1, Download sdk -- 2dmap? Demo
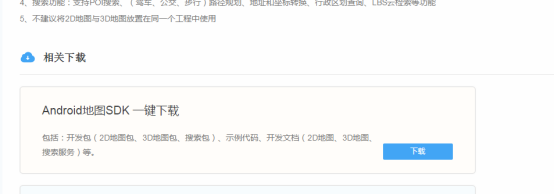
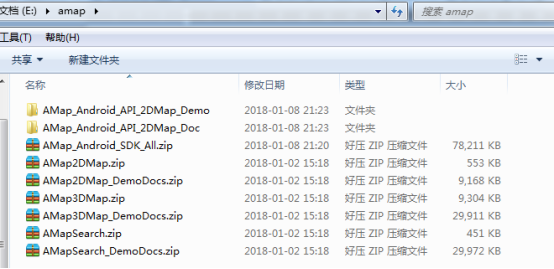
2, Get appkey (first get the shaw1 value of keystore)
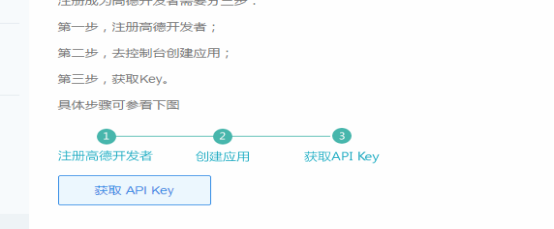
(1) , find debug.keystore in the directory C:\Users\Administrator.android,
(2) next, enter the cmd command in the address bar of the directory
Absolute path of Keytool -v -list -keystore debug.keystore
(3. Enter the password (the password is android)
(4) copy the shaw1 value
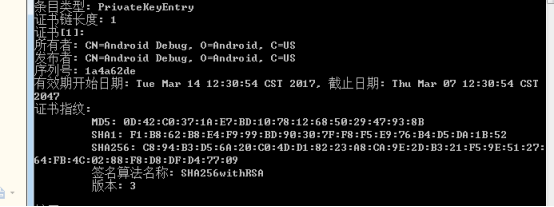
3, Import the jar package and add it to the dependency Library (copy the jar of the 2D map demo to the project)
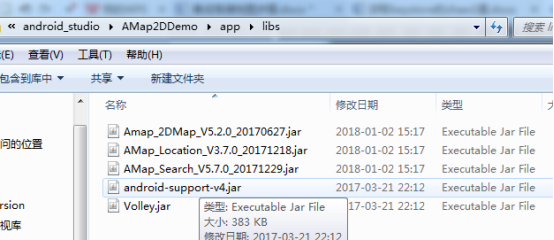
4, Copy Demo's manifest file
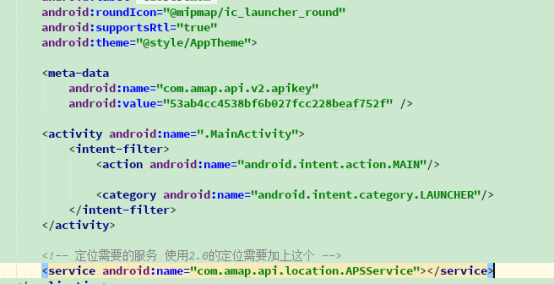
5, copy the Demo code of Goldberg sdk
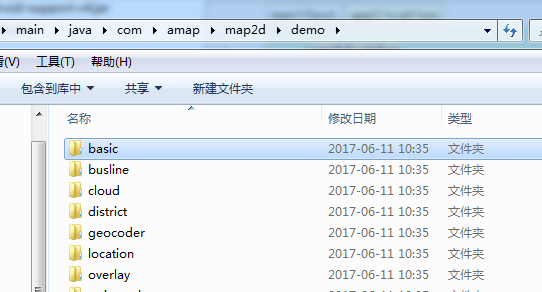
6, Code of map page:
public class SecondLocationActivity extends AppCompatActivityimplements LocationSource, AMapLocationListener, PoiSearch.OnPoiSearchListener, View.OnClickListener {private AMap aMap; private OnLocationChangedListener mListener; private AMapLocationClient mlocationClient; private AMapLocationClientOption mLocationOption; private int currentPage; private String keyWord = ""; private String city = "Shenzhen City"; private PoiSearch.Query query; private PoiSearch poiSearch; private LatLng lp; private PoiResult poiResult; private ArrayList<PoiItem> poiItems; private AroundRvAdapter mAroundRvAdapter; private ActivitySecondLocationBinding mBinding; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); mBinding = DataBindingUtil.setContentView(this, R.layout.activity_second_location); mBinding.mapView.onCreate(savedInstanceState); mBinding.recycleView.setLayoutManager(new LinearLayoutManager(this));private void intiUI() {
if (aMap == null) { aMap = mBinding.mapView.getMap(); setUpMap(); } } /** * Set some properties of amap */ private void setUpMap() { // Customized system positioning small blue dot MyLocationStyle myLocationStyle = new MyLocationStyle(); myLocationStyle.myLocationIcon(BitmapDescriptorFactory .fromResource(R.drawable.location_marker));// Set small blue dot Icon myLocationStyle.strokeColor(Color.BLACK);// Set the border color of the circle myLocationStyle.radiusFillColor(Color.argb(100, 0, 0, 180));// Set the fill color for circles // myLocationStyle.anchor(int,int) / / set the anchor point of the small blue dot myLocationStyle.strokeWidth(1.0f);// Set the border thickness of the circle aMap.setMyLocationStyle(myLocationStyle); aMap.setLocationSource(this);// Set location monitoring aMap.getUiSettings().setMyLocationButtonEnabled(true);// Set whether the default positioning button is displayed aMap.setMyLocationEnabled(true);// Set to true to show location layer and trigger location, false to hide location layer and not trigger location, default is false // aMap.setMyLocationType() } /** * Methods must be rewritten and associated with life cycle to reduce consumption and improve performance */ @Override protected void onResume() { super.onResume(); mBinding.mapView.onResume(); } /** * Methods must be rewritten and associated with life cycle to reduce consumption and improve performance */ @Override protected void onPause() { super.onPause(); mBinding.mapView.onPause(); } /** * Methods must be rewritten and associated with