I. JPA
1. Creating projects
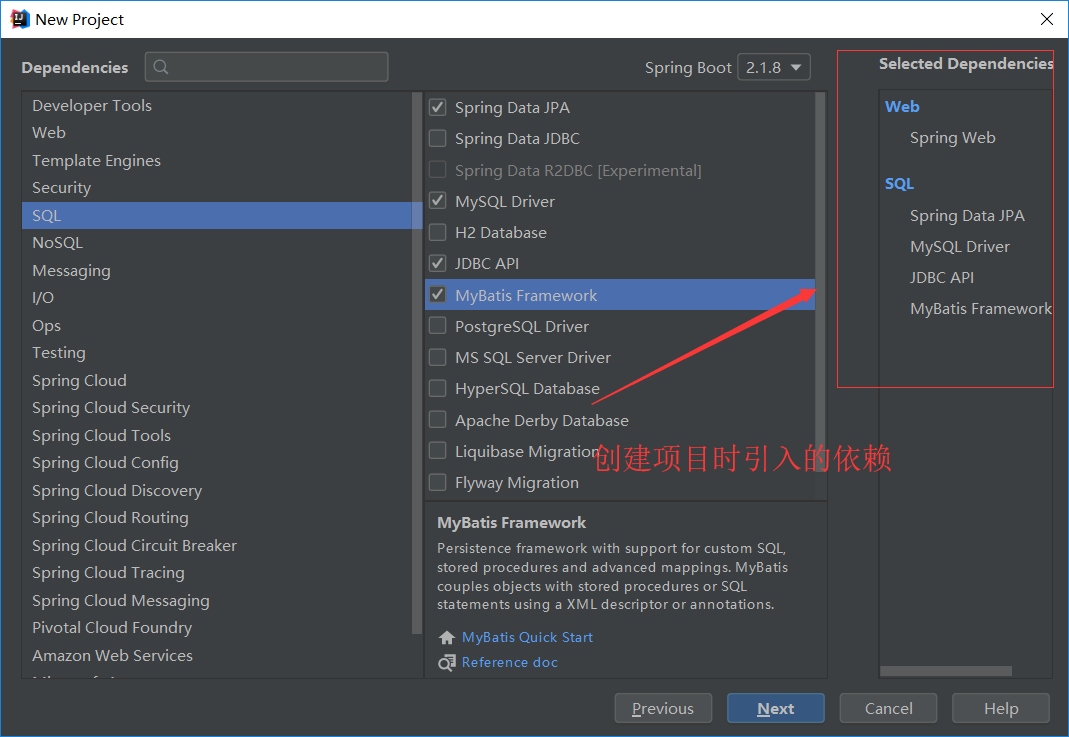
2. Importing Thymeleaf dependencies in pom.xml files
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.8.RELEASE</version>
<relativePath/>
</parent>
<groupId>com.xjj</groupId>
<artifactId>springboot</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
<thymeleaf.version>3.0.11.RELEASE</thymeleaf.version>
<thymeleaf-layout-dialect.version>2.2.2</thymeleaf-layout-dialect.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3. Configure connection database information and JPA, using yaml file writing here
spring:
datasource:
username: root
password: 1234
url: jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=utf-8&serverTimezone=UTC
driver-class-name: com.mysql.cj.jdbc.Driver
jpa:
hibernate:
ddl-auto: update
show-sql: true
4. Creating Entity Classes for Mapping Data Tables
package com.xjj.springboot.entity;
import javax.persistence.*;
@Entity
@Table
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer bookId;
@Column(length = 50)
private String bookName;
@Column
private Double bookPrice;
public Integer getBookId() {
return bookId;
}
public void setBookId(Integer bookId) {
this.bookId = bookId;
}
public String getBookName() {
return bookName;
}
public void setBookName(String bookName) {
this.bookName = bookName;
}
public Double getBookPrice() {
return bookPrice;
}
public void setBookPrice(Double bookPrice) {
this.bookPrice = bookPrice;
}
@Override
public String toString() {
return "Book{" +
"bookId=" + bookId +
", bookName='" + bookName + '\'' +
