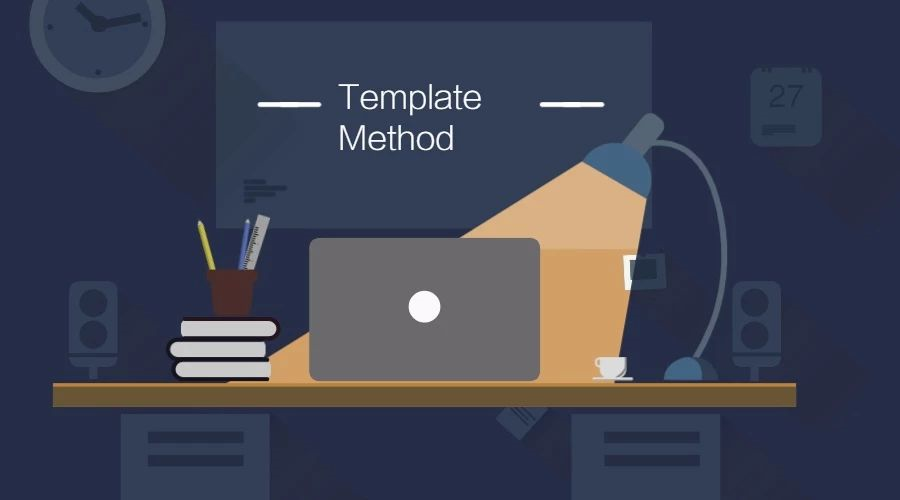
_Template method mode defines the skeleton of an algorithm in an operation and delays some steps to a subclass, which allows the subclass to redefine certain steps of the algorithm without changing the structure of the algorithm.
_Popular point of understanding is to complete a thing, there are fixed number of steps, but each step depends on the object, and the implementation details are different; you can define a general method to complete the thing in the parent class, and call the implementation method of each step according to the steps required to complete the event.The implementation of each step is done by subclasses.
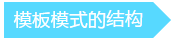
-
Abstract class template
_Implements the template method and defines the framework of the algorithm. -
Concrete class template
_Implement abstract methods in abstract classes, that is, the specific implementation details of different objects.
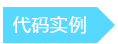
The story of A and B going to take the exam.
- This is A's questionnaire:
public class TestPageA { // Question 1 public void testQuestion1() { System.out.println("Yang passed it and later gave it to Guo Jing. It is possible that the basalt that was made into a skyscraper or a dragon slaughter knife is () a.Ball-ground iron b.Tinplate c.High Speed Alloy Steel " + "Carbon fiber"); System.out.println("Answer: b"); } // Question 2 public void testQuestion2 () { System.out.println("Yang Cuo, Cheng Ying and Lu Wushuang shovel the love flower, causing[] a. Make this plant harmless b.Extinction of rare species" + "C. Destroying the ecological balance of that biosphere d. Causes desertification in this area"); System.out.println(" Answer: a"); } // Question 3 public void testQuestion3() { System.out.println("Blue Phoenix causes Master Hua Shan and disciple Taogu Six Immortals to swallow and spit up. If you are a doctor, what medicine will you prescribe to them?[ 1a.Aspirin b.Niuhuang Jiedu Tablet c.Fluoroleucic Acid d.Let them drink a lot of raw milk e.Above:Not at all"); System.out.println("Answer: c"); } }
- This is the questionnaire for B:
public class TestPageB { // Question 1 public void testQuestion1() { System.out.println("Yang passed it and later gave it to Guo Jing. It is possible that the basalt that was made into a skyscraper or a dragon slaughter knife is () a.Ball-ground iron b.Tinplate c.High Speed Alloy Steel " + "Carbon fiber"); System.out.println("Answer: a"); } // Question 2 public void testQuestion2 () { System.out.println("Yang Cuo, Cheng Ying and Lu Wushuang shovel the love flower, causing[] a. Make this plant harmless b.Extinction of rare species" + "C. Destroying the ecological balance of that biosphere d. Causes desertification in this area"); System.out.println(" Answer: b"); } // Question 3 public void testQuestion3() { System.out.println("Blue Phoenix causes Master Hua Shan and disciple Taogu Six Immortals to swallow and spit up. If you are a doctor, what medicine will you prescribe to them?[ 1a.Aspirin b.Niuhuang Jiedu Tablet c.Fluoroleucic Acid d.Let them drink a lot of raw milk e.Above:Not at all"); System.out.println("Answer: a"); } }
- Test class:
public class Test { public static void main(String[] args) { System.out.println("Small A Test Paper for"); TestPageA testPageA = new TestPageA(); testPageA.testQuestion1(); testPageA.testQuestion2(); testPageA.testQuestion3(); System.out.println("Small B Test Paper for"); TestPageB testPageB = new TestPageB(); testPageB.testQuestion1(); testPageB.testQuestion2(); testPageB.testQuestion3(); } }
_From the code above, we can see that the test papers of A and B are not the same except for the different answers. It is easy to make mistakes and difficult to maintain.If the teacher changes the subject, both of them change the code.Next, let's solve it using the template approach.
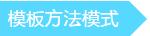
- Abstract Class Role:
public abstract class TestPage { public void testQuestion1() { System.out.println("Yang passed it and later gave it to Guo Jing. It is possible that the basalt that was made into a skyscraper or a dragon slaughter knife is () a.Ball-ground iron b.Tinplate c.High Speed Alloy Steel " + "Carbon fiber"); if (isAnswer()) { System.out.println("Answer:"+answer1()); } else { System.out.println("Answer: Students did not fill in the answer"); } } public void testQuestion2 () { System.out.println("Yang Cuo, Cheng Ying and Lu Wushuang shovel the love flower, causing[] a. Make this plant harmless b.Extinction of rare species" + "C. Destroying the ecological balance of that biosphere d. Causes desertification in this area"); System.out.println("Answer:"+answer2()); } // Question 3 public void testQuestion3() { System.out.println("Blue Phoenix causes Master Hua Shan and disciple Taogu Six Immortals to swallow and spit up. If you are a doctor, what medicine will you prescribe to them?[ 1a.Aspirin b.Niuhuang Jiedu Tablet c.Fluoroleucic Acid d.Let them drink a lot of raw milk e.Above:Not at all"); System.out.println("Answer: "+answer3()); } // Answer Question Method 1 public abstract String answer1(); // Answer Question Method 2 public abstract String answer2(); // Answer Question Method 3 public abstract String answer3(); // Whether to fill in the answer public abstract boolean isAnswer () ; }
- Specific class (small A):
public class TestPageA extends TestPage{ @Override public String answer1() { return "b"; } @Override public String answer2() { return "a"; } @Override public String answer3() { return "c"; } @Override public boolean isAnswer() { return false; } }
- Specific Class (Small B)
public class TestPageB extends TestPage { @Override public String answer1() { return "a"; } @Override public String answer2() { return "b"; } @Override public String answer3() { return "a"; } @Override public boolean isAnswer() { return true; } }
- Test Class
public class Test { public static void main(String[] args) { System.out.println("---------Small A Test Paper for--------"); TestPage testPageA = new TestPageA(); testPageA.testQuestion1(); testPageA.testQuestion2(); testPageA.testQuestion3(); System.out.println("---------Small B Test Paper for---------"); TestPage testPageB = new TestPageB(); testPageB.testQuestion1(); testPageB.testQuestion2(); testPageB.testQuestion3(); } }
_At this time, more students will answer the test, but only fill in the answer to the multiple choice questions on the test answer template, which is the only difference for everyone.The isAnswer method added to the code, also known as the hook method in the template method pattern, determines whether a particular step needs to be executed by overriding the parent's hook method with a subclass.