life cycle to reduce consumption and improve performance */ @Override protected void onSaveInstanceState(Bundle outState) { super.onSaveInstanceState(outState); mBinding.mapView.onSaveInstanceState(outState); } /** * Methods must be rewritten and associated with life cycle to reduce consumption and improve performance */ @Override protected void onDestroy() { super.onDestroy(); mBinding.mapView.onDestroy(); } /** * Activate positioning. Positioning can only be performed after activation */ @Override public void activate(OnLocationChangedListener listener) { mListener = listener; if (mlocationClient == null) { mlocationClient = new AMapLocationClient(this); mLocationOption = new AMapLocationClientOption(); //Set location monitoring mlocationClient.setLocationListener(this); //Set to high precision positioning mode mLocationOption.setLocationMode(AMapLocationClientOption.AMapLocationMode.Hight_Accuracy); //Set positioning parameters mlocationClient.setLocationOption(mLocationOption); // In order to reduce power consumption or network traffic consumption, a location request is sent every fixed time, // Pay attention to setting the interval of the appropriate location time (the minimum interval is 2000 ms), and call stopLocation() method to cancel the location request at the appropriate time // After positioning, call the onDestroy() method in the appropriate lifecycle // In the case of a single location, no matter whether the location is successful or not, there is no need to call the stopLocation() method to remove the request, and the internal location sdk will be removed mlocationClient.startLocation(); } } /** * Stop positioning */ @Override public void deactivate() { mListener = null; if (mlocationClient != null) { mlocationClient.stopLocation(); mlocationClient.onDestroy(); } mlocationClient = null; } /** * Callback function after successful positioning */ @Override public void onLocationChanged(AMapLocation amapLocation) { if (mListener != null && amapLocation != null) { if (amapLocation != null && amapLocation.getErrorCode() == 0) { // 1. Small blue dot of display system mListener.onLocationChanged(amapLocation); lp = new LatLng(amapLocation.getLatitude(), amapLocation.getLongitude()); // aMap.moveCamera(CameraUpdateFactory.changeLatLng(new LatLng(amapLocation.getLatitude(),amapLocation.getLongitude()))); aMap.moveCamera(CameraUpdateFactory.changeLatLng(lp)); aMap.moveCamera(CameraUpdateFactory.zoomTo(19)); //Range 3-20 Log.e("date", "search result:" + amapLocation.getAddress()); //2. Only locate once, terminate (to improve performance) if (mlocationClient != null) { mlocationClient.stopLocation(); mlocationClient.onDestroy(); } //3. Try to search the surrounding area and all poi points within 500m doSearchQuery(); } else { String errText = "seek failed," + amapLocation.getErrorCode() + ": " + amapLocation.getErrorInfo(); Log.e("AmapErr", errText); } } } protected void doSearchQuery() { currentPage = 0; // The first parameter represents the search string, the second parameter represents the poi search type, and the third parameter represents the poi search area (the empty string represents the whole country) query = new PoiSearch.Query(keyWord, "", city); query.setPageSize(50);// Set the maximum number of poiitem s returned per page query.setPageNum(currentPage);// See