I. Project structure
1. Project structure:
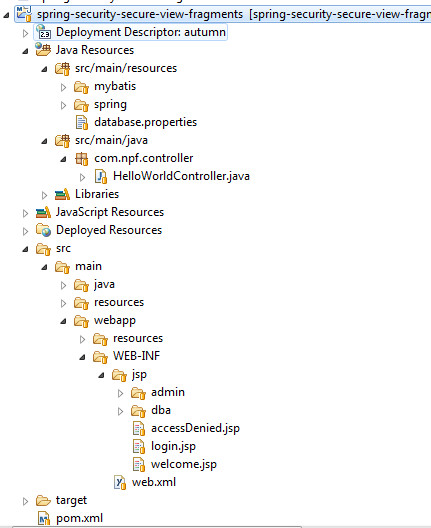
2.
The pom.xml file is as follows:
3. The spring-security.xml file is as follows:<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.npf</groupId> <artifactId>spring-security-secure-view-fragments</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>spring-security-secure-view-fragments Maven Webapp</name> <url>http://maven.apache.org</url> <properties> <spring.version>4.1.6.RELEASE</spring.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.10</version> <scope>test</scope> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.10</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> <version>1.2</version> </dependency> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>1.3.0</version> </dependency> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.4.0</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.30</version> </dependency> <dependency> <groupId>commons-dbcp</groupId> <artifactId>commons-dbcp</artifactId> <version>1.4</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.5</version> </dependency> <dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.3.2</version> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-web</artifactId> <version>4.0.1.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-config</artifactId> <version>4.0.1.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-taglibs</artifactId> <version>4.0.1.RELEASE</version> </dependency> </dependencies> <profiles> <profile> <id>jdk-1.7</id> <activation> <activeByDefault>true</activeByDefault> <jdk>1.7</jdk> </activation> <properties> <maven.compiler.source>1.7</maven.compiler.source> <maven.compiler.target>1.7</maven.compiler.target> <maven.compiler.compilerVersion>1.7</maven.compiler.compilerVersion> </properties> </profile> </profiles> <build> <finalName>spring-security-secure-view-fragments</finalName> </build> </project>
