public boolean clipRect(float left, float top, float right, float bottom) public boolean clipRect(int left, int top, int right, int bottom) public boolean clipRect(@NonNull Rect rect) public boolean clipRect(@NonNull RectF rect)
2,clipOutPath
public boolean clipOutPath(@NonNull Path path)
Description: only the canvas area outside the path is left, while those within the path range are not displayed( (opposite to the scope of clipPath)
It is worth noting that this method can only be called above API26. In the lower version, we use the method described in the next section
for instance:
We first prepare a heart-shaped path Path, and then call clipOutPath to cut out the area beyond this path from the canvas. Finally, for our observation, we use drawColor to dye the wine red.
// Step 1: create heart path mPath ....Omit, please move github // Step 2: cut the area outside the heart-shaped path from the canvas canvas.clipOutPath(mPath); // Step 3: paint wine red canvas.drawColor(mBgColor);
Rendering [external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-bwrff2k2-1630841978227)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce605a01f641? imageView2/0/w/1280/h/960/ignore-error/1)]
There are several other methods of this type, but their clipping range is rectangular
public boolean clipOutRect(float left, float top, float right, float bottom) public boolean clipOutRect(int left, int top, int right, int bottom) public boolean clipOutRect(@NonNull Rect rect) public boolean clipOutRect(@NonNull RectF rect)
3,clipPath
public boolean clipPath(@NonNull Path path, @NonNull Region.Op op)
Description: use the path path to operate on the canvas. The function is determined by the op.
The description is abstract. We can experience it through examples. However, before the above example, we need to understand the enumeration type Region.Op. the specific content code is as follows
public enum Op { // A: Cut the path for us first // B: Cut the path for us // The part of A shape different from B is displayed DIFFERENCE(0), // The shape of the intersection of A and B INTERSECT(1), // Complete sets of A and B UNION(2), // The complete set shape of A and B, excluding the part after the intersection shape XOR(3), // The part of B shape different from A is displayed REVERSE_DIFFERENCE(4), // Show only the shape of B REPLACE(5); // ... omit irrelevant code }
There are six types through the source code. It is worth mentioning the following two points:
1) The type used in clipOutPath method is DIFFERENCE. In other words, we can use the following code instead to solve the problem that clipOutPath method cannot be used below API26
clipPath(mPath, Region.Op.DIFFERENCE)
2) The type used in the clipPath method is INTERSECT. In other words, we can use the following code instead
clipPath(mPath, Region.Op.INTERSECT)
For example:
Next, we will explain the six types one by one. The two cutting comparisons can reflect the role of the Region.Op parameter, so our next examples need to use two paths:
1. Cardioid path (A in the following example)
[the external chain picture transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-vtuskehu-1630841978230)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce605bd1fd35? Imageview2 / 0 / w / 1280 / h / 960 / ignore error / 1)] 2. Circular path (B in the following example)
[external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (IMG xegmwnnh-1630841978232)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce605ee9123d?imageView2/0/w/1280/h/960/ignore -error/1)]
(1)DIFFERENCE
Description: the part of A shape different from B is displayed
Rendering: red is the area left by the final clipping
[the external chain picture transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-gdifujmc-1630841978234)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce6064ea9f69? imageView2/0/w/1280/h/960/ignore-error/1)]
(2)INTERSECT
Description: the shape of the intersection of A and B
Rendering: red is the area left by the final clipping
[external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-l3ltuzj-1630841978235)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce606556e410? imageView2/0/w/1280/h/960/ignore-error/1)]
(3)UNION
Description: complete set of A and B
Rendering: red is the area left by the final clipping
[the external chain picture transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-rlg85dsy-1630841978236)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce6065741fdf?imageView2/0/w/1280/h/960/ignore -error/1)]
(4)XOR
Description: the complete set shape of A and B, and the part after removing the intersection shape
Rendering: red is the area left by the final clipping
(5)REVERSE_DIFFERENCE
Description: the part of B shape different from A is displayed
Rendering: red is the area left by the final clipping
(6)REPLACE
Description: displays only the shape of B
Rendering: red is the area left by the final clipping
There are several other methods of this type, but their clipping range is rectangular
public boolean clipRect(float left, float top, float right, float bottom, @NonNull Region.Op op) public boolean clipRect(@NonNull Rect rect, @NonNull Region.Op op) public boolean clipRect(@NonNull RectF rect, @NonNull Region.Op op)
4, Actual combat
We have learned in the previous section that the role of these API s is tailoring, so we will use them in this section.
