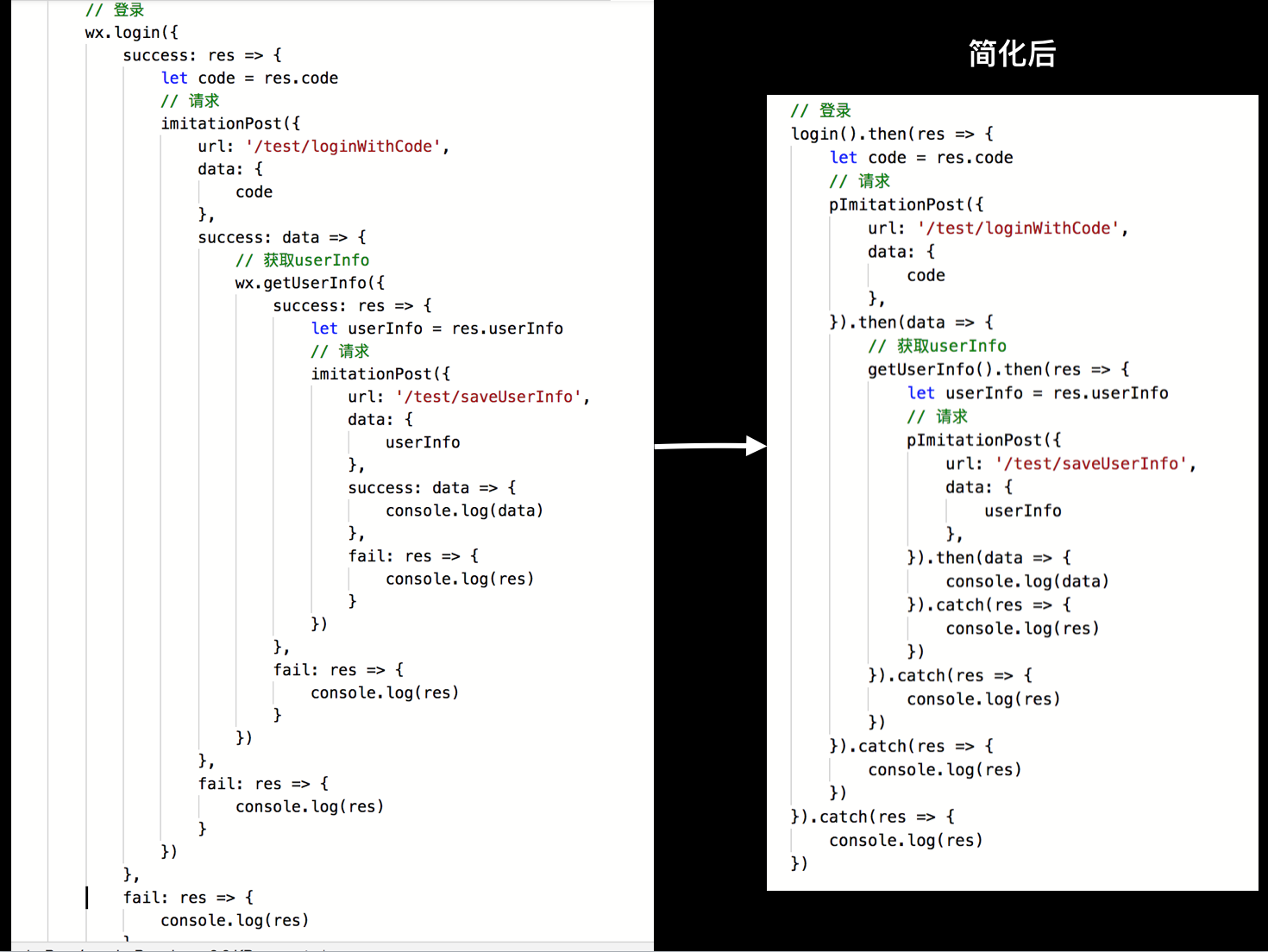
You can see that the simplification effect is very obvious.
It is also applicable to web pages or nodejs.
Reference resources
source code
- tomfriwel/MyWechatAppDemo The promise page of