4. Add the following configuration to the web.xml file:<?xml version="1.0" encoding="UTF-8"?> <beans:beans xmlns="http://www.springframework.org/schema/security" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd http://www.springframework.org/schema/security http://www.springframework.org/schema/security/spring-security-4.0.xsd"> <beans:bean id="securityContextLogoutHandle" class="org.springframework.security.web.authentication.logout.SecurityContextLogoutHandler"/> <http auto-config="true" use-expressions="true"> <intercept-url pattern="/" access="hasRole('ADMIN') or hasRole('DBA') or hasRole('USER')" /> <intercept-url pattern="/home" access="hasRole('ADMIN') or hasRole('DBA') or hasRole('USER')" /> <intercept-url pattern="/admin/**" access="hasRole('ADMIN')" /> <intercept-url pattern="/dba/**" access="hasRole('ADMIN') and hasRole('DBA')" /> <access-denied-handler error-page="/accessDenied"/> <form-login login-page="/login" username-parameter="ssoId" password-parameter="password" login-processing-url="/login" authentication-failure-url="/authenticationFailure"/> </http> <authentication-manager > <authentication-provider> <user-service> <user name="jack" password="jack123" authorities="ROLE_USER" /> <user name="admin" password="admin123" authorities="ROLE_ADMIN" /> <user name="dbaOnly" password="dba123" authorities="ROLE_DBA" /> <user name="dba" password="dba123" authorities="ROLE_ADMIN,ROLE_DBA" /> </user-service> </authentication-provider> </authentication-manager> </beans:beans>
5. HelloWorldController:<filter> <filter-name>springSecurityFilterChain</filter-name> <filter-class>org.springframework.web.filter.DelegatingFilterProxy</filter-class> </filter> <filter-mapping> <filter-name>springSecurityFilterChain</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
