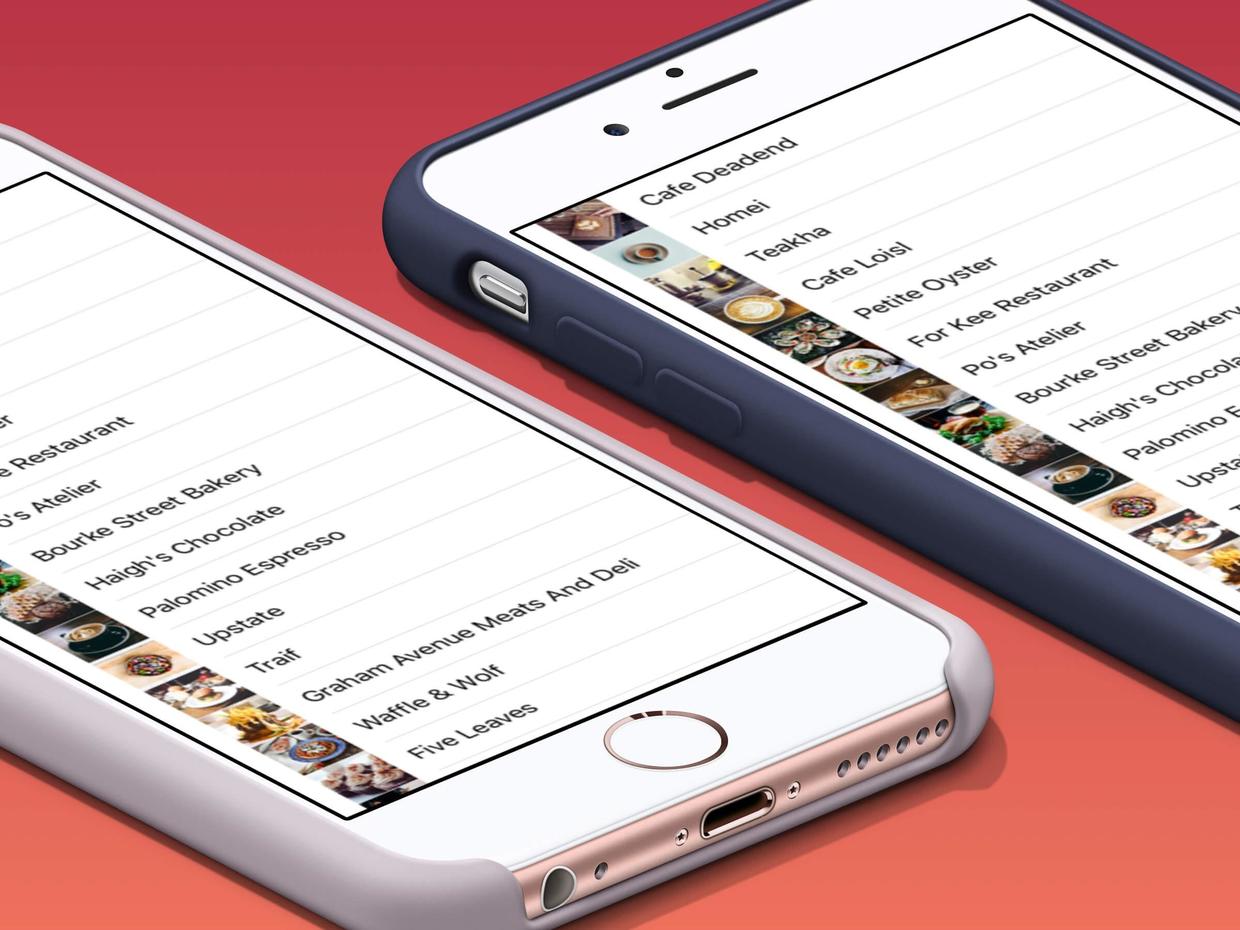
table view should be the most commonly used UI element in iOS applications. The best examples are some of the applications that come with the iPhone, such as phones, emails, settings, etc. TED, Google+, Airbnb, Wechat and so on are good examples.
Create a project
- The project name is SimpleTable and the template is "Single View application"
Design UI
- Select Main.storyboard and drag Table View from Object library to view
- Change the size of Table View to the entire view, and modify the property Prototype Cells to 1
- Select Table View Cell, modify Style to Basic and Identifier to Cell. The standard types of table view cell are basic, right detail, left detail and subtitle, as well as custom types.
