This bad smell results when implementation changes are not encapsulated in abstractions and hierarchies.
The forms are usually as follows:
-
The client program is closely coupled with the service variants it needs. Whenever it needs to support new variants or modify existing variants, it will affect the client program.
-
Whenever new variants need to be supported in the hierarchy, a large number of unnecessary classes are added, which increases the complexity of the design.
The open close principle (OCP) points out that types should be open to extensions and closed to modifications. That is, the behavior of types should be changed by extension (not modification). OCP is violated when implementation changes are not encapsulated in a type or hierarchy.
Potential causes of missing packages
Don't realize that the focus will change
The possible changes of concerns were not predicted, and these concerns were not properly encapsulated in the design.
Mixed concerns
When the independent concerns are aggregated in a hierarchy instead of separated, if the concerns change, the number of classes may increase explosively.
Naive design decisions
Using too simple methods, such as creating a class for each combination of changes, can lead to unnecessary complexity in the design.
Suppose there is an entry class that needs to encrypt data using an encryption algorithm. There are many encryption algorithms to choose from, including des (data encryption standard), AES (Advanced Encryption Standard), TDES (Triple Data Encryption Standard), etc. The entry class uses DES algorithm to encrypt data.
public class Encryption { /// <summary> ///Encryption using DES algorithm /// </summary> public void Encrypt() { // Encryption using DES algorithm } }
Assuming that there are new requirements, AES algorithm is required to encrypt data.
The worst scenario emerged:
public class Encryption { /// <summary> ///Encryption using DES algorithm /// </summary> public void EncryptUsingDES() { // Encryption using DES algorithm } /// <summary> ///Encryption using AES algorithm /// </summary> public void EncryptUsingAES() { // Encryption using AES algorithm } }
There are many unsatisfactory aspects of this scheme:
-
The Encryption class becomes larger and more difficult to maintain because it implements multiple Encryption algorithms, but uses only one at a time.
-
It is difficult to add new algorithms and modify existing algorithms because Encryption algorithms are an integral part of Encryption class.
-
The Encryption algorithm provides services to the Encryption class, but it is tightly coupled with the Encryption class and cannot be reused elsewhere.
Refactoring if you are not satisfied. First, use inheritance for refactoring. There are two options:
Select 1:
Let the Encryption class inherit AESEncryptionAlgorithm or DESEncryptionAlgorithm class according to requirements, and provide the method Encrypt(). The problem caused by this scheme is that the Encryption class will be associated with a specific Encryption algorithm in the compilation stage. What is more serious is that the relationship between classes is not an is-a relationship.
/// <summary> ///AES algorithm encryption class /// </summary> public class AESEncryptionAlgorithm { /// <summary> ///Encryption using AES algorithm /// </summary> public void EncryptUsingAES() { // Encryption using AES algorithm } } /// <summary> ///DES algorithm encryption class /// </summary> public class DESEncryptionAlgorithm { /// <summary> ///Encryption using DES algorithm /// </summary> public void EncryptUsingDES() { // Encryption using DES algorithm } } public class Encryption: AESEncryptionAlgorithm { /// <summary> ///Encryption using algorithms /// </summary> public void Encrypt() { EncryptUsingAES(); } }
Option 2:
Create subclasses AESEncryption and DESEncryption, which both extend the Encryption class and include the implementation of Encryption algorithms AES and DES respectively. The client program can create references to Encryption that point to objects of a particular subclass. By adding new subclasses, it is easy to support new Encryption algorithms. However, the problem with this scheme is that AESEncryption and DESEncryption will inherit other methods of Encryption class, which reduces the reusability of Encryption algorithm.
