1. What is template mode?
Define the skeleton of an algorithm in an operation, deferring some steps to subclasses. Template Method lets subclasses redefine certain steps of an algorithm without changing the algorithm's structure.
Template Method Pattern: defines the framework of an algorithm in operation, and delays some steps to subclasses. So that subclasses can not be changed Changing the structure of an algorithm can redefine some specific steps of the algorithm.
Speaking: the parent class template method defines the same process, and the child class rewrites the methods in the process.
2. Template schema definition
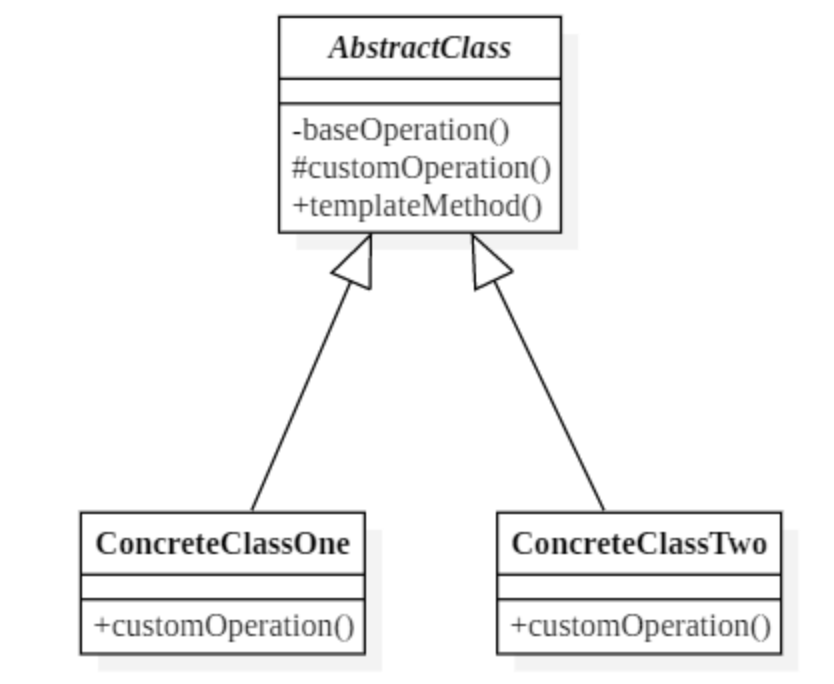
① AbstractClass abstract template
1, Basic method
The above baseOperation() or customOperation() method, also known as basic operation, is a method implemented by subclasses and called in template methods.
The basic method shall be designed as protected type as far as possible, which conforms to the Demeter rule. The attributes or methods that do not need to be exposed shall not be set as protected type as far as possible. If the implementation class is not necessary, try not to expand the access permission in the parent class.
Two. Template method
The above templateMethod() method can have one or more to schedule the basic methods and complete the fixed logic.
In order to prevent malicious operations, the final keyword is usually added to template methods, and overwriting is not allowed.
② Concrete class specific template
Implement one or more abstract methods defined by the parent class, that is, the basic methods defined by the parent class can be implemented in the child class.
3. Template mode common code
public abstract class AbstractClass { // Common and cumbersome operations private void baseOperation() { // do something } // Operations customized by subclasses protected abstract void customOperation(); // Framework for template method definition public final void templateMethod() { /** * Call the basic method to complete the fixed logic */ baseOperation(); customOperation(); } }
public class ConcreteClass1 extends AbstractClass{ @Override protected void customOperation() { // Specific template 1 business logic System.out.println("Specific template 1: customOperation()"); } }
public class ConcreteClass2 extends AbstractClass{ @Override protected void customOperation() { // Specific template 2 business logic System.out.println("Specific template 2: customOperation()"); } }
Test:
public class TemplateClient { public static void main(String[] args) { AbstractClass abstractClass1 = new ConcreteClass1(); AbstractClass abstractClass2 = new ConcreteClass2(); applyTemplate(abstractClass1); applyTemplate(abstractClass2); } public static void applyTemplate(AbstractClass abstractClass){ abstractClass.templateMethod(); } }
4. Advantages of template mode
① Encapsulate the unchanged part and expand the variable part
The algorithm considered as the invariant part is encapsulated into the parent class implementation, while the variable part can be extended through inheritance.
