udp protocol and process
I. udp protocol
#- sever
import socket
#Socket.sock? Dgram - > UPD protocol
sever = socket.socket(type=socket.SOCK_DGRAM)
#The server needs to bind an address to let others know where you are
sever.bind(
('127.0.0.1', 9002)
)
while True:
#Send data to the server's user address
data, addr = sever.recvfrom(1024)
print(addr)
print(data.decode('utf-8'))
#- client
import socket
client = socket.socket(type=socket.SOCK_DGRAM)
address = ('127.0.0.1', 9002)
while True:
msg = input('client---->sever:').strip().encode('utf-8')
client.sendto(msg, address)
('127.0.0.1', 57697)
//Hot bar
('127.0.0.1', 59959)
//It's so beautiful
('127.0.0.1', 59960)
//Jeba Yafeng
II. Theoretical knowledge
1. udp protocol (understanding)
Called packet protocol
Characteristic:
1) no link needed
2) do not need to know whether the other party has received
3) unsafe data
4) fast transmission speed
5) be able to support concurrency
6) no sticking
7) no need to start the server first and then the client
Advantage:
-Fast transmission speed
-Can support concurrency
-Does not stick
Disadvantages:
-Data is insecure and easy to lose
Application scenario: early QQ chat room
-tcp protocol (called Streaming Protocol)
Advantage:
-Data security
Disadvantages:
-Slow transmission speed
- Sticky bag
The interview may ask: the difference between tcp and udp, simply describe the advantages and disadvantages (******)
2. Concurrent programming
1) operating system development history
-1.1 punch card
-Reading data is very slow
-Very low CPU utilization
-Single user (one code use)
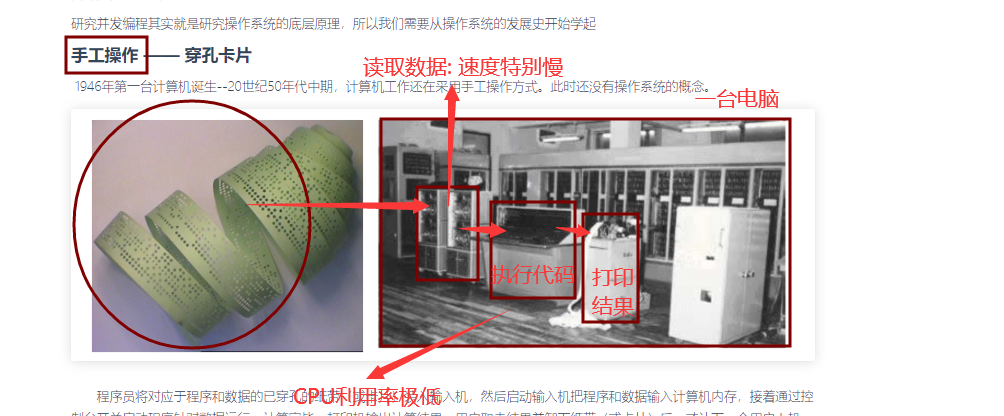
-1.2 batch processing
-Reading data is very slow
-Very low CPU utilization
-Online (using multiple codes)
-Efficiency is still very low
-1.3 offline batch processing (design principle of modern operating system)
-High speed of reading data
-Increased CPU utilization
2) multichannel technology (based on single core background):
-Single channel: one channel goes to black -- > serial
-For example: a,b need to use CPU, a use it first, b wait for a to use it before using CPU
-Multichannel: (*****)
-For example: a,b need to use CPU,a uses it first, b waits for a until a enters "io or execution time is too long"
a will (switch + save), and then b can use CPU. When b executes, it will encounter "io or execution time is too long"
Then give the CPU execution permission to a until the end of the two programs
-Reuse of space
Multiple programs use one CPU
-Time reuse (******)
Switch + save state
1) when the executing program encounters io, the operating system will deprive the CPU of execution authority.
