The Difference and Relation between hashCode and equals
Keywords:
Java
Attribute
I. The Role of equals Method
1. By default (without overriding equals method), equals method calls the equals method of Object class, while Object's equals method is mainly used to determine whether the memory address reference of the object is the same address (the same object).
2. If the equals method is covered in the class, then the function of the equals method should be determined according to the specific code. After the coverage, the object is usually judged by whether the content of the object is equal or not.
The equals method code is not overwritten as follows:
- //Student class
- public class Student {
- private int age;
- private String name;
-
- public Student() {
- }
- public Student(int age, String name) {
- super();
- this.age = age;
- this.name = name;
- }
- public int getAge() {
- return age;
- }
- public String getName() {
- return name;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setName(String name) {
- this.name = name;
- }
- }
The test code is as follows:
- import java.util.HashSet;
- import java.util.LinkedList;
- import java.util.Set;
-
-
- public class EqualsTest {
- public static void main(String[] args) {
- LinkedList<Student> list = new LinkedList<Student>();
- Set<Student> set = new HashSet<Student>();
- Student stu1 = new Student(3,"Zhang San");
- Student stu2 = new Student(3,"Zhang San");
- System.out.println("stu1 == stu2 : "+(stu1 == stu2));
- System.out.println("stu1.equals(stu2) : "+stu1.equals(stu2));
- list.add(stu1);
- list.add(stu2);
- System.out.println("list size:"+ list.size());
-
- set.add(stu1);
- set.add(stu2);
- System.out.println("set size:"+ set.size());
- }
-
- }
Operation results:
stu1 == stu2 : false
stu1.equals(stu2) :
false
list size:2
set size:2
Result analysis: Student class does not override equals method, stu1 calls equals method is actually called Object's equals method. So the object memory address is equal to judge whether the object is equal. Because two new objects have different memory addresses, stu1.equals(stu2) is false.
3. Let's override the equals method (age and name attributes) so that the Student class can determine whether the objects are equal by judging whether the contents of the objects are equal.
Overwritten Student class:
- //Student class
- public class Student {
- private int age;
- private String name;
-
- public Student() {
- }
- public Student(int age, String name) {
- super();
- this.age = age;
- this.name = name;
- }
- public int getAge() {
- return age;
- }
- public String getName() {
- return name;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setName(String name) {
- this.name = name;
- }
- @Override
- public boolean equals(Object obj) {
- if (this == obj)
- return true;
- if (obj == null)
- return false;
- if (getClass() != obj.getClass())
- return false;
- Student other = (Student) obj;
- if (age != other.age)
- return false;
- if (name == null) {
- if (other.name != null)
- return false;
- } else if (!name.equals(other.name))
- return false;
- return true;
- }
-
- }
Operation results:
stu1 == stu2 : false
stu1.equals(stu2) : true
list size:2
set size:2
Result analysis: Because the age and name attributes of the two objects of Student are equal, and they are judged by covering equals method. The stu1.equals(stu2) shown is true. Note that the size s of list s and set s tested above are both 2
HashCode
4. By running the above code, we know that the equals method has taken effect. Next, we will cover the hashCode method (which generates hashcode by the age and name attributes) and not the equals method, where the Hash code is generated by age and name.
The Student class after overriding hashcode:
- //Student class
- public class Student {
- private int age;
- private String name;
-
- public Student() {
- }
- public Student(int age, String name) {
- super();
- this.age = age;
- this.name = name;
- }
- public int getAge() {
- return age;
- }
- public String getName() {
- return name;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setName(String name) {
- this.name = name;
- }
- @Override
- public int hashCode() {
- final int prime = 31;
- int result = 1;
- result = prime * result + age;
- result = prime * result + ((name == null) ? 0 : name.hashCode());
- return result;
- }
- }
Operation results:
stu1 == stu2 : false
stu1.equals(stu2) : false
list size:2
hashCode :775943
hashCode :775943
set size:2
Result analysis: We did not cover equals method but hashCode method. Although the two objects have the same hashCode, when stu1 and stu2 are put into set set set set, because the two objects compared by equals method are false, the hashcode values of the two objects are not compared.
5. Let's cover the equals method and hashCode method.
The Student code is as follows:
- //Student class
- public class Student {
- private int age;
- private String name;
- public Student() {
- }
- public Student(int age, String name) {
- super();
- this.age = age;
- this.name = name;
- }
- public int getAge() {
- return age;
- }
- public String getName() {
- return name;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setName(String name) {
- this.name = name;
- }
- @Override
- public int hashCode() {
- final int prime = 31;
- int result = 1;
- result = prime * result + age;
- result = prime * result + ((name == null) ? 0 : name.hashCode());
- System.out.println("hashCode : "+ result);
- return result;
- }
- @Override
- public boolean equals(Object obj) {
- if (this == obj)
- return true;
- if (obj == null)
- return false;
- if (getClass() != obj.getClass())
- return false;
- Student other = (Student) obj;
- if (age != other.age)
- return false;
- if (name == null) {
- if (other.name != null)
- return false;
- } else if (!name.equals(other.name))
- return false;
- return true;
- }
-
- }
Operation results:
stu1 == stu2 : false
stu1.equals(stu2) :true
list size:2
hashCode :775943
hashCode :775943
set size:1
Result analysis: stu1 and stu2 are equal by equals method, and the hashCode value returned is the same, so only one object is put into set set set.
