JSP:
1. instruction
*Function: used to configure JSP pages and import resource files
* format:
<% @ instruction name property name 1 = property value 1 property name 2 = property value 2...% >
* classification:
1. page: configure the
*contentType: equivalent to response.setContentType()
1. Set mime type and character set of response body
2. Set the encoding of the current jsp page (only advanced IDE can take effect. If you use low-level tools, you need to set the pageEncoding property to set the character set of the current page)
*Import: import package
*errorPage: when an exception occurs to the current page, it will automatically jump to the specified error page
*isErrorPage: identifies whether or not it is currently an error page.
*true: Yes, you can use the built-in object exception
*false: No. Default value. Built in object exception is not allowed
*General indication on error page
<%@ page contentType="text/html;charset=UTF-8" isErrorPage="true" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Server busy...</h1>
<%
String message = exception.getMessage();
out.print(message);
%>
</body>
</html>
2. include: included in the page. Import resource file for page
* <%@include file="top.jsp"%>
3. taglib: import resources
* <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
*Prefix: prefix, custom
<%@ page import="java.util.ArrayList" %>
<%@ page import="java.util.List" %>
<%@ page contentType="text/html;charset=gbk" errorPage="500.jsp" pageEncoding="GBK" language="java" buffer="16kb" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>$Title$</title>
</head>
<body>
<%
List list = new ArrayList();
int i = 3/0;
%>
</body>
</html>
2. note:
1. html comment:
<! ---- >: only html snippets can be annotated
2. jsp comment: Recommended
[% ---%]: all can be annotated
3. Built in objects
*Objects that do not need to be created and used directly in jsp pages
*There are nine:
Variable name real type action
*PageContext pagecontext the current page shares data, and you can get eight other built-in objects
*Request HttpServletRequest multiple resources (forwarding) accessed by one request
*Session HttpSession multiple requests in a session
*application ServletContext data sharing among all users
*Response HttpServletResponse response object
*Page Object the Object of the current page (Servlet)
*out JspWriter output object, data output to page
*config ServletConfig Servlet configuration object
*Exception Throwable exception object
MVC: development mode
1. jsp evolution history
1. In the early days, there were only servlet s and only response could be used to output tag data, which was very troublesome
2. Later, jsp simplifies the development of Servlet. If you overuse jsp, you can write a lot of java code in jsp, including html tables, which makes it difficult to maintain and work together
3. Later, java's web development, drawing lessons from mvc development mode, makes the program design more reasonable
2. MVC:
1. M: Model. JavaBean
*Complete specific business operations, such as querying databases and encapsulating objects
2. V: View, View. JSP
*Show data
3. C: Controller. Servlet
*Get user input
*Call model
*Give the data to the view for display
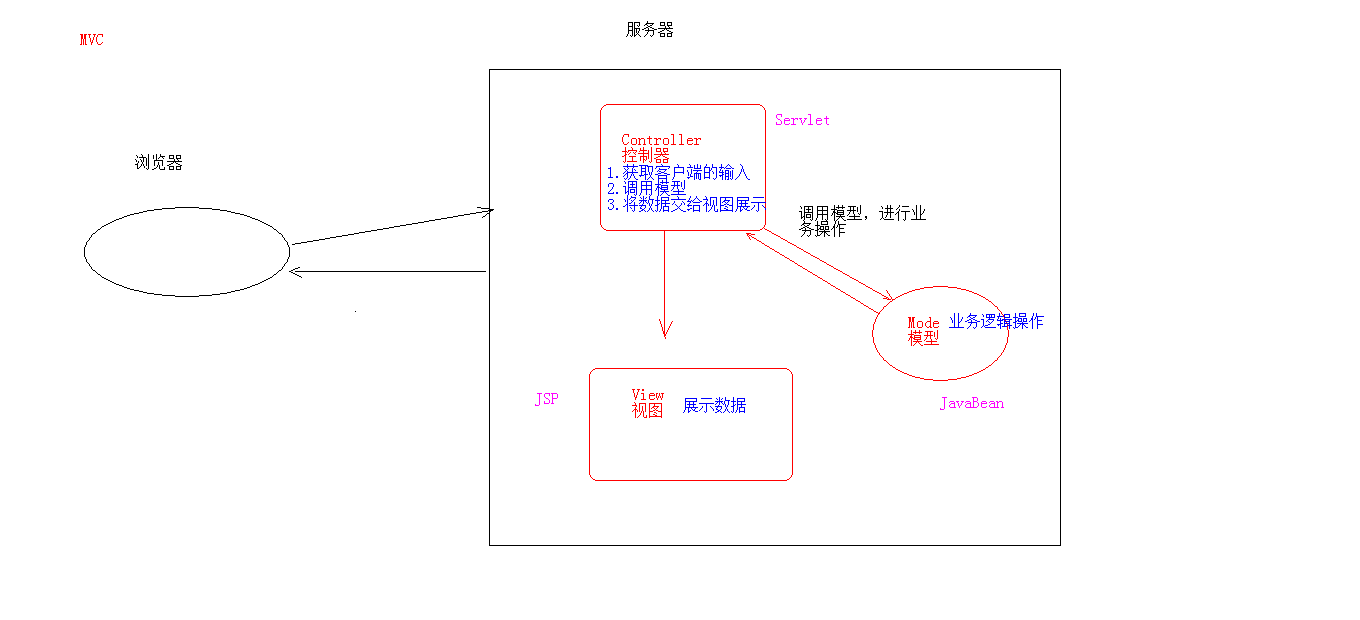
*Advantages and disadvantages:
1. advantages:
1. Low coupling, easy to maintain, and conducive to division of labor and cooperation
2. High reusability
2. disadvantages:
1. Make the project architecture complex and require high developers
EL expression
1. Concept: Expression Language
2. Function: replace and simplify the writing of java code in jsp page
3. Syntax: ${expression}
${3>4}
4. note:
*jsp supports el expression by default. If you want to ignore el expressions
1. Set: isELIgnored="true" in the page instruction of jsp to ignore all el expressions in the current jsp page