", bookPrice=" + bookPrice +
'}';
}
}
5. Creating interfaces to inherit JpaRepository
package com.xjj.springboot.dao;
import com.xjj.springboot.entity.Book;
import org.springframework.data.jpa.repository.JpaRepository;
public interface BookRepository extends JpaRepository<Book,Integer> {
}
6. Create service layer and controller, and query all information in book table
package com.xjj.springboot.service;
import com.xjj.springboot.entity.Book;
import java.util.List;
public interface BookService {
List<Book> findAll();
}
package com.xjj.springboot.service.impl;
import com.xjj.springboot.dao.BookRepository;
import com.xjj.springboot.entity.Book;
import com.xjj.springboot.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookServiceImpl implements BookService {
@Autowired
private BookRepository bookRepository;
@Override
public List<Book> findAll(){
return bookRepository.findAll();
}
}
package com.xjj.springboot.controller;
import com.xjj.springboot.entity.Book;
import com.xjj.springboot.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
@Controller
public class BookController {
@Autowired
private BookService bookService;
@RequestMapping("/findAll")
@ResponseBody
public List<Book> findAll(){
return bookService.findAll();
}
}
7. Start-up project
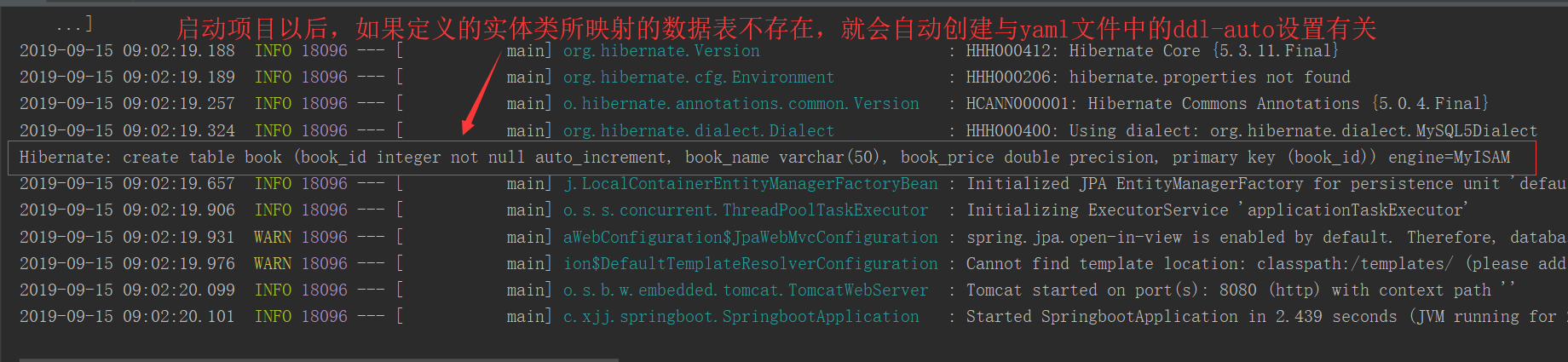
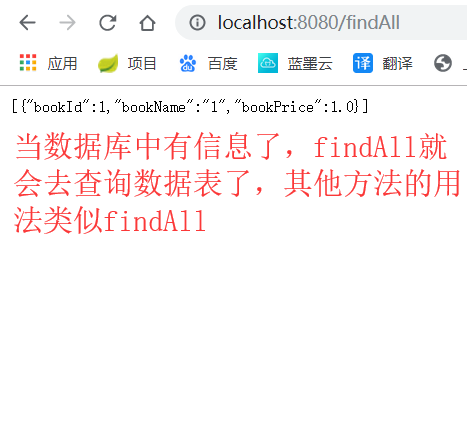
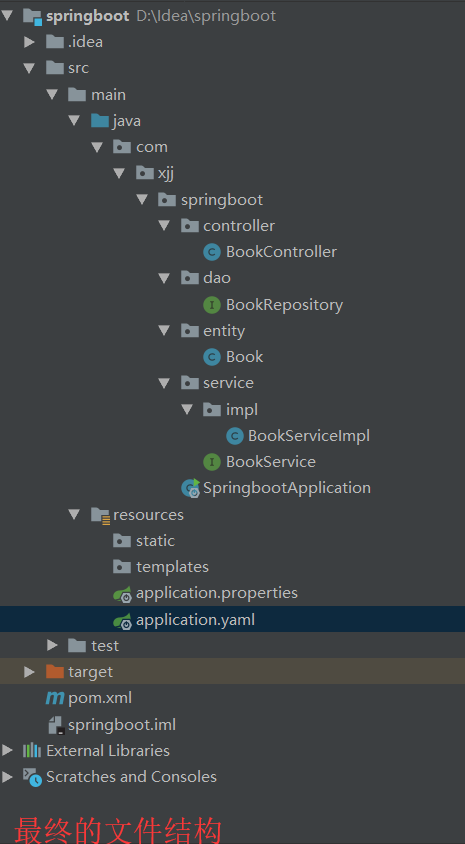
Mybatis - Next, the Jpa above operates to create a method that can query the book table by id
1. Create Mapper's interface file
package com.xjj.springboot.dao;
