Original: http://blog.csdn.net/hudan2714/article/details/50990359
MVC
The full name of MVC is Model View Controller. It is the abbreviation of Model View Controller. It is a model of software design. It organizes code by a method of separating business logic, data and interface display. It aggregates business logic into a component. It does not need to rewrite business logic while improving and personalizing customized interface and user interaction. MVC has been uniquely developed to map traditional input, processing and output functions in a logical graphical user interface structure.
Data relations
- View accepts user interaction requests
- View forwards the request to Controller
- Controller Operating Model for Data Update
- After data updates, Model notifies View to update data changes
- View Updates Change Data
mode
All modes are one-way communication
Structural Realization
View: Use Composite mode
View and Controller: Use Strategy mode
Model and View: Synchronize information using Observer mode
Use
View in MVC can access Model directly! Thus, the View will contain Model information, and inevitably some business logic. In MVC model, more attention is paid to the unchanged model, while there are multiple different displays of the model, and View. So, in MVC model, Model does not depend on View, but View depends on Model. Not only that, because some business logic is implemented in View, it is difficult to change View, at least those business logic can not be reused.
MVP
The full name of MVP is Model-View-Presenter. Model provides data, View is responsible for display, and Controller/Presenter is responsible for logical processing. MVP differs from MVC in that View does not use Model directly in MVP, but communicates with each other through Presenter (Controller in MVC). All interactions occur within Presenter, and in MVC View reads data directly from Model rather than through it. Controller.
Data relations
- View receives user interaction requests
- View forwards the request to Presenter
- Presenter Operates Model to Update Data
- Model notifies Presenter that the data has changed
- Presenter updates View data
Advantages of MVP
- Model and View are completely separated, and modifications do not affect each other.
- More efficient use, because all logical interactions occur in one place -- inside Presenter
- A Preseter can be used for multiple Views without changing the logic of the Presenter (because Views change more frequently than Model s).
- It's easier to test. By putting logic in Presenter, you can test logic (unit test) out of the user interface.
mode
All parts communicate in two directions.
Structural Realization
View: Use Composite mode
View and Presenter: Use Mediator mode
Model and Presenter: Synchronize information using Command mode
Differences between MVC and MVP
One of the biggest differences between MVP and MVC is whether there should be communication (or even two-way communication) between Model and View layers.
MVC and MVP Relations
MVP: It's a variant of MVC mode. In project development, UI is easy to change, and is diverse, the same data will have N display ways; business logic is relatively easy to change. In order to make the application more flexible, we expect to separate UI, logic (UI logic and business logic) and data, and MVP is a good choice. Presenter replaces Controller, which takes on more tasks and is more complex than Controller. Presenter handles events and executes the corresponding logic, which maps to the Model operation model. The code that handles how the UI works is basically in Presenter. Models and Views in MVC use Observer mode to communicate; Presenter and View in MPV use Mediator mode to communicate; Presenter operation model uses Command mode to communicate. The basic design is the same as MVC: Model stores data, View represents Model, Presenter coordinates communication between the two. View receives events in MVP and then passes them to Presenter. How to deal with these events in detail will be done by Presenter. If the UI to be implemented is complex and the related display logic is also related to the Model, an Adapter can be placed between View and Presenter. This adapter accesses Model and View to avoid the association between them. At the same time, because the Adapter implements the View interface, it can ensure that the interface with Presenter remains unchanged. This ensures the simplicity of the interface between View and Presenter without losing the flexibility of the UI.
Use
MVP implementations vary according to View implementations. Some tend to place simple logic in View and complex logic in Presenter, while others tend to place all logic in Presenter. These are called Passive View and Superivising Controller, respectively.
MVVM
MVVM is short for Model-View-ViewModel. Microsoft's WPF brings new technological experiences, such as Silverlight, audio, video, 3D, animation... This leads to more detailed and customizable software UI layer. At the technical level, WPF also brings new features such as Binding, Dependency Property, Routed Events, Command, Data Template, Control Template and so on. The origin of MVVM (Model-View-View Model) framework is a new type of application mode developed when MVP (Model-View-Presenter) mode is combined with WPF. Framework Framework. It is based on the original MVP framework and integrates the new features of WPF to meet the increasingly complex needs of customers.
Data relations
- View receives user interaction requests
- View forwards the request to ViewModel
- ViewModel Operational Model Data Update
- The Model updates the data and notifies the ViewModel that the data has changed
- ViewModel Updates View Data
mode
Two-way binding. Changes in View/Model are automatically reflected in ViewModel, and vice versa.
Use
- It is compatible with the MVC/MVP framework you are using today.
