Suppose you are developing a logistics management application. The original version could only handle truck transportation, so most of the code was in a class called truck.
After a while, this app became extremely popular. You can receive more than ten requests from shipping companies every day, hoping that the application can support maritime logistics functions.
If the rest of the code is already coupled to an existing class, adding a new class to the program is not that easy.
How to deal with code problems? At present, most of the codes are related to the truck class. Adding a ship class to the program requires modifying all the codes. Worse, if you need to support another mode of transportation in your program in the future, you may need to make significant changes to these codes again.
Finally, you will have to write complex code to deal with different transportation object classes in the application.
The factory method pattern recommends using special factory methods instead of direct calls to object constructors (that is, using the new operator). Don't worry, the object will still be created through the new operator, but the operator is changed to the factory method. The objects returned by factory methods are often referred to as "products".
Subclasses can modify the object types returned by factory methods.
At first glance, this change may be pointless: we just changed the location of the calling function in the program. However, think about it carefully. Now you can override the factory method in a subclass to change the type of product it creates.
However, it should be noted that only when these products have a common base class or interface can subclasses return products of different types. At the same time, the factory method in the base class should also declare its return type as this common interface.
All products must use the same interface.
For example, both Truck and Ship classes must implement the Transport interface, which declares a method called deliver.
Each class will implement the method in different ways: trucks deliver goods by land and ships deliver goods by Sea. Land transportation Road The factory method in the Logistics class returns the truck object, while the Sea transport The Logistics class returns the ship object.
As long as the product class implements a common interface, you can pass its objects to the customer code without providing additional data.
Code that calls factory methods (often referred to as_ Client_ Code) there is no need to understand the difference between the actual objects returned by different subclasses. The client treats all products as abstract transportation. The client knows that all transport objects provide delivery methods, but does not care about their specific implementation.
-
The Product will declare the interface. These interfaces are common to all objects built by creators and their subclasses.
-
Concrete Products are different implementations of product interfaces.
-
The Creator class declares a factory method that returns a product object. The return object type of the method must match the product interface.
You can declare a factory method as an abstract method, forcing each subclass to implement the method in a different way. Alternatively, you can return the default product type in the base factory method.
Note that although its name is the creator, its main responsibility is not to create the product. In general, the creator class contains some core business logic related to the product. Factory methods separate these logical processes from specific product classes. For example, a large software development company has a programmer training department. However, the main job of these companies is to write code, not production programmers.
-
Concrete Creators will override the underlying factory method to return different types of products.
Note that a new instance is not necessarily created every time a factory method is called. Factory methods can also return existing objects from caches, object pools, or other sources.
General product interface
package com.yitiao.demo.factoryMethod; /** * @Author: One IT * @Date: 2021/6/8 22:46 */ public interface Transport { void deliver(); }
Specific products
package com.yitiao.demo.factoryMethod; /** * @Author: One IT * @Date: 2021/6/8 23:00 */ public class Ship implements Transport { @Override public void deliver() { System.out.println("Ship"); } }
package com.yitiao.demo.factoryMethod;
/**
-
@Author: an IT
-
@Date: 2021/6/8 23:00
*/
public class Truck implements Transport {
@Override public void deliver() { System.out.println("Truck"); }
}
### [] () foundation Creator
package com.yitiao.demo.factoryMethod;
/**
-
@Author: an IT
-
@Date: 2021/6/8 23:07
*/
public abstract class Logistics {
public abstract Transport createTransprot();
}
### [] () specific Creator
package com.yitiao.demo.factoryMethod;
/**
-
@Author: an IT
-
@Date: 2021/6/8 23:13
*/
public class RoadLogistics extends Logistics{
@Override public Transport createTransprot() { return new Ship(); }
}
package com.yitiao.demo.factoryMethod; /** * @Author: One IT * @Date: 2021/6/8 23:14 */ public class SeaLogistics extends Logistics { @Override public Transport createTransprot() { return new Truck(); } } ``` ### [] () client ``` package com.yitiao.demo.factoryMethod; /** * @Author: One IT * @Date: 2021/6/8 23:15 */ public class Test { public static void main(String[] args) { Logistics roadLogistics = new RoadLogistics(); roadLogistics.createTransprot().deliver(); Logistics seaLogistics = new SeaLogistics(); seaLogistics.createTransprot().deliver(); } } ``` 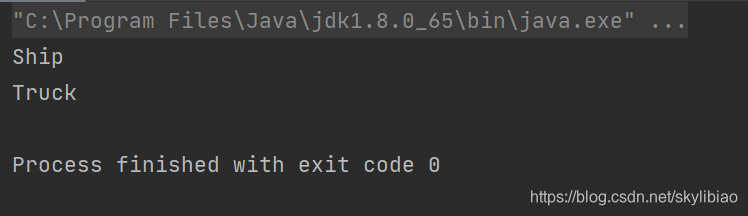 []( )Java Application in -------------------------------------------------------------------- # last According to the above process, four months is just right. of course Java The system is huge, and there are many more advanced skills to master, but don't worry. These can be used and learned in future work. Learning programming is a process from chaos to order, so if you encounter some knowledge points you can't understand at the moment in the learning process, you don't have to be depressed and don't be discouraged. This is normal and can't be normal anymore. It's just a temporary process of "people share the same heart and reason". "**The road is tortuous and the future is bright**!" 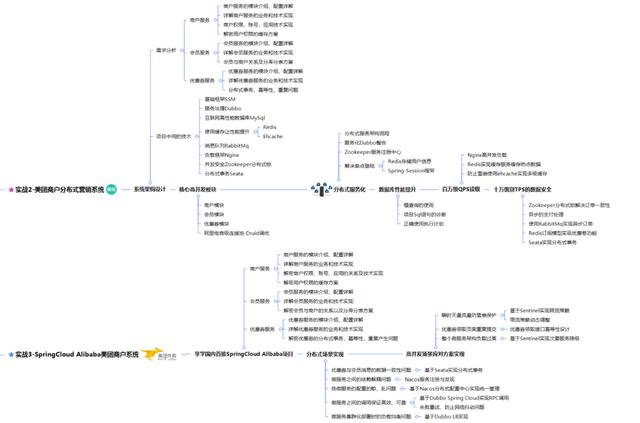 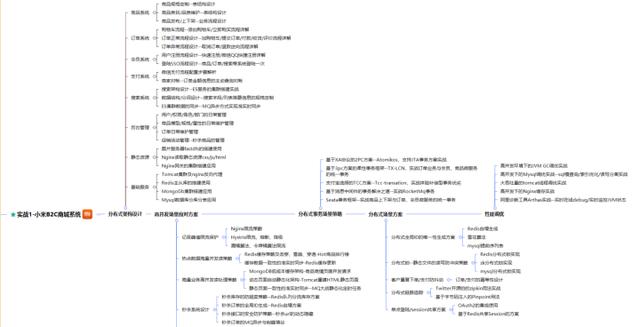 use -------------------------------------------------------------------- # last According to the above process, four months is just right. of course Java The system is huge, and there are many more advanced skills to master, but don't worry. These can be used and learned in future work. Learning programming is a process from chaos to order, so if you encounter some knowledge points you can't understand at the moment in the learning process, you don't have to be depressed and don't be discouraged. This is normal and can't be normal anymore. It's just a temporary process of "people share the same heart and reason". "**The road is tortuous and the future is bright**!" [External chain picture transfer...(img-OB5bIw0V-1630930141109)] [External chain picture transfer...(img-7FQEXBED-1630930141112)] **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)**