I. What is v-if?
v-if is used to render elements according to the true or false conditions of the values of expressions, and can be applied automatically when Vue inserts/updates/deletes elements Transitional effect.
v-if is a true conditional rendering, because it ensures that conditional blocks properly destroy and reconstruct event listeners and subcomponents within conditional blocks when switching.
II. v-if analysis
v-if generates or removes an element in DOM based entirely on the value of the expression. If the v-if expression is assigned false, the corresponding element will be removed from the DOM; otherwise, a clone of the corresponding element will be re-inserted into the DOM.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>pejeco</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<div id="example01">
<p v-if="yes">yes</p>
<p v-if="no">no</p>
<p v-if="age>25">Age:{{age}}</p>
<p v-if="name.indexOf('lin')>0">Name:{{name}}</p>
</div>
<script src="js/vue.js"></script>
<script type="text/javascript">
var vm= new Vue({
el:"#example01",
data:{
yes:true,//Really, insert
no: false,//False, deleted
age:29,
name:'colin'
}
})
</script>
</body>
</html>
Design sketch:
Because v-if is an instruction, it needs to be added to an element. But if you want to switch multiple elements, you can use the < template > element as a wrapping element and use v-if on it. The final rendering result will not contain it.
<div id="example">
<template v-if="ok">
<h1>Title</h1>
<p>Paragraph1</p>
<p>Paragraph2</p>
</template>
</div>
js:
var vm= new Vue({
el:"#example",
data:{
ok:true
}
})
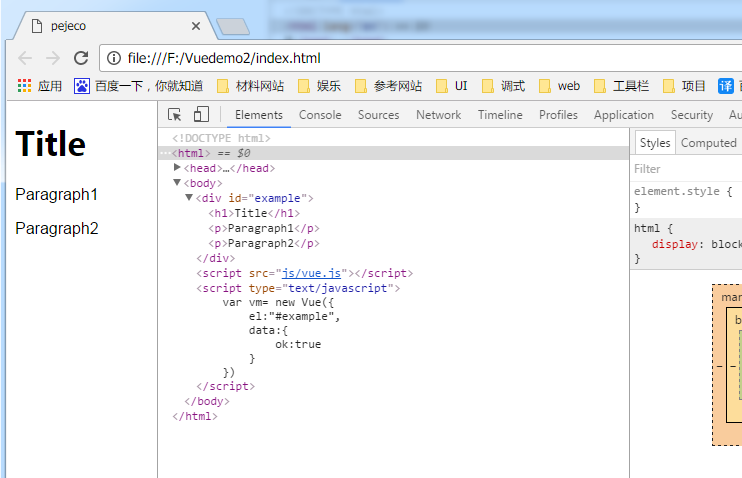
v-if is inert -- if the initial rendering condition is false, do nothing, and start local compilation when the condition first becomes true (the compilation is cached)
Do you think of display: none in css? It is also useful in vue to represent the v-show of displaying.
3.v-show: Elements are always compiled and retained, simply switched based on CSS.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>pejeco</title>
</head>
<div id="example"v-show="isShow" style="display: none;">
<h1>Title</h1>
<p>Paragraph1</p>
<p>Paragraph2</p>
</div>
<script src="js/vue.js"></script>
<script type="text/javascript">
var vm= new Vue({
el:"#example",
data:{
isShow:true
}
})
</script>
</body>
</html>
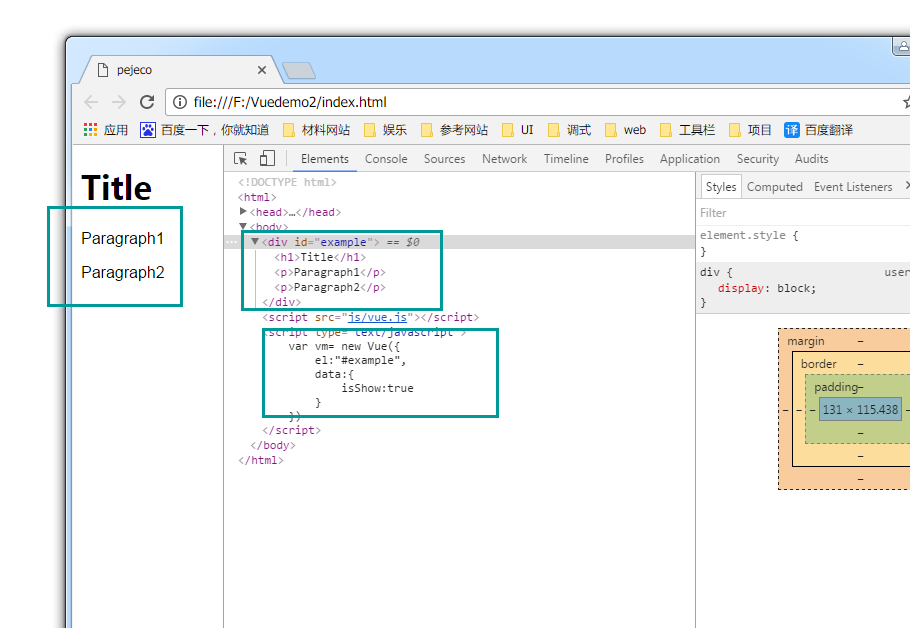
When this is the case
<div id="example"v-show="isShow">
<h1>Title</h1>
<p>Paragraph1</p>
<p>Paragraph2</p>
</div>
<script src="js/vue.js"></script>
<script type="text/javascript">
var vm= new Vue({
el:"#example",
data:{
isShow:false
}
})
</script>
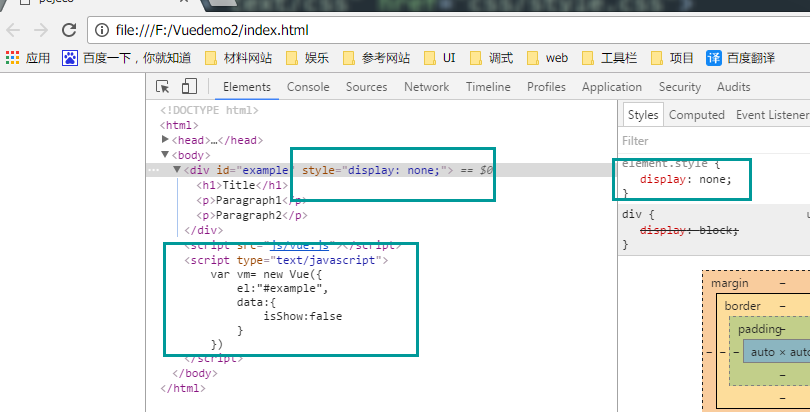
By contrast, we can get that: v-show control is implicit, it modifies the element style of elements through js code, if value is false, set display: none; if value is true, set display:'; so when value is true, only displaying effect in element style can be removed, and displaying effect in css can not be overwritten;