Advantage:
-CPU execution efficiency improvement
2) when the execution time is too long, the operating system will deprive the CPU of execution authority
Disadvantages:
-Low efficiency of program execution
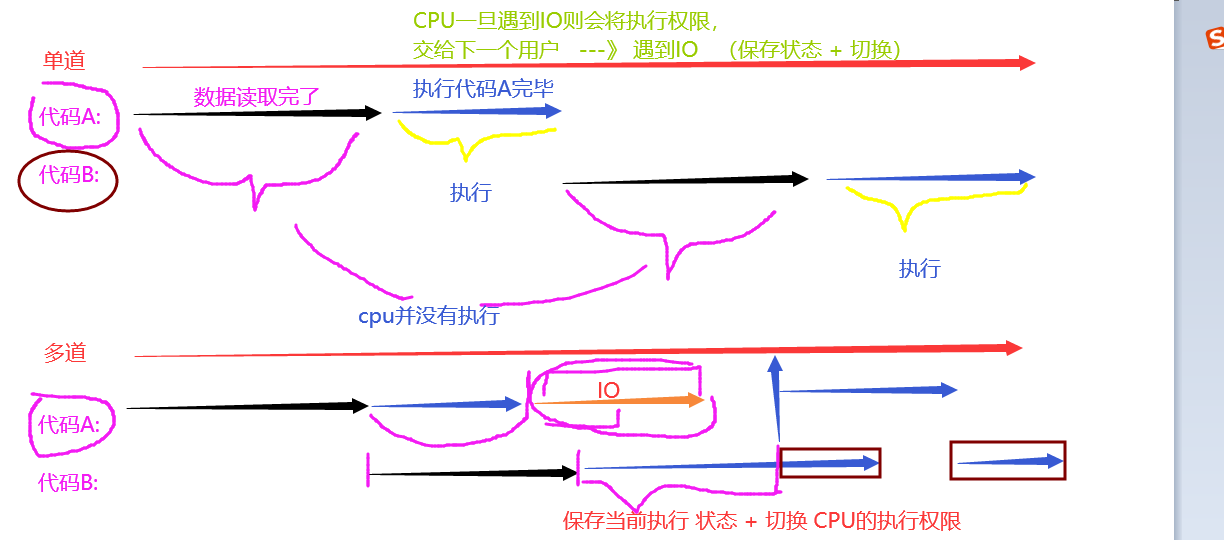
-Concurrent and parallel; (*****)
-Concurrent (one person):
In the case of a single core (one CPU), when two a and b programs are executed, a executes first. When a encounters io, b begins to scramble for the CPU permission, and then let b execute. They look like they are running at the same time.
-Parallel (two or more people):
In the case of multi-core (multiple CPU s), when two a,b programs are executed, a and b are executed at the same time. They are really running.
Interview question: can we achieve parallel in the case of single core? Answer: No.
3) process
1. What is the process?
Process is a resource unit
2. Process and procedure:
-Program: a pile of code files
-Process: the process of executing code, called process
3. Process scheduling (understanding)
1) first come first serve scheduling algorithm (understand)
-For example, program: a,b. If a comes first, let a serve first. After a service is completed, b serves again.
-Disadvantages: inefficient execution
2) short job priority scheduling algorithm priority (understanding)
-If the execution time is short, schedule first.
-Disadvantages: programs that take a long time to execute need to wait for all short-term programs to complete before they can be executed
Process scheduling algorithm of modern operating system: time slice Round method + multilevel feedback queue (known)
3) time slice method
-For example, if there are 10 programs to execute at the same time, the operating system will give you 10 seconds, and then the time slice method will divide 10 seconds into 10 equal minutes.
4) multi level feedback queue:
Level 1 queue: highest priority, execute the program in the queue first
Level 2 queue: priority next
Level 3 queues: and so on
4) synchronous and asynchronous
Synchronization and asynchrony refer to the way tasks are submitted.
Synchronization (serial equivalent):
Both a and b programs need to be submitted and executed. If a submits for execution first, b must wait for a to finish execution before submitting tasks.
Asynchronous (equivalent to concurrent):
Two a and b programs need to be submitted and executed. If a submits and executes first, b can directly submit tasks without waiting for a to finish executing.
5) blocking and non blocking (*****):
-Blocking (waiting):
-Every io will block
- io:
input()
output()
time.sleep(3)
Reading and writing of documents
Data transmission
-Non blocking (no waiting):
-All but io are non blocking (e.g. from 1 + 1 to 1 million)
6) three states of the process (*****):
-Ready state:
-Synchronous and asynchronous
-Running state: the execution time of the program is too long ----- > return the program to ready state
- non blocking
-Blocking state:
- meet io
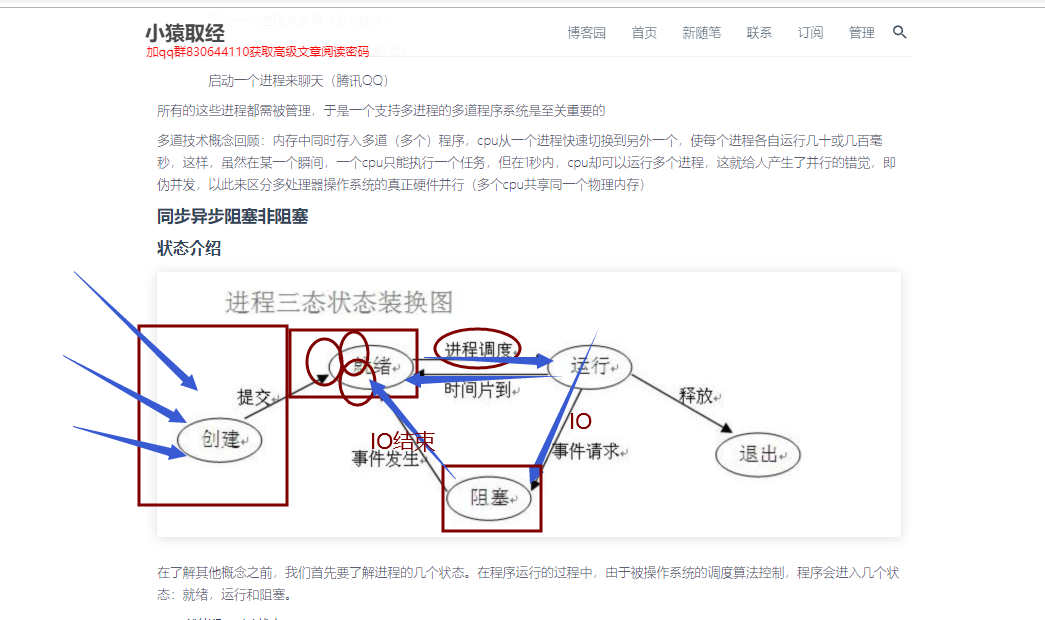
Is blocking the same as synchronization? Is non blocking the same as asynchronous?