page 1 for settings if (lp != null) { poiSearch = new PoiSearch(this, query); poiSearch.setOnPoiSearchListener(this); poiSearch.setBound(new PoiSearch.SearchBound(new LatLonPoint(lp.latitude, lp.longitude), 5000, true));//Within 200m // Set the search area to take lp point as the center, and the range around it is 5000 meters poiSearch.searchPOIAsyn();// Asynchronous search } } @Override public void onPoiSearched(PoiResult result, int rcode) { if (rcode == 1000) { if (result != null && result.getQuery() != null) {// Search for poi results if (result.getQuery().equals(query)) {// Is it the same article poiResult = result; poiItems = poiResult.getPois();// Get the poiitem data of the first page, starting with the number 0 Toast.makeText(this, "There are" + poiItems.size() + "Results", Toast.LENGTH_SHORT).show(); mAroundRvAdapter.setPoiItemList(poiItems); } } } } @Override public void onPoiItemSearched(PoiItem poiItem, int i) { } @Override public void onClick(View view) { switch (view.getId()) { case R.id.rl_exit: finish(); if(Integer.valueOf(android.os.Build.VERSION.SDK) >= 5) { overridePendingTransition(0,0); //2 zeros indicate that the activity switch does not need animation effect } break; } }
}
7, Adapter
public class AroundRvAdapter extends RecyclerView.Adapter {
private Context mContext;
public AroundRvAdapter(Context context) {
mContext = context;
}
private List<PoiItem> mPoiItemList = new ArrayList<>();
public void setPoiItemList(List<PoiItem> poiItemList) {
mPoiItemList = poiItemList;
notifyDataSetChanged();
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(mContext).inflate(R.layout.item_around, parent, false);
ViewHolder viewHolder = new ViewHolder(itemView);
return viewHolder;
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
ViewHolder viewHolder = (ViewHolder) holder;
viewHolder.setData(mPoiItemList.get(position));
}
@Override
public int getItemCount() {
if(mPoiItemList!=null){
return mPoiItemList.size();
}
return 0;
}
class ViewHolder extends RecyclerView.ViewHolder{
TextView mTitle;
TextView mAddress;
ViewHolder(View view) {
super(view);
mTitle = view.findViewById(R.id.title);
mAddress = view.findViewById(R.id.address);
}
public void setData(PoiItem poiItem) {
mTitle.setText(poiItem.getTitle());
mAddress.setText(poiItem.getSnippet()); //Summary information
}
}
}
8, activity layout
<layout><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
android:orientation="vertical">
<com.amap.api.maps2d.MapView
android:id="@+id/map_view"
android:layout_width="match_parent"
android:layout_height="200dp">
</com.amap.api.maps2d.MapView>
<android.support.v7.widget.RecyclerView
android:id="@+id/recycle_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1">
</android.support.v7.widget.RecyclerView>
</LinearLayout> </layout>
9, Adapter layout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:padding="5dp"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:padding="5dp"
android:id="@+id/title"
android:text="The height of the sun"
android:textSize="20sp"
android:textColor="#000000"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:padding="5dp"
android:id="@+id/address"
android:textSize="16sp"
android:textColor="#666666"
android:text="Shennan Dadao"
android:layout_width="match_parent"
android:layout_height="wrap_content"/> </LinearLayout>