csdn blog: http://blog.csdn.net/hjjdehao
I. Basic introduction of EventBus 3.0:
Previously, Android components used to communicate with each other using Intent or Broadcast. EventBus greatly simplifies the communication between components, components and background threads in the application.
ThreadMode: This is an enumeration with four values that determine which thread the subscription function is executed on.
PostThread: The event sender executes in which thread. This is the default value.
MainThread: The subscription function must be executed on the main thread. The same onEventMainThread method
BackgroundThread: If an event is emitted from a sub-thread, the subscription function executes on that sub-thread, and no new sub-thread is created; if the main thread emits an event, a sub-thread is created. The same onEventBackgroundThread method
Async: Make sure you create subthreads. The same onEventAsync method.
sticky: The default is false. If true, when an event is sent through postSticky, the last event of this type of event is cached, and when a subscriber registers, the previously cached event is sent directly to it. For example, when the event sender starts first and the subscriber does not start yet.
Priority: The default value is 0. Subscribe to the subscription function for the same event, and receive the priority of the event on the premise that the ThreadMode value is the same.
II. Actual Operation
Of course, the first step is to configure the environment:
The configuration in build.gradle is as follows
buildscript { dependencies { classpath 'com.neenbedankt.gradle.plugins:android-apt:1.8' } } apply plugin: 'com.neenbedankt.android-apt' dependencies { compile 'org.greenrobot:eventbus:3.0.0' apt 'org.greenrobot:eventbus-annotation-processor:3.0.1' } apt { arguments { eventBusIndex "com.whoislcj.eventbus.MyEventBusIndex" } }
Let's take a look at the rendering first.
post sending
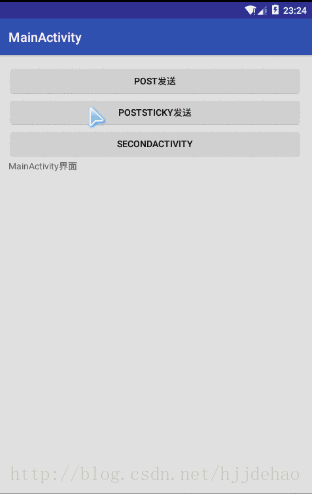
PosSticky Send
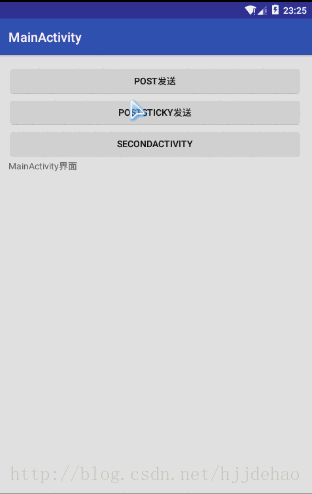
As can be seen from the effect chart, the Second Activity can not receive the message sent by MainActivity except that it can receive the information itself. This is because Second Activity did not register events at the time of posting, and naturally no information was received. With postSticky to send events, both MainActivity and EcondActivity can receive messages sent.
To sum up, you can see the difference between post and postSticky is that post is to send events directly, provided you have registered events before posting. PosSticky, on the other hand, caches events when they are sent, and triggers them when an event with a registered sticky occurs. If you don't understand, take a closer look at the code.
Note: When registering, try to register with onCreate or onStart method, and cancel the registration on onDestroy.
Code example:
public class MainActivity extends AppCompatActivity { TextView mTextView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mTextView= (TextView) findViewById(R.id.tv); EventBus.getDefault().register(this); } public void post(View view){ EventBus.getDefault().post("stay MainActivity Use post(Direct release)"); } public void postSticky(View view){ EventBus.getDefault().postSticky("stay MainActivity Use postSticky(Stay released)"); } public void second(View view){ Intent intent = new Intent(MainActivity.this,SecondActivity.class); startActivity(intent); } @Subscribe(threadMode = ThreadMode.MAIN) public void onEvent(String msg){ mTextView.setText(msg); } @Override protected void onDestroy() { super.onDestroy(); EventBus.getDefault().unregister(this); }
public class SecondActivity extends AppCompatActivity { private TextView mTextView; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); mTextView = (TextView) findViewById(R.id.show); EventBus.getDefault().register(this); } public void post(View view) { EventBus.getDefault().post("stay SecondActivity Use post(Direct release)"); } public void postSticky(View view) { EventBus.getDefault().postSticky("stay SecondActivity Use postSticky(Stay released)"); } public void back(View view) { Intent intent = new Intent(SecondActivity.this,MainActivity.class); startActivity(intent); } @Subscribe(threadMode = ThreadMode.MAIN,sticky = true) public void onEvent(String msg){ mTextView.setText(msg); } @Override protected void onDestroy() { super.onDestroy(); EventBus.getDefault().unregister(this); } }
Summary
To ensure that messages are received in EcondActivity. MainActivity and EcondActivity open mode is singleTask or singleInstancce, make sure to go back and see that mainActivity is the same instance. To receive a message. (Other demo s return messages by killing their own activity through finish es, returning directly to the previous MainActivity, or guaranteeing that they are the same sample object ~)
I have shown the operation on MainActivity, and the same is true for the operation on SENDActivity. I will try it myself and leave a message under the comments that are inadequate or questionable.
In practical projects, registers and unregister s are usually related to the life cycle of activities and fragments. ThreadMode.MainThread can solve the problem that Android interface refresh must be done in UI threads without callback. Handler is used to transfer after no callback. Viscous events can solve the problem of simultaneous execution of Posts and registers. The asynchronization problem and the transmission of events do not consume the performance of serialization and deserialization, which is enough to meet the communication requirements between modules in most of our cases.
This article only knows what it is, but it does not know why it is. If you want to know why, you have to go deep into the source code to understand.