Note: When hiding html, don't write display: none in css
<style type="text/css">
#example{
display: none;
}
</style>
<div id="example"v-show="isShow">
<h1>Title</h1>
<p>Paragraph1</p>
<p>Paragraph2</p>
</div>
In this case, v-show is ineffective
So: if you use v-show to hide DOM before vue parsing, try to set the display value in the style attribute instead of in the css file.
<div id="example"v-show="isShow" style="display: none;">
Speaking of else, do you think that there is a corresponding instruction v-else in Vue?
IV.v-else: As the name implies, v-else means else in Javascript. It must follow v-if and act as an else function.
(1) v-if and v-else
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>pejeco</title> </head> <div id="example"> <p v-if="ok">I want to eat apples.</p> <p v-else="ok">I want to eat pears.</p> </div> <script src="js/vue.js"></script> <script type="text/javascript"> var vm3 = new Vue({ el:'#example', data:{ ok:false } }) </script> </body> </html>
The effect is as follows:
(2) v-else-if: Represents multiple times, between v-if and v-else
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>pejeco</title>
</head>
<div id="app">
<div v-if="type === 'A'">
A
</div>
<div v-else-if="type === 'B'">
B
</div>
<div v-else-if="type === 'C'">
C
</div>
<div v-else>
Not A/B/C
</div>
</div>
<script src="js/vue.js"></script>
<script type="text/javascript">
var ve = new Vue({
el: '#app',
data: {
type: 'C'
}
})
</script>
</body>
</html>
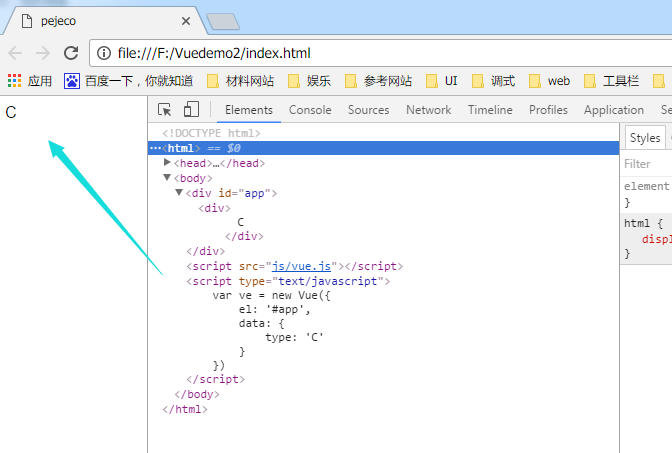
V. Differences between v-if and v-show
(1) Similarities
All DOM elements are dynamically displayed
(2) Differences
1. Means:
v-if adds or deletes DOM elements dynamically to the DOM tree;
v-show controls explicit and implicit by setting display ing style attributes of DOM elements.
2. Compiling process:
The v-if handover has a local compilation/uninstallation process, in which the internal event monitoring and sub-components are properly destroyed and reconstructed.
v-show is simply based on css handover;
3. Compilation conditions:
v-if is lazy and does nothing if the initial condition is false; only when the condition first becomes true does local compilation begin (is the compilation cached? After the compilation is cached, local unloading is performed when switching.
v-show is compiled under any conditions (whether the first condition is true) and then cached, and DOM elements are retained;
4. Performance consumption:
v-if has higher switching consumption;
v-show has higher initial rendering consumption;
5. Use scenarios:
v-if is suitable for operating conditions and is unlikely to change;
v-show is suitable for frequent switching.
Generally speaking, v-if has higher switching consumption, while v-show has higher initial rendering consumption. Therefore, it is better to use v-show if frequent switching is required, and it is better to use v-if if if runtime conditions are unlikely to change.