1, Tool preparation
1.maven Version maven-3.8.2
2.tomcat Version tomcat-8.5.65
3. Database Version 8.0.24
4. The database source is c3p0 Version 0.9.5.2
2, Database construction statement
Note: the following sql statements can be executed sequentially in the dolphin.
CREATE DATABASE ssmbuild; USE ssmbuild; CREATE TABLE books( bookId INT(10) PRIMARY KEY NOT NULL AUTO_INCREMENT COMMENT 'book ID', bookName VARCHAR(100) NOT NULL COMMENT 'title', bookCount INT(11) NOT NULL COMMENT 'quantity', detail VARCHAR(100) NOT NULL COMMENT 'describe' )ENGINE=INNODB DEFAULT CHARSET = utf8 INSERT INTO books (bookName,bookCount,detail) VALUES ("JAVA",1,"From getting started to giving up"), ("Mysql",10,"From deleting the library to running away"), ("Linux",5,"From entry to prison");
3, Create project
The maven project is basically created successfully. The following is the package introduction, because we created the maven project through template. The constraints in webapp -- > WEB-INF -- > web.xml are not very good, and version 4.0 needs to be adopted. Therefore, the following operations need to be carried out (clean up the unused parts in pom):
pom file:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>****</artifactId> <!--Own project name--> <version>1.0-SNAPSHOT</version> <packaging>war</packaging> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <!--junit test--> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <!--Database connection driver--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.24</version> </dependency> <!--c3p0 Database source--> <dependency> <groupId>com.mchange</groupId> <artifactId>c3p0</artifactId> <version>0.9.5.2</version> </dependency> <!--servlet+jsp--> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <!--mybatis--> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.2</version> </dependency> <!--mybatis integration spring--> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>2.0.2</version> </dependency> <!--spring frame--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.1.9.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>5.1.9.RELEASE</version> </dependency> <!--annotation--> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.12</version> </dependency> </dependencies> </project>
The next step is to modify the constraint of webapp -- > WEB-INF -- > web.xml
If it is the address of the following path, directly select ok in the above dialog box, and then save (apply).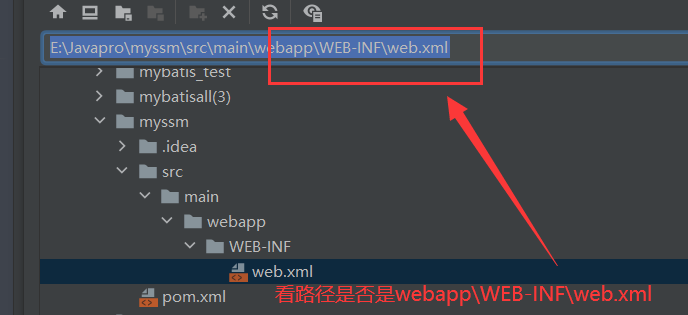
Click web.xml and the following screen will appear, indicating that we have replaced webxml (that is, the above operation is completely correct)
maven's framework is roughly as shown in the following figure (if java and resource under main are missing, you can right-click main and select new -- > directory to create it):
The following maven framework shows that the maven project has been built successfully.
4, xml file configuration
First of all, we need to find out what the ssm framework has and what it is used for. Let me briefly talk about its functions:
Spring: it is the adhesive of mybatis and spring MVC, and it is the main code area of the business layer
Spring MVC: mainly view control, which is the controller in java code
mybatis: it operates on the persistence layer (dao layer / database).
After understanding the above functions, we will know how to cooperate with the general idea of xml.
(1) web.xml file configuration
There are two main things to do. The first thing is to set the character encoding, and the second thing is to set the CPU (and you need to specify the core file < paramvalue > classpath: ApplicationContext. XML < / param value >) that must be loaded when the project is started)
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0"> <!--encodingFilter--> <filter> <filter-name>encodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>utf-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>encodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!--DispatcherServlet--> <servlet> <servlet-name>dispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:applicationContext.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>dispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
(2) spring-dao.xml configuration file
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"> <!--Import external profile--> <context:property-placeholder location="classpath:database.properties"/> <!--scanning service of bean--> <context:component-scan base-package="com.fan"> <context:exclude-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan> <!--Configure data sources C3P0--> <bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"> <property name="driverClass" value="${jdbc.driver}"/> <property name="jdbcUrl" value="${jdbc.url}"/> <property name="user" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </bean> <!--to configure SqlSessionFactory--> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!--Inject database connection pool--> <property name="dataSource" ref="dataSource"/> <!--to configure mybatis Core profile for--> <property name="configLocation" value="classpath:mybatis-config.xml"/> </bean> <!--Configure scan dao Interface package--> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <!--injection SqlSessionFactory --> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/> <property name="basePackage" value="com.fan.dao"/> </bean> <!--stay service Inside injection dao Layer object--> <bean id="bookServiceImpl" class="com.fan.service.BookServiceImpl"> <property name="bookMapper" ref="bookMapper"/> </bean> <!--Open transaction--> <bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"/> </bean> </beans>
Configuration file for mybatis
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <package name="com.fan.entity"/> </typeAliases> <mappers> <mapper resource="com/fan/dao/BookMapper.xml"/> </mappers> </configuration>
(3) spring-mvc.xml configuration file
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd"> <!--Annotation driven--> <mvc:annotation-driven/> <!--Static resource processing--> <mvc:default-servlet-handler/> <!--yes controller Scan layer--> <context:component-scan base-package="com.fan.controller" use-default-filters="false"> <context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan> <!--view resolver --> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="viewClass" value="org.springframework.web.servlet.view.JstlView"/> <property name="prefix" value="/WEB-INF/views/"/> <property name="suffix" value=".jsp"/> </bean> </beans>
(4) applicationContext.xml file configuration
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <import resource="spring-dao.xml"/> <import resource="spring-mvc.xml"/> </beans>
(5) database.properties file configuration (the database should write its own)
jdbc.driver = com.mysql.cj.jdbc.Driver jdbc.url = jdbc:mysql://localhost:3306/ssmbuild?characterEncoding=utf8&useSSL=true jdbc.username=root jdbc.password=123456
At this point, the configuration of spring is completely completed.