② . extract the public part code for easy maintenance
③ The behavior is controlled by the parent class and implemented by the child class
The basic method is implemented by subclasses, so subclasses can add corresponding functions through expansion, which conforms to the opening and closing principle.
5. Disadvantages of template mode
① The execution results of and subclasses affect the results of the parent class, which is contrary to our usual design habits. In complex projects, it will bring difficulty in reading.
② , which may cause the proliferation of subclasses and the problem of inheritance for inheritance
6. Callback
In order to solve the shortcomings of template pattern, we can use callback function instead of subclass inheritance.
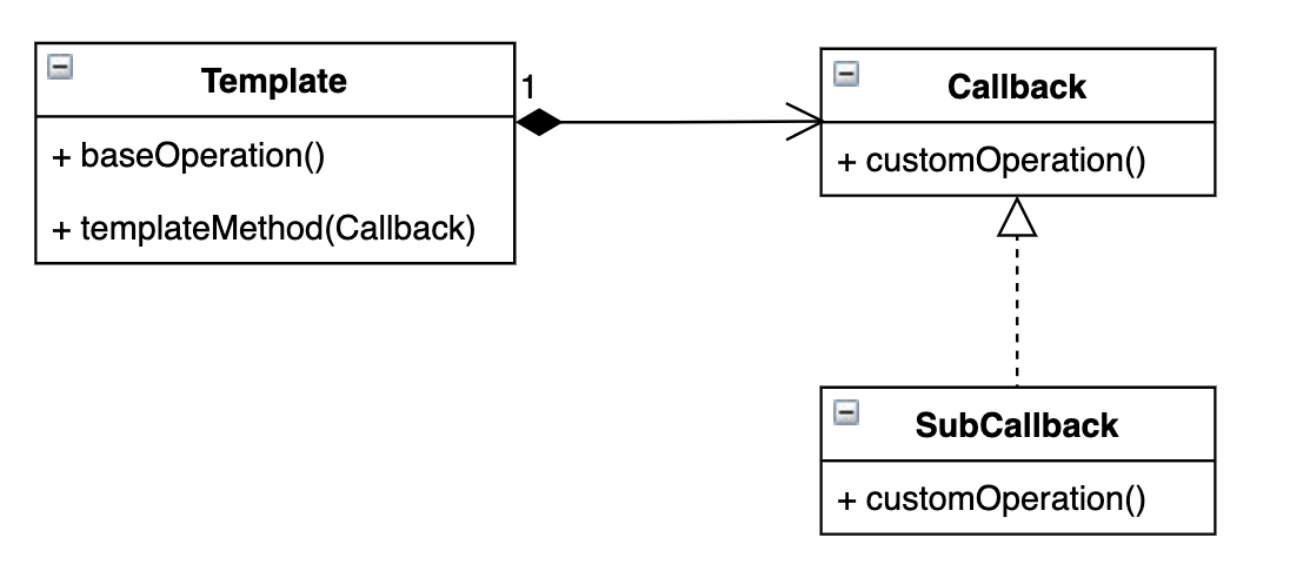
public interface Callback { void customOperation(); }
public class SubCallback implements Callback{ @Override public void customOperation() { System.out.println("SubCallback customOperation"); } }
/** * template class * Declared as final and cannot be inherited */ public final class Template { private void baseOperation(){ System.out.println("Template class public operation"); } public void templateMethod(Callback callback){ baseOperation(); callback.customOperation(); } }
Test:
public class TemplateClient { public static void main(String[] args) { Template template = new Template(); applyTemplate(template); } public static void applyTemplate(Template template){ Callback callback = new SubCallback(); template.templateMethod(callback); } }
Template is a stable final class and cannot be inherited. There is no problem that the behavior of subclasses affects the results of parent classes. Callback is an interface. The problem of inheritance for inheritance disappears.