_Template method patterns remove duplicate code from subclasses by moving unchanged behavior to abstract classes.

Advantage:
_Template method pattern defines an algorithm in a class, and its subclasses implement detailed processing.
_Template method is a basic technology of code reuse.They are particularly important in class libraries, which extract common behavior from them.
_Template method mode leads to a reverse control structure sometimes called the Hollywood Rule, that is,'Don't Find Us, We Find You'invokes the operations of its subclasses through a parent class (instead of invoking the parent class by the opposite subclass), which complies with the Open and Close Principle by adding new behavior to the extension of the subclasses.
Disadvantages:
_Each different implementation requires a subclass to be defined, which results in an increase in the number of classes, a larger system, and a more abstract design, but more in line with the Single Responsibility Principle, which improves the cohesion of classes.

Servlet is short for Java Servlet. Server-side programs written in Java mainly function to browse and modify data interactively and generate dynamic Web content.Every Servlet must implement a Servlet interface. GenericServlet is a generic, protocol-independent Servlet that implements the Servlet interface. HttpServlet inherits from GenericServlet and implements the Servlet interface, which provides a generic implementation for the Servlet interface to handle the HTTP protocol, so the Servlet we define only needs to inherit the HttpServletYes.
_In the service Method of HttpServlet, first get the Method name of the request, then call the corresponding doXXX Method according to the Method name, for example, if the request Method is GET, then call the doGet Method; if the request Method is POST, then call the doPost Method, HttpServlet is equivalent to defining a set of templates to handle HTTP requests; service Method is template Method, determineDefines the basic process for handling HTTP requests; methods such as doXXX are the basic methods, which can be overridden by subclasses according to the request Method; and methods in HttpServletRequest act as hook methods.

_Segments some complex algorithms, designs the fixed parts of them as template methods and parent specific methods, and implements some details that can be changed by their subclasses.
Common behavior in a subclass is extracted and aggregated into an abstract class to avoid code duplication.
_Use subclasses to determine whether a step in the parent class algorithm is executed or not, so that the subclass can reverse control the parent class.