Web static server
Web static server-1-display fixed page
#coding=utf-8 import socket def handle_client(client_socket): "Serving a client" recv_data = client_socket.recv(1024).decode("utf-8") request_header_lines = recv_data.splitlines() for line in request_header_lines: print(line) # Organize corresponding header response_headers = "HTTP/1.1 200 OK\r\n" # 200 means this resource is found response_headers += "\r\n" # Separate the body with an empty row # Organization content (body) response_body = "hello world" response = response_headers + response_body client_socket.send(response.encode("utf-8")) client_socket.close() def main(): "As the main control entry of the program" server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Set that when the server close s first, that is, after the server waves four times, the resources can be released immediately, so that the 7788 port can be bound immediately the next time the program runs server_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) server_socket.bind(("", 7788)) server_socket.listen(128) while True: client_socket, client_addr = server_socket.accept() handle_client(client_socket) if __name__ == "__main__": main()
Server side
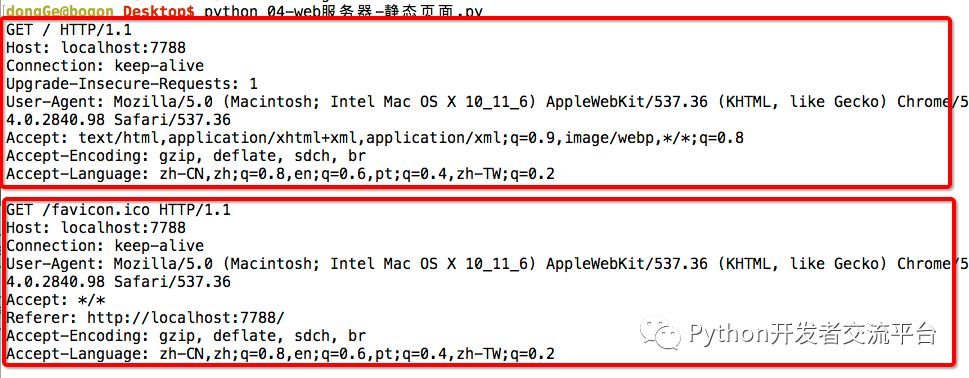
image
client
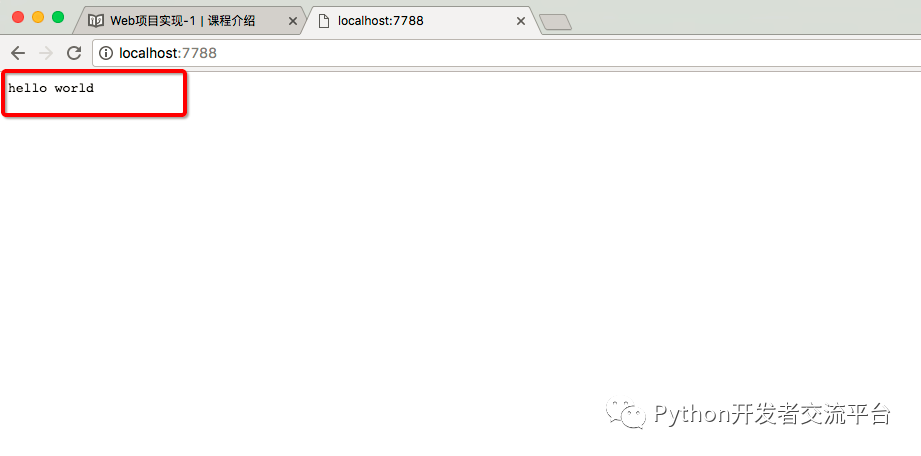
image
**Web static server-2-display required pages**
#coding=utf-8 import socket import re def handle_client(client_socket): "Serving a client" recv_data = client_socket.recv(1024).decode('utf-8', errors="ignore") request_header_lines = recv_data.splitlines() for line in request_header_lines: print(line) http_request_line = request_header_lines[0] get_file_name = re.match("[^/]+(/[^ ]*)", http_request_line).group(1) print("file name is ===>%s" % get_file_name) # for test # If you do not specify which page to visit. For example, index.html # GET / HTTP/1.1 if get_file_name == "/": get_file_name = DOCUMENTS_ROOT + "/index.html" else: get_file_name = DOCUMENTS_ROOT + get_file_name print("file name is ===2>%s" % get_file_name) #for test try: f = open(get_file_name, "rb") except IOError: # 404 means there is no such page response_headers = "HTTP/1.1 404 not found\r\n" response_headers += "\r\n" response_body = "====sorry ,file not found====" else: response_headers = "HTTP/1.1 200 OK\r\n" response_headers += "\r\n" response_body = f.read() f.close() finally: # Since the header information is organized according to string, it cannot be combined with the data read by binary open file, so it is sent separately # Send the response header first client_socket.send(response_headers.encode('utf-8')) # Resend body client_socket.send(response_body) client_socket.close() def main(): "As the main control entry of the program" server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) server_socket.bind(("", 7788)) server_socket.listen(128) while True: client_socket, clien_cAddr = server_socket.accept() handle_client(client_socket) #Configure the server here DOCUMENTS_ROOT = "./html" if __name__ == "__main__": main()
Server side

image.gif
client

image.gif
**Web static server-3-multiprocess**
#coding=utf-8 import socket import re import multiprocessing class WSGIServer(object): def __init__(self, server_address): # Create a tcp socket self.listen_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Allow the last bound port to be used immediately self.listen_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) # binding self.listen_socket.bind(server_address) # Becomes passive and determines the length of the queue self.listen_socket.listen(128) def serve_forever(self): "cycle operation web Server, waiting for client link and serving client" while True: # Waiting for new clients to arrive client_socket, client_address = self.listen_socket.accept() print(client_address) # for test new_process = multiprocessing.Process(target=self.handleRequest, args=(client_socket,)) new_process.start() # Because the child process has copied the socket and other resources of the parent process, the parent process call close will not close their corresponding link client_socket.close() def handleRequest(self, client_socket): "Use a new process to serve a client" recv_data = client_socket.recv(1024).decode('utf-8') print(recv_data) requestHeaderLines = recv_data.splitlines() for line in requestHeaderLines: print(line) request_line = requestHeaderLines[0] get_file_name = re.match("[^/]+(/[^ ]*)", request_line).group(1) print("file name is ===>%s" % get_file_name) # for test if get_file_name == "/": get_file_name = DOCUMENTS_ROOT + "/index.html" else: get_file_name = DOCUMENTS_ROOT + get_file_name print("file name is ===2>%s" % get_file_name) # for test try: f = open(get_file_name, "rb") except IOError: response_header = "HTTP/1.1 404 not found\r\n" response_header += "\r\n" response_body = "====sorry ,file not found====" else: response_header = "HTTP/1.1 200 OK\r\n" response_header += "\r\n" response_body = f.read() f.close() finally: client_so
Web static server-4-multithreading