public abstract class Encryption { /// <summary> ///Encryption using algorithms /// </summary> public abstract void Encrypt(); } /// <summary> ///AES algorithm encryption class /// </summary> public class AESEncryption : Encryption { /// <summary> ///Encryption using AES algorithm /// </summary> public override void Encrypt() { // Encryption using AES algorithm # Let's share my review interview materials > These interviews are all from the real interview questions and interview collection of large factories. Xiaobian has sorted them out for you( PDF (version) > > **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** * **Part I: Java Basics-intermediate-senior** 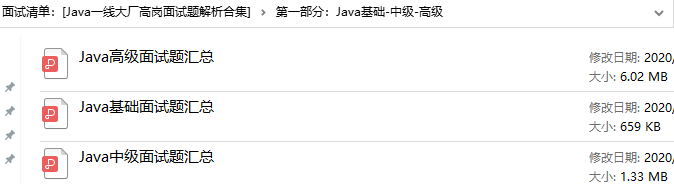 * **Part II: open source framework( SSM: Spring+SpringMVC+MyBatis)** 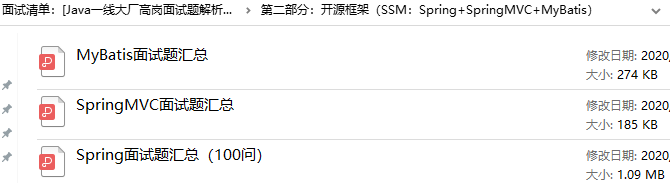 * **Part III: performance tuning( JVM+MySQL+Tomcat)** 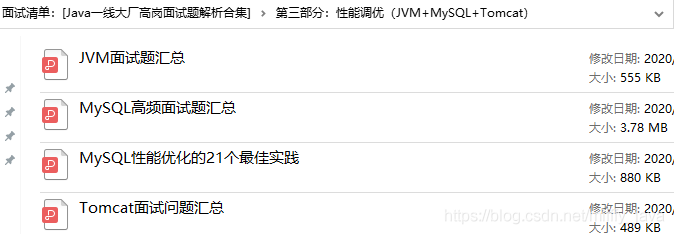 * **Part IV: distributed (current limiting): ZK+Nginx;Cache: Redis+MongoDB+Memcached;Communication: MQ+kafka)** 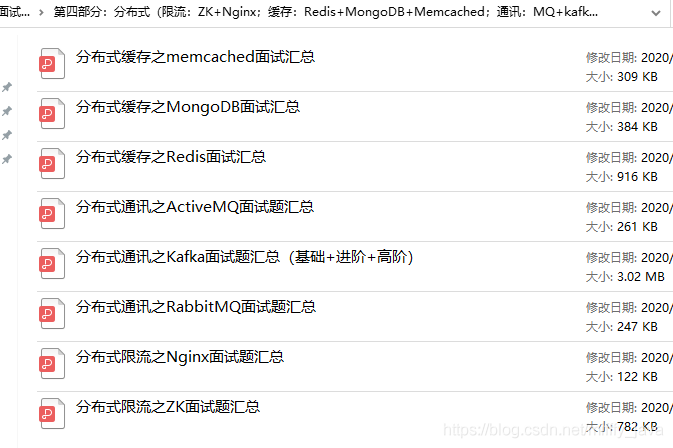 * **Part V: Micro services( SpringBoot+SpringCloud+Dubbo)** 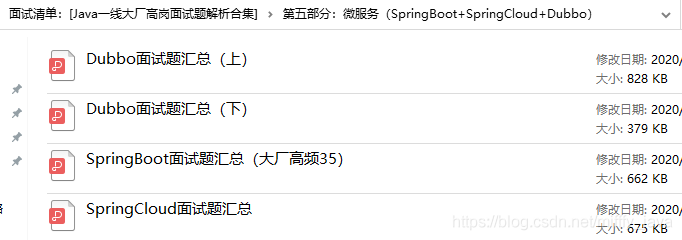 * **Part VI: others: concurrent programming+Design pattern+Data structure and algorithm+network** 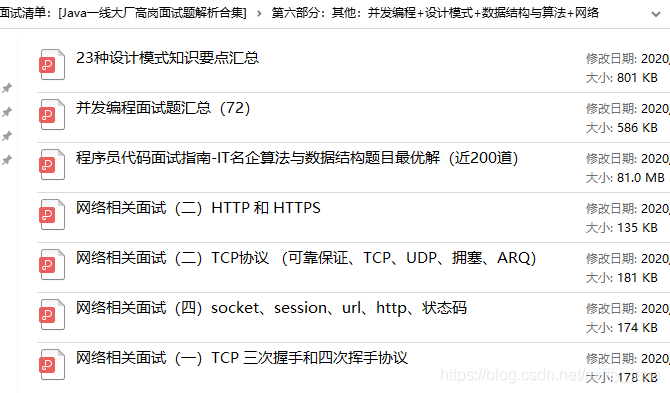 # Advanced