I. create the spring boot project
1.File->New->Project
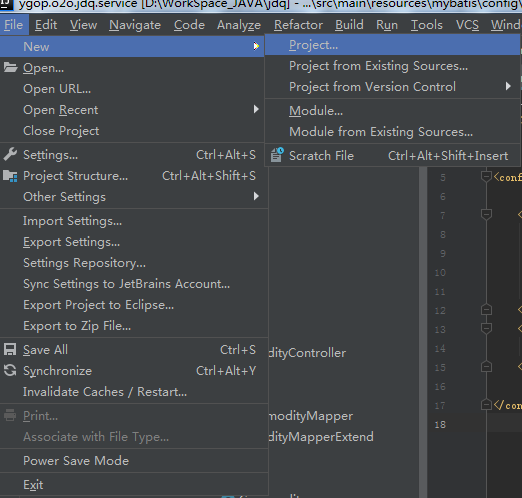
2. Select Spring Initializr, then select the default url and click [Next]:
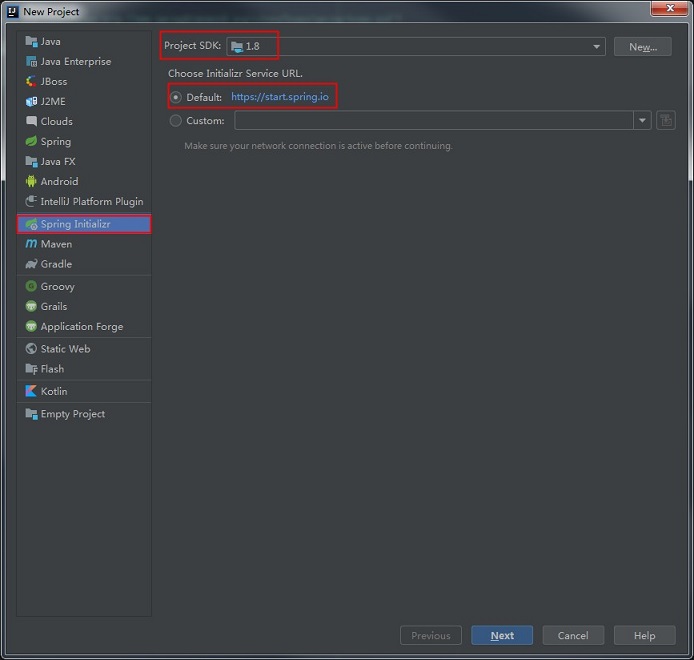
3. Modify project information
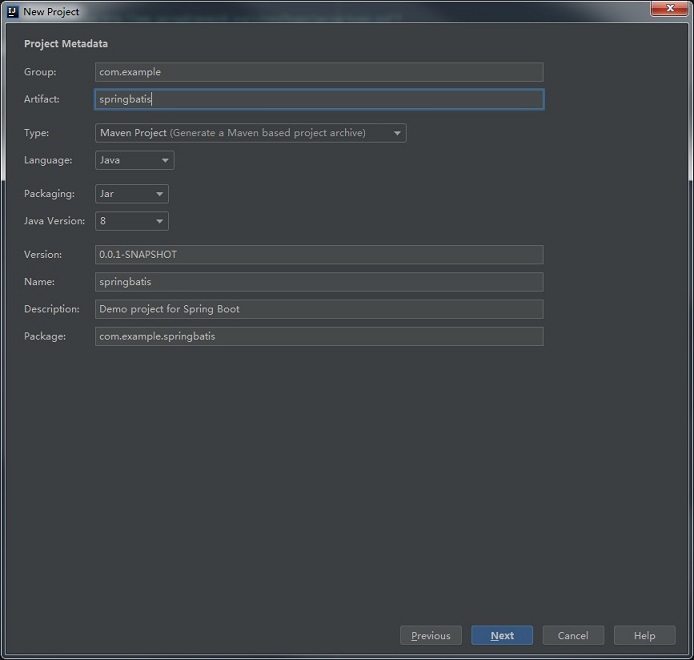
4. Select the template of the project
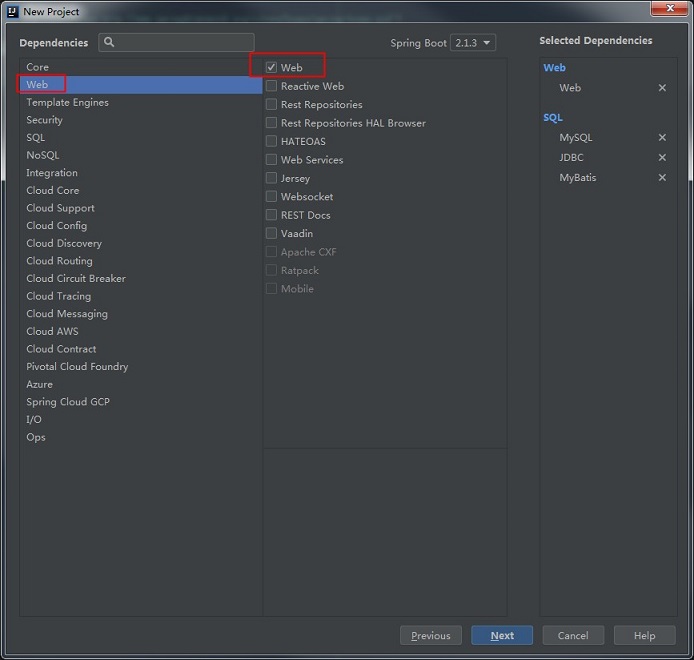
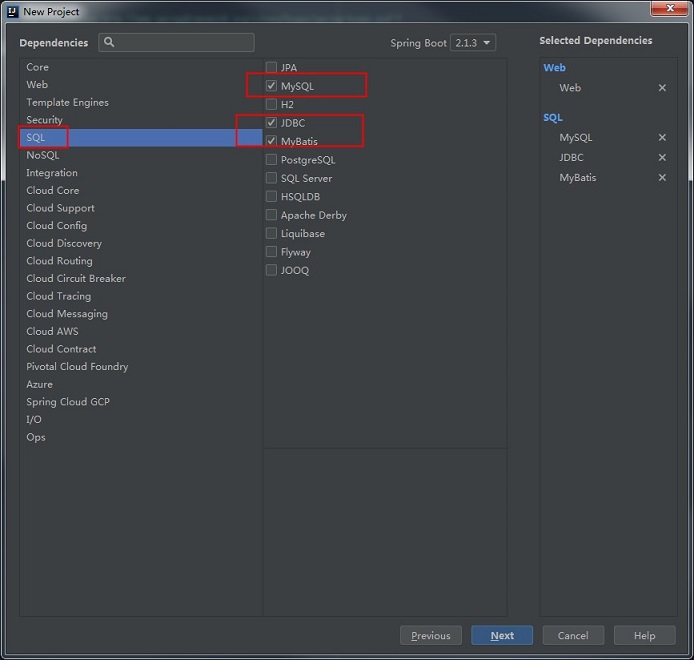
5. Fill in Project name and project location, and then click Finish.
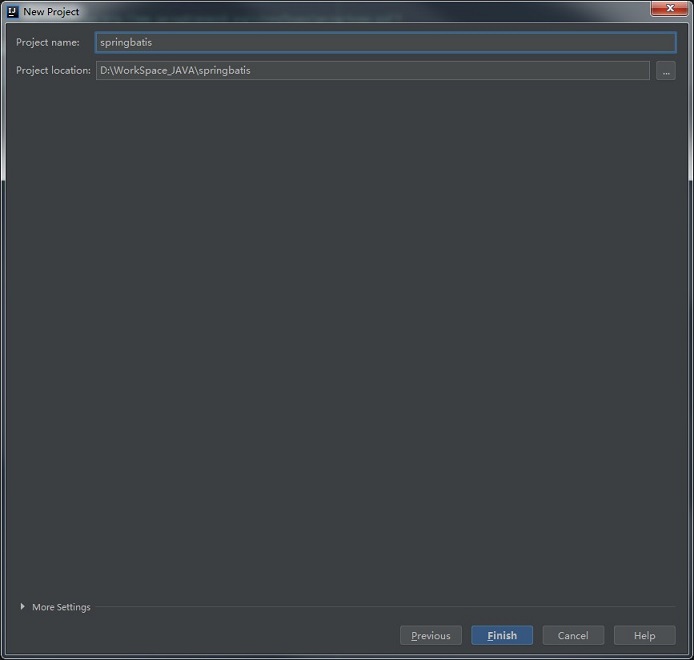
Click to complete the code structure of production:
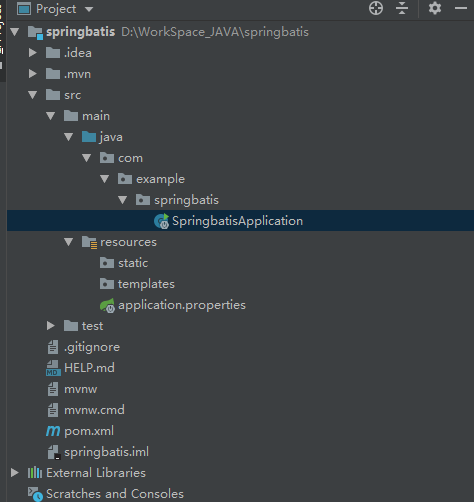
II. Configure pom file
Because you need to integrate Mybatis insertion, you need to add dependency package in pom.xml
So the content of the final pom.xml file is as follows:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.2.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>ygop.o2o.jdq</groupId> <artifactId>service</artifactId> <version>1.0.1</version> <name>service</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.0.0</version> </dependency> <!--Database connection pool--> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.1.11</version> </dependency> <!