Write in front
the project is designed for front-end and back-end separation, using Nginx as the front-end resource server, and realizing the reverse proxy of the back-end service. The background is Java Web project, which uses Tomcat to deploy services.
- Front end framework: bootstrap 4, Vue.js2
- Background framework: spring boot, spring data JPA
- Development tools: IntelliJ IDEA, Maven
Effect:
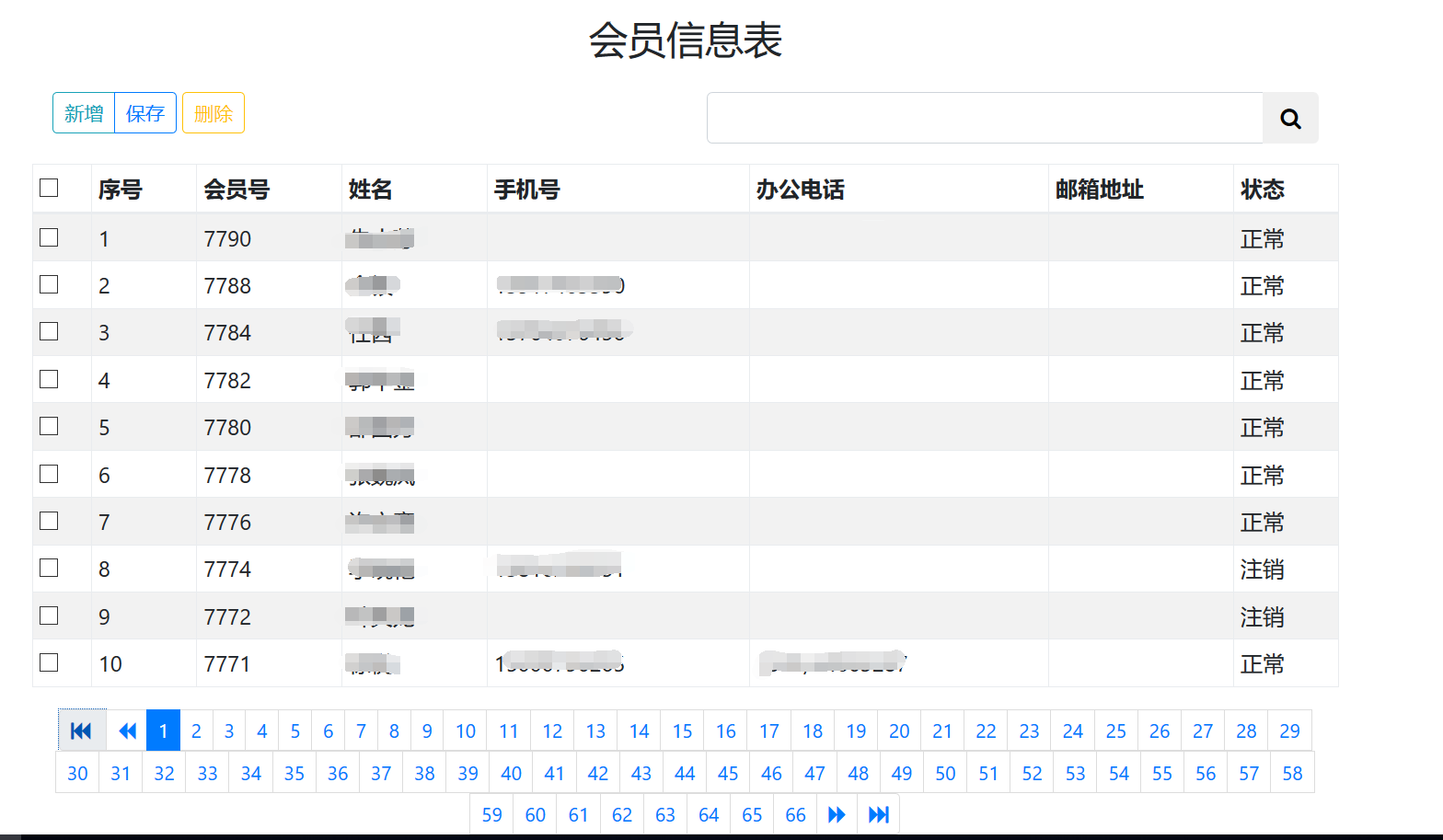
How to use Bootstrap+Vue to implement dynamic table, data addition and deletion, etc. Please check Using Bootstrap + Vue.js to dynamically display, add and delete tables . After that, the topic of this article begins.
