1 create an empty project first (name yourself)
2 create two modules in the empty project created in step 1, and the creation method is as shown in the following figure.
1) create initiator module with maven project my initiator name is Mao spring boot starter
pom.xml As shown below <?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mao.starter</groupId> <artifactId>mao-spring-boot-starter</artifactId> <version>1.0-SNAPSHOT</version> <!-- starter--> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <!-- Introduce automatic configuration module--> <dependency> <groupId>com.mao-starter</groupId> <artifactId>mao-spring-boot-starter-autoconfigurer</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> </dependencies>
</project>
2) create the auto configuration module. My name is Mao spring boot starter autoconfigurer, as shown in the following figure. 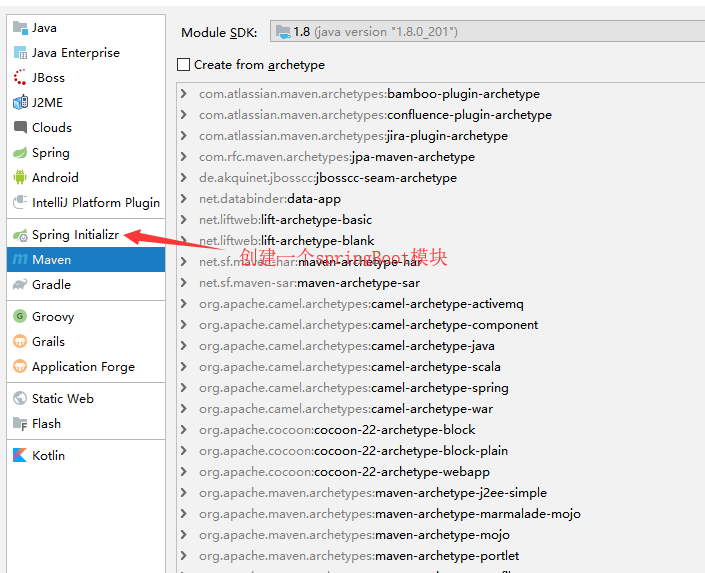 pom.xml looks like this <?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.21.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.mao-starter</groupId> <artifactId>mao-spring-boot-starter-autoconfigurer</artifactId> <version>0.0.1-SNAPSHOT</version> <name>mao-spring-boot-starter-autoconfigurer</name> <description>Demo project for Spring Boot</description> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties>
<! -- all basic configurations to be introduced -- >
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> </dependencies>
</project>
3 create three classes as shown in the following figure
helloProperties.java / / property class
package com.maostarter.mao;
import org.springframework.boot.context.properties.ConfigurationProperties;
@ConfigurationProperties(prefix = "map.hello")
public class helloProperties {
public String prefix; public String getPrefix() { return prefix; } public void setPrefix(String prefix) { this.prefix = prefix; } public String getSuffix() { return suffix; } public void setSuffix(String suffix) { this.suffix = suffix; } private String suffix;
}
HelloService.java / / the class that provides the service to the outside
public class HelloService {
helloProperties helloPropertie; public helloProperties getHelloPropertie() { return helloPropertie; } public void setHelloPropertie(helloProperties helloPropertie) { this.helloPropertie = helloPropertie; } public String sayHelloMao(String name){ return helloPropertie.getPrefix()+'*'+name+helloPropertie.getSuffix(); }
}
helloServiceAutoConfiguration.java / / service auto injection class
@Configuration
@Conditionalonwebapplication / / Web application takes effect
@EnableConfigurationProperties(helloProperties.class)
public class helloServiceAutoConfiguration {
@Autowired helloProperties helloPropertie; @Bean public HelloService helloService(){ HelloService service = new HelloService(); service.setHelloPropertie(helloPropertie); return service; }
}
4. Configure the spring.factories file, as shown in the following figure.
spring.factories
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.maostarter.mao.helloServiceAutoConfiguration
5. How to generate a starter? In this process, there may be various problems. Generally, the jdk version is wrong, and the maven version is wrong. If there are problems, first read the console information carefully.
Don't Baidu as soon as something goes wrong
6 test the custom starter just created and create a springBoot project
pom.xml looks like this
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.6.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.autostart.test</groupId> <artifactId>autostart</artifactId> <version>0.0.1-SNAPSHOT</version> <name>autostart</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>com.mao.starter</groupId> <artifactId>mao-spring-boot-starter</artifactId> <version>1.0-SNAPSHOT</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
</project>
6. Copy the previously configured property file as shown in the figure below.
7 create a test case
@Controller
public class HelloController {
@Autowired HelloService helloService; @ResponseBody @RequestMapping("/hello") public String Hello(){ return helloService.sayHelloMao("haha"); }
}
8 test results are shown in the figure below