My girlish heart
Rendering [external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-qq2uwdsh-1630841978240)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce6024652dbe?imageslim )]
Github entrance: Portal
Coding idea
Let's use the following idea map drawn by our friends (see if one picture can win a thousand words) 😄)
[external chain picture transfer failed. The source station may have anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-q2pjltzy-1630841978241)( https://user-gold-cdn.xitu.io/2019/4/27/16a5ce6097139350? imageView2/0/w/1280/h/960/ignore-error/1)]
Here, for the sake of visual effect and easy explanation, red is the pink in our demo, blue is the cyan in our demo, and orange is the final gradient
Step 1 (green heart part): we first cut the heart-shaped area on the canvas, which lays the foundation for the canvas area finally presented to the user as a "heart".
Step 2 (red part): we dye the canvas red, and then write "fierce little pot friend" in blue in the center of the canvas. Finally, we use the red box in the figure (that is, the upper side is a horizontal line and the lower side is a Path red area drawn with Bezier curve) to cut down the upper part of the canvas and place it in the final rendered canvas.
Step 3 (blue part): Contrary to step 2, we dye the canvas blue, and then write "fierce little basin friend" in red in the center of the canvas. Finally, we use the blue box in the figure (that is, the upper side is drawn with Bezier curve and the lower side is the lazy color area of horizontal line) to cut down the lower part of the canvas and place it in the final rendered canvas.
Step 4: after the first three steps, our pattern has formed the image on the right. When we start the animation, we actually control the y-axis coordinates of the middle Bezier curve and make it rise from the bottom to the top, which presents a heart-shaped animation effect. Therefore, we can achieve this effect by offsetting the canvas to a certain value, and let the Bezier curve move horizontally, which has a wave motion.
Core code