-- Spring Boot Mybatis rely on --> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.0.0</version> </dependency> <!--Mybatis paging--> <!-- <dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper</artifactId> <version>5.1.2</version> </dependency>--> <!--Mybatis generator Automatically generate dependent files--> <dependency> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-core</artifactId> <version>1.3.5</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <!-- mybatis generator Auto generate code plug-in --> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <configuration> <configurationFile>${basedir}/src/main/resources/generator/generatorConfig.xml</configurationFile> <overwrite>true</overwrite> <verbose>true</verbose> </configuration> </plugin> </plugins> </build> </project>
among
<!-- mybatis generator Auto generate code plug-in --> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <configuration> <configurationFile>${basedir}/src/main/resources/generator/generatorConfig.xml</configurationFile> <overwrite>true</overwrite> <verbose>true</verbose> </configuration> </plugin>
Is the address where mybatis generator automatically generates the code plug-in configuration file;
3. Configure mybatis
1. Add mybatis generator to automatically generate code plug-in configuration file
Add the generator folder under the resources file and create the generatorConfig.xml file
The contents are as follows:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration> <!-- Database driven:Select the database driver package on your local hard disk--> <classPathEntry location="D:\mysql-connector-java-5.1.46.jar"/> <context id="DB2Tables" targetRuntime="MyBatis3"> <commentGenerator> <property name="suppressDate" value="true"/> <!-- Remove automatically generated comments or not true: Yes, false:no --> <property name="suppressAllComments" value="true"/> </commentGenerator> <!--Database links URL,User name, password --> <jdbcConnection driverClass="com.mysql.jdbc.Driver" connectionURL="jdbc:mysql://localhost:3306/ygop_eps_commodity" userId="test" password="test"> </jdbcConnection> <javaTypeResolver> <property name="forceBigDecimals" value="false"/> </javaTypeResolver> <!-- Package name and location of the build model--> <javaModelGenerator targetPackage="com.example.springbatis.model.entities" targetProject="src/main/java"> <property name="enableSubPackages" value="true"/> <property name="trimStrings" value="true"/> </javaModelGenerator> <!