- Increase the testability of your application.
- It works best with a binding mechanism.
Advantages of MVVM
Like MVC mode, MVVM mode aims at separating View and Model. It has several advantages:
1. Low coupling. View can be independent of model changes and modifications. A View Model can be bound to different "Views". The Model can be unchanged when the View changes, and the View can be unchanged when the Model changes.
2. Reusability. You can put some view logic in a ViewModel and let many views reuse this view logic.
3. Independent development. Developers can focus on business logic and data development (ViewModel), designers can focus on page design, generate xml code.
4. may test . Interfaces have always been difficult to test, but now tests can be written for ViewModel.
Evolution of mvc, MVP and MVVM
Explain
Any project framework serves the project. There is no absolute difference between good and bad, only a more appropriate choice. At different stages of project progress, making the most appropriate adjustments is the more suitable framework for team project development. Project designers should bear in mind that any project design is based on the project development stage, the size of team members, and the overall ability of the team. Never design for design, but frame for framework. Quick and efficient cooperation with the whole team progress project is the most appropriate architecture. It is the only way for a programmer to become a leader and an architect.
Reproduced, please explain the source: http://blog.csdn.net/hudan2714/article/details/50990359
Reference: https://blog.nodejitsu.com/scaling-isomorphic-javascript-code/
MVC
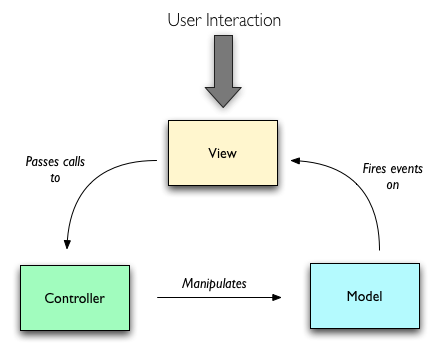
Response instructions are captured from the screen, View is transmitted to Controller. After Controller completes business logic, it requests the Model to change the state model to send new data to View, and the user gets feedback.
MVCViewController.m
#import "MVCViewController.h"
#import "MVCView.h"
#import "MVCModel.h"
@interface MVCViewController ()<MVCDelegate>
@end
@implementation MVCViewController
- (void)viewDidLoad {
[super viewDidLoad];
MVCView *view = [[MVCView alloc]initWithFrame:[UIScreen mainScreen].bounds];
view.delegate = self;
MVCModel *model = [MVCModel new];
model.name = @"name1";
[view setViewWithModel:model];
}
#pragma mark - delegate
//Agent returns view control of viewController
-(void)clickChange{
NSLog(@"chang from View!");
}
II. MVP
MVP mode changes Controller to Presenter, disconnects View from Model, and communicates through Presenter as a bridge.
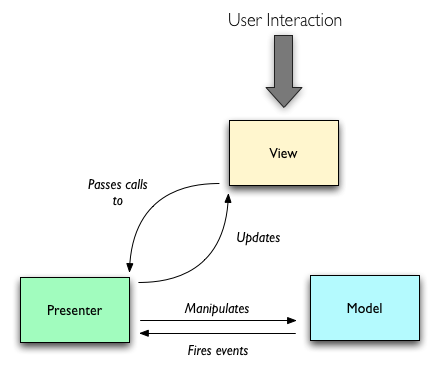
- Communication between different parts is bidirectional.
- View is not associated with Model, but is passed through Presenter.
- View is very thin and does not deploy any business logic, called Passive View, that is, there is no initiative, and Presenter is very thick, where all the logic is deployed.
- Presenter can also be understood as the butler of View and Model.
MVPViewController.m
#import "MVPViewController.h"
#import "MVPPresenter.h"
#import "MVPCell.h"
#import "MVPModel.h"
@implementation MVPViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
self.view.backgroundColor = [UIColor whiteColor];
MVPPresenter *present = [MVPPresenter new];
MVPCell *view = [MVPCell new];
MVPModel *model = [MVPModel new];
//get data
model.name = @"name1";
//View Layout
[self.view addSubview:view];
//Hand it over to presenter to avoid interaction between view and model
[present setPreModel:model];
[present setPreView:view];
}
MVPPresenter .h /.m
#import <Foundation/Foundation.h>
#import "MVPModel.h"
#import "MVPCell.h"
@interface MVPPresenter : NSObject
@property(nonatomic,strong)MVPCell *MVPView;
@property(nonatomic,strong)MVPModel *model;
-(void)setPreView:(MVPCell *)view;
-(void)setPreModel:(MVPModel *)model;
-(void)clickChangName;
#import "MVPPresenter.h"
@implementation MVPPresenter
- (instancetype)init
{
self = [super init];
if (self) {
}
return self;
}
-(void)setPreModel:(MVPModel *)model{
self.model = model;
}
-(void)setPreView:(MVPCell *)view{
self.MVPView = view;
[self.MVPView setlabel:_model.name];
}
-(void)clickChangName{
NSLog(@"name change %d",arc4random()%10);
}
MVVM
MVVM mode renames Presenter as ViewModel, which is basically identical to MVP mode.
