If you want to get the data returned after the closure of the newly opened Activity in Activity, you need to open the new Activity using the Start Activity ForResult (Intent intent, int request Code) method provided by the system. When the new Activity is closed, the data will be returned to the front Activity. In order to get the data returned, you must override the onActivity Result (int request Code) in the previous Activity. Int resultCode, Intent data) method:
public class MainActivity extends Activity { @Overrideprotected void onCreate(Bundle savedInstanceState) { Button button =(Button)this.findViewById(R.id.button); button.setOnClickListener(new View.OnClickListener(){//Clicking this button opens a new Activity publicvoid onClick(View v) { //The second parameter is the request code, which can be numbered according to business requirements. startActivityForResult(new Intent(MainActivity.this, NewActivity.class), 1); }}); } //The first parameter is the request code, which calls startActivityForResult() to pass past values. //The second parameter is the result code, which identifies the new Activity from which the returned data comes. @Override protected voidonActivityResult(int requestCode, int resultCode, Intent data) { String result =data.getExtras().getString("result"));//Get the data returned when the new Activity is closed }}
When the new Activity is closed, the data returned by the new Activity is transferred through Intent. The android platform calls the onActivityResult() method of the previous Activity, and passes in the Intent which stores the returned data as the third input parameter. In the onActivityResult() method, the data returned by the new Activity can be retrieved by using the third input parameter.
The role of request codes:
To open a new activity using the startActivityForResult (Intent intent. intrequestcode) method, we need to pass a request code for that method. The value of the request code is set by itself according to the business needs.
Used to identify the source of the request. For example, an Activity has two buttons. Clicking on these two buttons will open the same Activity. Whether that button opens the new Activity, when the new Activity is closed, the system will call the onActivity Result (int request Code, int result Code, Intent data) method of the previous Activity. In the onActivityResult() method, if you need to know that the new Activity is opened by that button and do the corresponding business processing, you can do this:
@Override public void onCreate(Bundle savedInstanceState) { .... button1.setOnClickListener(newView.OnClickListener(){ public void onClick(View v) { startActivityForResult (newIntent(MainActivity.this, NewActivity.class), 1); }}); button2.setOnClickListener(newView.OnClickListener(){ public void onClick(View v) { startActivityForResult (newIntent(MainActivity.this, NewActivity.class), 2); }}); @Override protected voidonActivityResult(int requestCode, int resultCode, Intent data) { switch(requestCode){ case 1: //Requests from button 1 are processed accordingly case 2: //Requests from button 2 for corresponding business processing } }
The role of result codes: Used to indicate the source of the returned results.
In an Activity, you may use the startActivityForResult() method to open multiple different activities to handle different businesses. When these new activities are closed, the system will call the onActivityResult (int request Code, int resultCode, Intentdata) method of the previous Activity. In order to know which new Activity the returned data comes from, you can do this in the onActivityResult() method (ResultActivity and NewActivity are new activities to open):
public class ResultActivity extends Activity { ..... ResultActivity.this.setResult(1, intent); ResultActivity.this.finish();}public class NewActivity extends Activity { ...... NewActivity.this.setResult(2,intent); NewActivity.this.finish();}public class MainActivity extends Activity { // In this activity, ResultActivity and New Activity are opened @Override protected voidonActivityResult(int requestCode, int resultCode, Intent data) { switch(resultCode){ case 1: // ResultActivity's return data case 2: // Return data of New Activity } }}
If data or results need to be returned, start Activity ForResult (Intent intent, intrequestCode) is used, and the value of requestCode is self-defined to identify the target activity of the jump.
All the jump target Activity has to do is return data / results. setResult(int resultCode) only returns results without data, or setResult(int resultCode, Intent data) both return! The processing function to receive the returned data/results is onActivityResult (int requestCode, int resultCode, Intent data), where the request Code is the request Code of startActivityForResult, resultCode is the resultCode in setResult, and the returned data is in data.
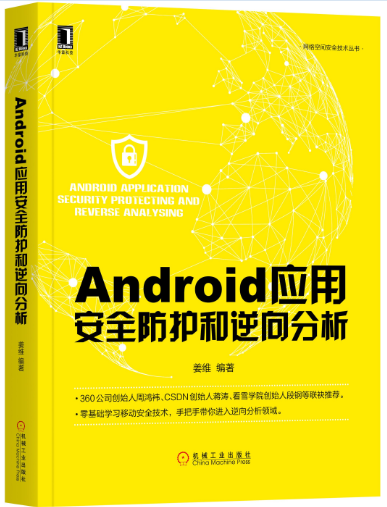
More: click here
follow WeChat Public Number, Real-time Delivery of Latest Technology Dry Goods
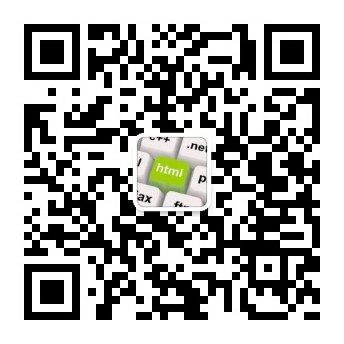
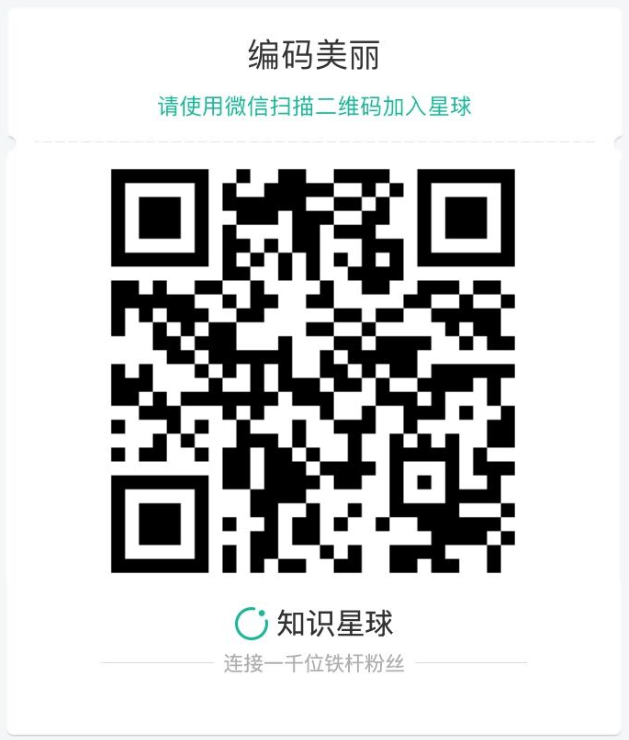