RxRouter
A Lightweight, Simple, Intelligent and Powerful Android Routing Library
Getting started
Add dependency
Add the following dependencies to the build.gradle file:
dependencies { implementation 'zlc.season:rxrouter:x.y.z' annotationProcessor 'zlc.season:rxrouter-compiler:x.y.z' }
(Replace the x, y and z above with the latest version number)
If Kotlin is used, replace annotation Processor with kapt
Hello World
First, add the @Url annotation to Activeness that we need to route:
@Url("this is a url") class UrlActivity : AppCompatActivity() { ... }
Then create a class annotated with @Router to tell RxRouter that there is a router:
@Router class MainRouter{ }
This class does not need any other code. RxRouter automatically generates a RouterProvider based on the class name of this class. For example, MainRouter here will generate MainRouterProvider.
Then we need to add these routers to RxRouter Providers:
class CustomApplication : Application() { override fun onCreate() { super.onCreate() RxRouterProviders.add(MainRouterProvider()) } }
Finally, we can begin our performance:
RxRouter.of(context) .route("this is a uri") .subscribe()
Parameter passing
Carry parameter jump:
With the with method, you can add a series of parameters to this routing.
RxRouter.of(context) .with(10) //int value .with(true) //boolean value .with(20.12) //double value .with("this is a string value") //string value .with(Bundle()) //Bundle value .route("this is a uri") .subscribe()
The onActivityResult method is no longer needed
Want to get the value returned by the jump? No more writing a bunch of on Activity Result methods!
RxRouter.of(context) .with(false) .with(2000) .with(9999999999999999) .route("this is a uri") .subscribe { if (it.resultCode == Activity.RESULT_OK) { val intent = it.data val stringResult = intent.getStringExtra("result") result_text.text = stringResult stringResult.toast() } }
If the result is returned, it will be processed in subscribe.
Class jump
Don't want to use Url annotations? No problem, RxRouter also supports the original jump of specified class names in the same way as URL jumps:
RxRouter.of(context) .routeClass(ClassForResultActivity::class.java) .subscribe{ if (it.resultCode == Activity.RESULT_OK) { val intent = it.data val stringResult = intent.getStringExtra("result") result_text.text = stringResult stringResult.toast() } }
Action jump
Similarly, RxRouter also supports Actions and custom Actions jumps.
Custom Action Jump:
<activity android:name=".ActionActivity"> <intent-filter> <action android:name="zlc.season.sample.action" /> <category android:name="android.intent.category.DEFAULT" /> </intent-filter> </activity>
RxRouter.of(context) .routeAction("zlc.season.sample.action") .subscribe({ "no result".toast() }, { it.message?.toast() })
System Action Jump:
//Call up RxRouter.of(this) .addUri(Uri.parse("tel:123456")) .routeSystemAction(Intent.ACTION_DIAL) .subscribe() //Sending SMS val bundle = Bundle() bundle.putString("sms_body", "This is information content.") RxRouter.of(this) .addUri(Uri.parse("smsto:10086")) .with(bundle) .routeSystemAction(Intent.ACTION_SENDTO) .subscribe()
firewall
RxRouter has a small and powerful firewall that can be intercepted according to firewall rules before routing. You can add one or more firewalls.
//Create a LoginFirewall class LoginFirewall : Firewall { override fun allow(datagram: Datagram): Boolean { if (notLogin) { "You haven't logged in yet. Please log in first.".toast() return false } return true } } //Adding Firewall to Routing RxRouter.of(this) .addFirewall(LoginFirewall()) .route("this is a url") .subscribe()
Last
In the current interview season, I have collected a lot of information on the Internet and made documents and architectures for free to share with you [including advanced UI, performance optimization, architect courses, NDK, Kotlin, React Native + Weex, Flutter and other architectures technical information]. I hope to help you review before the interview and find a good job. It also saves time for people to search for information on the Internet.
Data acquisition method: join Android framework to exchange QQ group chat: 513088520, get information when you enter the group!!!
Click on the link to join the group chat [Android Mobile Architecture Group]: Join group chat
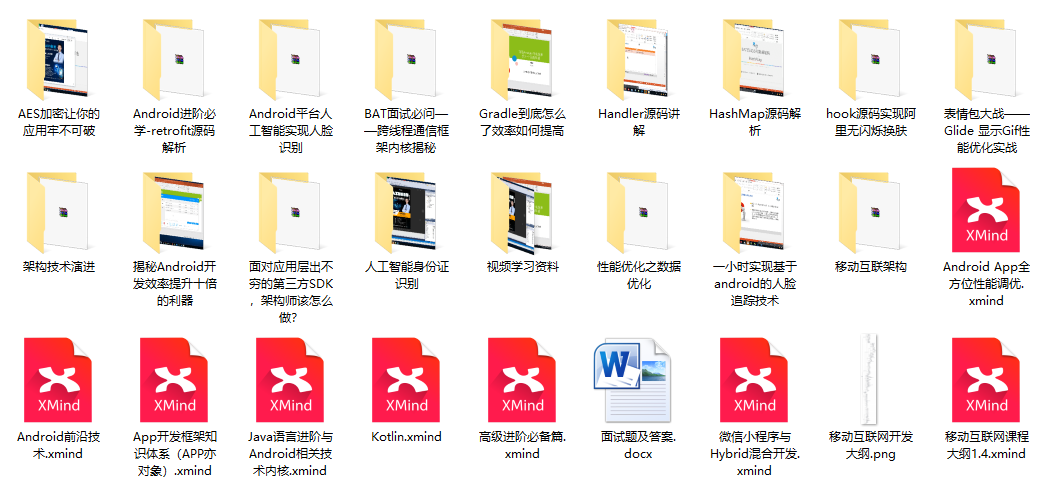