import com.xjj.springboot.entity.Book;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Component;
@Mapper
@Component
public interface BookMapper {
Book queryBookByBookId(@Param("bookId") Integer bookId);
}
2. Create Mybatis Core Profile
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
</configuration>
3. Create Mapper's mapping file
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.xjj.springboot.dao.BookMapper">
<select id="queryBookByBookId" resultType="com.xjj.springboot.entity.Book">
select * from book
</select>
</mapper>
4. service layer and conotroler layer
package com.xjj.springboot.service;
import com.xjj.springboot.entity.Book;
import java.util.List;
public interface BookService {
List<Book> findAll();
Book queryBookByBookId(Integer bookId);
}
package com.xjj.springboot.service.impl;
import com.xjj.springboot.dao.BookMapper;
import com.xjj.springboot.dao.BookRepository;
import com.xjj.springboot.entity.Book;
import com.xjj.springboot.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookServiceImpl implements BookService {
@Autowired
private BookRepository bookRepository;
@Autowired
private BookMapper bookMapper;
@Override
public List<Book> findAll(){
return bookRepository.findAll();
}
@Override
public Book queryBookByBookId(Integer bookId) {
return bookMapper.queryBookByBookId(bookId);
}
}
package com.xjj.springboot.controller;
import com.xjj.springboot.dao.BookMapper;
import com.xjj.springboot.entity.Book;
import com.xjj.springboot.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
@Controller
