First, let us have a global understanding, direct to the last project, to see that only through these lines of code, can we complete such a powerful function. In the next part, I will combine API to explain why I write this way carefully.
Design sketch:
The sliding between three view s is realized.
The first VIEW slides to the second VIEW and the second VIEW slides to the third VIEW.
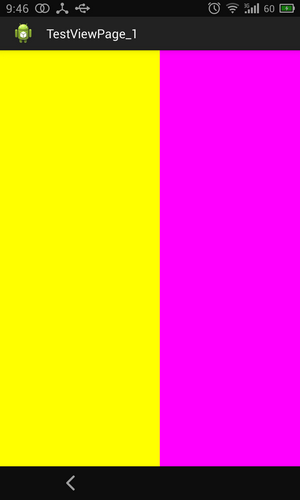
1. New project, introducing ViewPager control
ViewPager. It is a class of additional packages in google SDk, which can be used to switch between screens.
1. Add in the main layout file
-
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
xmlns:tools="http://schemas.android.com/tools"
-
android:layout_width="fill_parent"
-
android:layout_height="fill_parent"
-
tools:context="com.example.testviewpage_1.MainActivity" >
-
-
<android.support.v4.view.ViewPager
-
android:id="@+id/viewpager"
-
android:layout_width="wrap_content"
-
android:layout_height="wrap_content"
-
android:layout_gravity="center" />
-
-
</RelativeLayout>
< < Android Support. v4. view. ViewPager /> is the component corresponding to ViewPager, which is placed where you want to slide.
2. Create three new layout s for sliding switching views
As can be seen from the effect map, our three views are very simple. There are no controls in them. Of course, you can add various controls to them. But here is a DEMO, just explain the principle, so I just use the background to distinguish the layout without layout.
The layout codes are as follows:
layout1.xml
-
<?xml version="1.0" encoding="utf-8"?>
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent"
-
android:background="#ffffff"
-
android:orientation="vertical" >
-
-
-
</LinearLayout>
layout2.xml
-
<?xml version="1.0" encoding="utf-8"?>
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent"
-
android:background="#ffff00"
-
android:orientation="vertical" >
-
-
-
</LinearLayout>
layout3.xml
-
<?xml version="1.0" encoding="utf-8"?>
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
-
android:layout_width="match_parent"
-
android:layout_height="match_parent"
-
android:background="#ff00ff"
-
android:orientation="vertical" >
-
-
-
</LinearLayout><span style="color:#660000;">
-
</span>
II. Code Actual Warfare
First, the whole code, and then step by step.
-
package com.example.testviewpage_1;
-
-
-
-
-
import java.util.ArrayList;
-
import java.util.List;
-
import java.util.zip.Inflater;
-
-
import android.app.Activity;
-
import android.os.Bundle;
-
import android.support.v4.view.PagerAdapter;
-
import android.support.v4.view.ViewPager;
-
import android.view.LayoutInflater;
-
import android.view.View;
-
import android.view.ViewGroup;
-
-
-
public class MainActivity extends Activity {
-
-
private View view1, view2, view3;
-
private ViewPager viewPager;
-
-
private List<View> viewList;
-
-
-
@Override
-
protected void onCreate(Bundle savedInstanceState) {
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.activity_main);
-
-
viewPager = (ViewPager) findViewById(R.id.viewpager);
-
LayoutInflater inflater=getLayoutInflater();
-
view1 = inflater.inflate(R.layout.layout1, null);
-
view2 = inflater.inflate(R.layout.layout2,null);
-
view3 = inflater.inflate(R.layout.layout3, null);
-
-
viewList = new ArrayList<View>();
-
viewList.add(view1);
-
viewList.add(view2);
-
viewList.add(view3);
-
-
-
PagerAdapter pagerAdapter = new PagerAdapter() {
-
-
@Override
-
public boolean isViewFromObject(View arg0, Object arg1) {
-
-
return arg0 == arg1;
-
}
-
-
@Override
-
public int getCount() {
-
-
return viewList.size();
-
}
-
-
@Override
-
public void destroyItem(ViewGroup container, int position,
-
Object object) {
-
-
container.removeView(viewList.get(position));
-
}
-
-
@Override
-
public Object instantiateItem(ViewGroup container, int position) {
-
-
container.addView(viewList.get(position));
-
-
-
return viewList.get(position);
-
}
-
};
-
-
-
viewPager.setAdapter(pagerAdapter);
-
-
}
-
-
-
}
The amount of code is very small, all in the OnCreate() function.
1. Look first at the meaning of the declared variable:
-
private View view1, view2, view3;
-
private List<View> viewList;
-
private ViewPager viewPager;
First viewPager corresponds to the <android.support.v4.view.ViewPager/> control.
View1, view2, and view3 correspond to our three layouts: layout1. xml, layout2. xml, and layout3. xml.
The viewList is a View array that dresses up the three VIEW above.
2. Next is their initial process:
-
viewPager = (ViewPager) findViewById(R.id.viewpager);
-
LayoutInflater inflater=getLayoutInflater();
-
view1 = inflater.inflate(R.layout.layout1, null);
-
view2 = inflater.inflate(R.layout.layout2,null);
-
view3 = inflater.inflate(R.layout.layout3, null);
-
-
viewList = new ArrayList<View>();
-
viewList.add(view1);
-
viewList.add(view2);
-
viewList.add(view3);
Initialization process is not very difficult, that is, to link resources and variables layout, and finally to instantiate view1,view2,view3 added to the viewList
3. PageAdapter - PageView's adapter
The adapter must be something that everyone can't live without. There are adapters in ListView. ListView obtains the Item to load by rewriting the GetView () function. PageAdapter is not the same, after all, PageAdapter is a collection of individual VIew s.
PageAdapter must rewrite four functions:
- boolean isViewFromObject(View arg0, Object arg1)
- int getCount()
- void destroyItem(ViewGroup container, int position,Object object)
- Object instantiateItem(ViewGroup container, int position)
Let's look at the functions first. What have we done above?
-
@Override
-
public int getCount() {
-
-
return viewList.size();
-
}
getCount(): Returns the number of VIew s to slide
-
@Override
-
public void destroyItem(ViewGroup container, int position,
-
Object object) {
-
-
container.removeView(viewList.get(position));
-
}
destroyItem (): Delete the View at the specified position from the current container;
-
@Override
-
public Object instantiateItem(ViewGroup container, int position) {
-
-
container.addView(viewList.get(position));
-
-
-
return viewList.get(position);
-
}
-
};
instantiateItem(): Two things are done. First, add the current View to the container, and second, return to the current View.
-
@Override
-
public boolean isViewFromObject(View arg0, Object arg1) {
-
-
return arg0 == arg1;
-
}
isViewFromObject(): Let's not explain this function first. Now you know that it needs to be rewritten like this. We'll rewrite it later.