Switching pages is the most basic function of app. This function needs to be implemented with Navigation components.
RN is developing too fast (v49). The Navigation component that came with it is abandoned. If it is only for ios, it can also be used NavigatorIOS
There are some good ones in the community
https://github.com/react-community/react-navigation
https://github.com/wix/react-native-navigation
https://github.com/happypancake/react-native-tab-navigator
Taking react native tab navigator as an example, it is easy to achieve the following tab switching effects:
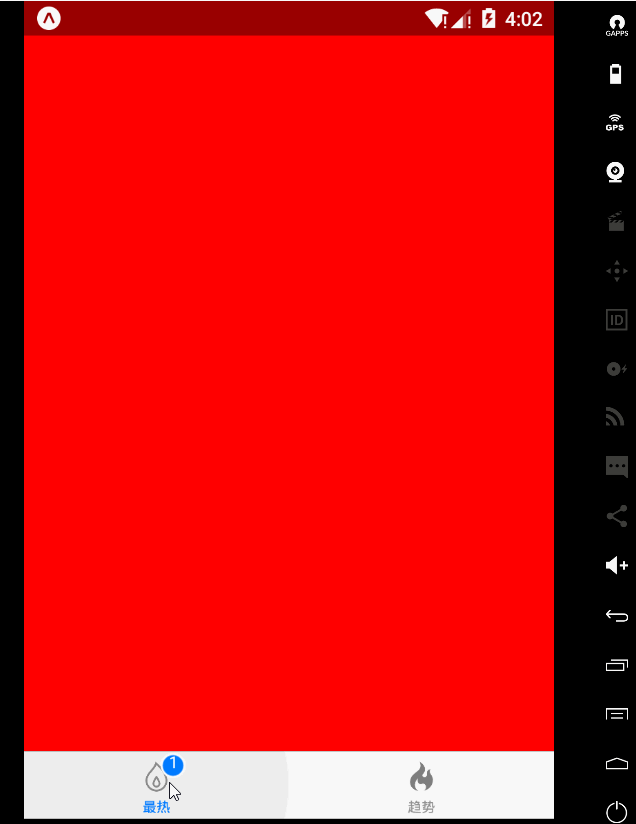
The complete code is as follows, which is to adjust the example in the document slightly.
import React from 'react'; import TabNavigator from 'react-native-tab-navigator'; import { StyleSheet, Text, Button, TextInput, View, Alert, Image } from 'react-native'; export default class App extends React.Component { constructor(props) { super(props); this.state = {selectedTab: 'home'}; } render() { return ( <View style={styles.container}> <TabNavigator> <TabNavigator.Item selected={this.state.selectedTab === 'home'} title="Hottest" renderIcon={() => <Image style={styles.image} source={require('./res/images/ic_polular.png')} />} renderSelectedIcon={() => <Image style={styles.image} source={require('./res/images/ic_polular.png')} />} badgeText="1" onPress={() => this.setState({ selectedTab: 'home' })}> <View style={styles.page1}></View> </TabNavigator.Item> <TabNavigator.Item selected={this.state.selectedTab === 'profile'} title="trend" renderIcon={() => <Image style={styles.image} source={require('./res/images/ic_trending.png')} />} renderSelectedIcon={() => <Image style={styles.image} source={require('./res/images/ic_trending.png')} />} onPress={() => this.setState({ selectedTab: 'profile' })}> <View style={styles.page2}></View> </TabNavigator.Item> </TabNavigator> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#F5FCFF', }, page1: { flex: 1, backgroundColor: 'red' }, page2: { flex: 1, backgroundColor: 'yellow' }, image: { height: 22, width: 22 } });
By default, a tab named home is selected. Click to switch.
Style elements through StyleSheet.
Note:
- Do not set the unit of size. In RN, the size is independent of the equipment.
- The picture is transparent png, which can be found here download
- For example, the size of ic_polar.png is 5757, and the size of ic_polar @ 2x.png is 114114. Only the most basic ic_polar.png is introduced. As long as the naming rules are followed in different devices, it will automatically adapt (to be verified).
image: { height: 22, width: 22 }