-- Package name and location of the build mapping file--> <sqlMapGenerator targetPackage="mybatis/mapper/auto" targetProject="src/main/resources"> <property name="enableSubPackages" value="true"/> </sqlMapGenerator> <!-- generate DAO Package name and location for--> <javaClientGenerator type="XMLMAPPER" targetPackage="com.example.springbatis.dto.auto" targetProject="src/main/java"> <property name="enableSubPackages" value="true"/> </javaClientGenerator> <!-- Table to generate tableName Is the table or view name in the database domainObjectName Is the entity class name--> <table tableName="commodity" domainObjectName="Commodity" enableCountByExample="false" enableUpdateByExample="false" enableDeleteByExample="false" enableSelectByExample="false" selectByExampleQueryId="false"></table> </context> </generatorConfiguration>
IV. configure application.yml
server: port: 8089 spring: datasource: mame: type: com.alibaba.druid.pool.DruidDataSource url: jdbc:mysql://localhost:3306/ygop_eps_commodity username: test password: test driver-class-name: com.mysql.jdbc.Driver filters: stat maxActive: 20 initialSize: 1 maxWait: 60000 minIdle: 1 timeBetweenEvictionRunsMillis: 60000 minEvictableIdleTimeMillis: 300000 validationQuery: select 'x' testWhileIdle: true testOnBorrow: false testOnReturn: false poolPreparedStatements: true maxOpenPreparedStatements: 20 #Mybatis configuration mybatis: mapper-locations: classpath:mybatis/mapper/**/*.xml type-aliases-package: ygop.o2o.jdq.service.model.entities
V: set build to generate code automatically
1.Run->Edit Configurations
Click to add+
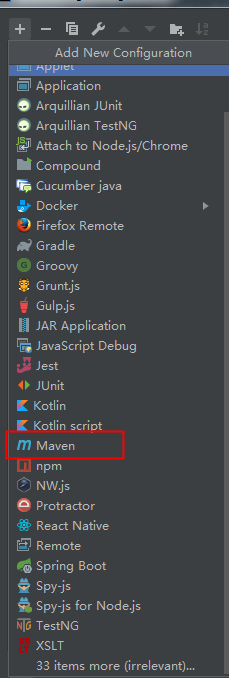
Then configure mybatis generator information
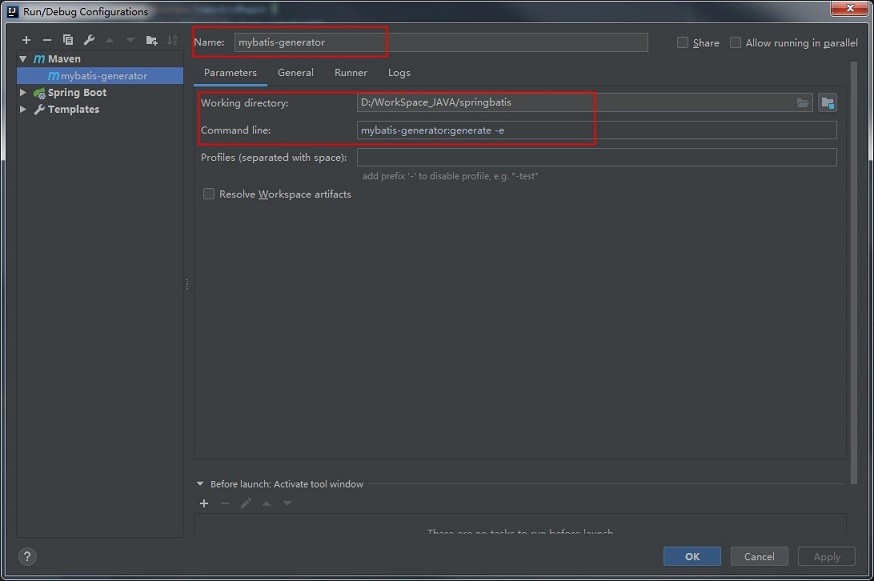
Shop owners Apply and OK will finally have one in the upper right corner of the main interface
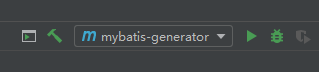
2. Generate code automatically
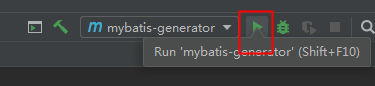
Generate code structure chart:
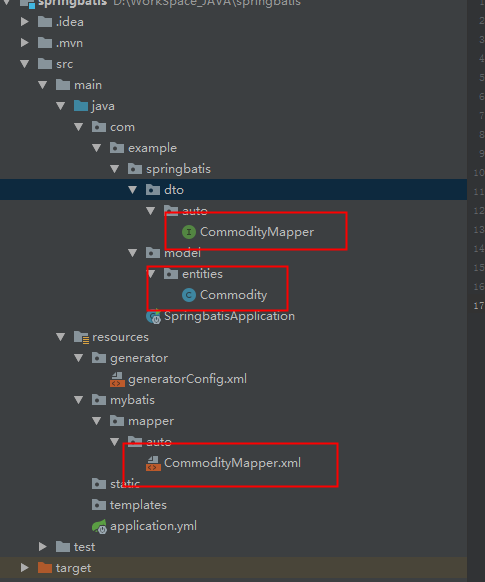
By now, the spring boot+mybatis construction project has been completed;
Vi. operation procedure
1. Add notes
Add annotation to SpringbatisApplication
Inject mapper into Spring
@MapperScan("com.example.springbatis.dto")
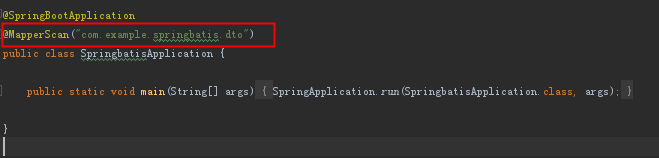
2. Create Controller
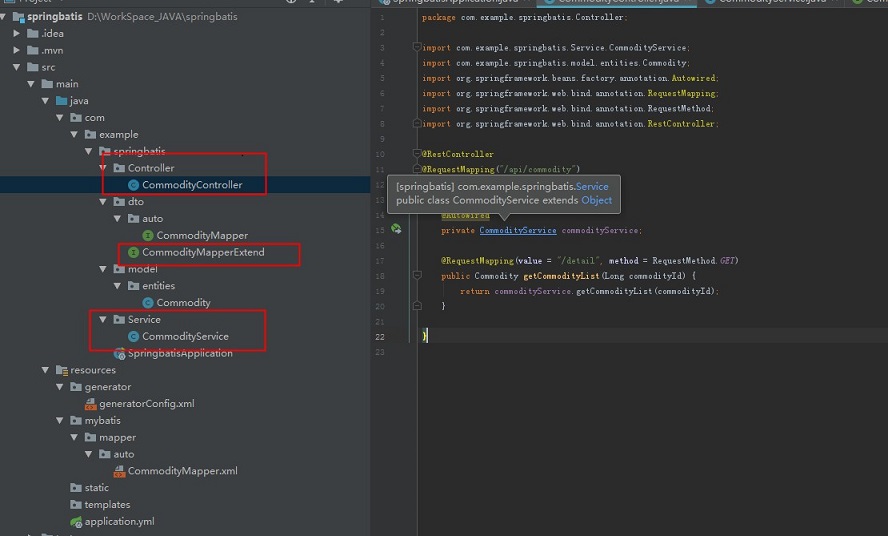
3. operation:
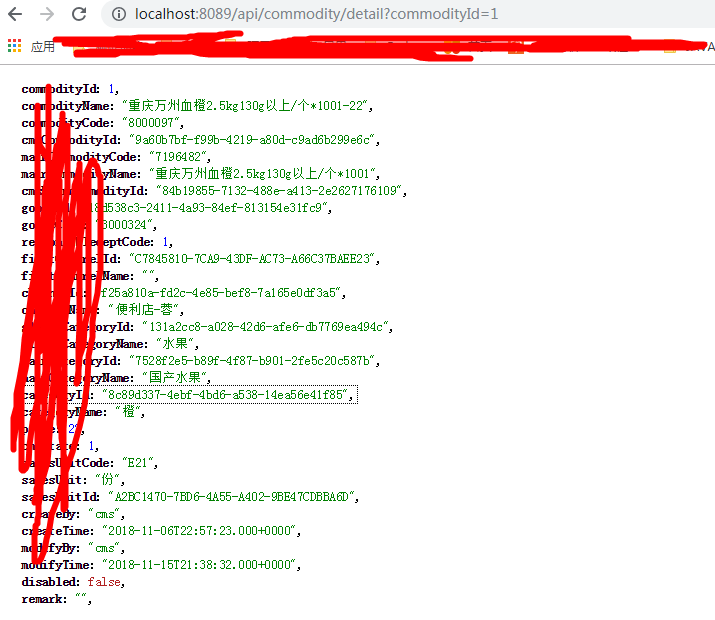