
Web front end - Vue.js essential framework (4)
Calculation properties:
<div id="aaa"> {{ message.split('').reverse().join('') }} </div>
<div id="b"> <p>dashu: "{{ aaaa}}"</p> <p>dashu: "{{ ddd}}"</p> </div> var vm = new Vue({ el: '#b', data: { aaaa: 'Hello' }, computed: { ddd: function () { return this.message.split('').reverse().join('') } } })
<p>{{ rMessage() }}</p> methods: { rMessage: function () { return this.message.split('').reverse().join('') } }
watch callback:
<div id="demo">{{ fullName }}</div> var vm = new Vue({ el: '#demo', data: { firstName: 'da', lastName: 'shu', fullName: 'da shu' }, watch: { firstName: function (val) { this.fullName = val + ' ' + this.lastName }, lastName: function (val) { this.fullName = this.firstName + ' ' + val } } })
var vm = new Vue({ el: '#demo', data: { firstName: 'da', lastName: 'shu' }, computed: { fullName: function () { return this.firstName + ' ' + this.lastName } } })
computed: { fullName: { // getter get: function () { return this.firstName + ' ' + this.lastName }, // setter set: function (newValue) { var names = newValue.split(' ') this.firstName = names[0] this.lastName = names[names.length - 1] } } }
Data changes, asynchronous or expensive operations:


// Binding properties <div v-bind:class="{ active: isActive }"></div> <div class="static" v-bind:class="{ active: isActive, 'text-danger': hasError }" ></div> data: { isActive: true, hasError: false }
<div v-bind:class="classObject"></div> data: { classObject: { active: true, 'text': false } }
Calculation properties:
<div v-bind:class="classObject"></div> data: { isActive: true, error: null }, computed: { classObject: function () { return { active: this.isActive && !this.error, 'text-danger': this.error && this.error.type === 'fatal' } } }
// array <div v-bind:class="[activeClass, errorClass]"></div> data: { activeClass: 'active', errorClass: 'text' }
Custom components:
Vue.component('my-component', { template: '<p class="foo">Hi</p>' }) <my-component class="boo"></my-component>
<div v-bind:style="styleObject"></div> data: { styleObject: { color: 'red', fontSize: '10px' } }
key's importance:

// Every time the key is rendered, it will be re rendered <template v-if="loginType === 'username'"> <label>Username</label> <input placeholder="username" key="username-input"> </template> <template v-else> <label>Email</label> <input placeholder="email address" key="email-input"> </template>
v-if and v-show, v-if for destruction and reconstruction, only for real rendering, v-show will render, frequent switching use of v-show.
key:
<div v-for="item in items" :key="item.id"> </div>
Method:
push(); pop(); shift(); unshift(); splice(); sort(); reverse();
Vue.set(object, key, value) Vue.set(vm.userProfile, 'age', 27) vm.$set(vm.userProfile, 'age', 27) <my-component v-for="item in items" :key="item.id"></my-component> <my-component v-for="(item, index) in items" v-bind:item="item" v-bind:index="index" v-bind:key="item.id" ></my-component>

Event modifier:
Event modifier:
.stop .prevent .capture .self .once .passive

// Click event will only trigger once <a v-on:click.once="doThis"></a>

.enter .tab .delete .esc .space .up .down .left .right
.ctrl .alt .shift .meta .left .right .middle
<input v-model="message" placeholder="dashucoding"> <p> {{ message }}</p>
Modifier:
After the. lazy input event is triggered, synchronize the value of the input box with the data
<input v-model.lazy="msg">
< input V-model. Number = "age" type = "number" > user's input value is converted to numeric type
Filter the first and last blank characters of the input < input V-model. Trim = "MSG" >
Vue.component('blog', { props: ['title'], template: '<h3>{{ title }}</h3>' })
afterword
Well, welcome to leave a message in the message area and share your experience and experience with you.
Thank you for learning today's content. If you think this article will help you, please share it with more friends. Thank you.
Author brief introduction
Da Shu, a man of science and engineering, a writer of simple books & an engineer of the whole stack, a writer with both sensibility and rationality, and an individual independent developer. I believe you can too! Reading his articles will be addictive! , to help you become a better self. Long press the QR code below to follow, welcome to share, it's better to top.
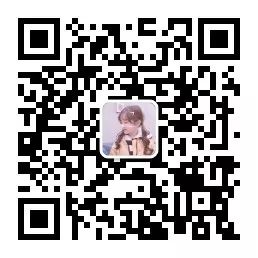