Interface Document Problems in Development
In project development, it is generally divided into front-end UI development and back-end interface development. The front-end obtains data by calling the RESTful API interface of the back-end and then displays it. So UI development engineers need to know what the back-end interface looks like, such as how many URL s are, what input and output parameters are, and what the specific data format is. Often back-end development engineers need to provide an interface document, which often takes a lot of time to write the document, and once the interface changes, the interface document also needs to be maintained together, which consumes a lot of time. So how to solve this problem? It's SWAGGER that we talked about today.
What is Swagger2
Borrowing the definition of Swagger 2:
Swagger2 is a RESTFUL interface document online automatic generation + functional testing software. Swagger is a normative and complete framework for generating, describing, invoking and visualizing RESTful-style Web services. The overall goal is to update both the client and the file system as servers at the same speed. File methods, parameters and models are tightly integrated into server-side code, allowing APIs to keep synchronization at all times. Swagger2 has never been so simple to deploy, manage and use powerful APIs.
Swagger2's official website: https://swagger.io/getting-started/
Configuring Swagger2 in Spring Boot
1. Configure Swagger2 related jar in pom. XML
Based on a Spring Book project, see the article: Hello World of Spring Boot
Add the following configuration to pom.xml:
<!--swagger Dependent jar package --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.4.0</version> </dependency> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.4.0</version> </dependency>
2. Configuring Swagger2
The construction class SwaggerConfig serves as Swagger's initialization class. The code is as follows:
package springboot; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.ApiInfoBuilder; import springfox.documentation.builders.PathSelectors; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket; import springfox.documentation.swagger2.annotations.EnableSwagger2; /** * Created by Administrator on 2017/9/27. */ @Configuration @EnableSwagger2 public class SwaggerConfig { @Bean public Docket createRestApi() { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() .apis(RequestHandlerSelectors.basePackage("springboot.controller")) .paths(PathSelectors.any()) .build(); } private ApiInfo apiInfo() { return new ApiInfoBuilder() .title("Spring Boot Use in Swagger2 structure RESTful APIs") .description("Spring Boot REST API with Swagger") .termsOfServiceUrl("http://blog.didispace.com/") .contact(new Contact("Li Chengbang", "", "robin_lcb@sina.com")) .license("Apache License Version 2.0") .licenseUrl("http://www.apache.org/licenses/LICENSE-2.0.html") .version("1.0") .build(); } }
The springboot.controller in the basePackage("springboot.controller") is the package path that needs to be annotated to generate the document.
3. Write api documents by annotations in controller
The code is as follows:
@RestController @Api(tags = "swagger test", description = "Main display swagger") public class HelloWorldController { @ApiOperation(value="sayHello-test", notes="test swagger") @RequestMapping(value="/",method = RequestMethod.GET) public String sayHello() { return "Hello,World!"; } }
The main comment is @ApiOperation
4. Running App to See the Effect
Open after running App: http://localhost:8080/swagger-ui.html See the following page for successful integration.
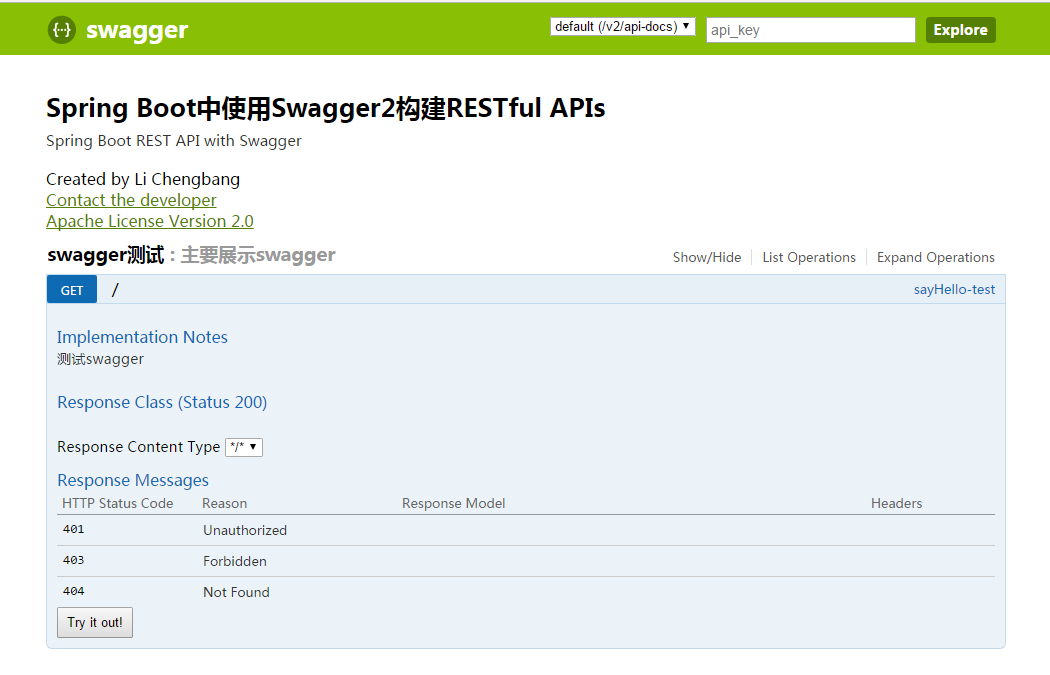
See github for specific code: https://github.com/liuahang/spring-boot-helloworld The swagger branch.