The next step is the writing of java code and front-end. I write the more important steps. The others are paste and copy. The overall project creation is as follows:
(1) controller layer code
package com.fan.controller; import com.fan.entity.Books; import com.fan.service.BookService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import java.util.List; @Controller @RequestMapping("/book") public class BooksController { @Autowired @Qualifier("bookServiceImpl") private BookService bookService; @RequestMapping("/allBook") public String list(Model model){ List<Books> books = bookService.queryAllList(); model.addAttribute("list",books); return "allBook"; } // The function of this method is to jump to the page @RequestMapping("/toAddPage") public String toAddPage(){ return "addBook"; } @RequestMapping("/addBook") public String addBook(Books books,Model model){ int i = bookService.addBook(books); if (i>0){ return "redirect:/book/allBook"; }else { model.addAttribute("mess","Add failed"); return "addBook"; } } @RequestMapping("/delete/{bookId}") public String delete(@PathVariable("bookId") int id){ bookService.deleteBookById(id); return "redirect:/book/allBook"; } @RequestMapping("/toUpdatePage") public String toUpdatePage(Model model,int bookID){ Books books = bookService.queryBookById(bookID); model.addAttribute("book",books); return "update"; } @RequestMapping("/update") public String update(Books books){ int i = bookService.updateBook(books); return "redirect:/book/allBook"; } }
(2) dao layer code
package com.fan.dao; import com.fan.entity.Books; import java.util.List; public interface BookMapper { // Add a book int addBook(Books books); // Delete a book by id int deleteBookById(int id); // Update Book int updateBook(Books books); // Returns a Book by ID Books queryBookById(int id); // Query all books List<Books> queryAllList(); }
(3) entity layer code
package com.fan.entity; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @Data @AllArgsConstructor @NoArgsConstructor public class Books { private int bookID; private String bookName; private int bookCount; private String detail; }
(4) Code of service layer
package com.fan.service; import com.fan.entity.Books; import java.util.List; public interface BookService { // Add a book int addBook(Books books); // Delete a book by id int deleteBookById(int id); // Update Book int updateBook(Books books); // Returns a Book by ID Books queryBookById(int id); // Query all books List<Books> queryAllList(); }
package com.fan.service; import com.fan.dao.BookMapper; import com.fan.entity.Books; import java.util.List; public class BookServiceImpl implements BookService { private BookMapper bookMapper; // Function: prepare for later Spring to inject bookMapper public void setBookMapper(BookMapper bookMapper) { this.bookMapper = bookMapper; } @Override public int addBook(Books books) { return bookMapper.addBook(books); } @Override public int deleteBookById(int id) { return bookMapper.deleteBookById(id); } @Override public int updateBook(Books books) { return bookMapper.updateBook(books); } @Override public Books queryBookById(int id) { return bookMapper.queryBookById(id); } @Override public List<Books> queryAllList() { return bookMapper.queryAllList(); } }
An important step: create in the resource (equivalent to the implementation of BookMapper). Remember that it is the file format (hierarchy is required)
In the file box, enter: com/fan/dao/BookMapper.xml (that is, the path format should be the same as that of dao layer)
Here is the sql of mybatis. The code is as follows (Note: namespace should specify its own dao):
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.fan.dao.BookMapper"> <!--// Add a book -- > <insert id="addBook" parameterType="books"> insert into books(bookname,bookcount,detail) values (#{bookName},#{bookCount},#{detail}) </insert> <!-- according to id Delete a book deleteBookById--> <delete id="deleteBookById" parameterType="int"> delete from books where bookid = #{bookID} </delete> <!-- // Update book -- > <update id="updateBook" parameterType="books"> update books set bookname=#{bookName},bookcount=#{bookCount},detail=#{detail} where bookid = #{bookID} </update> <!-- // Returns a book -- > by ID <select id="queryBookById" resultType="books"> select * from books where bookId = #{bookID} </select> <!-- // Returns a book -- > by ID <select id="queryAllList" resultType="books"> select * from books </select> </mapper>
5, Test results
(1) Query function
Click the following button to return the information of all books in the database
The results are as follows:
(2) Add function
Click the Add button to display the following screen.
Success screen:
(3) Delete function (just delete the newly added Books)
Success screen (align with the above figure and click delete):
(4) Modify function
After clicking the modify link, you should enter the following screen (we modify the number of books):
In the success screen, we can see that the number of books has been successfully modified: