Struts 2 Framework (8) - - Input Check of Struts 2
Keywords:
Java
xml
Struts
JSP
Input Check of Struts 2
In the actual development of our project, there are two kinds of data validation: front-end validation and server validation.
Client-side verification: js script is written mainly through jsp. Its advantage is obvious. It reminds the server in time if the input error is wrong, which can lighten the burden of the server. But client-side verification is not safe. Simply speaking, it prevents the gentleman from guarding against the villain.
Server-side validation: The most important feature is data security, but if only server-side validation, it will greatly increase the burden of server-side.
So in our development, it is the combination of client-side and server-side verification.
In that article, I'll just talk about server-side validation, which supports two types of validation in Struts2:
Code validation: Complete data validation on the server by editing java code
Configuration checking: xml configuration checking completes data checking through xml configuration file
(1) Code validation:
Code validation is divided into three steps:
Step 1. Encapsulation of data
Step 2. ActionSupport must be inherited to implement Actions to be checked
Step 3. Override the Validate method and complete the verification of business logic data
User interface register.jsp
1 <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
2 <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
3 <%@ taglib uri="/struts-tags" prefix="s" %>
4 <html>
5 <head>
6 <title>User registration</title>
7 </head>
8 <body style="text-align: center;">
9 <table align="center" width="50%">
10 <tr>
11 <td style="color: red">
12 <!-- <s:fielderror></s:fielderror> --> <!-- This is where the error is displayed. -->
13 </td>
14 </tr>
15 </table>
16
17 <form action="${pageContext.request.contextPath }/login" method="post" >
18
19 User name:<input type="text" name="username"><br><br>
20 dense Code:<input type="text" name="password"><br><br>
21 Confirm password:<input type="text" name="password2"><br><br>
22 <input type="reset" value="empty">
23 <input type="submit" value="register">
24
25 </form>
26 </body>
27 </html>
struts.xml
1 <?xml version="1.0" encoding="UTF-8" ?>
2 <!DOCTYPE struts PUBLIC
3 "-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
4 "http://struts.apache.org/dtds/struts-2.3.dtd">
5 <struts>
6 <!-- Configuration Constant Information -->
7 <constant name="struts.devMode" value="true"></constant>
8
9 <package name="struts2" extends="struts-default" >
10 <action name="login" class="com.study.Login.RegisterAction">
11 <result name="success">/success.jsp</result>
12 <!-- stay input Views can be accessed by<s:fielderror/>Display Failure Information -->
13 <result name="input">/register.jsp</result>
14 </action>
15 </package>
16 </struts>
RegisterAction.java
1 import com.opensymphony.xwork2.ActionSupport;
2 public class RegisterAction extends ActionSupport{
3
4 private String username;
5 private String password;
6 private String password2;
7 //Here I pass set Method encapsulation data
8 public void setUsername(String username) {
9 this.username = username;
10 }
11 public void setPassword(String password) {
12 this.password = password;
13 }
14 public void setPassword2(String password2) {
15 this.password2 = password2;
16 }
17
18 @Override
19 public String execute() throws Exception {
20 return NONE;
21 }
22
23 //Data validation needs to be completed on the server side
24 @Override
25 public void validate() {
26 //Test whether attribute values are obtained and verify that they have been obtained
27 System.out.println(username+"---"+password+"---"+password2);
28 if(username==null || username.length()<6 || username.length()>20){
29 //Save error information to fields
30 this.addFieldError("username", "Illegal input of household name");
31 }
32
33 if(password==null || password.length()<6 || password.length()>20){
34 //Save error information to fields
35 this.addFieldError("password", "Illegal password input");
36 }else if( password2==null || password2.length()<6 || password2.length()>20){
37 this.addFieldError("password2", "Illegal password input");
38 }else if(!password.equals(password2)){
39 this.addFieldError("password2", " Two password inconsistencies");
40 }
41
42 super.validate();
43 }
44 }
Operation results:
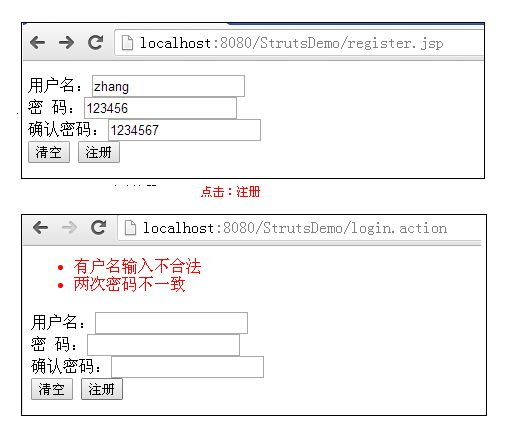
(2) Framework verification
Framework validation is data validation through XML configuration, which is also the mainstream validation in enterprise development.