learning notes pdf >* **Java Architecture foundation of advanced architecture(**Java Basics+Concurrent programming+JVM+MySQL+Tomcat+network+Data structure and algorithm**)** 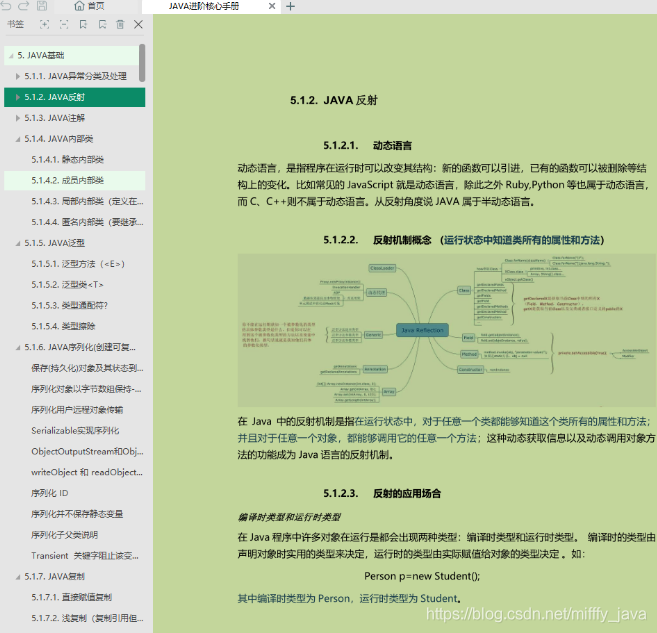 * **Java Advanced open source framework(**Design pattern+Spring+SpringMVC+MyBatis**)** 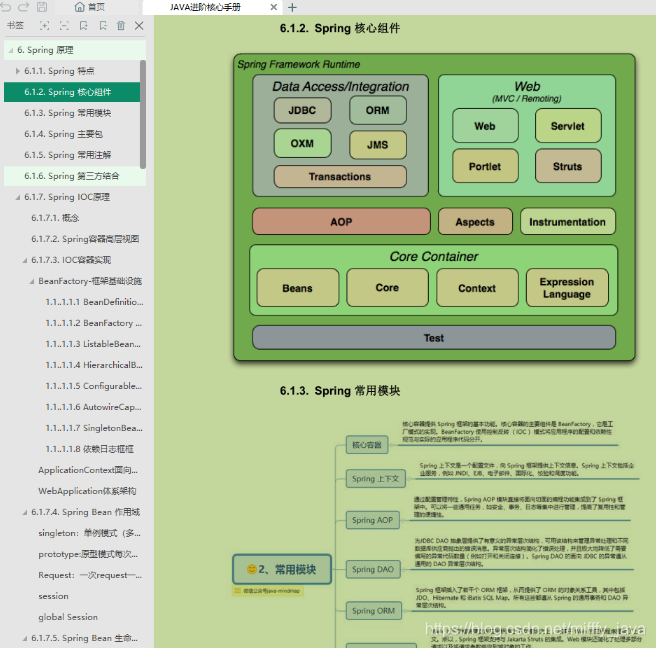 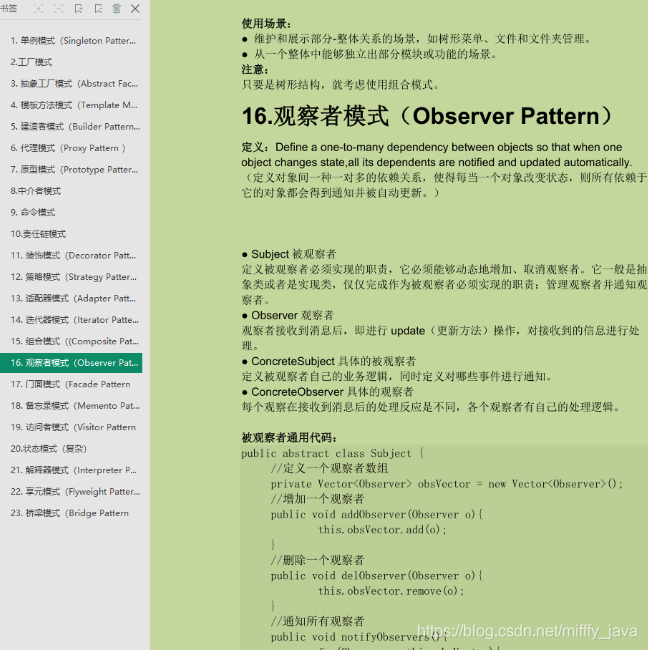 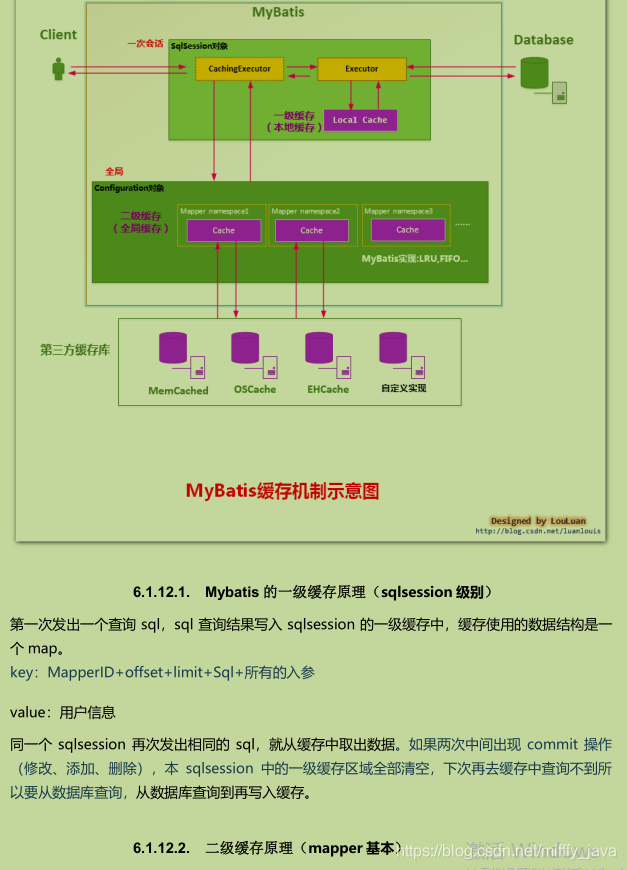 * **Java Advanced distributed architecture(**Current limiting( ZK/Nginx)+Cache( Redis/MongoDB/Memcached)+Communication( MQ/kafka)**)** 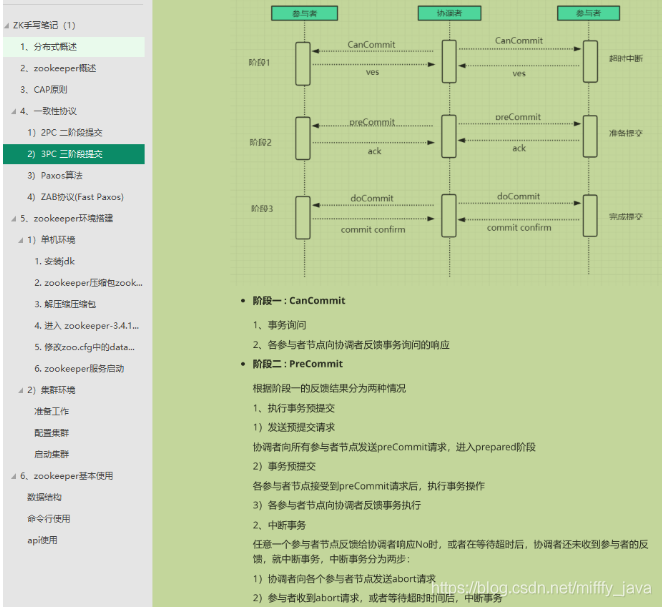 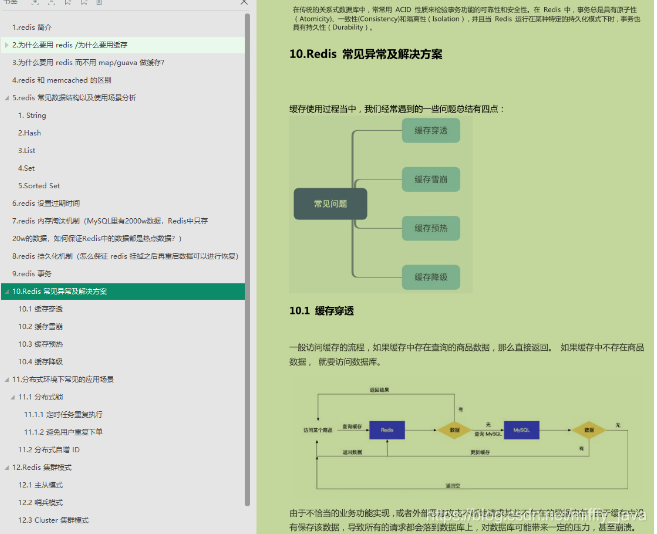 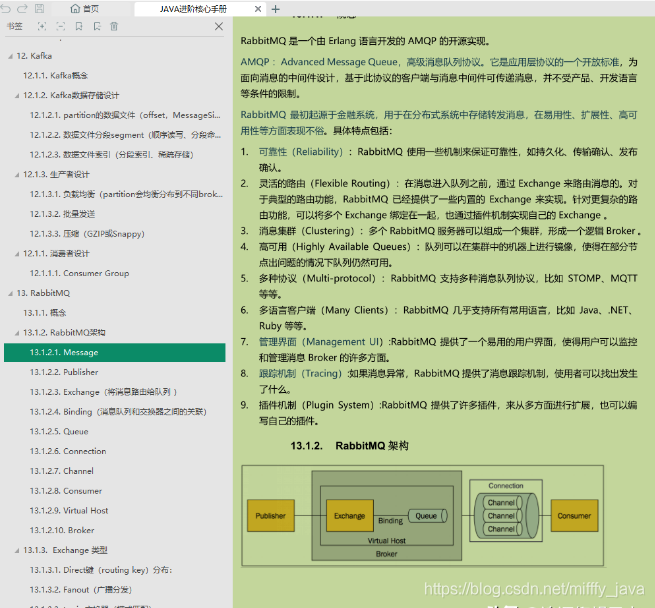 * **Java Advanced microservice architecture( RPC+SpringBoot+SpringCloud+Dubbo+K8s)** 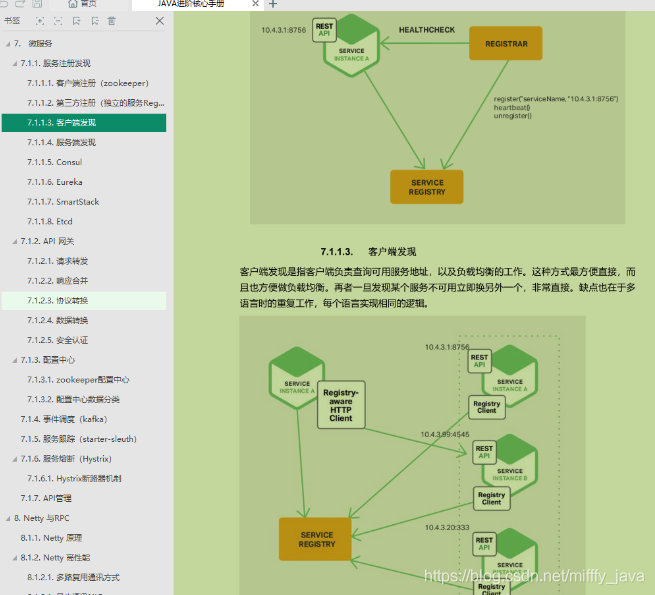 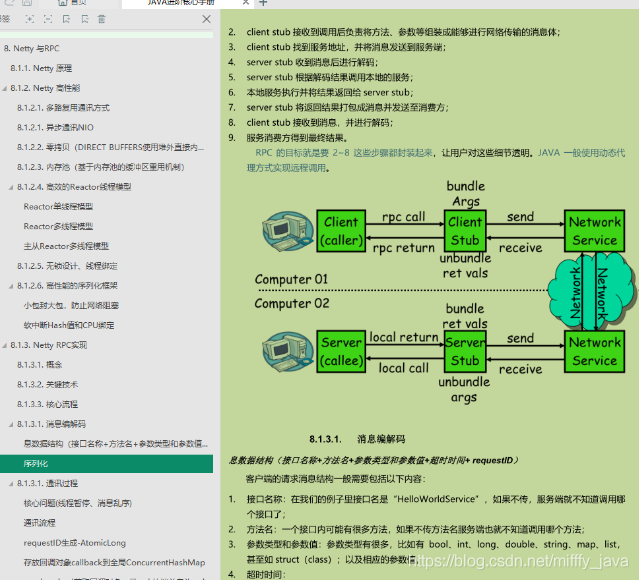 (ZK/Nginx)+Cache( Redis/MongoDB/Memcached)+Communication( MQ/kafka)**)** [External chain picture transfer...(img-esHd9sIq-1630855700243)] [External chain picture transfer...(img-8BL9EX8H-1630855700244)] [External chain picture transfer...(img-o5VGpoLo-1630855700245)] * **Java Advanced microservice architecture( RPC+SpringBoot+SpringCloud+Dubbo+K8s)** [External chain picture transfer...(img-PCvvz0r4-1630855700246)] [External chain picture transfer...(img-xxpA7ayC-1630855700247)]