Photo by Markus spike on unsplash
Python has built us a large number of function functions, 69 of which are listed in the official documents. Some of them are often encountered in normal development, and some of them are rarely used. Here are the eight functions most frequently used by developers and their detailed usage
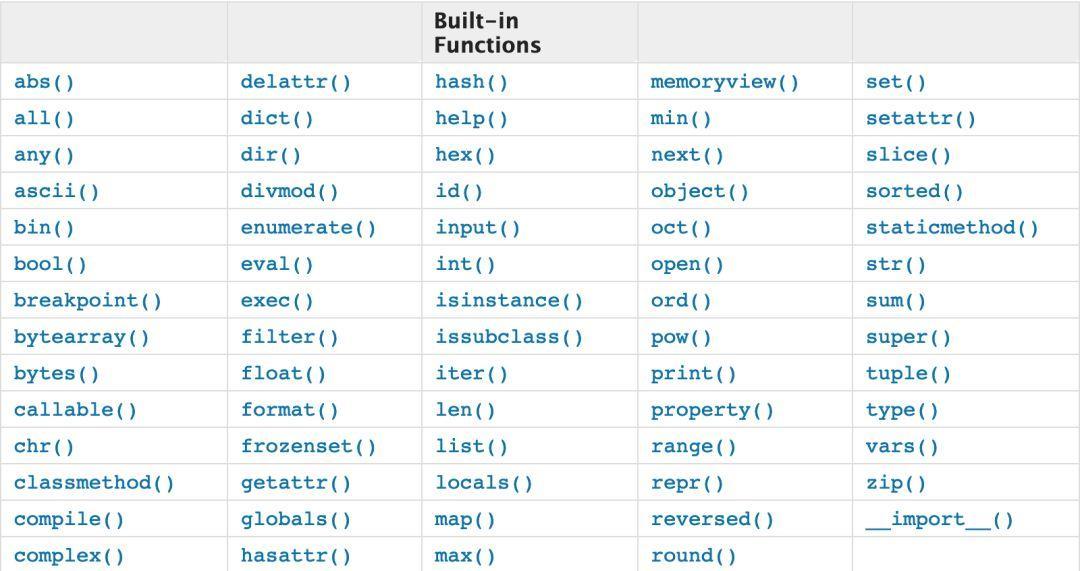
print()
Print function is the first function you learn from Python. It outputs objects to standard output stream and can print any number of objects. The specific definition of the function is as follows:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
Objects are variable parameters, so you can print any number of objects at the same time
>>> print(1,2,3)1 2 3
Each object is separated by a space by default, and can be separated by commas by specifying the sep parameter
>>> print(1,2,3, sep=',') 1,2,3
The default output of the object is the standard output stream. You can also save the content to a file
>>> print(1,2,3, sep=',', file=open("hello.txt", "w"))
isinstance()
The isinstance function can be used to determine whether an object belongs to an instance of a class and the definition of the function
isinstance(object, classinfo)
classinfo can be a single type object or a tuple composed of multiple types of objects. If the object type is any one of the tuples, it will return True. Otherwise, it will return False
>>> isinstance(1, (int, str)) True >>> isinstance("", (int, str)) True >>> isinstance([], dict) False
range()
The range function is a factory method, which is used to construct a continuous immutable integer sequence object from [start, stop) (excluding stop). The sequence function is very similar to the list. Function definition:
range([start,] stop [, step]) -> range object
-
start optional parameter, starting point of sequence, default is 0
-
stop required parameter, end of sequence (not included)
-
Step optional parameter, step length of sequence, default is 1, the generated element rule is r[i] = start + step*i
Generate a list of 0-5
>>> >>> range(5) range(0, 5) >>> >>> list(range(5)) [0, 1, 2, 3, 4] >>>
Starting from 0 by default, 5 integers between 0 and 4 are generated, excluding 5. The default value of step is 1. Each time, 1 is added to the previous one
If you want to repeat an operation n times, you can use the for loop to configure the range function
>>> for i in range(3): ... print("hello python") ... hello python hello python hello python
The step length is 2.
>>> range(1, 10, 2) range(1, 10, 2) >>> list(range(1, 10, 2)) [1, 3, 5, 7, 9]
Starting from 1 and ending at 10, step size is 2. Each time, add 2 to the previous element to form an odd number between 1 and 10.
enumerate()
It is used to enumerate the objects that can be iterated. At the same time, the following table index values of each element can be obtained. Function definition:
enumerate(iterable, start=0)
For example:
>>> for index, value in enumerate("python"): ... print(index, value) ... 0 p 1 y 2 t 3 h 4 o 5 n
Index starts from 0 by default. If you explicitly specify the parameter start, the subscript index starts from start
>>> for index, value in enumerate("python", start=1): ... print(index, value) ... 1 p 2 y 3 t 4 h 5 o 6 n
If you do not use the enumerate function, you need more code to get the subscript index of the element:
def my_enumerate(sequence, start=0): n = start for e in sequence: yield n, e n += 1 >>> for index, value in my_enumerate("python"): print(index, value) 0 p 1 y 2 t 3 h 4 o 5 n
len
Len is used to get the number of elements in the container object. For example, len function can be used to judge whether the list is empty
>>> len([1,2,3]) 3 >>> len("python") 6 >>> if len([]) == 0: pass
Not all objects support len operation, for example:
>>> len(True) Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: object of type 'bool' has no len()
In addition to sequence objects and collection objects, custom classes must implement methods that can act on len functions
reversed()
reversed() reverses the sequence object. You can reverse the string, the list, and the tuple
>>> list(reversed([1,2,3]))
[3, 2, 1]
open()
The open function is used to construct a file object, which can be read or written after construction
open(file, mode='r', encoding=None)
Read operation
#Python Learning group 592539176 # Open file from current path test.txt, Read by default >>>f = open("test.txt") >>>f.read() ...
Sometimes you need to specify the encoding format, otherwise you will encounter garbled code
f = open("test.txt", encoding='utf8')
Write operation
>>>f = open("hello.text", 'w', encoding='utf8') >>>f.write("hello python"))
When there is content in the file, the original content will not be overwritten. If you don't want to be overwritten, you can directly append the new content to the end of the file. You can use the "a" mode
f = open("hello.text", 'a', encoding='utf8') f.write("!!!")
sorted()
sroted is to reorder the list. Of course, other objects that can be iterated can be reordered. A new object is returned and the original object remains unchanged
>>> sorted([1,4,2,1,0])
[0, 1, 1, 2, 4]