package com.npf.controller; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.core.Authentication; import org.springframework.security.core.context.SecurityContextHolder; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.web.authentication.logout.SecurityContextLogoutHandler; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller public class HelloWorldController { @Autowired private SecurityContextLogoutHandler securityContextLogoutHandle; @RequestMapping(value = {"/home","/"}, method = RequestMethod.GET) public String homePage(ModelMap model) { Object principal = SecurityContextHolder.getContext().getAuthentication().getPrincipal(); String userName = principal instanceof UserDetails ? ((UserDetails) principal) .getUsername() : principal.toString(); model.addAttribute("user", userName); return "welcome"; } @RequestMapping(value = "/admin/index", method = RequestMethod.GET) public String adminPage(ModelMap model) { Object principal = SecurityContextHolder.getContext().getAuthentication().getPrincipal(); String userName = principal instanceof UserDetails ? ((UserDetails) principal) .getUsername() : principal.toString(); model.addAttribute("user", userName); return "admin/index"; } @RequestMapping(value = "/dba/index", method = RequestMethod.GET) public String dbaPage(ModelMap model) { Object principal = SecurityContextHolder.getContext().getAuthentication().getPrincipal(); String userName = principal instanceof UserDetails ? ((UserDetails) principal) .getUsername() : principal.toString(); model.addAttribute("user", userName); return "dba/index"; } @RequestMapping(value = "/login", method = RequestMethod.GET) public String loginPage() { return "login"; } @RequestMapping(value = "/logout", method = RequestMethod.GET) public String