6. Let's make the two object equals methods equal, but hashCode values are not equal.
The code for the Student class is as follows:
- //Student class
- public class Student {
- private int age;
- private String name;
- <span style="color:#ff0000;">private static int index=5;</span>
- public Student() {
- }
- public Student(int age, String name) {
- super();
- this.age = age;
- this.name = name;
- }
- public int getAge() {
- return age;
- }
- public String getName() {
- return name;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setName(String name) {
- this.name = name;
- }
- @Override
- public int hashCode() {
- final int prime = 31;
- int result = 1;
- result = prime * result + <span style="color:#ff0000;">(age+index++)</span>;
- result = prime * result + ((name == null) ? 0 : name.hashCode());
- <span style="color:#ff0000;">System.out.println("result :"+result);</span>
- return result;
- }
- @Override
- public boolean equals(Object obj) {
- if (this == obj)
- return true;
- if (obj == null)
- return false;
- if (getClass() != obj.getClass())
- return false;
- Student other = (Student) obj;
- if (age != other.age)
- return false;
- if (name == null) {
- if (other.name != null)
- return false;
- } else if (!name.equals(other.name))
- return false;
- return true;
- }
-
- }
Operation results:
stu1 == stu2 : false
stu1.equals(stu2) : true
list size:2
hashCode :776098
hashCode :776129
set size:2
Result analysis: Although stu1 and stu2 are equal by equals method, the hashcode values of the two objects are not equal, so stu1 and stu2 are considered to be two different objects when they are put into set sets.
7. Modify an attribute value of stu1
The Student code is as follows:
- //Student class
- public class Student {
- private int age;
- private String name;
- public Student() {
- }
- public Student(int age, String name) {
- super();
- this.age = age;
- this.name = name;
- }
- public int getAge() {
- return age;
- }
- public String getName() {
- return name;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public void setName(String name) {
- this.name = name;
- }
- @Override
- public int hashCode() {
- final int prime = 31;
- int result = 1;
- result = prime * result + age;
- result = prime * result + ((name == null) ? 0 : name.hashCode());
- System.out.println("hashCode : "+ result);
- return result;
- }
- @Override
- public boolean equals(Object obj) {
- if (this == obj)
- return true;
- if (obj == null)
- return false;
- if (getClass() != obj.getClass())
- return false;
- Student other = (Student) obj;
- if (age != other.age)
- return false;
- if (name == null) {
- if (other.name != null)
- return false;
- } else if (!name.equals(other.name))
- return false;
- return true;
- }
-
- }
The test code is as follows:
- import java.util.HashSet;
- import java.util.LinkedList;
- import java.util.Set;
-
-
- public class EqualsTest {
- public static void main(String[] args) {
- LinkedList<Student> list = new LinkedList<Student>();
- Set<Student> set = new HashSet<Student>();
- Student stu1 = new Student(3,"Zhang San");
- Student stu2 = new Student(3,"Zhang San");
- System.out.println("stu1 == stu2 : "+(stu1 == stu2));
- System.out.println("stu1.equals(stu2) : "+stu1.equals(stu2));
- list.add(stu1);
- list.add(stu2);
- System.out.println("list size:"+ list.size());
-
- set.add(stu1);
- set.add(stu2);
- System.out.println("set size:"+ set.size());
- stu1.setAge(34);
- System.out.println("remove stu1 : "+set.remove(stu1));
- System.out.println("set size:"+ set.size());
- }
-
- }
Operation results:
stu1 == stu2 : false
stu1.equals(stu2) : true
list size:2
hashCode : 775943
hashCode : 775943
set size:1
hashCode : 776904
remove stu1 : false
set size:1
Result analysis:
When we store an object in a set, if the attribute of the object participates in hashcode calculation, then we can not modify those attributes of the object participates in hashcode calculation later, otherwise it will cause unexpected errors. As in testing, stu1 objects cannot be removed.
Conclusion:
1. The equals method is used to compare whether the content of the object is equal (after coverage)
2. hashcode methods are only used in Collections
3. When the equals method is overridden, whether the objects are equal or not will be compared by the equals method after overridden (to judge whether the contents of the objects are equal).
4. When putting an object into a collection, we first determine whether the hashcode value of the object to be put in is equal to that of any element in the collection, and if not, put the object directly into the collection. If the hashcode value is equal, then the equals method is used to determine whether the object to be placed is equal to any object in the set. If the equals judgment is not equal, the element is directly put into the set, otherwise it is not put.
5. A flow chart for putting elements into a collection:
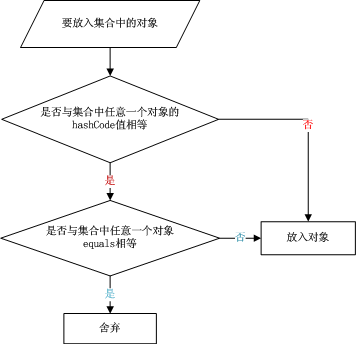


6. The source code of add method in HashSet:
- public boolean add(E e) {
- return map.put(e, PRESENT)==null;
- }
map.put source code:
- <pre name="code" class="java"> public V put(K key, V value) {
- if (key == null)
- return putForNullKey(value);
- int hash = hash(key.hashCode());
- int i = indexFor(hash, table.length);
- for (Entry<K,V> e = table[i]; e != null; e = e.next) {
- Object k;
- if (e.hash == hash && ((k = e.key) == key || key.equals(k))) {
- V oldValue = e.value;
- e.value = value;
- e.recordAccess(this);
- return oldValue;
- }
- }
-
- modCount++;
- addEntry(hash, key, value, i);
- return null;
- }</pre>
- <pre></pre>
- <pre></pre>
Reproduced in: https://my.oschina.net/u/580135/blog/612339
Posted by PBD817 on Thu, 12 Sep 2019 00:08:59 -0700