-Synchronous and asynchronous: how to submit tasks
-Blocking non blocking: state of the process
-Asynchronous and non blocking: -- Maximize CPU utilization
7) there are two ways to create a process:
III. synchronous demonstration
import time
def func():
print('start...')
time.sleep(3)
print('end...')
if __name__ == '__main__':
#Task 1:
func()
#Task two:
print('Program end')
#Synchronous execution: Task 2 will not be executed until task 1 is completed
start...
end...
//Program end
from multiprocessing import Process
import time
#Method 1: call Process directly
def task(): #task #name== 'jason_sb'
print(f'start....Child process')
time.sleep(3)
print(f'end...Child process')
if __name__ == '__main__':
#target = task (function address) -- > create a subprocess
#Submit three tasks asynchronously
p_obj = Process(target=task)
p_obj.start() #Tell the operating system to create a subprocess
p_obj.join() #Tell the operating system to wait for the subprocess to finish and then finish, which is equivalent to serial
print('Executing current main process')
start....Child process
end...Child process
//Executing current main process
- Asynchronous commit three processes
# #Submit three tasks asynchronously
# #Method 1: call Process directly
#
# def task(name): #task #name== 'jason_sb'
#
# Print (subprocess' of f'start... {name})
# time.sleep(3)
# Print (subprocess' of f'end... {name})
#
# if __name__ == '__main__':
# #target = task (function address) -- > create a subprocess
# #Submit three tasks asynchronously
# p_obj1 = Process(target=task, args=('jason_sb',))
# p_obj2 = Process(target=task, args=('sean_sb',))
# p_obj3 = Process(target=task, args=('tank_sb',))
#
#
# p_obj1.start() #Tell the operating system to create a subprocess
# p_obj2.start() #Tell the operating system to create a subprocess
# p_obj3.start() #Tell the operating system to create a subprocess
#
#
# print('executing current main process')
#
# # Subprocess of start
# # Child process of start
# # Subprocess of start
# # Subprocesses of end... Jason ﹣ sb
# # The subprocess of end... Sean
# # The subprocess of end... Tank
- Submit three processes synchronously
#Submit three synchronously
def task(name): #task #name== 'jason_sb'
print(f'start....{name}Child process')
time.sleep(3)
print(f'end...{name}Child process')
if __name__ == '__main__':
task(1)
task(2)
task(3)
# Subprocess of start
# Child process of end...1
# Subprocess of start....2
# Child process of end...2
# Subprocess of start....3
# Child process of end...3
- Submit multiple processes asynchronously
Executing multiple processes asynchronously
def task(name): #task #name== 'jason_sb'
print(f'start....{name}Child process')
time.sleep(3)
print(f'end...{name}Child process')
if __name__ == '__main__':
list1 = []
for line in range(10):
p_obj = Process(target=task, args=('jason_sb', ))
p_obj.start()
list1.append(p_obj)
for obj in list1:
obj.join()
print('Main process')
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
start....jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
end...jason_sb Child process
//Main process
#Mode two:
from multiprocessing import Process
import time
class MyProcess(Process):
def run(self):
print(f'start...{self.name}Child process')
time.sleep(3)
print(f'end...{self.name}Child process')
if __name__ == '__main__':
list1 = []
for line in range(10):
obj = MyProcess()
obj.start()
list1.append(obj)
for obj in list1:
obj.join()
print('Main process...')
# Subprocess of start...MyProcess-1
# Subprocess of start...MyProcess-2
# Subprocess of start...MyProcess-4
# Subprocess of start...MyProcess-5
# Subprocess of start...MyProcess-3
# Subprocess of start...MyProcess-6
# Subprocess of start...MyProcess-8
# Subprocess of start...MyProcess-7
# Subprocess of start...MyProcess-9
# Subprocess of start...MyProcess-10
# End... Subprocess of myprocess-1
# End... Subprocess of myprocess-2
# End... Subprocess of myprocess-4
# End... Subprocess of myprocess-5
# End... Subprocess of myprocess-3
# End... Subprocess of myprocess-6
# End... Subprocess of myprocess-7 end... Subprocess of myprocess-8
# End... Subprocess of myprocess-9
# End... Subprocess of myprocess-10
# Main process...