logoutPage(HttpServletRequest request,HttpServletResponse response) { Authentication auth = SecurityContextHolder.getContext().getAuthentication(); if (auth != null) { securityContextLogoutHandle.logout(request, response, auth); } return "redirect:/home"; } @RequestMapping(value = "/accessDenied", method = RequestMethod.GET) public String accessDeniedPage(ModelMap model) { Object principal = SecurityContextHolder.getContext().getAuthentication().getPrincipal(); String userName = principal instanceof UserDetails ? ((UserDetails) principal) .getUsername() : principal.toString(); model.addAttribute("user", userName); return "accessDenied"; } @RequestMapping(value = "/authenticationFailure", method = RequestMethod.GET) public String authenticationFailure(HttpServletRequest request){ request.setAttribute("authenticationFailureResult", "failure"); return "login"; } }
Two, test
1. Visit home page: http://localhost:8080/spring-security-secure-view-fragments/Because there is no access to the home page, it is directed to the login page:
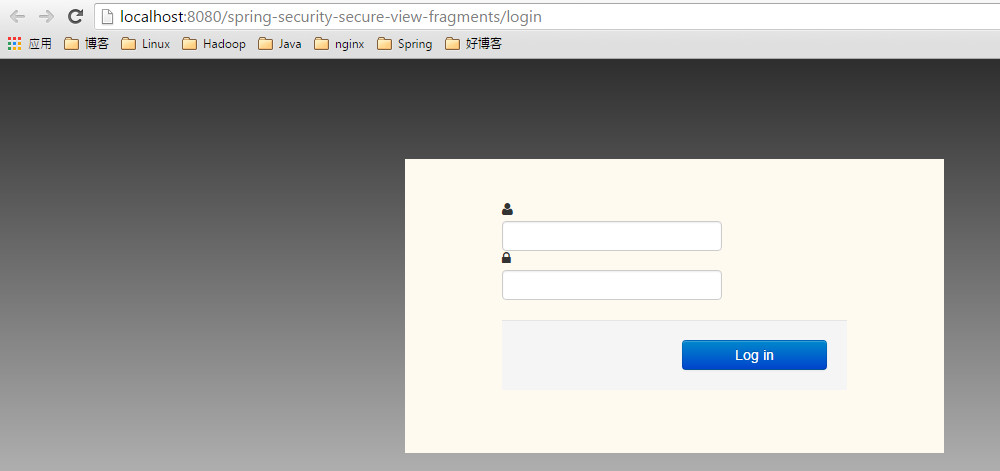
2. The test has "USER" permission:
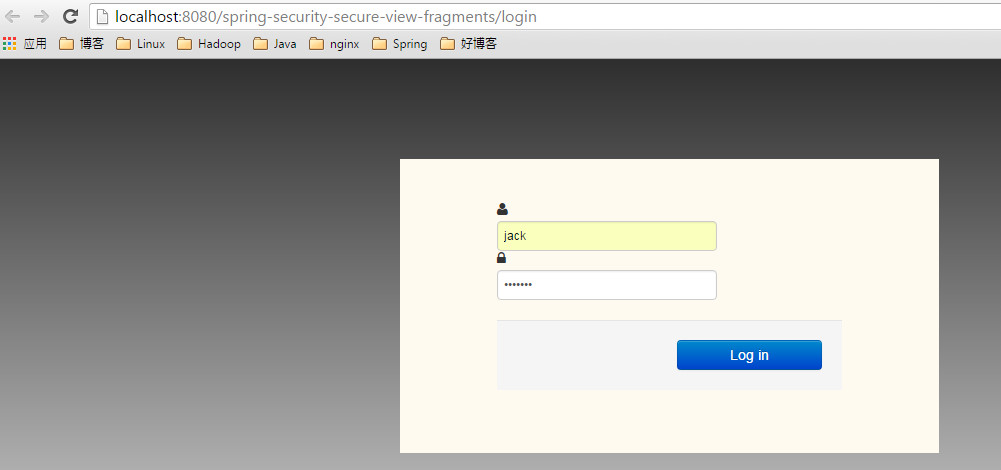
After successful login, you will see:
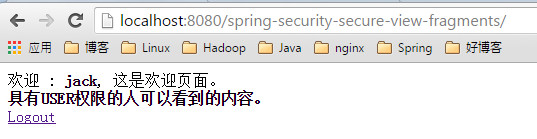
3. Exit, test has "ADMIN" authority:
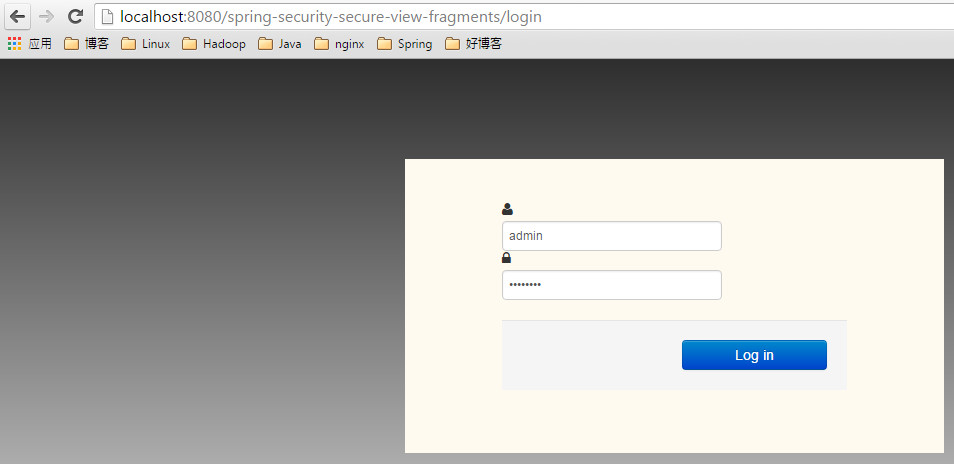
After successful login, you will see:
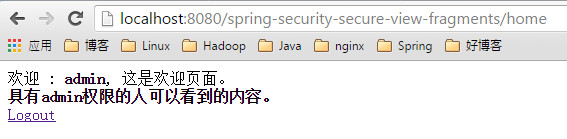
4. Exit, test has "DBA" permission:
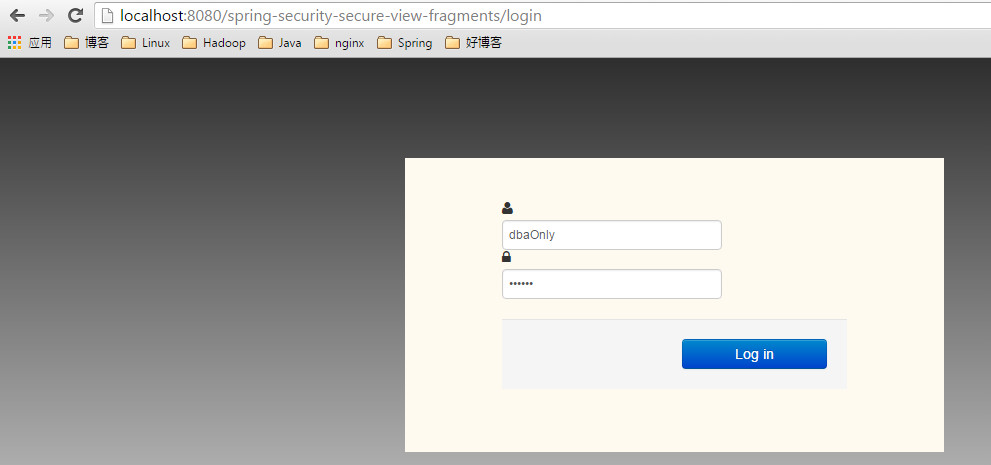
After successful login, you will see:
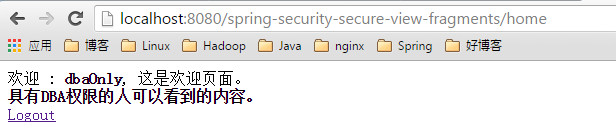
5. Exit, test has "DBA"
And "ADMIN" permissions:
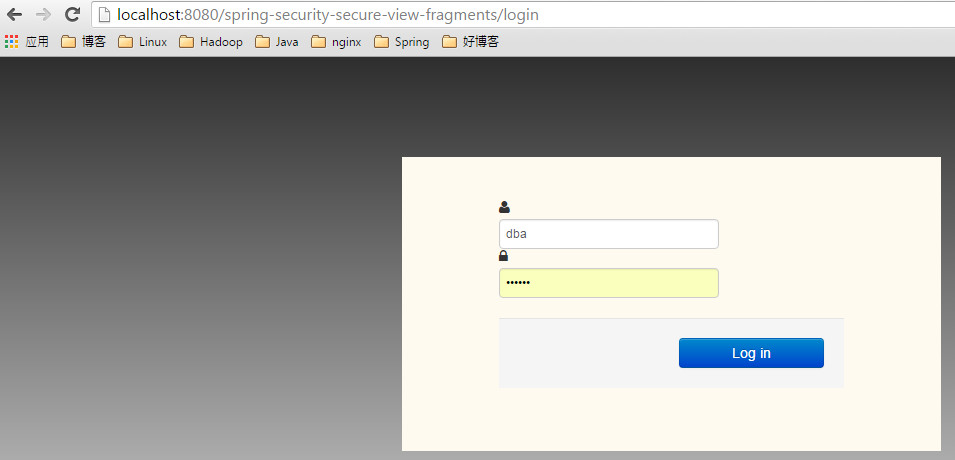
After successful login, you will see:
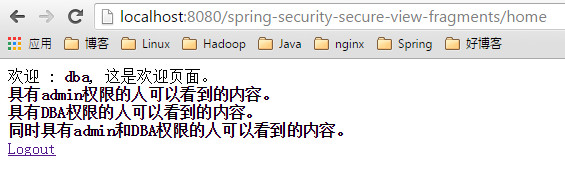
The source code address of the project: https://github.com/spring-security/spring-security-secure-view-fragments
Reference: