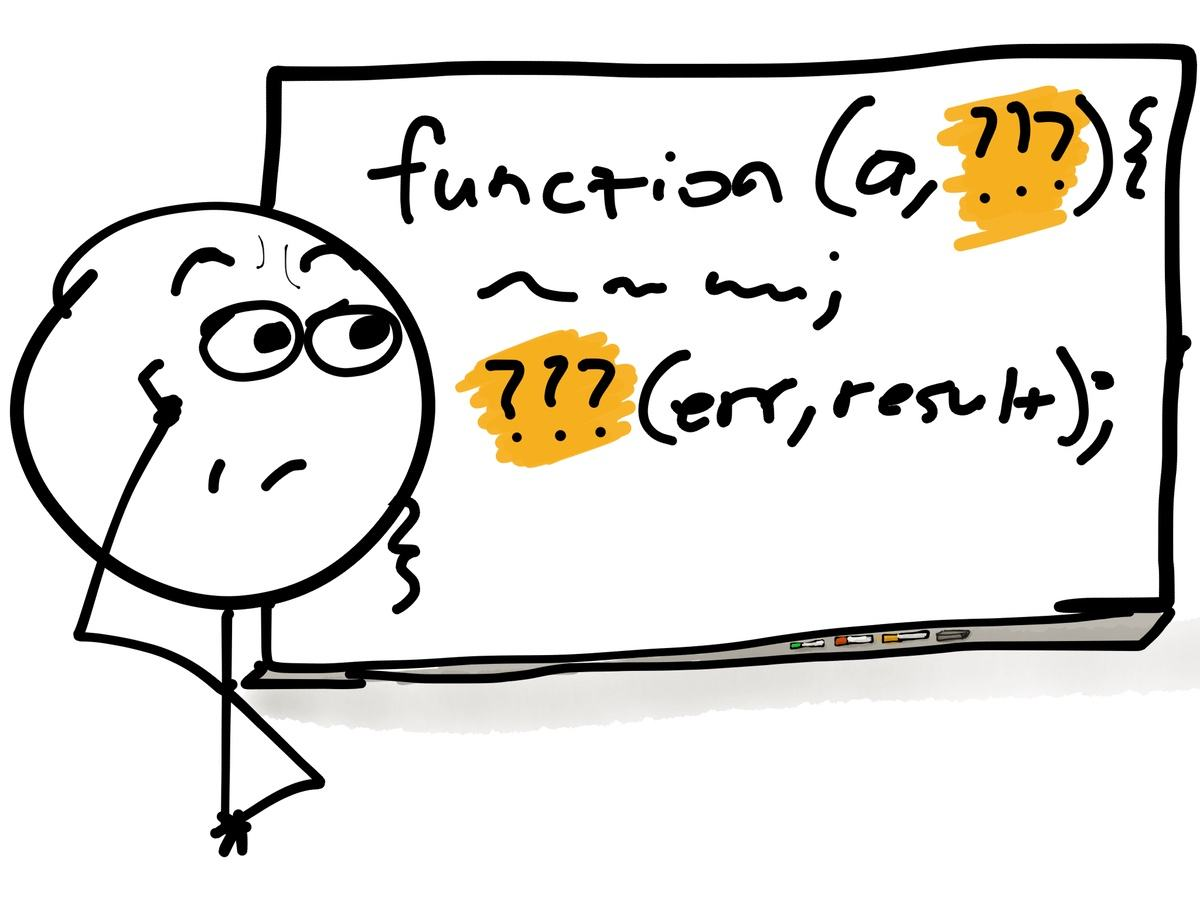
The Vue.js component provides a functional switch. When set to true, the component can become a stateless and instance free functional component. Because it's just a function, rendering costs are relatively small.
The Render function in the functional component provides the second parameter context as the context. data, props, slots, children and parent can all be accessed through context.
1 example
Here, we use functional functional components to implement an intelligent component.
html:
<div id="app"> <smart-component :data="data"></smart-component> <button @click="change('img')">Picture component</button> <button @click="change('video')">Video component</button> <button @click="change('text')">Text component</button> </div>
js:
//Picture component settings var imgOptions = { props: ['data'], render: function (createElement) { return createElement('div', [ createElement('p', 'Picture component'), createElement('img', { attrs: { src: this.data.url, height: 300, weight: 400 } }) ]); } }; //Video component settings var videoOptions = { props: ['data'], render: function (createElement) { return createElement('div', [ createElement('p', 'Video component'), createElement('video', { attrs: { src: this.data.url, controls: 'controls', autoplay: 'autoplay' } }) ]); } }; //Text component settings var textOptions = { props: ['data'], render: function (createElement) { return createElement('div', [ createElement('p', 'Text component'), createElement('p', this.data.content) ]); } }; Vue.component('smart-component', { //Set as functional component functional: true, render: function (createElement, context) { function get() { var data = context.props.data; console.log("smart-component/type:" + data.type); //Determine which type of component switch (data.type) { case 'img': return imgOptions; case 'video': return videoOptions; case 'text': return textOptions; default: console.log("data Illegal type:" + data.type); } } return createElement( get(), { props: { data: context.props.data } }, context.children ) }, props: { data: { type: Object, required: true } } }) var app = new Vue({ el: '#app', data: { data: {} }, methods: { change: function (type) { console.log("Input type:" + type); switch (type) { case 'img': this.data = { type: 'img', url: 'http://pic-bucket.ws.126.net/photo/0001/2019-02-07/E7D8PON900AO0001NOS.jpg' } break; case 'video': this.data = { type: 'video', url: 'http://wxapp.cp31.ott.cibntv.net/6773887A7F94A71DF718E212C/03002002005B836E73A0C5708529E09C1952A1-1FCF-4289-875D-AEE23D77530D.mp4?ccode=0517&duration=393&expire=18000&psid=bbd36054f9dd2b21efc4121e320f05a0&ups_client_netip=65600b48&ups_ts=1549519607&ups_userid=21780671&utid=eWrCEmi2cFsCAWoLI41wnWhW&vid=XMzc5OTM0OTAyMA&vkey=A1b479ba34ca90bcc61e3d6c3b2da5a8e&iv=1&sp=' } break; case 'text': this.data = { type: 'text', content: '<Science in wandering earth: when does the sun swallow up the earth? Scientists have given a timetable' } break; default: console.log("data Illegal type:" + type); } } }, created: function () { //Default to picture component this.change('img'); } });
Effect:
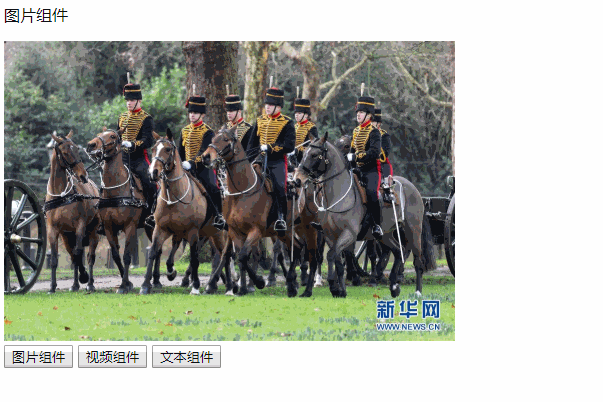
- Firstly, the image component setting object, video component setting object and text component setting object are defined, all of which take data as input parameters.
- The functional component, smart component, also takes data as the input parameter. Internally, the component type to be rendered is determined according to the get() function.
- The render function of the functional component smart component takes get() as the first parameter and the data passed in by smart component as the second parameter
return createElement( get(), { props: { data: context.props.data } }, context.children )
- The change method of the root instance app switches the data required by different components according to the type of input.
2 application scenario
Functional components are not commonly used. They should be used in the following scenarios:
- You need to select one of the various components through programming.
- children, props, or data process them before passing them to subcomponents.