Summary: Return data in json, xml format
@XmlRootElement
<dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-xml</artifactId> </dependency>
SpringBoot returns json and xml
https://www.jianshu.com/p/231b5ffa19b7
In some cases, the interface needs to return xml data. In spring boot, it does not need to convert the data format every time, just do some minor adjustments.
Create a new spring boot project and add a dependency on jackson-data format-xml. The pom file code is as follows:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.dalaoyang</groupId> <artifactId>springboot_xml</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>springboot_xml</name> <description>springboot_xml</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.9.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-xml</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Start the class by default, without any adjustments.
Create a new user class with the following code:
package com.dalaoyang.entity; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; /** * @author dalaoyang * @Description * @project springboot_learn * @package com.dalaoyang.entity * @email yangyang@dalaoyang.cn * @date 2018/4/8 */ @XmlRootElement public class User { String userName; String userAge; String userAddress; public User(String userName, String userAge, String userAddress) { this.userName = userName; this.userAge = userAge; this.userAddress = userAddress; } @XmlElement public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } @XmlElement public String getUserAge() { return userAge; } public void setUserAge(String userAge) { this.userAge = userAge; } @XmlElement public String getUserAddress() { return userAddress; } public void setUserAddress(String userAddress) { this.userAddress = userAddress; } }
Finally, controller, code as follows:
package com.dalaoyang.controller; import com.dalaoyang.entity.User; import org.springframework.http.MediaType; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; /** * @author dalaoyang * @Description * @project springboot_learn * @package com.dalaoyang.controller * @email yangyang@dalaoyang.cn * @date 2018/4/8 */ @RestController public class UserController { //http://localhost:8080/json @GetMapping(value = "/json",produces = MediaType.APPLICATION_JSON_VALUE) public User index(){ User user = new User("dalaoyang", "26", "Xie"); return user; } //http://localhost:8080/xml @GetMapping(value = "/xml",produces = MediaType.APPLICATION_XML_VALUE) public User XML(){ User user = new User("dalaoyang", "26", "Xie"); return user; } }
Here you can start the project, visit http://localhost:8080/json You can see the following figure

Visit http://localhost:8080/xml As follows
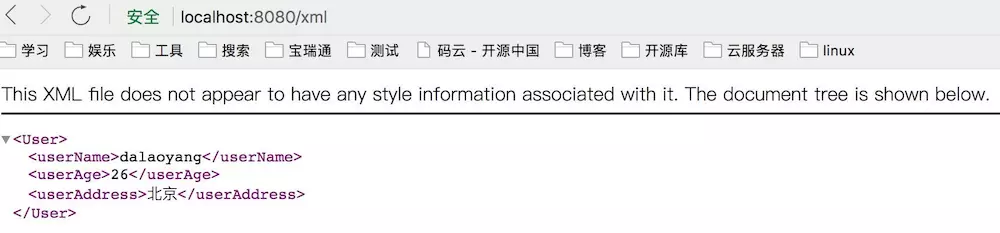
Author: dalaoyang
Link: https://www.jianshu.com/p/231b5ffa19b7
Source: Brief Book
The copyright of the brief book belongs to the author. For any form of reprinting, please contact the author for authorization and indicate the source.