public class BookController {
@Autowired
private BookService bookService;
@RequestMapping("/findAll")
@ResponseBody
public List<Book> findAll(){
return bookService.findAll();
}
@RequestMapping("/queryBookByBookId/{bookId}")
@ResponseBody
public Book queryBookByBookId(@PathVariable Integer bookId){
return bookService.queryBookByBookId(bookId);
}
}
5. Modify yaml file configuration
spring:
datasource:
username: root
password: 1234
url: jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=utf-8&serverTimezone=UTC
driver-class-name: com.mysql.cj.jdbc.Driver
jpa:
hibernate:
ddl-auto: update
show-sql: true
mybatis:
config-location: classpath:mybatis/config/mybatis-config.xml
mapper-locations: classpath:mybatis/mapper/BookMapper.xml
6. Start-up project
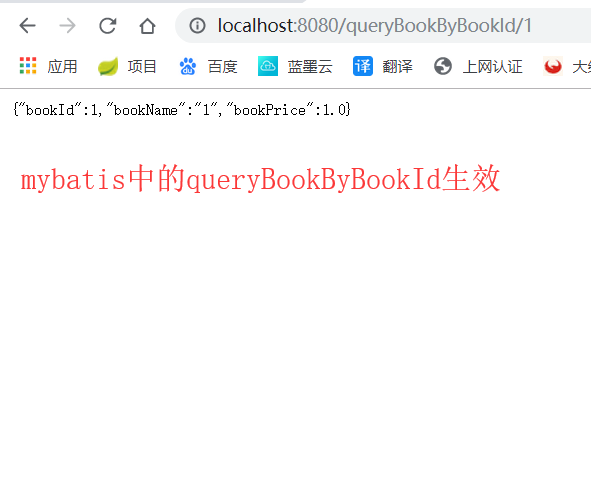
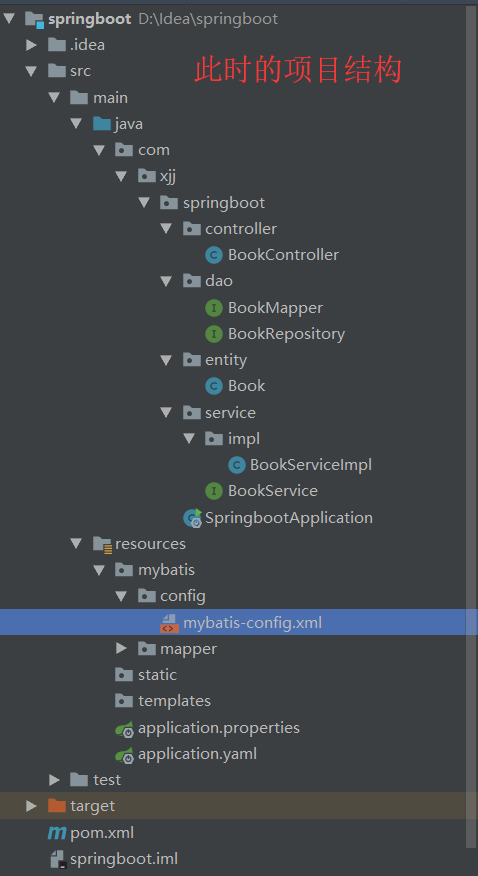
3. Druid - Next to the above file
1. Introducing Druid and log4j dependencies
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.16</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
2. Injecting attributes into Druid and modifying yaml files
spring:
datasource:
username: root
password: 1234
url: jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=utf-8&serverTimezone=UTC
driver-class-name: com.mysql.cj.jdbc.Driver
type: com.alibaba.druid.pool.DruidDataSource
initialSize: 5
minIdle: 5
maxActive: 20
maxWait: 60000
timeBetweenEvictionRunsMillis: 60000
minEvictableIdleTimeMillis: 300000
validationQuery: SELECT 1 FROM DUAL
testWhileIdel: true
testOnBorrow: false
testOnReturn: false
poolPreparedStatements: true
