1. Example code: Runnable + ThreadPoolExecutor
First create a
Runnable
Implementation class of interface (of course, it can also be
Callable
Interface. We also mentioned the area of the two above
Don't
MyRunnable.java
import java.util.Date; public class MyRunnable implements Runnable { private String command; public MyRunnable (String s){ this.command = s; } @Override public void run() { System.out.println(Thread.currentThread().getName() + " Start. Time = " + new Date()); processCommand(); System.out.println(Thread.currentThread().getName() + " End. Time = " + new Date()); } private void processCommand() { try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } } @Override public String toString() { return this.command; } }
Write the test program, which we use here as recommended by Alibaba
ThreadPoolExecutor
Constructor to customize parameters
Create a thread pool.
ThreadPoolExecutorDemo.java
public class CallableDemo { private static final int CORE_POOL_SIZE = 5; private static final int MAX_POOL_SIZE = 10; private static final int QUEUE_CAPACITY = 100; private static final Long KEEP_ALIVE_TIME = 1L; public static void main(String[] args) { //Use the method of creating thread pool recommended by Alibaba // Created with a custom parameter through the ThreadPoolExecutor constructor ThreadPoolExecutor executor = new ThreadPoolExecutor( CORE_POOL_SIZE, MAX_POOL_SIZE, KEEP_ALIVE_TIME, TimeUnit.SECONDS, new ArrayBlockingQueue<>(QUEUE_CAPACITY), new ThreadPoolExecutor.CallerRunsPolicy()); for (int i = 0; i < 10; i++) { //Create a WorkerThread object (the WorkerThread class implements the Runnable interface) Runnable worker = new MyRunnable("" + i); //Execute Runnable executor.execute(worker); } //Terminate thread pool executor.shutdown(); while (!executor.isTerminated()) { } System.out.println("Finished all threads"); } }
You can see that our code above specifies:
1.
corePoolSize
:
The number of core threads is
5
.
2.
maximumPoolSize
: maximum number of threads
10
3.
keepAliveTime
:
Waiting time is
1L
.
4.
unit
:
The unit of waiting time is
TimeUnit.SECONDS
.
5.
workQueue
: task queue is
ArrayBlockingQueue
, with a capacity of
100;
6.
handler
:
Saturation strategy is
CallerRunsPolicy
.
Schematic diagram of thread pool:
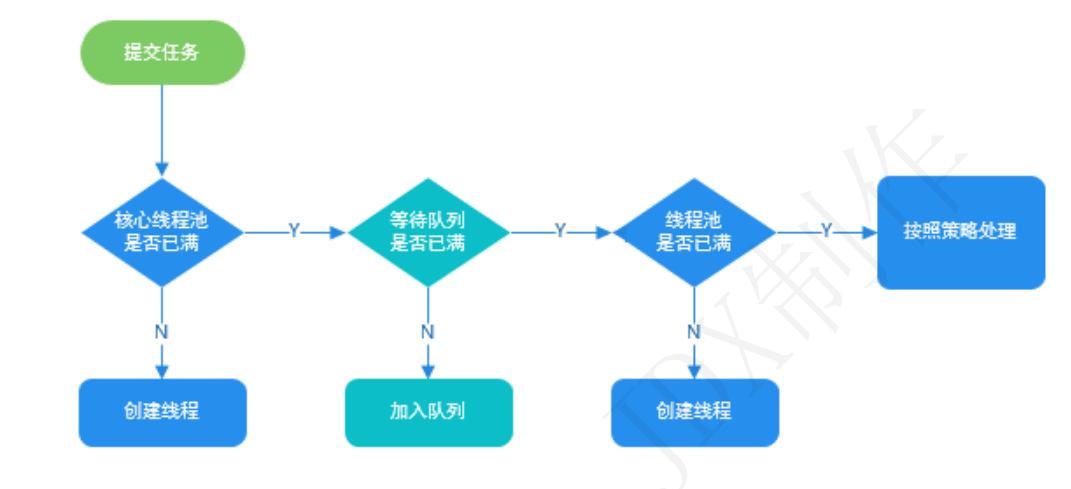
2.Runnable vs Callable
Runnable
since
Java 1.0
Has been there, but
Callable
Only in
Java 1.5
Introduced in
,
The purpose is to deal with it
Runnable
Unsupported use case.
Runnable
Interface
No result is returned or a check exception is thrown, but
Callable
Interface
sure.
Therefore, it is recommended if the task does not need to return results or throw exceptions
Runnable
Interface
This makes the code look more concise.
Tool class
Executors
Can achieve
Runnable
Object and
Callable
Conversion between objects.
(
Executors.callable
(
Runnable task
)Or
Executors.callable
(
Runnable task
,
Object
resule
)
)
3.
execute()
vs
submit()
execute()
Method is used to submit a task that does not need a return value, so it is impossible to judge whether the task is successfully executed by the thread pool;
submit()
Method is used to submit a task that requires a return value. The thread pool returns a
Future
Object of type through this
Future
Object can judge whether the task is executed successfully
, and you can
Future
of
get()
Method to get the return value,
get()
Method blocks the current thread until the task is completed
get
(
long timeout
,
TimeUnit unit
)
method
It will block the current thread and return immediately after a period of time. At this time, the task may not be completed.
shutdown()
VS
shutdownNow()
shutdown()
:
When the thread pool is closed, the state of the thread pool changes to
SHUTDOWN
. The thread pool no longer accepts new tasks, but the team
Lieri's mission has to be completed.
shutdownNow()
:
When the thread pool is closed, the status of the thread changes to
STOP
. The thread pool terminates the currently running task and
Stop processing queued tasks and return the tasks that are waiting to be executed
List
.
i
sTerminated()
VS
isShutdown()
isShutDown
When called
shutdown()
Method returns as
true
.
isTerminated
When called
shutdown()
Method, and when all submitted tasks are completed, it returns as
true
4.Callable + ThreadPoolExecutor example code
import java.util.concurrent.Callable; public class MyCallable implements Callable { @Override public String call() throws Exception { Thread.sleep(1000); return Thread.currentThread().getName(); } }
import java.util.concurrent.ArrayBlockingQueue; import java.util.concurrent.ThreadPoolExecutor; import java.util.concurrent.TimeUnit; public class ThreadPoolExecutorDemo { private static final int CORE_POOL_SIZE = 5; private static final int MAX_POOL_SIZE = 8; private static final int QUEUE_CAPACITY = 10; private static final Long KEEP_ALIVE_TIME = 1L; public static void main(String[] args) { ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor( CORE_POOL_SIZE, MAX_POOL_SIZE, KEEP_ALIVE_TIME, TimeUnit.SECONDS, new ArrayBlockingQueue<>(QUEUE_CAPACITY), new ThreadPoolExecutor.CallerRunsPolicy()); for (int i = 0; i < 40; i++) { Runnable worker = new MyRunnable(">>" + i); threadPoolExecutor.execute(worker); System.out.println("worker>>" + i); } //Terminate thread threadPoolExecutor.shutdown(); while (!threadPoolExecutor.isTerminated()){ } System.out.println("Finished All Threads"); } }