Official introduction: FreeMarker is a template engine: a general tool based on template and data to be changed, and used to generate output text (HTML web page, email, configuration file, source code, etc.). It is not for end users, but a Java class library, a component that programmers can embed in their products.
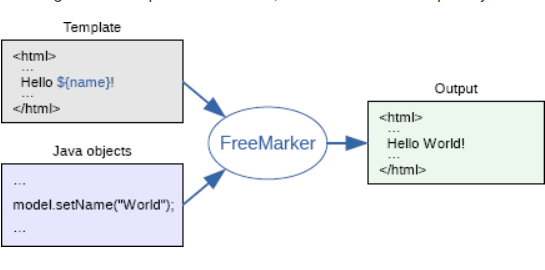
- The final project contents are as follows:
01. First of all, use maven to build a web project packaged in the way of war
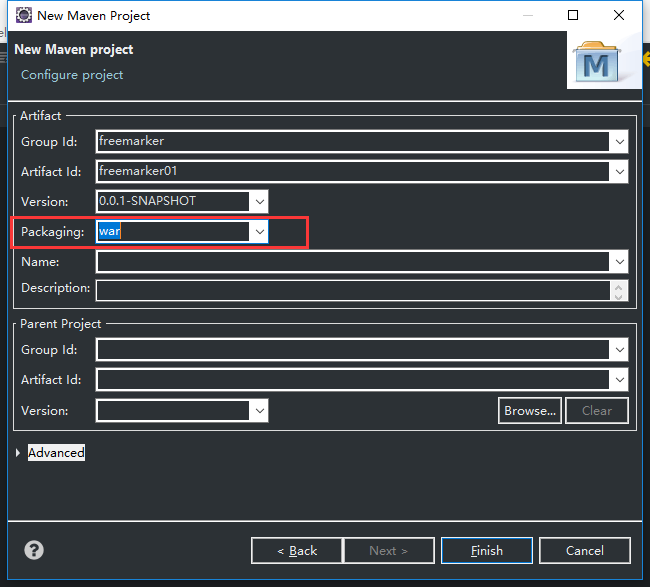
- After finish ing, you can see the following error
- And then solve it
02. Add dependency
- Spring MVC dependency
<!-- springmvc start -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.7.RELEASE</version>
</dependency>
<!-- springmvc end -->
- freemarker's dependence
<!-- freeMarker start -->
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.23</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>4.3.7.RELEASE</version>
</dependency>
<!-- freeMarker end -->
03. Configuration of web.xml
<!-- To configure DispatcherServlet -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<!-- Appoint SpringMVC Location and name of the profile
if SpringMVC The format and location of the configuration file name of: /WEB-INF/servlet-name + "-servlet.xml"
The following can be omitted init-param Code. There servlet-name yes springmvc. Namely/WEB-INF/springmvc-servlet.xml
-->
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:/applicationContext-web.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<!-- Web request -->
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<!-- servlet Knowledge point: handle all requests -->
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- Character set filter -->
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
04. Create a configuration file for springmvc
- applicationContext-web.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/contexthttp://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/mvchttp://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd">
<!-- Configure self scanning packages -->
<context:component-scan base-package="freemarker" />
<!-- integration Freemarker -->
<bean id="freemarkerConfig" class="org.springframework.web.servlet.view.freemarker.FreeMarkerConfigurer">
<property name="templateLoaderPath" value="/WEB-INF/templates"/>
</bean>
<!-- Configure view resolver -->
<bean id="viewResolver" class="org.springframework.web.servlet.view.freemarker.FreeMarkerViewResolver">
<property name="prefix" value=""/>
<property name="suffix" value=".ftl"/>
<property name="contentType" value="text/html; charset=UTF-8"/>
</bean>
<!-- Configure annotation driver -->
<mvc:annotation-driven />
<!-- Configure the page to jump directly without going through Controller layer http://localhost:8080/freemarker01/index
And then I'll jump to WEB-INF/templates/index.html page
-->
<mvc:view-controller path="/index" view-name="index"/>
</beans>
05. Create the templates directory in the WEB-INF directory, and set the index.ftl page to jump directly
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<!-- <meta http-equiv="refresh" content="0; url=hello.ftl" /> -->
<title>Insert title here</title>
</head>
<body>
<a href="helloFtl">hello</a>
</body>
</html>
06. Create a class in the controller layer
package freemarker.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class Hello {
@RequestMapping(value = "helloFtl")
public String helloFtl(Model model) {
model.addAttribute("hello", "hello world!");
return "helloFtl";
}
}
07. Create a hellofetl.ftl file in the templates directory
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
${hello}
</body>
</html>
- After starting tomcat, enter the following URL to see the effect:
- Click the hello link to get the data and jump to the page from the controller layer
java back end life