Principle of XML Verification: Many rule codes have been written, just need to define the validation rules needed in the XML file. So it greatly reduces our development time.
Let me first write down the steps:
Step 1: Write JSP
Step 2: Write Action Inheritance ActionSupport or Validateable Interface
Step 3: Encapsulate request parameters
Step 4: Write xml validation rules file
Naming rules for Xml validation files: Action class name - validation.xml validates all methods in Action
Examples of naming rules RegisterAction-validation.xml
Also satisfy: Xml validation files and Action classes should be in the same package
Step 1: register.jsp

<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@ taglib uri="/struts-tags" prefix="s" %>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>User registration</title>
</head>
<body style="text-align: center;">
<table align="center" width="50%">
<tr>
<td style="color: red">
<s:fielderror></s:fielderror>
</td>
</tr>
</table>
<form action="${pageContext.request.contextPath }/login.action" method="post" >
<table width="60%" >
<tr>
<td>User name</td>
<td>
<input type="text" name="username" >
</td>
</tr>
<tr>
<td>Password</td>
<td>
<input type="password" name="password" >
</td>
</tr>
<tr>
<td>Confirm password</td>
<td>
<input type="password" name="password2" >
</td>
</tr>
<tr>
<td>Age</td>
<td>
<input type="text" name="age" >
</td>
</tr>
<tr>
<td>mailbox</td>
<td>
<input type="text" name="email" >
</td>
</tr>
<tr>
<td>Birthday</td>
<td>
<input type="text" name="birthday" >
</td>
</tr>
<tr>
<td>Graduation date</td>
<td>
<input type="text" name="graduation" >
</td>
</tr>
<tr>
<td>
<input type="reset" value="empty">
</td>
<td>
<input type="submit" value="register">
</td>
</tr>
</table>
</form>
</body>
</html>
register.jsp
Step 2: RegisterAction.java
import com.opensymphony.xwork2.ActionSupport;
import com.opensymphony.xwork2.ModelDriven;
public class RegisterAction extends ActionSupport implements ModelDriven<User>{
//Manually create an object
private User user =new User();
@Override
public String execute() throws Exception {
return NONE;
}
public User getModel() {
return user;
}
}
Step 3: Encapsulate request parameters
In the case of manual validation above, I encapsulated the data with set method. Here I encapsulated the request data with ModelDriven interface.
I've talked before about three ways of encapsulating data that are not clear. Look at this article: Struts Framework (6) - - Action Receives Request Parameters
User.java
1 import java.util.Date;
2 public class User {
3
4 private String username;
5 private String password;
6 private String password2;
7 private Integer age;
8 private String email;
9 private Date birthday;
10 private Date graduation;
11
12 /*
13 * Provide get and set methods for attributes
14 */
15 }
Step 4: RegisterAction-validation.xml
<!DOCTYPE validators PUBLIC
"-//Apache Struts//XWork Validator 1.0.2//EN"
"http://struts.apache.org/dtds/xwork-validator-1.0.2.dtd">
<validators>
<!--dtd Constraint in xwork-core-**.jar In bag -->
<field name="username">
<field-validator type="requiredstring">
<param name="trim">true</param>
<message>Users can't be empty</message>
</field-validator>
<field-validator type="stringlength">
<param name="minLength">6</param>
<param name="maxLength">15</param>
<message>The length of a household name must be ${minLength} and ${maxLength}Between </message>
</field-validator>
</field>
<field name="password">
<field-validator type="requiredstring">
<param name="trim">true</param>
<message>Password cannot be empty</message>
</field-validator>
<field-validator type="stringlength">
<param name="minLength">6</param>
<param name="maxLength">15</param>
<message>The password must be ${minLength}and ${maxLength}Between </message>
</field-validator>
</field>
<field name="password2">
<field-validator type="fieldexpression">
<param name="expression"><![CDATA[password==password2]]></param>
<message>Two password inconsistencies</message>
</field-validator>
</field>
<field name="age">
<field-validator type="required">
<param name="trim">true</param>
<message>Age cannot be empty</message>
</field-validator>
<field-validator type="int">
<param name="min">1</param>
<param name="max">150</param>
<message>Age must be ${min} and ${max}Between</message>
</field-validator>
</field>
<field name="email">
<field-validator type="email">
<message>Not a legitimate mailbox address</message>
</field-validator>
</field>
<field name="birthday">
<field-validator type="date">
<param name="min">2001-01-01</param>
<param name="max">2003-12-31</param>
<message>Birthday must be ${min} and ${max}Between</message>
</field-validator>
</field>
</validators>
struts.xml doesn't need any changes, just like before.
