A download tool based on RxJava to support multi-threading and breakpoint continuation
Project address
Main functions:
- Use Retrofit+OKHTTP for network requests
- Build on RxJava to support chain invocation of various RxJava operators
- Continuous transmission of breakpoints, according to the response value of the server to automatically determine whether to support discontinuous transmission of breakpoints
- If discontinuous transmission is not supported, traditional download will be carried out.
- Multithread download, you can set the maximum thread, the default value is 3
- Automatic attempt to reconnect was detected when network connection failure was detected, and the maximum number of retries could be configured with a default value of 3.
- Support for judging server-side file changes based on Last-Modified fields
- In the process of validating with the server, only the response header can be obtained by using the lighter HEAD request mode, which reduces the burden on the server side.
Usage mode
1. Adding Gradle dependencies
dependencies{ compile 'zlc.season:rxdownload:1.1.0' }
2. Code calls
Subscription subscription = RxDownload.getInstance() .download(url, "weixin.apk", null) .subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) .subscribe(new Subscriber<DownloadStatus>() { @Override public void onCompleted() { } @Override public void onError(Throwable e) { } @Override public void onNext(final DownloadStatus status) { } });
download(String url, String saveName, String savePath) parameter description:
The parameters are download address, file name and address.
url and saveName are required parameters, savePath is optional parameters, and the default download address is / storage/emulated/0/Download / directory, which is the download directory with built-in storage.
3. Parameter Configuration
The configurable parameters are as follows:
Subscription subscription = RxDownload.getInstance() .maxThread(10) //Setting Maximum Threads .maxRetryCount(10) //Set the number of retries for download failures .retrofit(myRetrofit)//If you need your own retrofit client, you can specify it here .defaultSavePath(defaultSavePath)//Set the default download path .download(url,savename,savepath) //Start downloading .subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) .subscribe(new Subscriber<DownloadStatus>() { @Override public void onCompleted() { } @Override public void onError(Throwable e) { } @Override public void onNext(DownloadStatus status) { //Status represents the current download progress } });
4. Download Status download status
class DownloadStatus { private long totalSize; private long downloadSize; public boolean isChunked = false; //... //Returns the total file size in byte public long getTotalSize() {} //Returns the total downloaded size in byte public long getDownloadSize() {} //Returns the total size of the format, such as: 10MB public String getFormatTotalSize() {} //Returns the formatted downloaded size, such as: 5KB public String getFormatDownloadSize() {} //Returns a formatted status string, such as: 2MB/36MB public String getFormatStatusString() { } //Return the percentage of downloads, leaving two decimal places, such as 5.25% public String getPercent() {} }
5. Cancel or suspend Downloads
Subscription subscription = RxDownload.getInstance() .download(url, null, null) //... //Cancel subscription, you can suspend download, if the server does not support breakpoint continuation, the next download will be re-downloaded, otherwise continue to download. if (subscription != null && !subscription.isUnsubscribed()) { subscription.unsubscribe(); }
6. More functions will be gradually improved in the future.
If you have any questions about this project, please come to issues.
Last
In the current interview season, I have collected a lot of information on the Internet and made documents and architectures for free to share with you [including advanced UI, performance optimization, architect courses, NDK, Kotlin, React Native + Weex, Flutter and other architectures technical information]. I hope to help you review before the interview and find a good job. It also saves time for people to search for information on the Internet.
Data acquisition method: join Android framework to exchange QQ group chat: 513088520, get information when you enter the group!!!
Click on the link to join the group chat [Android Mobile Architecture Group]: Join group chat
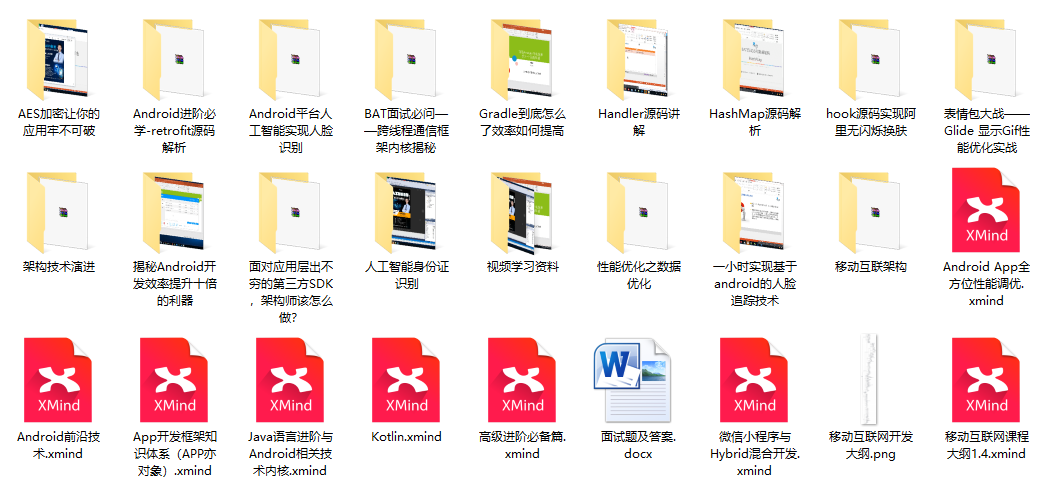