The only difference is that it uses bi-directional binding: View <-> ViewModel. ViewModel is a worthwhile mapping in Model. When data changes, it notifies View of changes. In the future, it does not need to consider the interactive updates between View and Model. It only needs to start with the interface layout logic.
MVVMViewController.m
#import "MVVMViewController.h"
#import "MVVMView.h"
#import "MVVMModel.h"
#import "MVVMViewModel.h"
@interface MVVMViewController ()
@end
@implementation MVVMViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
MVVMView *MView = [MVVMView new];
MVVMModel *model = [MVVMModel new];
model.name = @"name1";
MVVMViewModel *viewModel = [MVVMViewModel new];
[self.view addSubview:MView];
//* viewModel acts as a hub to communicate the relationship between view and model
[viewModel setWithModel:model];
[MView setWithViewMoel:viewModel];
}
Key points:
- Name Str and Model in viewModel Middle name corresponds.
- The text value of label in View will be bound to nameStr (KVO key observation)
- When the value of the model changes, the View changes automatically.
- View and Model implements dynamic association through ViewModel
MVVMModel
#import <Foundation/Foundation.h>
@interface MVVMModel : NSObject
@property(nonatomic,copy)NSString *name;
@end
MVVMViewModel.h
#import "MVVMModel.h"
@interface MVVMViewModel : NSObject
//name in corresponding Model
@property(nonatomic,copy)NSString *nameStr;
@property(nonatomic,strong)MVVMModel *model;
-(void)setWithModel:(MVVMModel *)model;
-(void)clickChangeName;
MVVMView.m monitors changes in values using KVO
#import "MVVMView.h"
#import "NSObject+FBKVOController.h"
@interface MVVMView ()
@property(nonatomic,strong)MVVMViewModel *vm;
@property(nonatomic,strong)UILabel *label;
@property(nonatomic,strong)UIButton *button;
@end
@implementation MVVMView
- (instancetype)init
{
self = [super init];
if (self) {
self.backgroundColor = [UIColor whiteColor];
self.frame = [UIScreen mainScreen].bounds;
self.label = [[UILabel alloc]initWithFrame:CGRectMake(150,100 , 100, 30)];
self.label.backgroundColor = [UIColor orangeColor];
[self addSubview:_label];
self.button = [UIButton new];
_button.backgroundColor = [UIColor redColor];
[_button setTitle:@"click" forState:UIControlStateNormal];
[_button addTarget:self action:@selector(mvvmClickChangModel) forControlEvents:UIControlEventTouchUpInside];
_button.frame = CGRectMake(150, 200, 50, 50);
[self addSubview:_button];
}
return self;
}
-(void)setWithViewMoel:(MVVMViewModel *)vm{
self.vm = vm;
//KVO
[self.vm addObserver:self forKeyPath:@"nameStr" options:NSKeyValueObservingOptionOld|NSKeyValueObservingOptionNew context:nil];
self.label.text = vm.nameStr;
// //* FBKVO Third Party Library
// [self.KVOController observe:self.vm keyPath:@"nameStr" options:NSKeyValueObservingOptionInitial|NSKeyValueObservingOptionNew block:^(id _Nullable observer, id _Nonnull object, NSDictionary<NSString *,id> * _Nonnull change) {
// self.label.text = change[NSKeyValueChangeNewKey];
// }];
}
-(void)observeValueForKeyPath:(NSString *)keyPath ofObject:(id)object change: (NSDictionary<NSKeyValueChangeKey,id> *)change context:(void *)context{
if ([keyPath isEqualToString:@"nameStr"]&&[change objectForKey:NSKeyValueChangeNewKey]) {
NSNumber *new = [change objectForKey:NSKeyValueChangeNewKey];
self.label.text = [NSString stringWithFormat:@"%@",new];
}
}
-(void)mvvmClickChangModel{
[self.vm clickChangeName];
}
-(void)dealloc{
[self.vm removeObserver:self forKeyPath:@"nameStr"];
}
Example Demo
GitHub : https://github.com/one-tea/MVC-MVP-MVVM.git