// First step canvas.clipPath(mHeartPath); // ========Step 2 start============== ## Learning sharing In the current era of information sharing, many resources can be found on the network, which only depends on whether you are willing to find it or whether the method is right Many friends are not without information, most of them have dozens or hundreds G,But they are disorganized. They don't know how to look, where to look, or even forget after looking If you feel that the information you find on the Internet is very messy and unsystematic, I also share a set for you. It is more systematic. I usually study it often myself. **2021 The latest tens of thousands of pages of real interview questions for large factories** 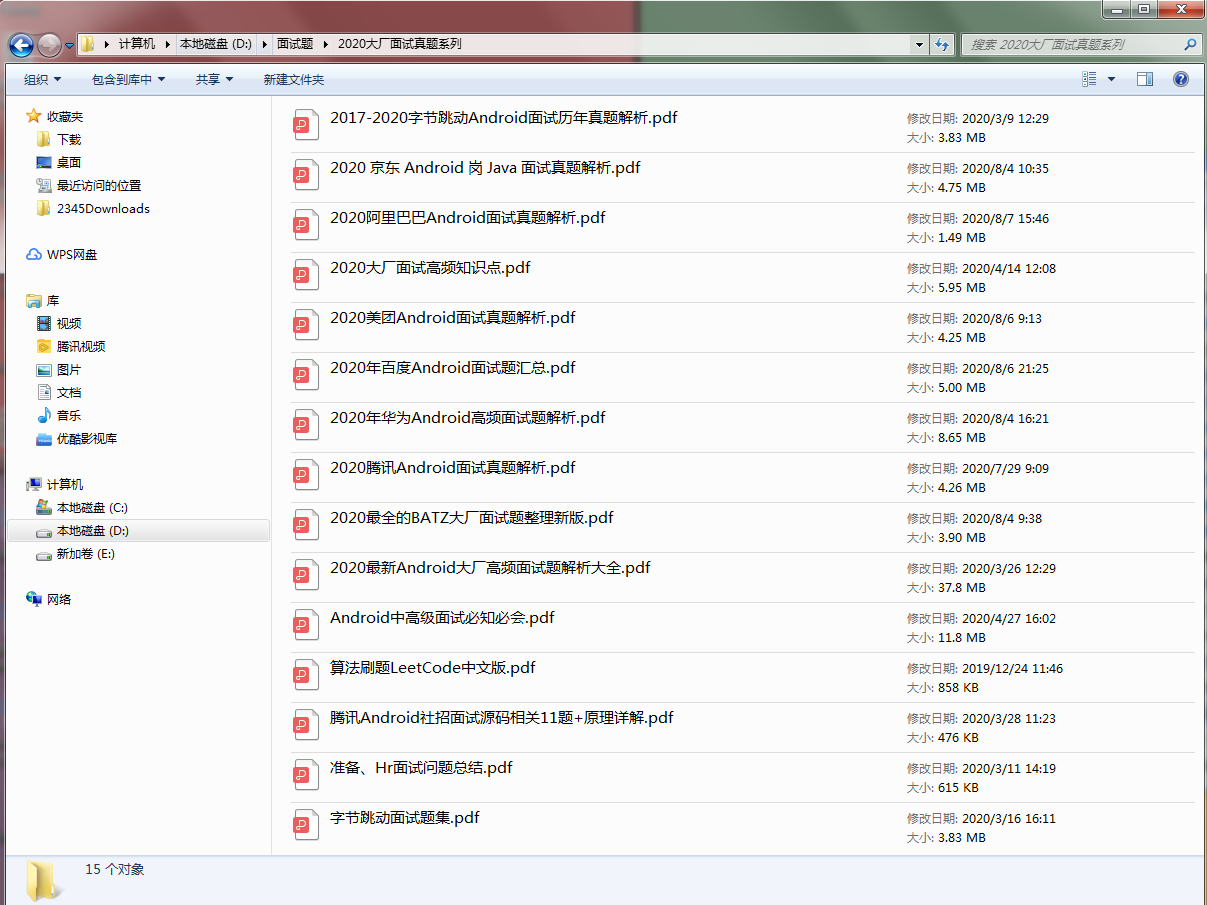 **Learning materials for seven modules: such as NDK Module development Android Framework architecture...** 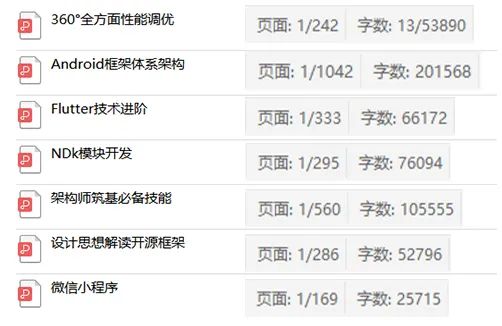 Only systematic and directional learning can quickly improve their skills in a period of time. **[CodeChina Open source projects:< Android Summary of study notes+Mobile architecture video+Real interview questions for large factories+Project practice source code](https://codechina.csdn.net/m0_60958482/android_p7)** > This system learning notes to adapt to the crowd: > **First,**Learning knowledge is fragmented, and there is no reasonable learning route and advanced direction. > **Second,**After several years of development, I don't know how to advance and go further. I'm confused. > **third**,At the right age, I don't know how to develop, transform management or strengthen technical research. If you need it, I happen to have why not come to get it! Maybe it can change your current state! > Due to the large content of the article, the length is not allowed, and some of the contents not displayed are displayed in the form of screenshots id_p7)** > This system learning notes to adapt to the crowd: > **First,**Learning knowledge is fragmented, and there is no reasonable learning route and advanced direction. > **Second,**After several years of development, I don't know how to advance and go further. I'm confused. > **third**,At the right age, I don't know how to develop, transform management or strengthen technical research. If you need it, I happen to have why not come to get it! Maybe it can change your current state! > Due to the large content of the article, the length is not allowed, and some of the contents not displayed are displayed in the form of screenshots