ActiveMQ is an open source messaging system provided by Apache, which is implemented entirely in Java, so it can support JMS (Java Message Service, or Java Message Service) specification very well. This article will introduce in detail the installation of ActiveMq, the integration with SpringBook to send queue messages, and the process of sending topic messages.
Contents of this article
ActiveMQ Installation under Linux1. Download and decompress2. Operation3. Enter the Management InterfaceSending queue messages3. Sending Subject Messages
ActiveMQ Installation under Linux
1. Download and decompress
wget https://mirrors.tuna.tsinghua.edu.cn/apache//activemq/5.15.9/apache-activemq-5.15.9-bin.tar.gz
tar zxvf apache-activemq-5.15.9-bin.tar.gz
2. Operation
cd bin/
./activemq start
3. Enter the Management Interface
The browser accesses 192.168.0.1:8161/admin/. The default user name and password are admin/admin. The console screenshot is as follows:
The information in the list has the following meanings:
-
Number Of Pending Messages: The number of messages waiting for consumption is the number of queues that are not currently queued.
-
Number Of Consumers: Consumers: This is the number of consumers on the consumer side.
-
Messages Enqueued: The total number of messages entering the queue, including those out of the queue.
-
Messages Dequeued: Messages coming out of the queue can be understood as consuming the amount consumed.
Sending queue messages
Queue mode characteristics:
- Client includes producer and consumer.
- One message in the queue can only be used by one consumer.
- Consumers can cancel interest at any time.
The application.properties configuration is as follows:
# Connection Address
spring.activemq.broker-url=tcp://192.168.0.1:61616
# If it is a queue, then the default should be false. If you publish a subscription, it must be set to true.
spring.jms.pub-sub-domain=false
ActivemqConfig.java configuration:
/**
* Point-to-point
*/
@Bean
public Queue queue() {
return new ActiveMQQueue("active.queue");
}
The message producer SendController.java sends the following code:
/*
* Send queue messages
*/
@RequestMapping("/sendQueue")
public String sendQueue() {
String message = UUID.randomUUID().toString();
//Designate the destination and content of the message
this.jmsMessagingTemplate.convertAndSend(this.queue, message);
return "Message sent successfully! message=" + message;
}
Message consumer QueueCustomerController.java sends the code as follows:
@RestController
public class QueueCustomerController {
/*
* Listen and receive queue messages
*/
@JmsListener(destination="active.queue")
public void readActiveQueue(String message) {
System.out.println("Accept:" + message);
}
}
Continuous browser access: http://localhost:8080/sendQueue, message sent successfully, the consumer received the message and printed the following log:
Accepted: 51d85d31-002d-4c9b-87df-a5ea64e8d6da
Accepted: 1c9dab0c-1d47-4556-95dc-601c8add44fe
Accepted: d199ff29-d6ff-41d2-ada0-921d636f7ed1
Accepted: 4d50ba07-a48a-42b6-a67e-805cdeea662c
Accepted: 31fc16a9-8aec-4ee6-bbb3-a0ca22c19686
3. Sending Subject Messages
Thematic pattern features:
- Clients include publishers and subscribers.
- Messages in a topic can be consumed by all subscribers.
- Consumers cannot consume messages sent before subscription.
Modify attributes in application.properties:
spring.jms.pub-sub-domain=true
ActivemqConfig.java configuration:
/**
* Publish/Subscribe
*/
@Bean
public Topic topic() {
return new ActiveMQTopic("active.topic");
}
The message producer SendController.java sends the following code:
/*
* Send Theme Messages
*/
@RequestMapping("/sendTopic")
public String sendTopic() {
String message = UUID.randomUUID().toString();
//Designate the destination and content of the message
this.jmsMessagingTemplate.convertAndSend(this.topic, message);
return "Message sent successfully! message=" + message;
}
Add two message consumers, TopicCustomerController.java code as follows:
/*
* Listening and Receiving Topic Message 1
*/
@JmsListener(destination = "active.topic")
public void readActiveTopic1(String message) {
System.out.println("Customer1 Accept:" + message);
}
/*
* Listening and Receiving Topic Messages 2
*/
@JmsListener(destination = "active.topic")
public void readActiveTopic2(String message) {
System.out.println("Customer2 Accept:" + message);
}
Continuous browser access: http://localhost:8080/sendTopic, message sent successfully, two consumers received the message and printed the following log:
Customer1 Accept:96c674b7-a310-487b-8088-2c5d049cfabf
Customer2 Accept:96c674b7-a310-487b-8088-2c5d049cfabf
Customer1 Accept: fee4fde8-6cbe-4d08-b179-e9462f6f1e74
Customer2 Accept: fee4fde8-6cbe-4d08-b179-e9462f6f1e74
Customer1 Accept:05d79335-ff33-41ec-ba13-a6f5c9d5bba0
Customer2 Accept:05d79335-ff33-41ec-ba13-a6f5c9d5bba0
Customer1 Accept: f8244df3-5504-478b-b86e-77823ab34dac
Customer2 Accept: f8244df3-5504-478b-b86e-77823ab34dac
Recommended reading
1. Coding artifact Lombok, after learning to develop at least twice the efficiency!
2. Two ways of configuring filters in Spring Boot
3.Spring Boot Unified Anomaly Handling Practice
4. From a technical point of view, why not send the original map online?
5. Profile of Spring Boot--Quickly Solve Multi-environment Use and Switching
Timely access to free Java-related information covers Java, Redis, MongoDB, MySQL, Zookeeper, Spring Cloud, Dubbo/Kafka, Hadoop, Hbase, Flink and other high-concurrent distributed, large data, machine learning technology.
Pay attention to the following public numbers for free:
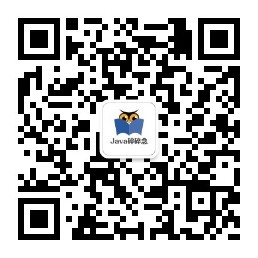