The first part talks about Spring Boot data access related knowledge, this article combines the actual project Spring Boot data connection, experience its powerful and fast.
We used the previous simple project demo, jumpable to view, follow the article can be quickly built in 10 minutes (previous article: 1 minute build,web development
The current project structure is as follows:

The web directory structure of MVC is adopted and explained simply.
domain layer: This layer is used to manage javaBean entity objects;
dao layer: data access layer, accessing database;
service layer: Business Logic Layer By calling the dao layer to access the database, the interface xxservice and the implementation xxservice Impl are generally separated to facilitate code reading and management.
Controller layer: Controller controller, interacting with the interface, calling service layer method to achieve interaction with the underlying layer
Dependency Introduction and Attribute Configuration
Spring Boot provides a variety of database connections, we choose mysql database here.
Introduce mysql dependency and hibernate dependency in pom.xml:
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-validator</artifactId> <version>5.2.4.Final</version> </dependency>
Configure your own local mysql database in application. property:
spring.datasource.url=jdbc:mysql://127.0.0.1:3306/demo?useUnicode=true&characterEncoding=UTF-8 spring.datasource.username=xxxxxx spring.datasource.password=xxxxxx spring.datasource.driver-class-name=com.mysql.jdbc.Driver
Build a library demo in local mysql, create a table person, and add several test data:

Hibernate mapping
-
hibernate can help us quickly build entity classes and associate them with annotations.
Select Project Structure from the menu to configure:menu -
Select Modules - > add "+" -> Hibernate to add:
hibernate injection -
Generate hibernate.cfg.xml configuration file:
Generate xml configuration files -
At this time, the left shortcut bar of Persistence appears in the lower left corner of IDEA, click on the selected item demo, and then select Generate Persistence Mapping - > By Database Schema to automatically convert tables to entity classes in the database.
Persistence - Automatic Generation of Entity Class PersonAutomatic generation of entity classes
Entity entity class
We see that the entity class Person is automatically generated, and the code is as follows:
package com.weber.demo.domain; import javax.persistence.Basic; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; /** * Created by weber on 2017/8/31. */ @Entity public class Person { private int id; private String name; private String sexy; @Id @Column(name = "id") public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } @Basic @Column(name = "name") public String getName() { return name; } public void setName(String name) { this.name = name; } @Basic @Column(name = "sexy") public String getSexy() { return sexy; } public void setSexy(String sexy) { this.sexy = sexy; } @Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; Person person = (Person) o; if (id != person.id) return false; if (name != null ? !name.equals(person.name) : person.name != null) return false; if (sexy != null ? !sexy.equals(person.sexy) : person.sexy != null) return false; return true; } @Override public int hashCode() { int result = id; result = 31 * result + (name != null ? name.hashCode() : 0); result = 31 * result + (sexy != null ? sexy.hashCode() : 0); return result; } }
Annotation @Entity indicates that this is an entity class, annotation @Id indicates the primary key, and @Column indicates that it corresponds to database tables and columns one by one, and that it corresponds to database through annotation.
dao layer Repository class:
All of the examples presented here are described in the previous section. We only use one as a demonstration.
package com.weber.demo.dao; import com.weber.demo.domain.Person; import org.springframework.data.jpa.domain.Specification; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.data.jpa.repository.JpaSpecificationExecutor; import org.springframework.data.jpa.repository.Query; import org.springframework.data.repository.query.Param; import javax.persistence.criteria.CriteriaBuilder; import javax.persistence.criteria.CriteriaQuery; import javax.persistence.criteria.Predicate; import javax.persistence.criteria.Root; import java.util.List; /** * Created by weber on 2017/9/1. */ public interface PersonRepository extends JpaRepository<Person,Integer>,JpaSpecificationExecutor<Person> { //Counting by sex Long countBySexy(String sexy); //Delete by id void deleteById(int id); //Search by name and gender List<Person> findByIdAndName(int id,String name); //Top 10 people with this name List<Person> findFirst10ByName(String name); List<Person> findTop10ByName(String name); @Query("select p from Person p where p.id=?1") List<Person> findStudentById(int id); @Query("select p from Person p where p.id=:stuId") List<Person> findStudentByStuId(@Param("stuId")int stuId); public static Specification<Person> personIsWeber(){ return new Specification<Person>() { @Override public Predicate toPredicate(Root<Person> root, CriteriaQuery<?> criteriaQuery, CriteriaBuilder criteriaBuilder) { return criteriaBuilder.equal(root.get("name"),"weber"); } }; } List<Person> findByName(String name); }
service layer method:
service interface:
package com.weber.demo.service; import com.weber.demo.domain.Person; import java.util.List; /** * Created by weber on 2017/9/8. */ public interface PersonService { List<Person> findByName(String name); } i
Realization:
package com.weber.demo.service.Impl; import com.weber.demo.dao.PersonRepository; import com.weber.demo.domain.Person; import com.weber.demo.service.PersonService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; /** * Created by weber on 2017/9/8. */ @Service public class PersonServiceImpl implements PersonService { @Autowired PersonRepository personRepository; @Override public List<Person> findByName(String name) { List<Person> people = personRepository.findByName(name); return people; } }
Controller class:
Call the Service method, where a simple query is executed to find all the people called weber, and return to the front-end display.
package com.weber.demo.controller; import com.weber.demo.domain.Person; import com.weber.demo.service.PersonService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import java.util.List; /** * Created by weber on 2017/8/31. */ @Controller @RequestMapping("/") public class PersonController { @Autowired private PersonService personService; @RequestMapping("") public String index(Model model) { List<Person> people = personService.findByName("weber"); model.addAttribute("people", people); return "index"; } }
Entry Application:
You need to delete the previous @EnableAutoConfiguration annotation to enable it to call the database properly.
package com.weber.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
Startup project
Run demo application caiton, access http://localhost:8080/ We see that a piece of data in the database has been successfully invoked and displayed to the front end. The effect is as follows:
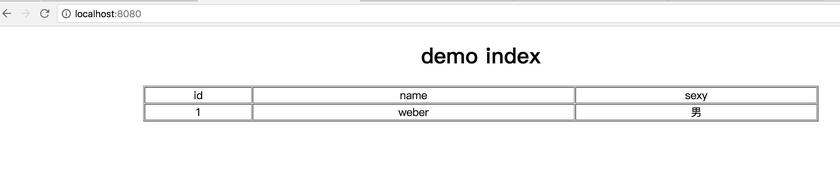
Summary
So far, we have successfully connected the database to the back end and the back end to the front end in demo.
- Hibernate allows us to quickly generate entity classes and add them to configuration files to automatically correspond to databases.
- In Spring Boot, the use of Spring Data JPA makes the previous tedious lookup operation very fast and efficient. It can easily generate query statements in the dao layer by the method of findByName, so that its data access avoids the problem of tedious sql statements.
- Continue to use Spring MVC's hierarchical structure for web development. The directory structure is clear and easy for people with old framework experience to develop and learn, and has played the role of retaining its essence and eliminating its dross.