Spring MVC uses ajax to download files
1, Explain the conclusion
1. ajax is unable to download files, because: ajax request is just a "character" request, that is, the content of the request is stored in a text type. The file is downloaded in binary form. Although the returned response can be read, it is only read and cannot be executed. ( Quoted here )
2, File download background code
@RequestMapping(value="/downloadFile") public String downloads(HttpServletResponse response) throws Exception{ String path = "C:/"; String fileName = "default.png"; File file = new File(path,fileName); response.reset(); response.setCharacterEncoding("UTF-8"); response.setContentType("multipart/form-data"); response.setHeader("Content-Disposition", "attachment;fileName="+URLEncoder.encode(fileName, "UTF-8")); InputStream input=new FileInputStream(file); OutputStream out = response.getOutputStream(); byte[] buff =new byte[1024]; int index=0; while((index= input.read(buff))!= -1){ out.write(buff, 0, index); out.flush(); } out.close(); input.close(); return null; }
3, Foreground code
1. html code
< button id = "download" > click download file < / button >
2. Using ajax to try to download files
<script type="text/javascript"> $("#download").click(function(){ var url="/downloads/ajax/file";//Download File url $.ajax({ url:url, type:'post', data:{}, success:function(data){ console.info(data,typeof data); } }); }); </script>
3. Result: there is no file download, as shown in the following figure:
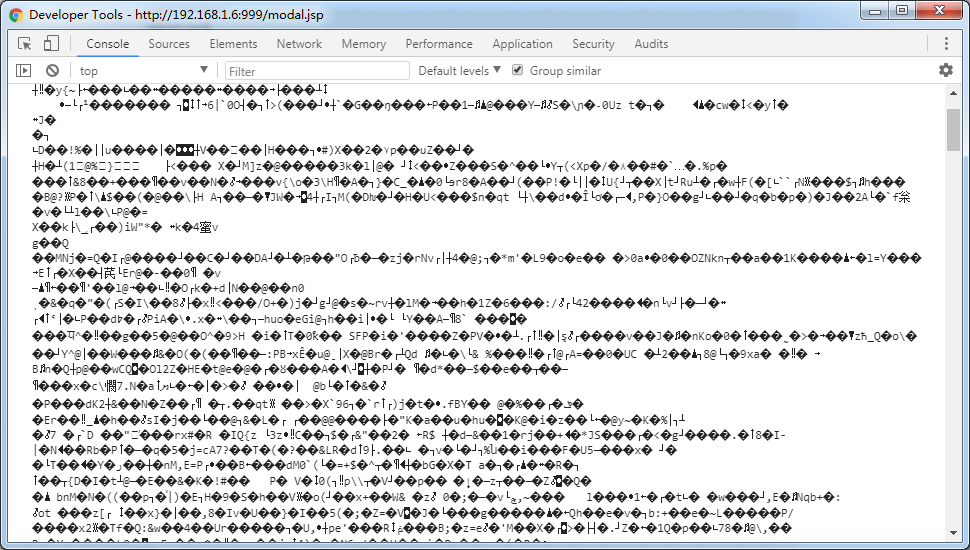
4, Solutions
1. Disadvantages: only get requests are supported.
<script type="text/javascript"> $("#download").click(function(){ var url="/downloads/ajax/file";//Download File url window.location.href=url; }); </script>
2. Use hidden form
a. html code
<form action="" id="fileForm" method="post" style="display: none;"></form>
b. js code
<script type="text/javascript"> $("#download").click(function(){ var url="/downloads/ajax/file";//Download File url $("#fileForm").attr('action',url); $("#fileForm").submit(); }); </script>