2. \ ${expression}: ignore the current el expression
5. use:
1. operations:
*Operator:
1. Arithmetic operator: + - * / (DIV)% (MOD)
2. Comparison operator: >=
3. Logical operator: & & (and) | (or)! (not)
4. Air transport operator: empty
*Function: used to judge whether the string, collection, array object is null or the length is 0
*${empty list}: judge whether the string, collection, array object is null or the length is 0
*${not empty str}: indicates whether the judgment string, collection, array object is not null and the length is > 0
```java
<%@ page import="java.util.List" %>
<%@ page import="java.util.ArrayList" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
${3 > 4}
\${3 > 4}
<hr>
<h3>Arithmetic operator </h3>
${3 + 4}<br>
${3 / 4}<br>
${3 div 4}<br>
${3 % 4}<br>
${3 mod 4}<br>
<h3>Comparison operator</h3>
${3 == 4}<br>
<h3>Logical operators</h3>
${3 > 4 && 3 < 4}<br>
${3 > 4 and 3 < 4}<br>
<h4>empty operator</h4>
<%
String str = "";
request.setAttribute("str",str);
List list = new ArrayList();
request.setAttribute("list",list);
%>
${not empty str}
${not empty list}
</body>
</html>
2. Get value
1. el Expressions can only get values from domain objects
2. Syntax:
1. ${Domain name.Key name}: Gets the value of the specified key from the specified domain
* Domain name:
1. pageScope --> pageContext
2. requestScope --> request
3. sessionScope --> session
4. applicationScope --> application(ServletContext)
* For example: in request Stored in domain name=Zhang San
* Obtain: ${requestScope.name}
2. ${Key name}: Indicates whether there is a value corresponding to the key from the smallest domain until it is found. (it can be used when the key names set in the four fields are inconsistent)
```java
<%@ page import="java.util.List" %>
<%@ page import="java.util.ArrayList" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>el Get data in domain</title>
</head>
<body>
<%
//Storing data in a domain
session.setAttribute("name","Li Si");
request.setAttribute("name","Zhang San");
session.setAttribute("age","23");
request.setAttribute("str","");
%>
<h3>el Get value</h3>
${requestScope.name}
${sessionScope.age}
${sessionScope.haha}
${name}
${sessionScope.name}
</body>
</html>
3. Get the value of object, List set and Map set
1. Object: ${domain name. Key name. Property name}
*In essence, it will call the getter method of the object
package cn.itcast.domain;
import java.text.SimpleDateFormat;
import java.util.Date;
public class User {
private String name;
private int age;
private Date birthday;
public User(String name, int age, Date birthday) {
this.name = name;
this.age = age;
this.birthday = birthday;
}
public User() {
}
public String getBirStr(){
if(birthday != null){
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
return sdf.format(birthday);
}else{
return "";
}
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
}
2. List set: ${domain name. Key name [index]}
3. Map set:
*${domain name. key name. key name}
*${domain name. Key name ["key name"]}
<%@ page import="cn.itcast.domain.User" %>
<%@ page import="java.util.*" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>el get data</title>
</head>
<body>
<%
User user = new User();
user.setName("Zhang San");
user.setAge(23);
user.setBirthday(new Date());
request.setAttribute("u",user);
List list = new ArrayList();
list.add("aaa");
list.add("bbb");
list.add(user);
request.setAttribute("list",list);
Map map = new HashMap();
map.put("sname","Li Si");
map.put("gender","male");
map.put("user",user);
request.setAttribute("map",map);
%>
<h3>el Get value in object</h3>
${requestScope.u}<br>
<%--
* Obtained by the properties of the object
* setter or getter Method, remove set or get,In the rest, the initial is lowercase.
