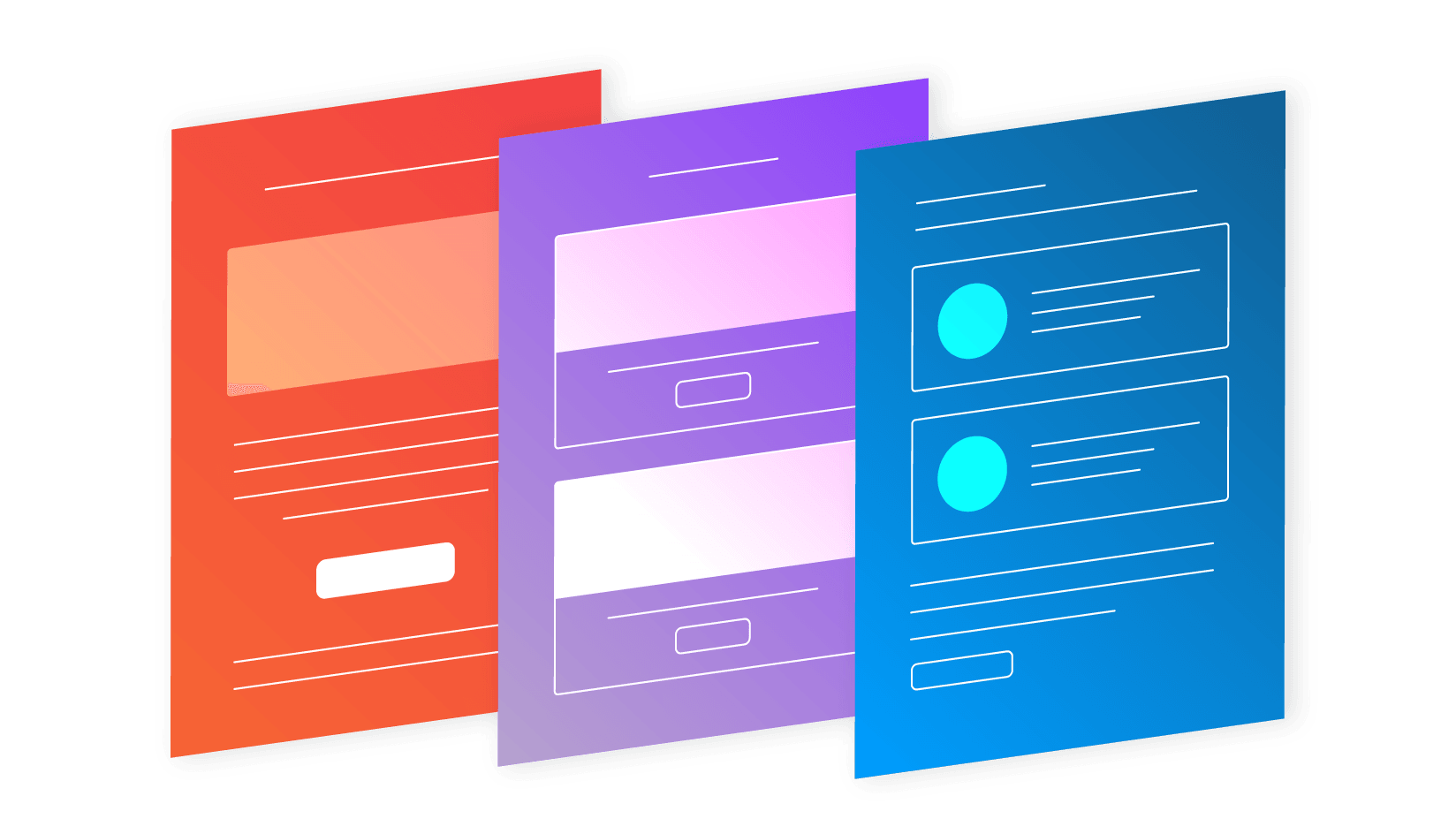
<header> <h1>Lorem ipsum dolor sit amet consectetur.</h1> <h2>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Doloremque, fuga!</h2> </header> <p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Modi aspernatur soluta sequi facilis voluptate deserunt minus pariatur necessitatibus asperiores itaque.</p> <div class="page">footer</div>
When we develop web applications, we are mostly developing user interface, that is, HTML files, and then getting data and filling html. Our data is changing, and our HTML structure is relatively stable. That is to say, the content in html is changing. We must first distinguish between the changing and the unchanging parts.