Operation results:
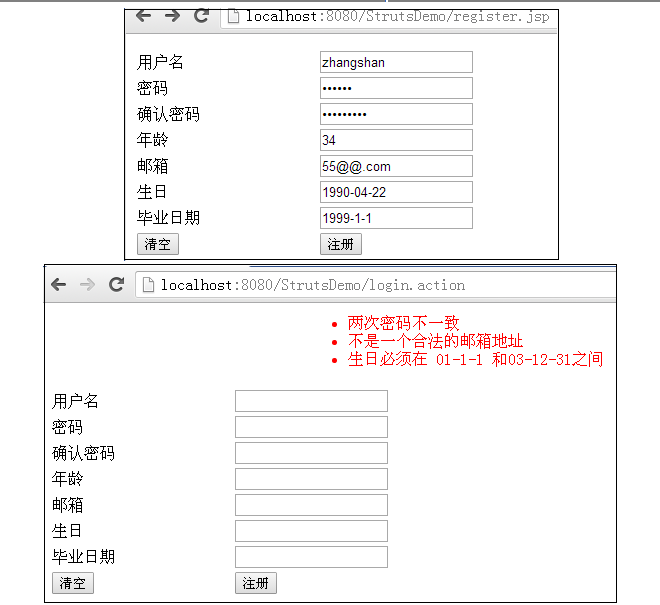
If you think the location of the error is a bit ugly, you can use Struts 2's < s: form > tag, and the effect will be better.
Now that we've talked about both ways, we'll summarize some of the key points of xml configuration.
Explanation of xml validation file:
< validators >: root element
<field>: Specify the properties to be validated in action, and the name attribute specifies the name of the form field to be validated
<field-validator>: Specify a validator, and type specifies validation rules
The requiredstring checker specified above is provided by the system, which can meet most of the verification requirements.
Checkers, whose definitions can be found in xwork-2.x.jar
Find it in default.xml under com.opensymphony.xwork2.validator.validators.
<param>: Subelements can pass parameters to validators
<message>: The child element is the message after the validation failure. If internationalization is required, it can be message.
Specify the key attribute whose value is the key in the property file.
Strts2 Check Rules:
The validators provided by the system are as follows:
required (mandatory validator requiring that the validated attribute value should not be null)
Required string
String length (string length checker requires that the property value to be checked must be within the specified range, otherwise the checking fails, minLength parameter specifies the minimum length, maxLength parameter specifies the maximum length, and trim parameter specifies whether to remove the blanks before and after the string is checked).
Regex (Regular Expression Checker, Check whether the checked attribute value matches a regular expression, the expression parameter specifies a regular expression, and the caseSensitive parameter specifies whether case-sensitive, default value is true) is used for regular expression matching.
Int (integer checker, requiring the integer value of field to be within the specified range, min to specify the minimum, max to specify the maximum)
Double (double-precision floating-point verifier, requires field's double-precision floating-point number must be within the specified range, min specifies the minimum value, max specifies the maximum value)
Field expression (field OGNL expression checker, requires field to satisfy an ognl expression, expression parameter specifies the ognl expression, the logical expression is evaluated based on ValueStack, when returning true, the verification passes, otherwise it does not pass)
Email (Mail Address Checker, requires that if the validated attribute value is not empty, it must be a legitimate mail address)
URL (url validator, requires that if the value of the property being validated is not empty, it must be a legitimate URL address)
Date (date checker, which requires the date value of field to be within the specified range, min to specify the minimum, max to specify the maximum)
Conversion (Conversion Checker, which specifies the error message prompted when the type conversion fails)
Visitor (for validating properties of composite types in action, which specifies a validation file for validating properties of composite types)
expression(OGNL expression checker, which is a non-field checker, the expression parameter specifies the ognl expression. The logical expression is evaluated based on ValueStack and checked when it returns true. Otherwise, if it does not pass, the checker cannot be used in the field checker style configuration)
Finally, I will give you one detail:
Help information cannot appear when writing validation files
When writing the ActionClassName-validation.xml validation file, if there is no help information, you can solve it in the following way:
windwos->preferences->myeclipse->files and editors->xml->xmlcatalog
Point "add" and select "File system" in the location of the window that appears. Then select xwork-validator-1.0.3.dtd in the src Java directory of the xwork-2.1.2 unzipped directory.
When you go back to setting the window, don't rush to close the window. Change the Key Type in the window to URI. Change Key to http://struts.apache.org/dtds/xwork-validator-1.0.2.dtd
So far as this article is concerned, there are some shortcomings. Welcome to leave a message and give some advice. Thank you.