* setName --> Name --> name
--%>
${requestScope.u.name}<br>
${u.age}<br>
${u.birthday}<br>
${u.birthday.month}<br>
${u.birStr}<br>
<h3>el Obtain List value</h3>
${list}<br>
${list[0]}<br>
${list[1]}<br>
${list[10]}<br>
${list[2].name}
<h3>el Obtain Map value</h3>
${map.gender}<br>
${map["gender"]}<br>
${map.user.name}
</body>
</html>
3. Implicit object:
*11 implicit objects in el expression
* pageContext:
*Get eight other jsp built-in objects
*${pageContext.request.contextPath}: get virtual directory dynamically
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>el Implicit object</title>
</head>
<body>
${pageContext.request}<br>
<h4>stay jsp Page dynamic access to virtual directory</h4>
${pageContext.request.contextPath}
<%
%>
</body>
</html>
JSTL
1. Concept: JavaServer pages tag library JSP standard tag library
*Is an open source free jsp tag provided by Apache organization < tag >
2. Function: used to simplify and replace java code on jsp page
3. Operation steps:
1. Import jstl related jar package
2. Import tag library: taglib instruction: <% @ taglib% >
3. Use label
4. Common JSTL Tags
1. if: the if statement equivalent to java code
1. properties:
*test must be attribute, accept boolean expression
*If the expression is true, the if label body content is displayed; if it is false, the label body content is not displayed
*In general, the test attribute value is used in conjunction with the el expression
2. note:
*The c:if tag has no else situation. If you want else situation, you can define a c:if tag
<%@ page import="java.util.List" %>
<%@ page import="java.util.ArrayList" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>ifLabel</title>
</head>
<body>
<%--
c:ifLabel
1. Properties:
* test Required property, acceptbooleanExpression
* If the expression istrue,DisplayifLabel body content, iffalse,The label body content is not displayed
* Normally, test Property values will be combined el Use with expressions
2. Be careful: c:ifNo labelelseSituation, wantelseIn case, you can define a c:ifLabel
--%>
<c:if test="true">
<h1>I am real....</h1>
</c:if>
<br>
<%
List list = new ArrayList();
list.add("aaaa");
request.setAttribute("list",list);
request.setAttribute("number",4);
%>
<c:if test="${not empty list}">
Ergodic set...