1, Using Bootstrap to build tables
- Table area
<div class="row"> <table class="table table-hover table-striped table-bordered table-sm"> <thead class=""> <tr> <th><input type="checkbox"></th> <th>Serial number</th> <th>Member ID</th> <th>Full name</th> <th>cell-phone number</th> <th>Office phone</th> <th>e-mail address</th> <th>state</th> </tr> </thead> <tbody> <tr v-for="(user,index) in userList"> <td><input type="checkbox" :value="index" v-model="checkedRows"></td> <td>{{pageNow*10 + index+1}}</td> <td>{{user.id}}</td> <td>{{user.username}}</td> <td>{{user.mobile}}</td> <td>{{user.officetel}}</td> <td>{{user.email}}</td> <td v-if="user.disenable == 0">normal</td> <td v-else>Cancellation</td> </tr> </tbody> </table> </div>
- Pagination area
<div class="row mx-auto"> <ul class="nav justify-content-center pagination-sm"> <li class="page-item"> <a href="#" class="page-link"><i class="fa fa-fast-backward" @click="switchToPage(0)"> </i></a> </li> <li class="page-item"> <a href="#" class="page-link"><i class="fa fa-backward" @click="switchToPage(pageNow-1)"></i></a> </li> <li class="page-item" v-for="n in totalPages" :class="{active:n==pageNow+1}"> <a href="#" @click="switchToPage(n-1)" class="page-link">{{n}}</a> </li> <li class="page-item"> <a href="#" class="page-link"><i class="fa fa-forward" @click="switchToPage(pageNow+1)"></i></a> </li> <li class="page-item"> <a href="#" class="page-link"><i class="fa fa-fast-forward" @click="switchToPage(totalPages-1)"></i></a> </li> </ul> </div>
2, Initializing Vue objects and data
- Creating Vue objects
var vueApp = new Vue({ el:"#vueApp", data:{ userList:[], perPage:10, pageNow:0, totalPages:0, checkedRows:[] }, methods:{ switchToPage:function (pageNo) { if (pageNo < 0 || pageNo >= this.totalPages){ return false; } getUserByPage(pageNo); } } });
- Initialization data
function getUserByPage(pageNow) { $.ajax({ url:"/user/"+pageNow, success:function (datas) { vueApp.userList = datas.content; vueApp.totalPages = datas.totalPages; vueApp.pageNow = pageNow; }, error:function (res) { console.log(res); } }); }
Complete js code:
<script> var vueApp = new Vue({ el:"#vueApp", data:{ userList:[], perPage:10, pageNow:0, totalPages:0, checkedRows:[] }, methods:{ switchToPage:function (pageNo) { if (pageNo < 0 || pageNo >= this.totalPages){ return false; } getUserByPage(pageNo); } } }); getUserByPage(0); function getUserByPage(pageNow) { $.ajax({ url:"/user/"+pageNow, success:function (datas) { vueApp.userList = datas.content; vueApp.totalPages = datas.totalPages; vueApp.pageNow = pageNow; }, error:function (res) { console.log(res); } }); } </script>
3, Using JPA to realize paging query
- controller receives request
/** * User related request controller * @author louie * @date 2017-12-19 */ @RestController @RequestMapping("/user") public class UserController { @Autowired private UserService userService; /** * Paging get users * @param pageNow Current page number * @return Paging user data */ @RequestMapping("/{pageNow}") public Page<User> findByPage(@PathVariable Integer pageNow){ return userService.findUserPaging(pageNow); } }
- JPA paging query
@Service public class UserServiceImpl implements UserService { @Value("${self.louie.per-page}") private Integer perPage; @Autowired private UserRepository userRepository; @Override public Page<User> findUserPaging(Integer pageNow) { Pageable pageable = new PageRequest(pageNow,perPage,Sort.Direction.DESC,"id"); return userRepository.findAll(pageable); } }
OK, now that the function is complete, the project code has been shared in GitHub, you can Click to view or download , embrace open source and share to make the world better.