#coding=utf-8 import socket import re import threading class WSGIServer(object): def __init__(self, server_address): # Create a tcp socket self.listen_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Allow the last bound port to be used immediately self.listen_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) # binding self.listen_socket.bind(server_address) # Becomes passive and determines the length of the queue self.listen_socket.listen(128) def serve_forever(self): "cycle operation web Server, waiting for client link and serving client" while True: # Waiting for new clients to arrive client_socket, client_address = self.listen_socket.accept() print(client_address) new_process = threading.Thread(target=self.handleRequest, args=(client_socket,)) new_process.start() # Because the thread shares the same socket, the main thread cannot close, otherwise the sub thread can no longer use the socket # client_socket.close() def handleRequest(self, client_socket): "Use a new process to serve a client" recv_data = client_socket.recv(1024).decode('utf-8') print(recv_data) requestHeaderLines = recv_data.splitlines() for line in requestHeaderLines: print(line) request_line = requestHeaderLines[0] get_file_name = re.match("[^/]+(/[^ ]*)", request_line).group(1) print("file name is ===>%s" % get_file_name) # for test if get_file_name == "/": get_file_name = DOCUMENTS_ROOT + "/index.html" else: get_file_name = DOCUMENTS_ROOT + get_file_name print("file name is ===2>%s" % get_file_name) # for test try: f = open(get_file_name, "rb") except IOError: response_header = "HTTP/1.1 404 not found\r\n" response_header += "\r\n" response_body = "====sorry ,file not found====" else: response_header = "HTTP/1.1 200 OK\r\n" response_header += "\r\n" response_body = f.read() f.close() finally: client_socket.send(response_header.enc
Web static server-5-non blocking mode
Single process non blocking model
#coding=utf-8 from socket import * import time # socket used to store all new links g_socket_list = list() def main(): server_socket = socket(AF_INET, SOCK_STREAM) server_socket.setsockopt(SOL_SOCKET, SO_REUSEADDR , 1) server_socket.bind(('', 7890)) server_socket.listen(128) # Set socket to non blocking # When it is set to non blocking, if there is no client connect ion when accepting, then accept will # An exception is generated, so you need to try to handle it server_socket.setblocking(False) while True: # For testing time.sleep(0.5) try: newClientInfo = server_socket.accept() except Exception as result: pass else: print("A new client comes:%s" % str(newClientInfo)) newClientInfo[0].setblocking(False) # Set to non blocking g_socket_list.append(newClientInfo) for client_socket, client_addr in g_socket_list: try: recvData = client_socket.recv(1024) if recvData: print('recv[%s]:%s' % (str(client_addr), recvData)) else: print('[%s]Client closed' % str(client_addr)) client_socket.close() g_socket_list.remove((client_socket,client_addr)) except Exception as result: pass print(g_socket_list) # for test if __name__ == '__main__': main()
web static server single process non blocking
import time import socket import sys import re class WSGIServer(object): """Define a WSGI Class of server""" def __init__(self, port, documents_root): # 1 \. Create socket self.server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # 2 \. Bind local information self.server_socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) self.server_socket.bind(("", port)) # 3 \. Change to listening socket self.server_socket.listen(128) self.server_socket.setblocking(False) self.client_socket_list = list() self.documents_root = documents_root def run_forever(self): """Run server""" # Waiting for the other party's link while True: # time.sleep(0.5) # for test try: new_socket, new_addr = self.server_socket.accept() except Exception as ret: print("-----1----", ret) # for test else: new_socket.setblocking(False) self.client_socket_list.append(new_socket) for client_socket in self.client_socket_list: try: request = client_socket.recv(1024).decode('utf-8') except Exception as ret: print("------2----", ret) # for test else: if request: self.deal_with_request(request, client_socket) else: client_socket.close() self.client_socket_list.remove(client_socket) print(self.client_socket_list) def deal_with_request(self, request, client_socket): """For this browser server""" if not request: return request_lines = request.splitlines() for i, line in enumerate(request_lines): print(i, line) # Extract the requested file (index.html) # GET /a/b/c/d/e/index.html HTTP/1.1 ret = re.match(r"([^/]*)([^ ]+)", request_lines[0]) if ret: print("Regular extraction data:", ret.group(1)) print("Regular extraction data:", ret.
Link to the original text: be the most professional and knowledgeable Python developer communication platform, and provide the development learning resources you need most. We focus on the study and exchange of Python development technology. We insist that we make a small step every day and a big step in life! Pay attention to [Python developer exchange platform], and learn and progress with us.