Preface:
I wrote an earlier article "Creating windows Services with C_#", https://www.cnblogs.com/huangwei1992/p/9693167.html, and then a blogger recommended an open source framework Topshelf to me.
Writing a bit of test code, I found that the Topshell framework is really very useful in creating windows services, so I modified my previous code.
Development process:
1. Without using the Topshell framework, we need to create a Windows service program, where we just need to create a console program.
2. Adding references
Use the Program Installation Command:
- Install-Package Topshelf
Search Topshelf directly in NuGet Package Manager and click Installation:
3. New core class CloudImage Manager
There are three main methods: LoadCloudImage, Start, Stop, and paste code directly.
/// <summary> /// Function Description: Satellite Cloud Map Download Manager /// Creator: Administrator /// Creation date: 2018/9/25 14:29:03 /// Last Modifier: Administrator /// Date of final revision: 2018/9/25 14:29:03 /// </summary> public class CloudImageManager { private string _ImagePath = System.Configuration.ConfigurationManager.AppSettings["Path"]; private Timer _Timer = null; private double Interval = double.Parse(System.Configuration.ConfigurationManager.AppSettings["Minutes"]); public CloudImageManager() { _Timer = new Timer(); _Timer.Interval = Interval * 60 * 1000; _Timer.Elapsed += _Timer_Elapsed; } void _Timer_Elapsed(object sender, ElapsedEventArgs e) { StartLoad(); } /// <summary> /// Start downloading clouds /// </summary> private void StartLoad() { LoadCloudImage(); } public void Start() { StartLoad(); _Timer.Start(); } public void Stop() { _Timer.Stop(); } /// <summary> /// Download all satellite images of the day /// </summary> private void LoadCloudImage() { CreateFilePath();//Determine whether a folder exists or not, and create it if it does not exist //Get the previous day's date string lastYear = DateTime.Now.AddDays(-1).Year.ToString(); string lastMonth = DateTime.Now.AddDays(-1).Month.ToString(); if (lastMonth.Length < 2) lastMonth = "0" + lastMonth; string lastDay = DateTime.Now.AddDays(-1).Day.ToString(); if (lastDay.Length < 2) lastDay = "0" + lastDay; //Get the date of the day string year = DateTime.Now.Year.ToString(); string month = DateTime.Now.Month.ToString(); if (month.Length < 2) month = "0" + month; string day = DateTime.Now.Day.ToString(); if (day.Length < 2) day = "0" + day; //Set all filenames string[] dates0 = { lastYear + "/" + lastMonth + "/" + lastDay, year + "/" + month + "/" + day }; string[] dates = { lastYear + lastMonth + lastDay, year + month + day }; string[] hours = { "00", "01", "02", "03", "04", "05", "06", "07", "08", "09", "10", "11", "12", "13", "14", "15", "16", "17", "18", "19", "20", "21", "22", "23" }; string[] minutes = { "15", "45" }; int hLength = hours.Count(); //Traverse all online clouds on the day of download for (int i = 0; i < 2; i++) { string date = dates[i]; string date0 = dates0[i]; for (int j = 0; j < hLength; j++) { string hour = hours[j]; for (int k = 0; k < 2; k++) { string minute = minutes[k]; string imageUrl = @"http://image.nmc.cn/product/" + date0 + @"/WXCL/SEVP_NSMC_WXCL_ASC_E99_ACHN_LNO_PY_" + date + hour + minute + "00000.JPG"; string[] s = imageUrl.Split('/'); string imageName = s[s.Count() - 1]; HttpWebRequest request = HttpWebRequest.Create(imageUrl) as HttpWebRequest; HttpWebResponse response = null; try { response = request.GetResponse() as HttpWebResponse; } catch (Exception) { continue; } if (response.StatusCode != HttpStatusCode.OK) continue; Stream reader = response.GetResponseStream(); FileStream writer = new FileStream(_ImagePath + imageName, FileMode.OpenOrCreate, FileAccess.Write); byte[] buff = new byte[512]; int c = 0; //Number of bytes actually read while ((c = reader.Read(buff, 0, buff.Length)) > 0) { writer.Write(buff, 0, c); } writer.Close(); writer.Dispose(); reader.Close(); reader.Dispose(); response.Close(); } } } } /// <summary> /// Determine whether a folder exists or not, and create it if it does not exist /// </summary> private void CreateFilePath() { if (Directory.Exists(_ImagePath)) { ClearImages(); return; } else { Directory.CreateDirectory(_ImagePath); } } /// <summary> /// Clear all files under folders /// </summary> private void ClearImages() { try { DirectoryInfo dir = new DirectoryInfo(_ImagePath); FileSystemInfo[] fileinfo = dir.GetFileSystemInfos(); //Returns all files and subdirectories in the directory foreach (FileSystemInfo i in fileinfo) { if (i is DirectoryInfo) //Determine whether a folder or not { DirectoryInfo subdir = new DirectoryInfo(i.FullName); subdir.Delete(true); //Delete subdirectories and files } else { File.Delete(i.FullName); //Delete the specified file } } } catch (Exception e) { Console.WriteLine(e.Message); } } }
Then call in Program.cs:
static void Main(string[] args) { HostFactory.Run(x => //1 { x.Service<CloudImageManager>(s => //2 { s.ConstructUsing(name => new CloudImageManager()); //3 s.WhenStarted(tc => tc.Start()); //4 s.WhenStopped(tc => tc.Stop()); //5 }); x.RunAsLocalSystem(); //6 x.SetDescription("Real-time download tool for satellite cloud images"); //7 x.SetDisplayName("CloudImageLoad"); //8 x.SetServiceName("CloudImageLoad"); //9 }); }
As you can see, the calls mainly involve constructors, Start methods, and Stop methods in the CloudImageManager class.
Installation, operation and unloading:
These operations for services under the Topshell framework are relatively simple
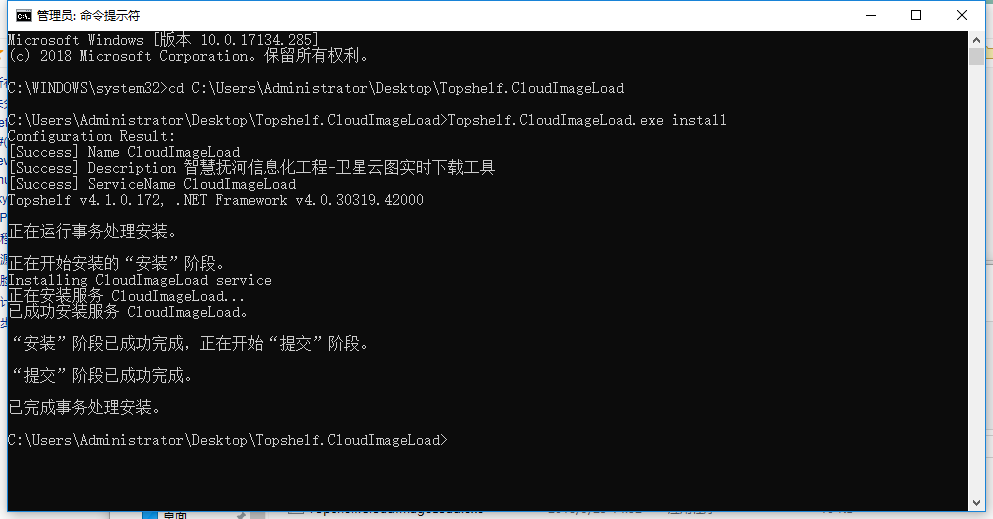
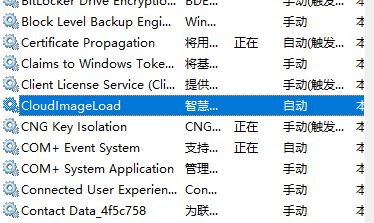