filters: stat,wall,log4j
maxPoolPreparedStatementPerConnectionSize: 20
connectionProperties: druid:stat.mergeSql=true;druid.stat.slowSqlMills=500
jpa:
hibernate:
ddl-auto: update
show-sql: true
mybatis:
config-location: classpath:mybatis/config/mybatis-config.xml
mapper-locations: classpath:mybatis/mapper/BookMapper.xml
3. Creating Druid Configuration Files
package com.xjj.springboot.config;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.support.http.StatViewServlet;
import com.alibaba.druid.support.http.WebStatFilter;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
@Configuration
public class DruidConfig {
@ConfigurationProperties(prefix = "spring.datasource")
@Bean
public DataSource druid(){
return new DruidDataSource();
}
@Bean
public ServletRegistrationBean statViewServlet(){
ServletRegistrationBean bean = new ServletRegistrationBean(new StatViewServlet(), "/druid/*");
Map<String,String> initParams=new HashMap<>();
initParams.put("loginUsername","admin");
initParams.put("loginPassword","admin");
initParams.put("allow","localhost");
bean.setInitParameters(initParams);
return bean;
}
@Bean
public FilterRegistrationBean webStatFilter(){
FilterRegistrationBean bean=new FilterRegistrationBean();
bean.setFilter(new WebStatFilter());
Map<String,String> map=new HashMap<>();
map.put("exclusions","*.js,*.css,/druid/*");
bean.setInitParameters(map);
bean.setUrlPatterns(Arrays.asList("/*"));
return bean;
}
}
4. Start-up project
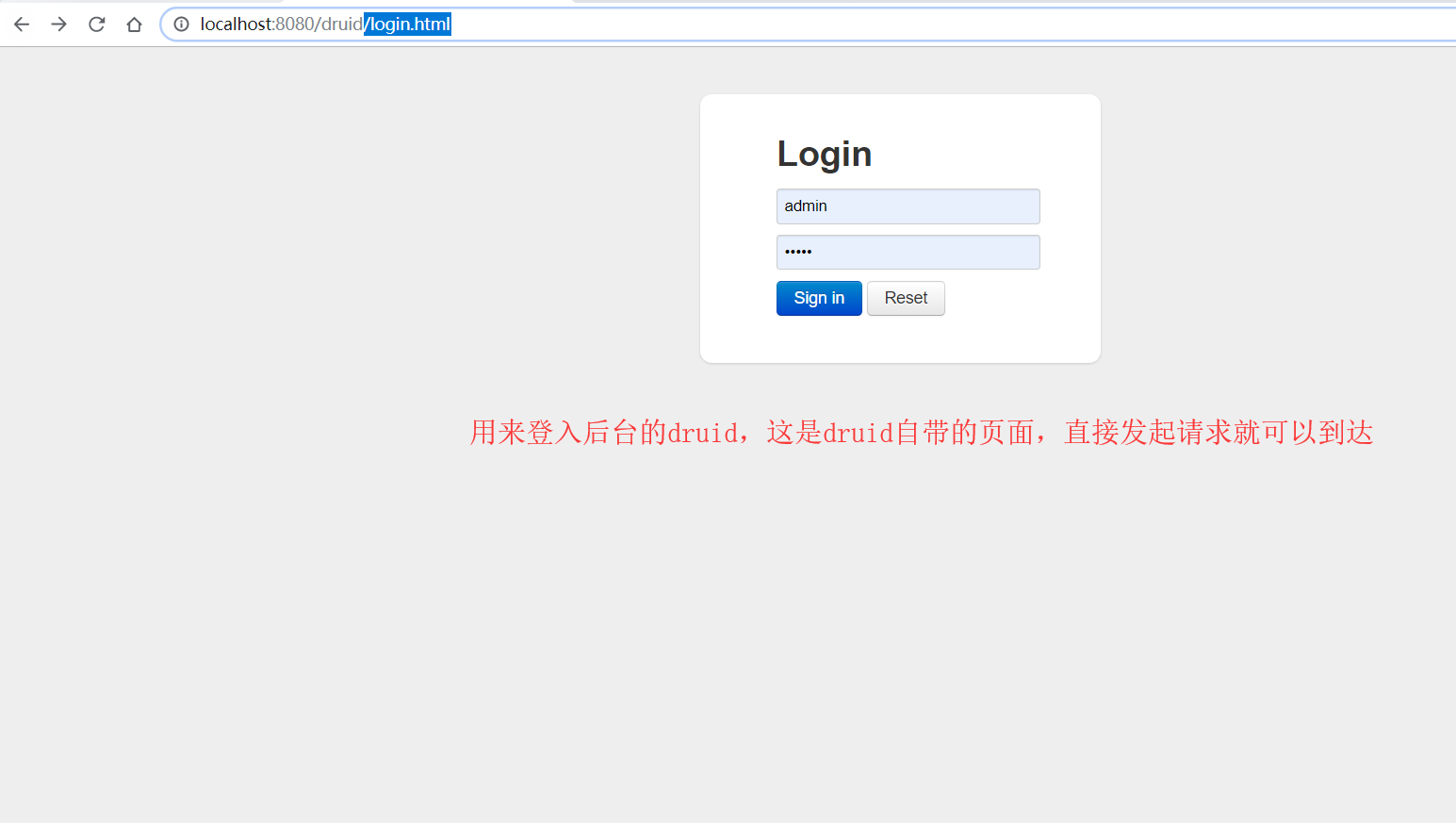

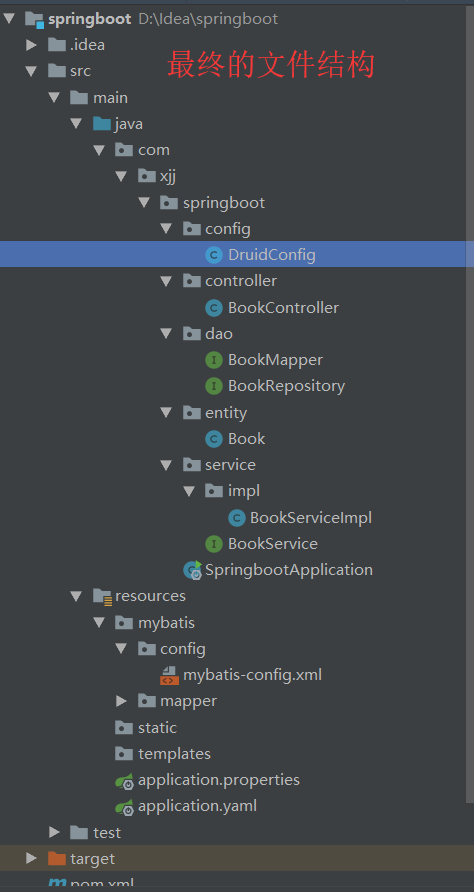