- Select Table View, set four spacing constraints, and set the distance from top to bottom to 0
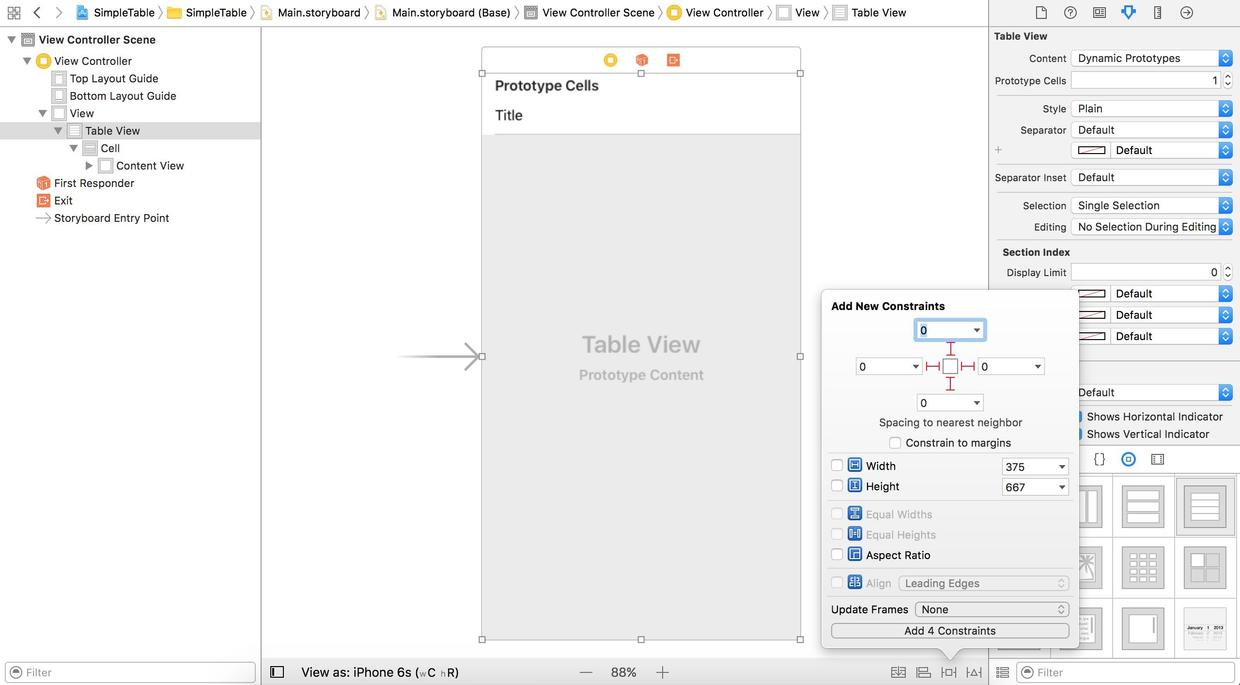
Adding two protocols to UITableView
- Each UI component in Object library corresponds to a class, such as Table View, which corresponds to UITableView. You can click and hover over the UI component to see the corresponding classes and descriptions.
- After the UIViewController of ViewController.swift file, add code, UITableViewDataSource, UITableViewDelegate, indicating that the ViewController class implements two protocols: UITableViewDataSource and UITableViewDelegate.
A red exclamation mark appears. This is the question prompt of xcode. Click on the question description.Type 'ViewController' does not conform to protocol
'UITableViewDataSource'
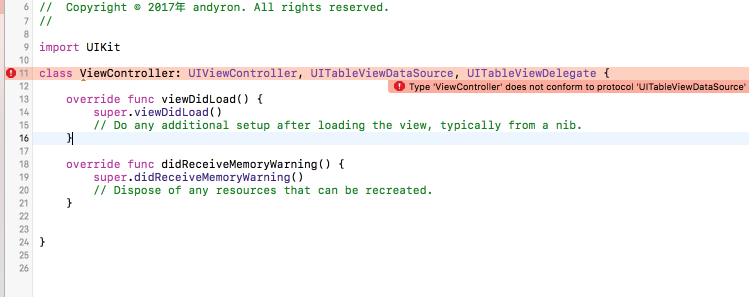
The problem is described as ViewController does not conform to the protocol UITableViewDataSource. See you in the UITableViewDataSource by command + click (the latest Xcode 9 becomes command+option + click):
public protocol UITableViewDataSource : NSObjectProtocol { @available(iOS 2.0, *) public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int // Row display. Implementers should *always* try to reuse cells by setting each cell's reuseIdentifier and querying for available reusable cells with dequeueReusableCellWithIdentifier: // Cell gets various attributes set automatically based on table (separators) and data source (accessory views, editing controls) @available(iOS 2.0, *) public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell @available(iOS 2.0, *) optional public func numberOfSections(in tableView: UITableView) -> Int // Default is 1 if not implemented @available(iOS 2.0, *) optional public func tableView(_ tableView: UITableView, titleForHeaderInSection section: Int) -> String? // fixed font style. use custom view (UILabel) if you want something different @available(iOS 2.0, *) optional public func tableView(_ tableView: UITableView, titleForFooterInSection section: Int) -> String? // Editing // Individual rows can opt out of having the -editing property set for them. If not implemented, all rows are assumed to be editable. @available(iOS 2.0, *) optional public func tableView(_ tableView: UITableView, canEditRowAt indexPath: IndexPath) -> Bool ...
-
There are many methods defined in UITableViewDataSource protocol. Except for the first two methods, there are no optional other methods. Some of them indicate that this method is not necessarily implemented, and none of them must be implemented. If these two methods are implemented, the problem prompt will disappear. These two methods also probably know what to do from the name and return value type:
- Public func tableView (tableView: UITableView, numberOfRowsInSection section: Int) - > Int has several rows of a section, that is, a section has several UITableViewCells, which means a set of UITableViewCells. Table View can define multiple sections, default to one.