</c:if>
<br>
<c:if test="${number % 2 != 0}">
${number}Odd number
</c:if>
<c:if test="${number % 2 == 0}">
${number}Even numbers
</c:if>
</body>
</html>
2. choose: switch statement equivalent to java code
1. Using choose label declaration is equivalent to switch declaration
2. Using the when tag for judgment is equivalent to case
3. Using the other way tag to make other statements is equivalent to default
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
< title > choose tab < / Title >
</head>
<body>
<%--
Complete the case of number number corresponding to the day of the week
1. Store a number in the domain
2. Using the choose tag to extract the number is equivalent to the switch declaration
3. Using when tag to make digital judgment is equivalent to case
4. Declaration of the other case label is equivalent to default
--%>
<%
request.setAttribute("number",51);
%>
<c:choose>
< C: when test = "${number = = 1}" > Monday < / C: when >
< C: when test = "${number = = 2}" > Tuesday < / C: when >
< C: when test = "${number = = 3}" > Wednesday < / C: when >
< C: when test = "${number = = 4}" > Thursday < / C: when >
< C: when test = "${number = = 5}" > Friday < / C: when >
< C: when test = "${number = = 6}" > Saturday < / C: when >
< C: when test = "${number = = 7}" > Sunday < / C: when >
< C: otherwise > wrong digital input
</c:choose>
</body>
</html>
3. foreach: for statement equivalent to java code
<%@ page import="java.util.ArrayList" %>
<%@ page import="java.util.List" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>foreach Label</title>
</head>
<body>
<%--
foreach:Amount to java CodeforSentence
1. Repeat operation complete
for(int i = 0; i < 10; i ++){
}
* Properties:
begin: Start value
end: End value
var: Temporary variable
step: step
varStatus:Loop state object
index:Index of the element in the container, from0start
count:Number of cycles, from1start
2. Traversal container
List<User> list;
for(User user : list){
}
* Properties:
items:Container object
var:Temporary variables for elements in the container
varStatus:Loop state object
index:Index of the element in the container, from0start
count:Number of cycles, from1start
--%>
<c:forEach begin="1" end="10" var="i" step="2" varStatus="s">
${i} <h3>${s.index}<h3> <h4> ${s.count} </h4><br>
</c:forEach>
<hr>
<%
List list = new ArrayList();
list.add("aaa");
list.add("bbb");
list.add("ccc");
request.setAttribute("list",list);
%>
<c:forEach items="${list}" var="str" varStatus="s">
${s.index} ${s.count} ${str}<br>
</c:forEach>
</body>
</html>
Three layer architecture: software design architecture
1. Interface layer (presentation layer): the user can see the interface. Users can interact with the server through the components on the interface
2. Business logic layer: it deals with business logic.
3. Data access layer: operate data storage files.
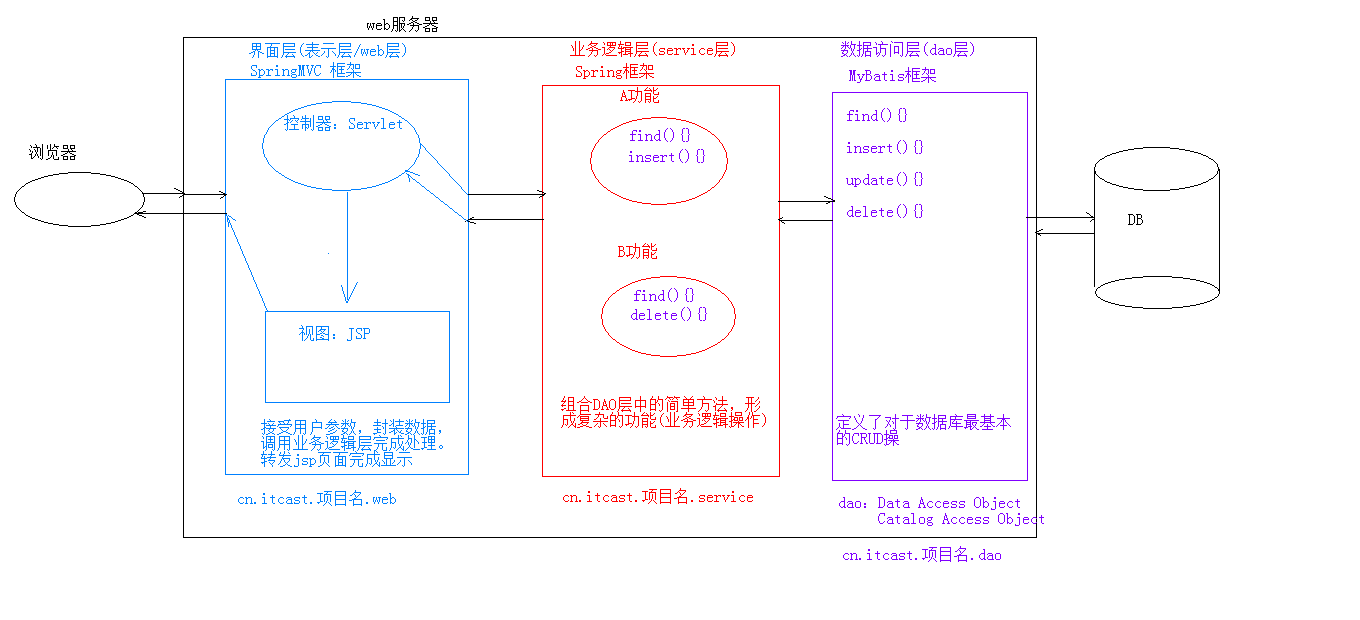