Most of our work is to integrate the dynamic content into the unchanging structure of html.
function createPost(title, content) { let post = document.querySelector(".wrapper"); let header = document.createElement("h1"); let body = document.createElement("p"); header.textContent = title; body.textContent = content; post.appendChild(header); post.appendChild(body) body.addpend(div); return { post } }
When we started, I think we all added templates dynamically. We created a dom tree with createElement and added it to html. Then the data was updated. We are updating the content of these nodes. It must be common for everyone to do this, though painfully. Because this is too abstract and not intuitive.
<template id="post"> <h1>{{title}}</h1> <template is="dom-repeat" items={{items}}> <div>{{item.name}}</div> </template> </template>
With the tag template, our work becomes much simpler, and the definition in template is closer to what we render in the end.
Template
If it is not easy to write a good template system, a good template system should have the following characteristics
- Development experience
As developers, the requirement for templates is WYSIWYG. Although jsx gives us a good experience, these templates are written in javascript. To develop templates, we usually need to study the expressions of some templates to write the required templates. These templates usually need to be compiled, so you also need to understand the tools for compiling templates. - quick start
The speed of rendering templates is also a problem - Instant updating
Apace - As a bridge between javascript and html
If our template is written in javascript, then the template must be less intuitive. If written in html, this is intuitive, but access to data is also a problem, data update we need to update the template.
That's good to say for half a day. Our protagonist is on the stage today.
- html templates that can be written in javascript
- Quick Start and Update
- Small volume
- Easy to use, API support extensible
html templates that can be written in javascript
- boot fast
- update Fast
- bridge javascript and html
const helloTemplate = (name) => html`<div>Hello ${name}!</div>`;
Here you may notice that using es6 template grammar, we can get WYG effect by supporting multiple lines, and using es6 expression ${name} here we don't need other costs to learn.
html` <div> <h1>${title}</h1> <body>${content}</body> </div> `
Let's assign our html string to JavaScript variables later.
- One question is whether we create html as a string or a DOM element?
It would be meaningless to simply generate an html string and then assign it as innerhtml. If the return is a dom, then we need to reconstruct the whole DOM tree.
Here returns a template Result result, which is an object with a template reference and data.
render(helloTemplate('zidea'), document.body);
The render method renders the template on the first call, and then updates the data of the template.
render(helloTemplate('zidea'), document.body); render(helloTemplate('matthew'), document.body); render(helloTemplate('jang'), document.body);
Is it different from react?
It looks like jsx on the surface, but it's just JavaScript grammar, not a new one. hit-html has no concept of virtual dom and no diff checking.
UI=f(state)
This reminds us of redux and f, where is a pure function, input state output UI, according to the determined state input, output UI is determined.
const postTemplate =(title,content) => html` <div> <h1>${title}</h1> <body>${content}</body> <div>${index}</div> </div> ` render(postTemplate('angular tutorial','angular tutorial content '), document.body);
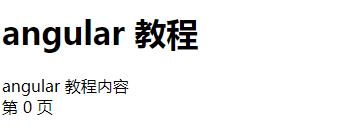
let header = (title) => html` <h1>${title}</h1> ` const postTemplate =(title,content) => html` <div> ${header(title)} <body>${content}</body> <div>The first ${index} page</div> </div> `
Nesting is supported in hit-html, nesting is supported in HTML method, nesting can be layer by layer.
let user = { loggedIn:true, name:'matthew' } const helloTemplate = (name) => html`<div>Hello ${name}!</div>`; let index = 0; let header = (title) => html` <h1>${title}</h1> ` let message = html` //Welcome ${user.name} ` const postTemplate =(title,content) => html` <div> ${message} ${header(title)} <body>${content}</body> <div>The first ${index} page</div> </div> `
You can control the template by branch statements, and you can see its benefits. We can easily control the logic without learning a new template language.
if(user.loggedIn){ message = html` Welcome ${user.name} ` }else{ message = html` Please login first. ` }
The traversal of sets is also native.
<div> ${['apple','banana','cherry']} </div>
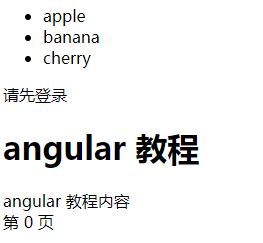
<ul> ${ ['apple','banana','cherry'].map((item)=> html`<li>${item}</li>` ) } </ul>
What amazes us is that it also supports DOM-style embedding, and you may already feel the joy of development.
const titleDom = document.createElement('h1'); titleDom.textContent = "Title" const postTemplate =(title,content) => html` <div> ${titleDom} </div> <ul> ${ ['apple','banana','cherry'].map((item)=> html`<li>${item}</li>` ) } </ul> <div> ${message} ${header(title)} <body>${content}</body> <div>The first ${index} page</div> </div> `