- Public func tableView (tableView: UITableView, CellForRow At IndexPath: IndexPath) - > UITableViewCell returns UITableViewCell for each row
-
In ViewController.swift, we define a variable restaurantNames, which is an array of types and represents the names of a series of restaurants.
var restaurantNames = ["Cafe Deadend", "Homei", "Teakha", "Cafe Loisl", "PetiteOyster", "For Kee Restaurant", "Po's Atelier", "Bourke Street Bakery", "Haigh'sChocolate", "Palomino Espresso", "Upstate", "Traif", "Graham Avenue Meats AndDeli", "Waffle & Wolf", "Five Leaves", "Cafe Lore", "Confessional","Barrafina", "Donostia", "Royal Oak", "CASK Pub and Kitchen"]
-
Two methods for defining UITableViewDataSource are:
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { // 1 return restaurantNames.count } func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { // 2 let cellIdentifier = "Cell" let cell = tableView.dequeueReusableCell(withIdentifier: cellIdentifier, for: indexPath) // 3 cell.textLabel?.text = restaurantNames[indexPath.row] return cell }
- 1 The number of restaurants is the number of rows of section.
- 2 "Cell" corresponds to the Identifier attribute of UITableViewCell defined earlier. The dequeueReusableCell method generates a UITableViewCell.
- 3 UITableViewCell has a countable attribute textLabel, which is actually a UILabel. Because it is an optional attribute, cell. textLabel?.
Connect DataSource and Delegate
Run app and no data is displayed. Although the above code has allowed ViewController to inherit UITableViewDataSource, UITableViewDelegate, storyboard does not know.
- Select Table View in document outline, use control-drag to View Controller, and select dataSource in the pop-up box. The same way to choose delegate
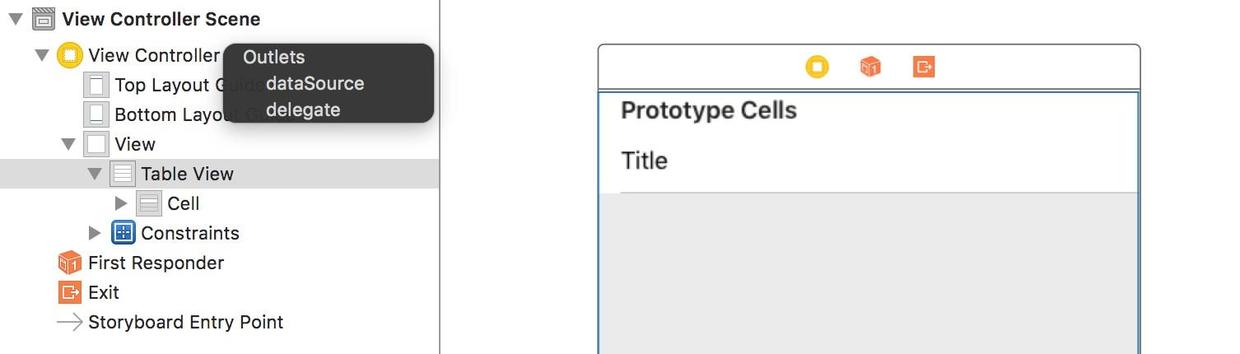
- Verify that the connection was successful. Select Table View to view in the connetion Checker; or right-click Table View directly in the document outline
- Run app
Add pictures to Table View
- from simpletable-image1.zip Download the picture and drag it to asset catalog after decompression
- Add the code cell.imageView?.image = UIImage(named: "restaurant") before the return cell of the tableView (: cell ForRowAtIndexPath:) method of ViewController.swift. Make every UITableViewCell image the same.
hide the status bar
The top state overlaps the table view data by adding a code to the ViewController:
override var prefersStatusBarHidden: Bool { return true }
PreersStatusBarHidden is an attribute in the parent UIViewController, so override is added to indicate override.
Different Cell s correspond to different pictures
- from simpletable-image2.zip Download the picture and drag it to asset catalog after decompression
-
Modify the tableView (: CellForRowAtIndexPath:) of ViewController.swift to:
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { let cellIdentifier = "Cell" let cell = tableView.dequeueReusableCell(withIdentifier: cellIdentifier, for: indexPath) // Configure the cell... let restaurantName = restaurantNames[indexPath.row] cell.textLabel?.text = restaurantName // 1 let imageName = restaurantName.lowercased().replacingOccurrences(of: " ", with: "") if let image = UIImage(named: imageName) { cell.imageView?.image = image } else { cell.imageView?.image = UIImage(named: "restaurant") } return cell }
- 1. Modify the name string of the restaurant into lowercase and then go to the space.
-
final result

Code
Beginning-iOS-Programming-with-Swift
Explain
This article is about learning. appcode A book published on the website <Beginning iOS 10 Programming with Swift> A Record